To silently uninstall software using PowerShell, you can use the following command which queries the installed software and removes it without user interaction.
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'SoftwareName'" | ForEach-Object { $_.Uninstall() }
Replace `'SoftwareName'` with the actual name of the software you wish to uninstall.
Understanding Software Uninstallation
What is Silent Uninstallation?
Silent uninstallation refers to the process of removing software from a system without displaying any user interface or requiring user interaction. This method is particularly valuable in scenarios where user experience is a priority or when automating processes for system administration.
The primary benefits of silent uninstallation include:
- Efficiency: It minimizes disruptions in user activity, as no prompts or alerts are displayed.
- Automation: Silent uninstallations can be scripted and scheduled, saving time for IT teams.
- Consistency: Ensures that software is removed in a uniform manner, reducing errors.
Common Scenarios for Silent Uninstallation
Silent uninstallation is commonly applied in several scenarios:
- Remote Administration in IT Environments: IT professionals often manage multiple machines simultaneously, requiring effective tools to remove unnecessary software remotely.
- Deployment Scenarios in Enterprise Settings: During software updates or transitions, old software may need to be uninstalled swiftly to ensure smooth operational continuity.
- Automated Scripts During Software Updates: Automated processes that include silent uninstallations help maintain system integrity without user intervention.
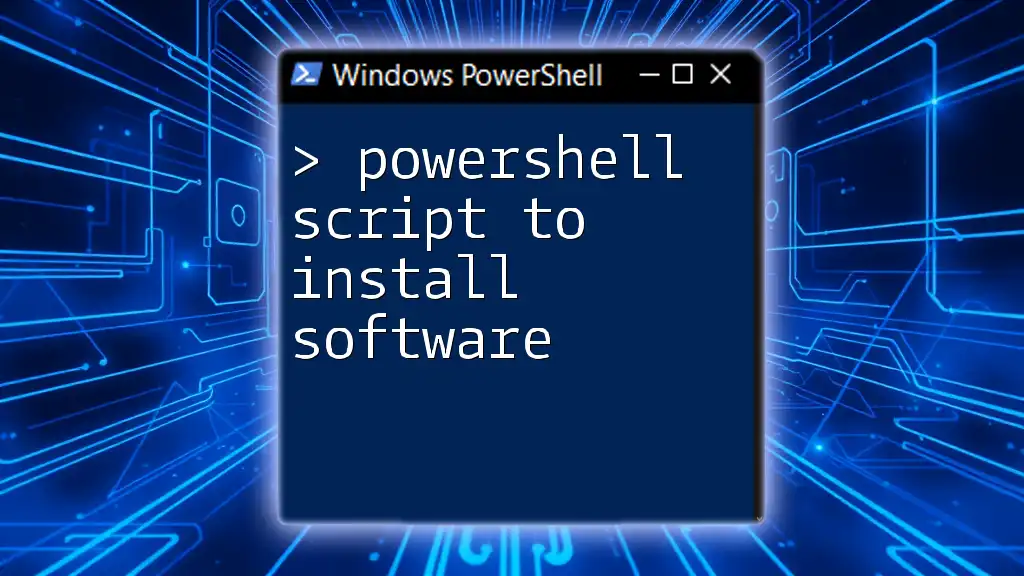
Getting Started with PowerShell
Setting Up Your PowerShell Environment
Before diving into the PowerShell script to uninstall software silently, you need to ensure your environment is set up correctly:
- Ensure PowerShell is Installed: Most Windows operating systems come with PowerShell pre-installed; however, verify its availability on your device.
- Run PowerShell with Administrative Privileges: Since software uninstallation often requires administrative rights, it’s critical to execute PowerShell as an administrator. You can do this by right-clicking on the PowerShell icon and selecting "Run as administrator."
- Overview of Execution Policies in PowerShell: PowerShell has different execution policies that control the running of scripts. To run scripts you’ve created, you may need to set the policy to "RemoteSigned" or "Unrestricted."
Set-ExecutionPolicy RemoteSigned
Basic PowerShell Commands for Software Management
To manage installed software, you can use the `Get-WmiObject` cmdlet. This command allows you to list all software installed on the system:
Get-WmiObject -Class Win32_Product
This command will return an object for each installed application, providing details such as names, versions, and installation dates. Understanding how to retrieve this data is crucial before proceeding with any uninstallation tasks.
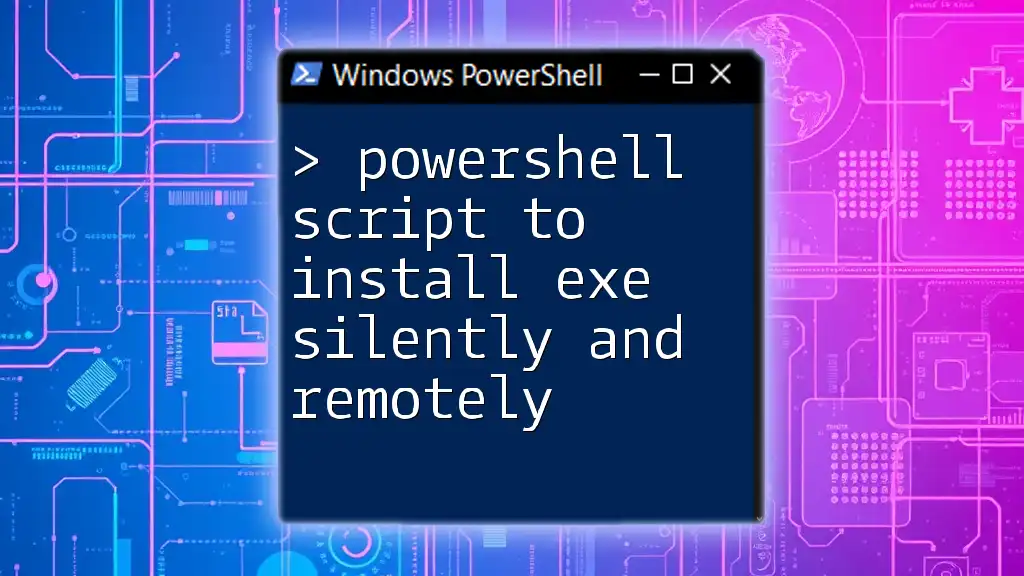
The Silent Uninstallation Process
Identifying the Software to Uninstall
Identifying the exact software name to uninstall is a pivotal step. Using the `Get-Package` cmdlet can help locate installed packages that match your criteria.
Get-Package | Where-Object { $_.Name -like "*SoftwareName*" }
This command will filter the installed packages, allowing you to find the exact name of the software you wish to uninstall.
Writing the Silent Uninstall Script
With the software identified, you can now write your PowerShell script. A typical uninstall script uses the `msiexec` command, which allows for the removal of installed software without user prompts.
Example Code Snippet
$softwareName = "YourSoftwareName"
$installerPath = "C:\Path\To\Installer.msi" # Adjust the path as necessary
Start-Process msiexec.exe -ArgumentList "/x $installerPath /quiet /norestart" -Wait
In this script:
- `$softwareName` stores the name of the software you intend to uninstall.
- `$installerPath` includes the path to the MSI file associated with the software.
- The `-ArgumentList` parameter specifies the uninstall command, where `/x` indicates uninstallation, `/quiet` ensures it runs silently, and `/norestart` prevents automatic system restarts.
Using PowerShell to Execute the Uninstall Command
After writing the script, you will use `Start-Process` to execute it. This command can take parameters that allow you to control the process behavior:
- The `-Wait` switch is particularly useful, as it ensures the script waits for the uninstallation process to complete before proceeding. This synchronous behavior can help in managing subsequent tasks in your script.
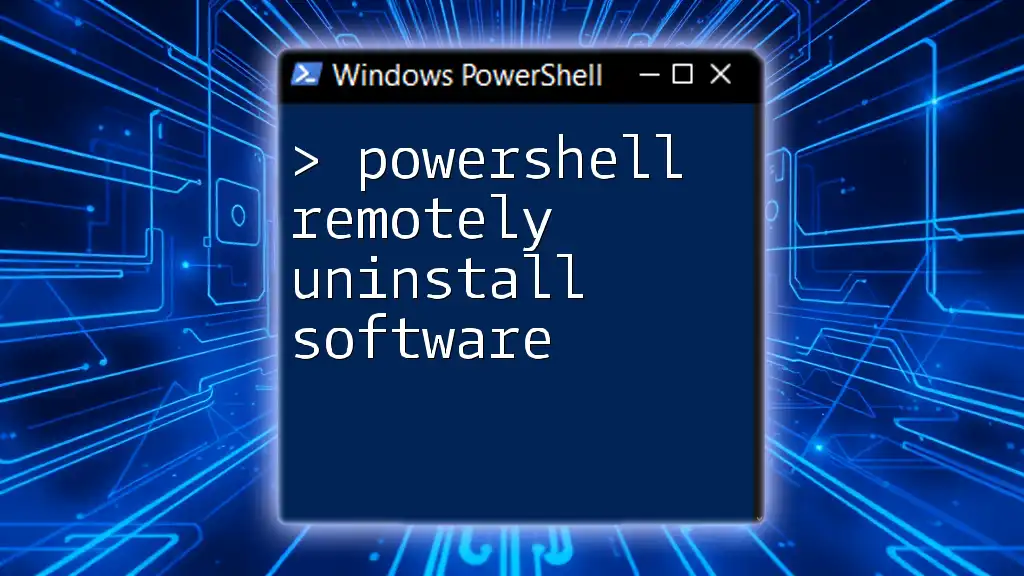
Handling Potential Issues
Common Errors During Silent Uninstallation
When running your silent uninstallation script, be prepared to encounter some common errors, such as:
- Permissions Issues: If the script is not run with administrative privileges, it may fail. Always ensure PowerShell is executed as an admin.
- Wrong Installer Path: If the path to the installer is incorrect, the script will not execute successfully. Double-check your paths.
Logging the Uninstallation Process
Implementing logging is invaluable for troubleshooting and ensuring your silent uninstallation went smoothly. Here’s how you can add logging to your PowerShell script.
Example Code Snippet for Logging
$logFilePath = "C:\Path\To\LogFile.txt"
Start-Process msiexec.exe -ArgumentList "/x $installerPath /quiet /norestart" -Wait -RedirectStandardOutput $logFilePath -RedirectStandardError $logFilePath
This code not only executes the uninstallation but also redirects the output and error messages to a log file. This practice allows you to keep track of any issues that may arise during the uninstallation process.
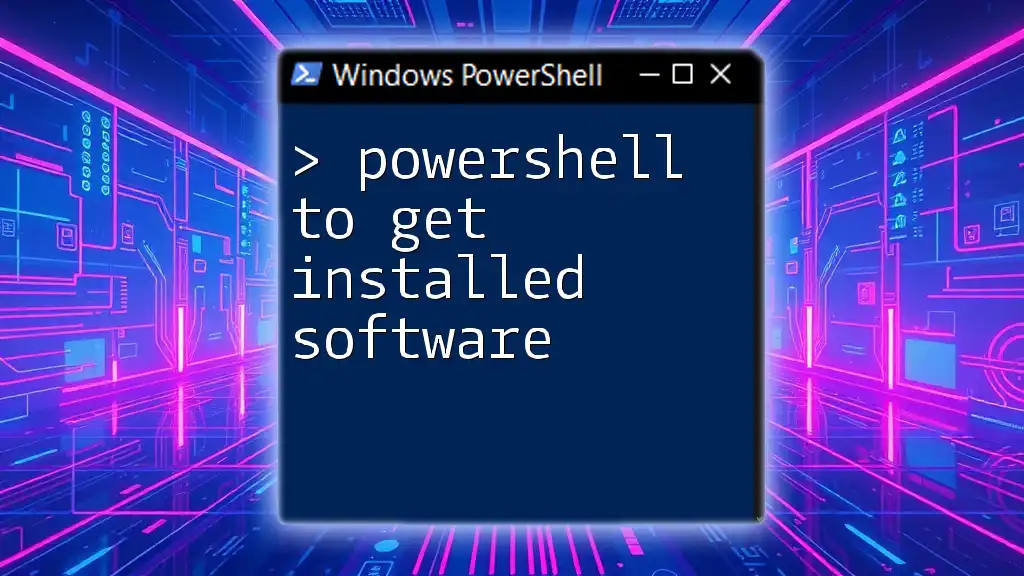
Testing Your Script
Verifying the Uninstallation
Once the script has run, it’s essential to verify that the software has been successfully uninstalled. You can use the following command:
Get-WmiObject -Class Win32_Product | Where-Object { $_.Name -eq "YourSoftwareName" }
If the command returns no objects, the software has been successfully removed.
Troubleshooting Common Issues
Having a troubleshooting checklist can expedite the resolution of issues:
- Double-check Names and Paths: Make sure software names and installer paths match those used in your script.
- Examine Log Files: Analyze the log files created during the uninstall process for detailed error messages.
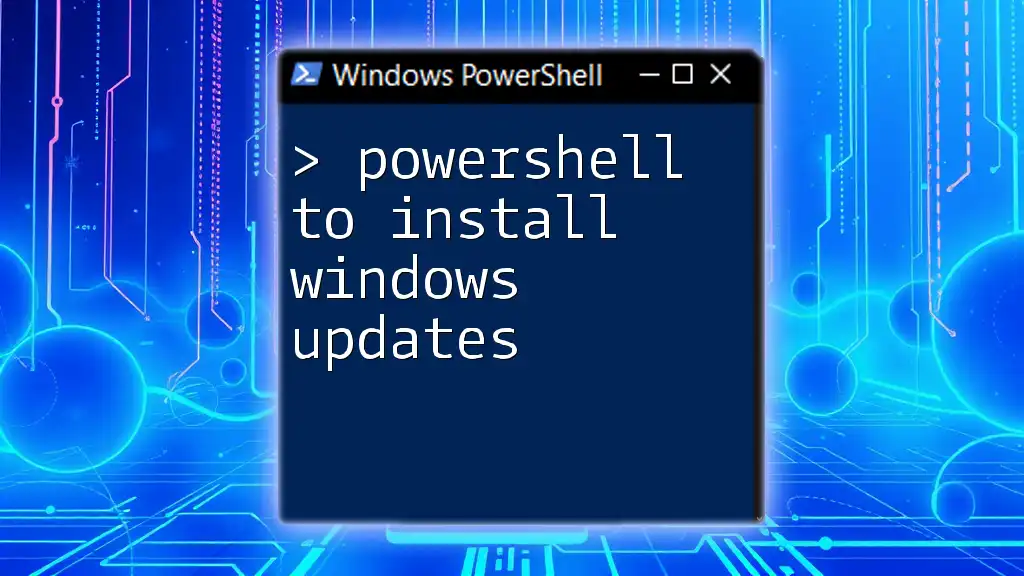
Conclusion
In this guide, we explored the process of creating a PowerShell script to uninstall software silently. We discussed the importance of silent uninstallation, how to set up PowerShell, and the specific commands needed to automate this task efficiently.
By understanding the concepts and examples provided, you should now be well-equipped to implement your silent uninstallation scripts. Practicing these scripts and adapting them to various software scenarios will enhance your PowerShell proficiency while streamlining software management processes in your environment.
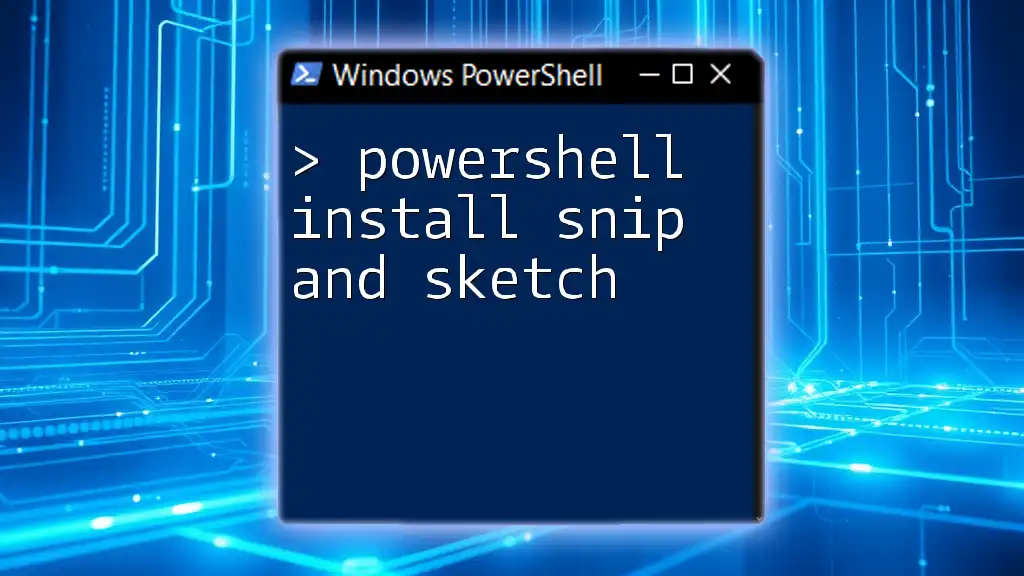
Call to Action
Try applying the script templates provided in various scenarios and feel free to modify them based on your specific needs. For ongoing updates and tips about PowerShell, consider subscribing to our newsletter and share your experiences or challenges in the comments below.
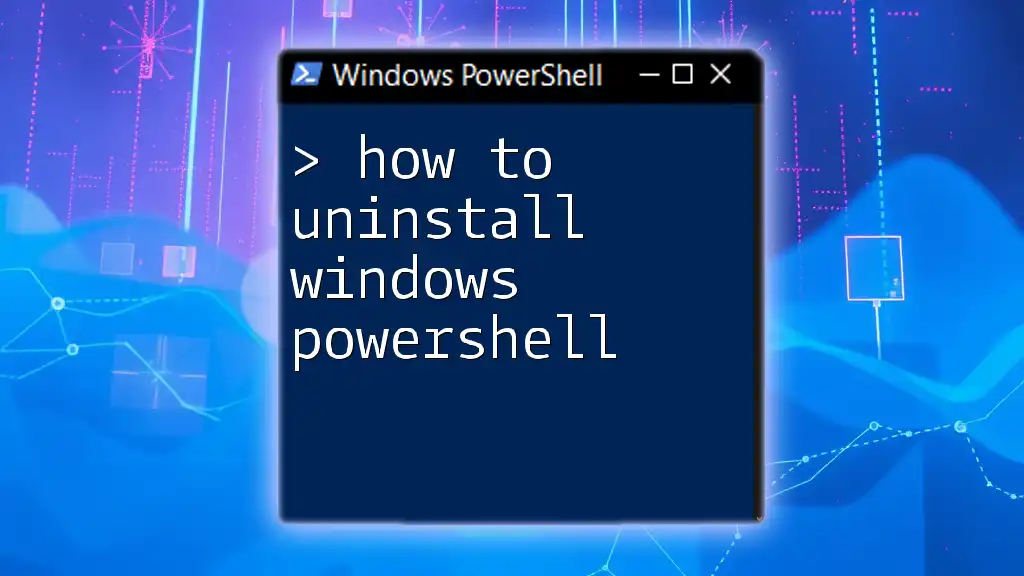
Further Reading
For additional insights, check these resources:
- The official Microsoft PowerShell documentation.
- Recommended books and online courses focused on PowerShell scripting and software management.