Certainly! Here's a concise explanation along with a code snippet for your topic:
To automate software installation using PowerShell, you can create a script that calls the installation executable with the required parameters.
Start-Process "C:\Path\To\SoftwareInstaller.exe" -ArgumentList "/SILENT" -Wait
Understanding PowerShell Commands
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework from Microsoft, consisting of a command-line shell and associated scripting language. Unlike the traditional Command Prompt, PowerShell is built on .NET and offers rich capabilities for complex scripting and automation tasks.
Why Use PowerShell for Software Installation?
Using PowerShell scripts for software installation provides numerous benefits:
- Efficiency: Automating the installation process can drastically reduce the time required to install multiple applications, especially in enterprise environments.
- Reusability: Once you create a script, you can reuse it across various systems or projects, ensuring consistency and reducing errors.
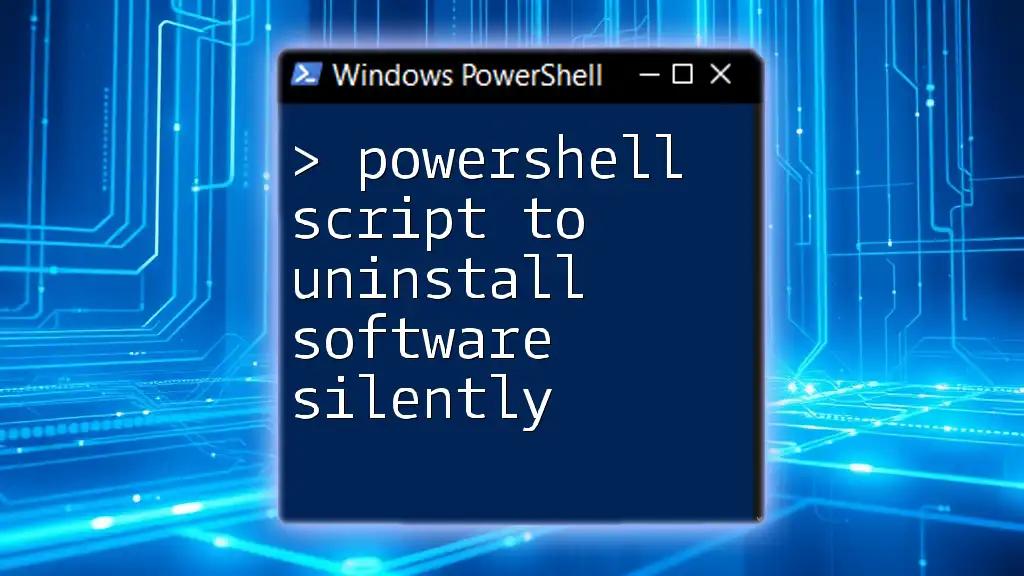
Basics of Software Installation with PowerShell
PowerShell Cmdlets for Installation
Cmdlets are the heart of PowerShell; they are lightweight commands used in the PowerShell environment. For installing software, two of the most commonly used cmdlets are:
- Invoke-WebRequest: This cmdlet allows you to make web requests, such as downloading files from the internet.
- Start-Process: Use this cmdlet to initiate processes and applications from PowerShell, including installers.
Setting Up Your Environment
Before diving into scripting, it’s crucial to set the appropriate execution policy to allow script execution. You can adjust this by opening PowerShell as an administrator and entering:
Set-ExecutionPolicy RemoteSigned
This command permits the execution of local scripts while requiring remote scripts to be signed by a trusted publisher. Additionally, ensure that you have the necessary permissions to install software on the target system.

Creating a PowerShell Script for Installing Software
Step-by-Step Guide to Writing a Script
Creating your PowerShell script can be broken down into a few simple steps.
1. Create the Script File: Begin by creating a new `.ps1` file using your favorite code editor or PowerShell Integrated Scripting Environment (ISE).
2. Open PowerShell ISE: PowerShell ISE is an excellent tool for beginners, allowing for easy editing and debugging.
Example Script Structure
Here is a simple script to demonstrate the basic flow of downloading and installing software:
# Example Script to Install Software
$SoftwareURL = "http://example.com/software-installer.exe"
$InstallerPath = "$env:TEMP\software-installer.exe"
# Download the installer
Invoke-WebRequest -Uri $SoftwareURL -OutFile $InstallerPath
# Run the installer
Start-Process -FilePath $InstallerPath -ArgumentList "/silent" -Wait
In this script:
- $SoftwareURL stores the URL of the software installer you want to download.
- $InstallerPath defines the path where the installer will be stored temporarily on your system.
- The Invoke-WebRequest cmdlet fetches the installer from the specified URL, saving it to the defined path.
- Finally, the Start-Process cmdlet runs the installer with the `/silent` argument, which allows it to be installed without user interaction. The -Wait parameter ensures the script pauses until the installer completes.
Adding Error Handling and Logging
To ensure that your scripts run smoothly, incorporating error handling is essential. You can use try-catch blocks to catch any exceptions that occur during execution. For example:
try {
Invoke-WebRequest -Uri $SoftwareURL -OutFile $InstallerPath
} catch {
Write-Host "Failed to download software. Error: $_"
}
Logging the outcome of your installations is also a best practice. You can direct output to a log file by appending:
Start-Process -FilePath $InstallerPath -ArgumentList "/silent" -Wait | Out-File -FilePath "$env:TEMP\install-log.txt" -Append

Advanced Techniques in PowerShell Script for Software Installation
Using Package Management in PowerShell
The `PackageManagement` module allows you to discover, install, and manage software packages easily. To leverage this feature, you can use the `Install-Package` cmdlet.
For example, if you are using Chocolatey (a popular package manager for Windows), the installation command would look like this:
Install-Package -Name "software_name" -Source "chocolatey"
This single line can save time by automating the entire package installation process without downloading installers.
Automating Updates
Once your software is installed, maintaining it is crucial. You can create another script to automate updates:
$SoftwareName = "software_name"
Update-Package -Name $SoftwareName -Source "chocolatey"
Running this command would update the specified software to the latest version available on Chocolatey. You can also schedule this script to run daily or weekly using Windows Task Scheduler, ensuring your installed software is always up-to-date.
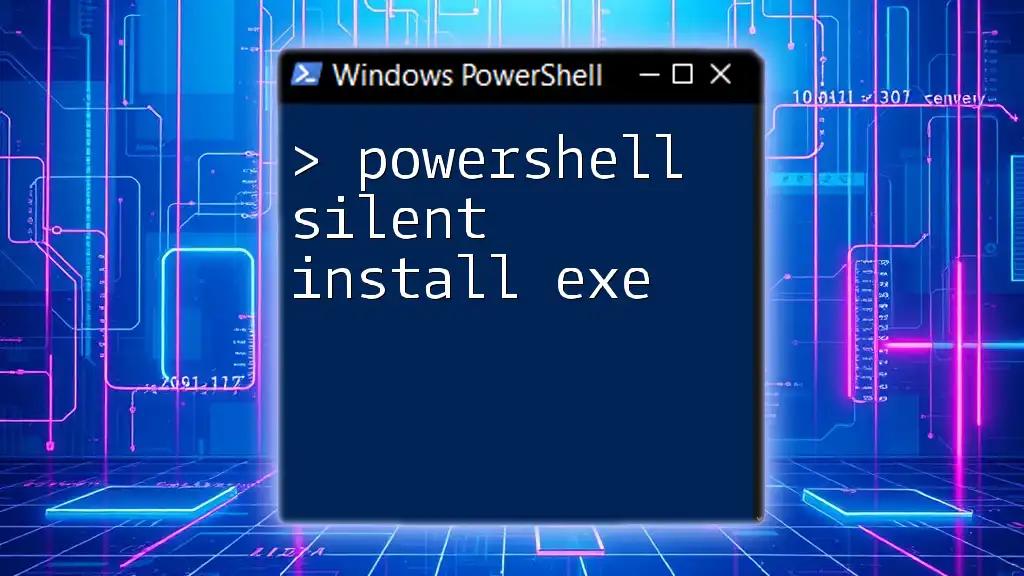
Best Practices for Writing PowerShell Installation Scripts
Commenting and Documentation
Adding comments to your scripts is crucial for maintainability and readability. Explain the purpose of each section clearly to help others (or yourself) understand the script later on.
Keeping Scripts Secure
To prevent security issues, avoid hardcoding sensitive information in your scripts, such as access credentials or API keys. Instead, use secure methods like storing them in encrypted files or leveraging Windows Credential Manager.
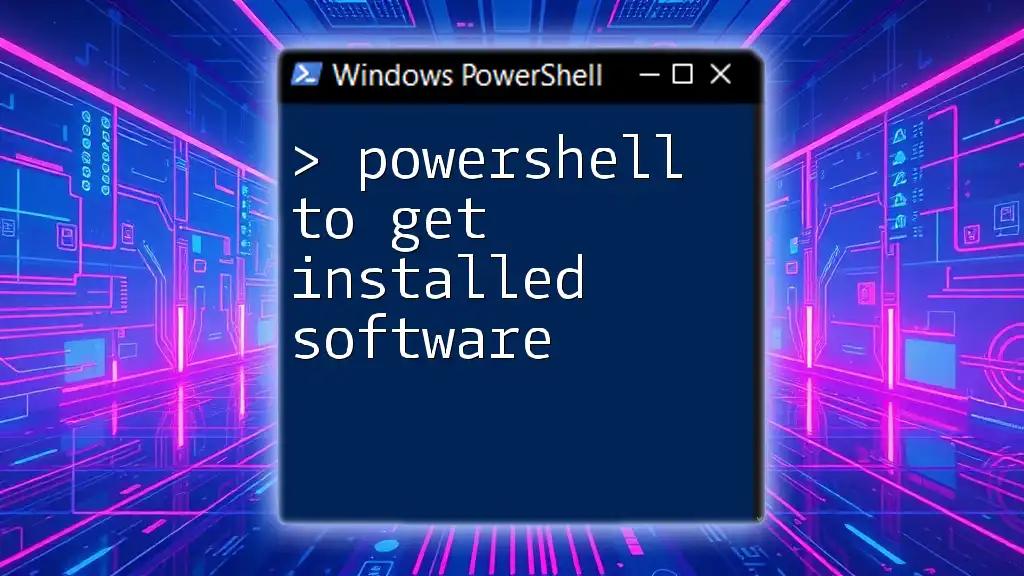
Troubleshooting Common Issues
Common Installation Errors
During script execution, you may encounter several issues. Always check for:
- HTTP errors (e.g., 404 Not Found) when downloading files.
- Permission issues that may prevent installations from completing.
Useful Cmdlets for Troubleshooting
If you run into problems, there are cmdlets available to help diagnose issues:
- Get-Command: Lists all cmdlets and their syntax.
- Get-Error: Displays detailed error information for troubleshooting.
- Get-Help: Provides guidance on cmdlets and their usage directly from the command line.
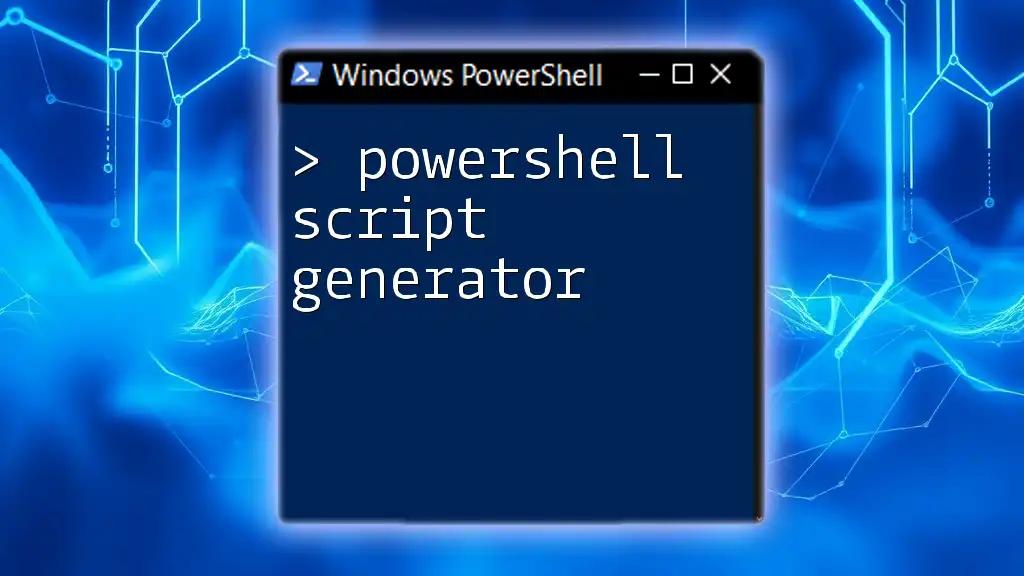
Conclusion
Using a PowerShell script to install software not only enhances efficiency but also adds a level of automative consistency that manual installations cannot provide. With the skills and techniques outlined in this guide, you can confidently create, manage, and troubleshoot scripts tailored to your software installation needs.
By honing your PowerShell skills, you're well on your way to automating not just software installations but a myriad of other tasks. Keep experimenting, and don’t hesitate to reach out to communities for support as you progress!
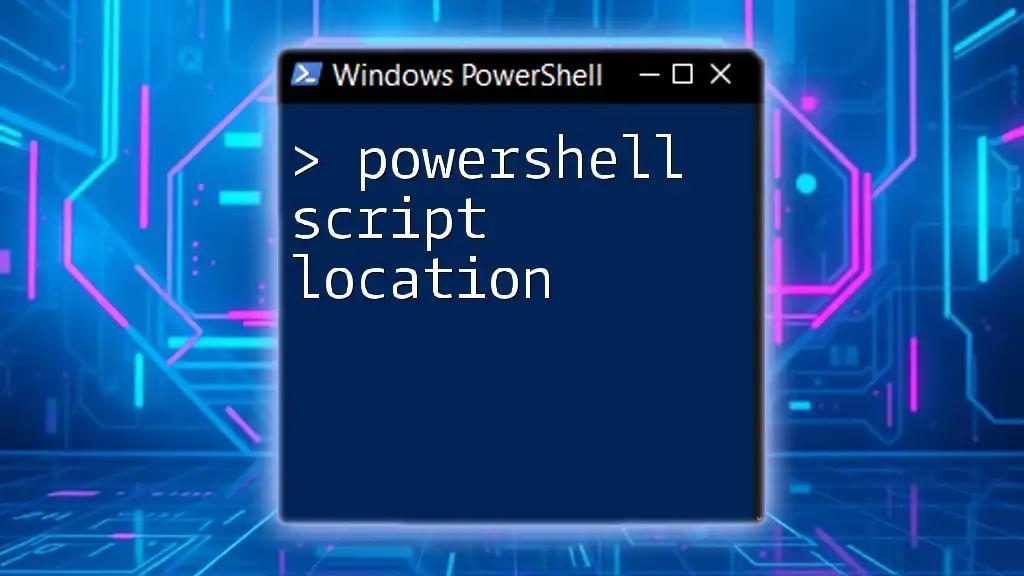
Call to Action
We encourage you to explore further into the world of PowerShell scripting. Consider subscribing for more insights and training opportunities to elevate your scripting abilities!