To perform a silent installation of an EXE file using PowerShell, you can run the following command that suppresses the installation interface and proceeds without user interaction.
Start-Process "C:\Path\To\YourInstaller.exe" -ArgumentList "/S /quiet" -Wait
In this example, replace `"C:\Path\To\YourInstaller.exe"` with the actual path to your installer, and the `/S /quiet` arguments are commonly used for silent installs, though you should verify the appropriate arguments for your specific installer.
Understanding Silent Installations
What is a Silent Install?
A silent install refers to the process of installing software without any user interaction. This means that the installation occurs in the background and does not require any prompts or decisions from the user. This is particularly useful in enterprise environments where applications need to be deployed across multiple machines without disturbing the users.
In essence, silent installs automate the installation process to ensure it is quick and efficient. They typically utilize specific parameters in the executable command that instruct the software to run without displaying any graphical user interface (GUI).
Benefits of Silent Installations
The advantages of doing silent installations are numerous:
- Reduced User Interaction: Users can continue working without interruptions, as installations happen seamlessly in the background.
- Faster Deployment: Enterprises can deploy applications more rapidly, reducing downtime and increasing productivity.
- Consistency Across Installations: Silent installs eliminate variability, ensuring that every installation proceeds with the same settings and parameters, thereby minimizing potential issues.
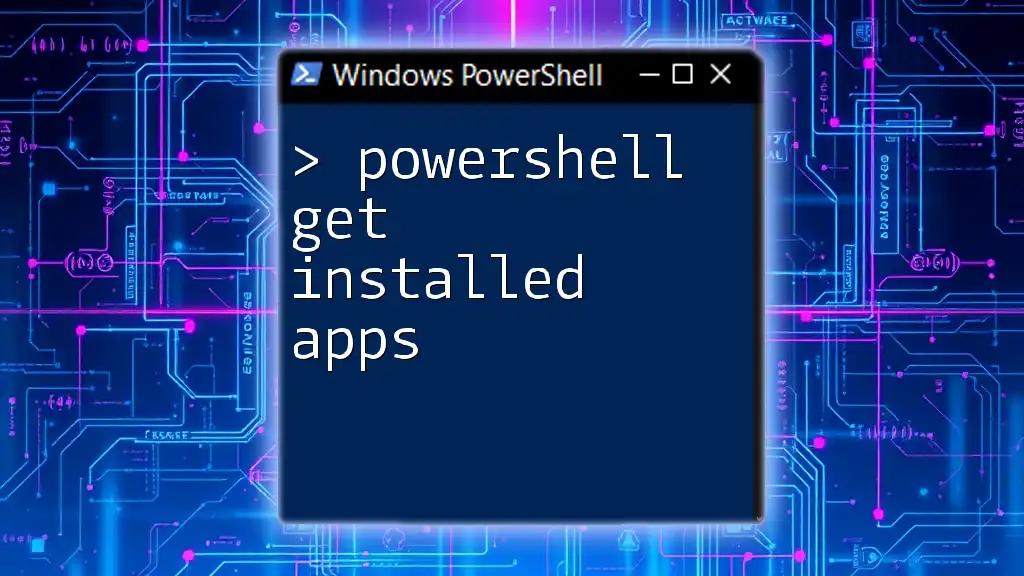
Preparing for Silent Installations
Prerequisites for PowerShell Silent Install
Before executing silent installations using PowerShell, it is essential to consider a few prerequisites:
- Ensure you have the necessary administrative permissions to install software on the target machines.
- Verify that any dependencies required by the application are already installed.
Finding the Right Installation Parameters
Different software providers have varying command-line options for silent installations. Common silent install parameters include:
- `/S` for Silent mode.
- `/silent` or `/quiet` to suppress all user interface messages.
For example, if you are working with a common EXE installer, you would need to consult the application's documentation to find the correct parameters for a silent installation.
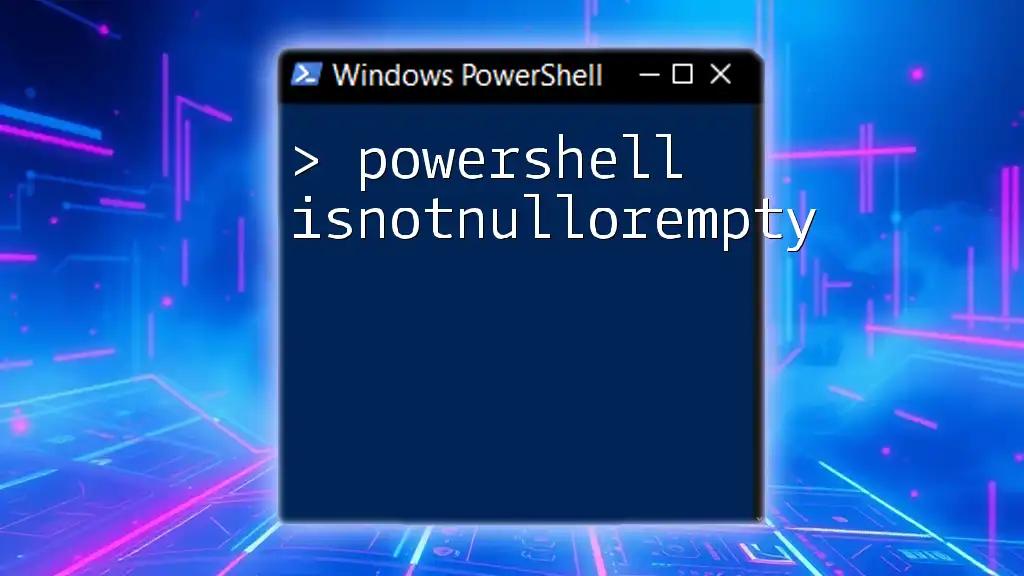
Using PowerShell for Silent Installations
Basic PowerShell Commands for Executing EXEs
To initiate a silent installation through PowerShell, you can utilize the `Start-Process` cmdlet. The basic structure is as follows:
Start-Process "C:\Path\To\Installer.exe" -ArgumentList "/S" -Wait
In this command, replace `"C:\Path\To\Installer.exe"` with the actual path to your installer. The `-ArgumentList` parameter allows you to specify the silent installation argument.
Detailed Breakdown of the Command
- Start-Process: This command initiates a new process in Windows. It is specifically useful for launching executables.
- -ArgumentList: Use this parameter to attach the silent install flags to the executable. Always ensure that you’re using the appropriate arguments designed for the specific installer you're working with.
- -Wait: This crucial parameter makes the script pause until the installation process is complete. This ensures that you can manage sequential installations without conflicts.
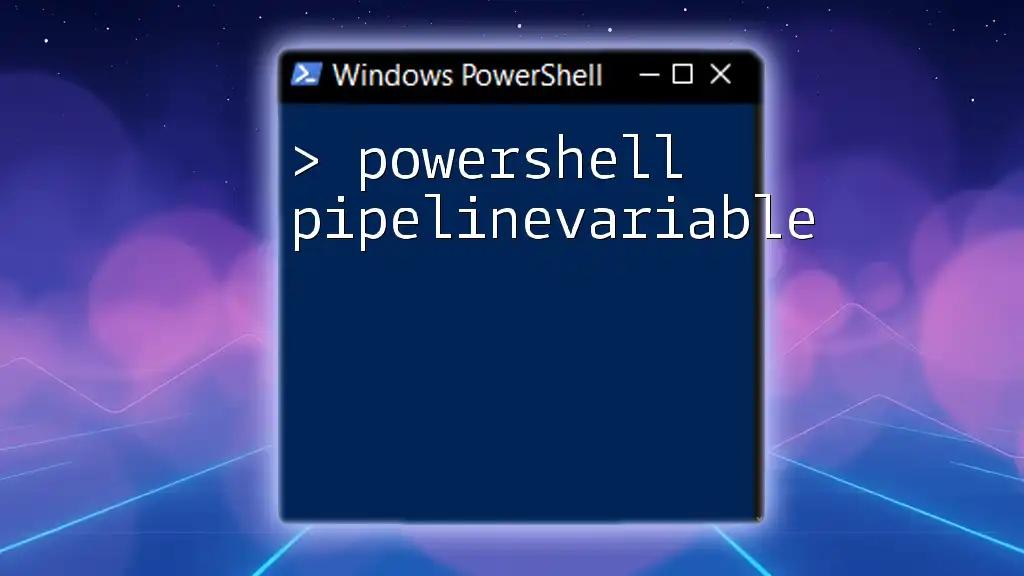
Advanced Techniques for Silent Installations
Error Handling and Logging
Error handling during installations is critical, especially in production environments. You can capture and log any errors that occur during the installation process using a `try-catch` block. Here's how you can implement it:
try {
Start-Process "C:\Path\To\Installer.exe" -ArgumentList "/S" -Wait -ErrorAction Stop
} catch {
Write-Output "Installation failed: $_"
}
This snippet will attempt to run the installer silently. If an error occurs, it will catch the exception and output a message detailing the failure.
Using PowerShell Scripting for Multiple Installations
When you need to install several applications, PowerShell allows you to streamline this with a simple loop. You can create an array of installers and iterate through them like so:
$installers = @(
"C:\Path\To\Installer1.exe",
"C:\Path\To\Installer2.exe"
)
foreach ($installer in $installers) {
Start-Process $installer -ArgumentList "/S" -Wait
}
This code snippet sequentially runs each installer with the specified silent flag, making it easy to manage multiple installations in one go.
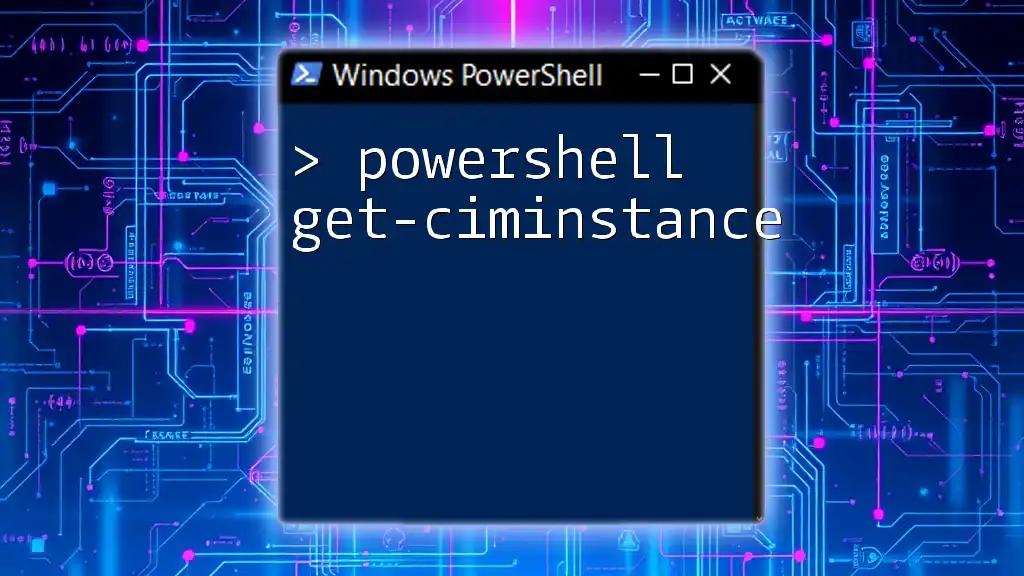
Testing Silent Install Executions
Validating Successful Installs
Once the installations are complete, verifying their success is vital. You can check if the software installed correctly by trying to locate the application using PowerShell:
if (Get-Command "SoftwareName" -ErrorAction SilentlyContinue) {
Write-Output "Installation successful."
} else {
Write-Output "Installation failed."
}
This command checks if the software is available in the system and outputs either a success or failure message based on the result.
Rollback Strategies for Failed Installations
Having a rollback plan is crucial in case an installation does not go as expected. Depending on the application, you may want to uninstall or revert to a previous version. This may involve saving the state or having an uninstallation command ready to go.
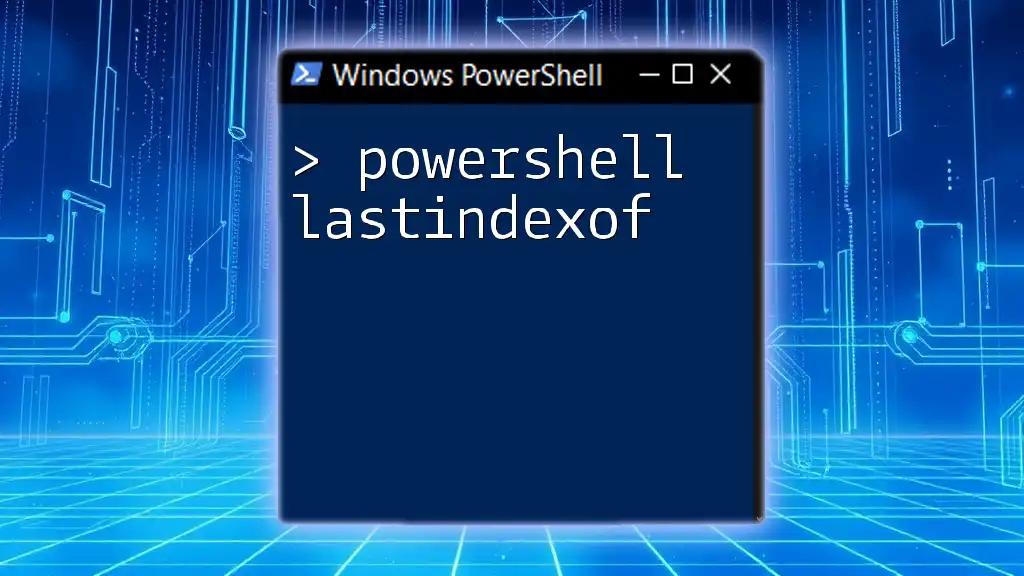
Common Issues and Troubleshooting
Common Silent Install Problems
While silent installations simplify the process, issues can still arise. Common problems might include missing dependencies, incorrect parameters, or lack of administrative rights. For instance, if you encounter an installation that hangs, a common fix is to verify that you are running PowerShell as an administrator.
Debugging Silent Installs with PowerShell
Debugging can be a challenge due to the lack of visible prompts during a silent installation. Adding logging within your PowerShell scripts can help track issues. Consider appending logging to any critical commands. For example, logging the output of the installation process can help identify issues post-failure.
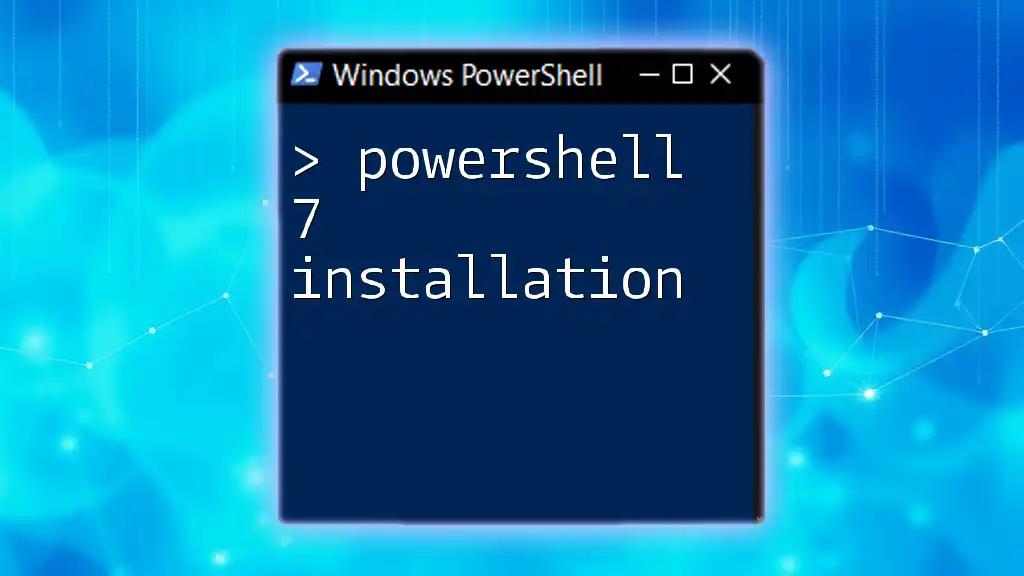
Conclusion
Mastering the art of executing a PowerShell silent install exe is invaluable for software deployment, particularly in enterprise environments. By leveraging PowerShell commands and understanding silent installation parameters, you can significantly enhance your automation processes. Practice these techniques and experiment with different EXEs to solidify your skills in PowerShell automation.
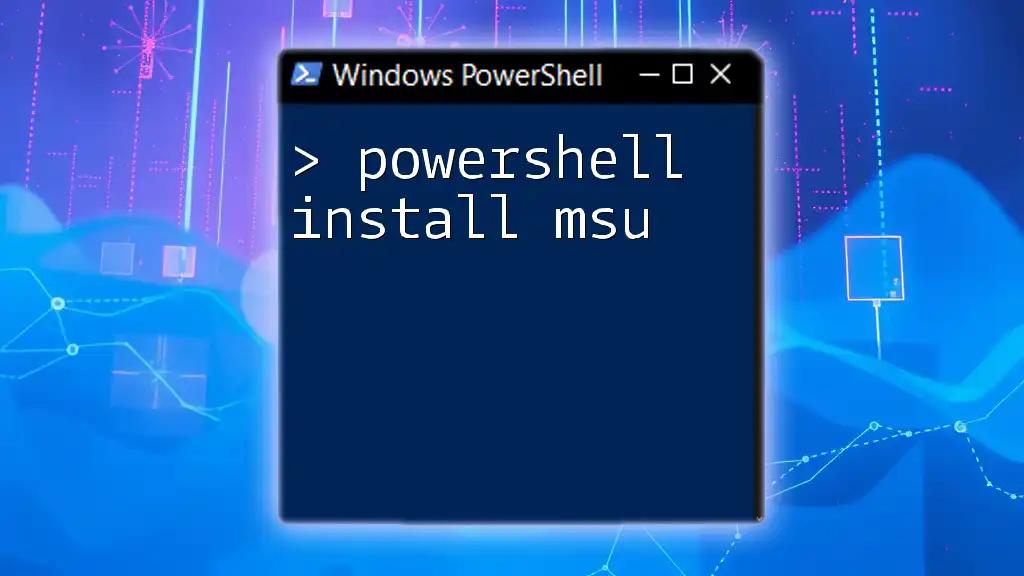
Additional Resources
For further reading and enhancement of your PowerShell skills, don’t hesitate to explore online communities, forums, and detailed documentation that discuss advanced scripting techniques and troubleshooting methods in PowerShell.