PowerShell script remote execution allows users to run commands or scripts on remote computers, streamlining management tasks across multiple systems.
Invoke-Command -ComputerName "RemotePC" -ScriptBlock { Get-Process }
Understanding PowerShell Remote Execution
What is Remote Execution?
PowerShell remote execution refers to the ability to run commands or scripts on a remote computer or multiple computers from a local machine. This capability is essential for system administrators and IT professionals who need to manage systems efficiently without being physically present at each location.
Benefits of Using Remote Execution
The advantages of using remote execution in PowerShell are numerous:
- Improved Efficiency: Rather than logging into each machine, you can execute commands across multiple devices simultaneously.
- Centralized Management: Manage all your systems from a single console, making it easier to maintain and troubleshoot issues.
- Automation Capabilities: Automate repetitive tasks and scheduled jobs across multiple systems without manual intervention.
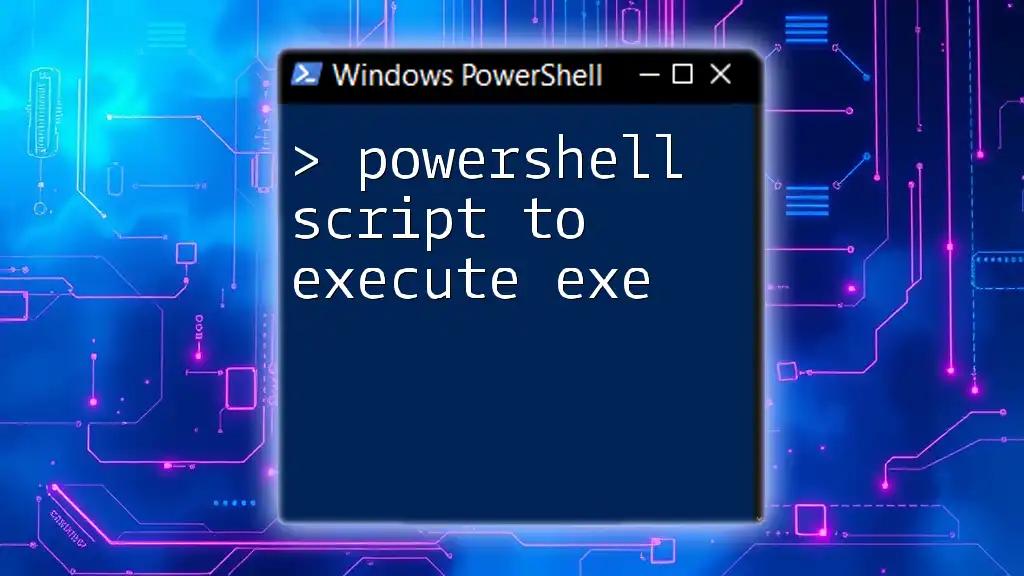
Preparing for Remote Execution
Before you can run scripts remotely, it's essential to prepare both your local and remote computers.
Configuring the Remote Computer
To enable PowerShell remoting on the target machine, you need to follow these critical steps:
Enabling PowerShell Remoting
To allow remote execution through PowerShell, execute the following command:
Enable-PSRemoting -Force
This command sets the necessary configurations for PowerShell remoting, ensuring that the WinRM (Windows Remote Management) service is running.
Firewall Configuration
Ensure that the firewall on the remote computer allows PowerShell remoting traffic. You can enable the appropriate rules using:
Set-NetFirewallRule -Name "WINRM-HTTP-In-TCP-Public" -Enabled True
Trusted Hosts Configuration
If you are connecting to computers in untrusted domains, you must set the trusted hosts list using the command:
Set-Item WSMan:\localhost\Client\TrustedHosts -Value "RemotePC" -Concatenate
Replace "RemotePC" with the corresponding names of your remote computers.
Considerations for Security
Security cannot be overlooked when executing scripts remotely. Here are several considerations to keep in mind:
- Using HTTPS for Secure Connections: For a secure channel, configure WinRM to use HTTPS rather than HTTP.
- Implementing Proper Authentication Methods: Ensure that you use robust authentication mechanisms such as Kerberos or NTLM to safeguard sensitive information.
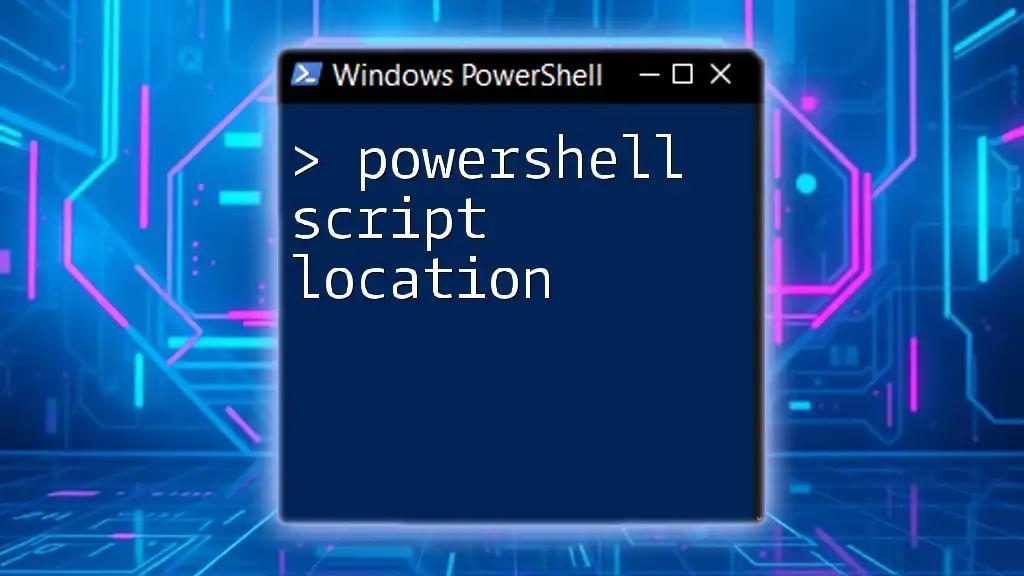
Running PowerShell Scripts Remotely
Once your environment is set up, you can begin running scripts remotely.
Using Enter-PSSession
The `Enter-PSSession` command allows you to create an interactive session with a remote computer. Here's how to use it:
Enter-PSSession -ComputerName RemotePC -Credential UserCredential
With this command, you're establishing a connection to "RemotePC" using your credentials. Once connected, you can run commands as if you were sitting at the remote machine.
Using Invoke-Command
For non-interactive execution of commands or entire scripts, use the `Invoke-Command` cmdlet:
Invoke-Command -ComputerName RemotePC -FilePath C:\Scripts\MyScript.ps1 -Credential UserCredential
This command allows you to execute a PowerShell script located at the specified path on the remote machine. You can also include multiple computers in the `-ComputerName` parameter for batch execution.
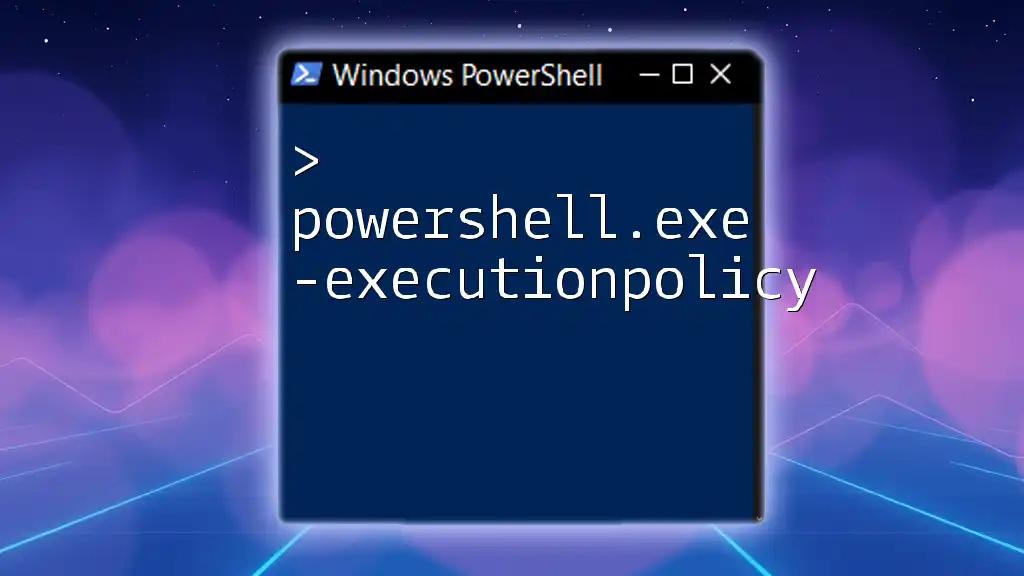
Best Practices for Remote Script Execution
Debugging Remote Scripts
Debugging is crucial, especially when working with remote scripts. Use the `-Verbose` and `-Debug` parameters for enhanced output that can help identify issues quickly. Here’s an example:
Invoke-Command -ComputerName RemotePC -FilePath C:\Scripts\MyScript.ps1 -Verbose
Logging and Monitoring
Always implement proper logging and monitoring for your remote scripts. Utilize PowerShell transcripts to log all command output and errors, which can be beneficial for audits and troubleshooting. You can enable transcripts in your scripts with:
Start-Transcript -Path C:\Logs\ScriptLog.txt
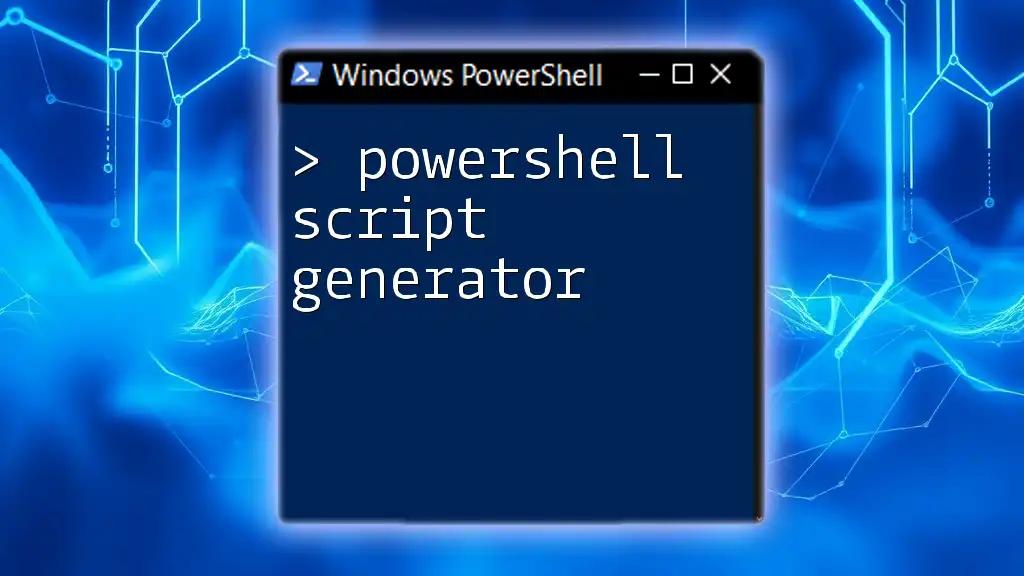
Common Scenarios and Solutions
Running Batch Scripts on Multiple Computers
PowerShell makes it simple to run scripts on multiple machines concurrently. Use the `Invoke-Command` with the `-AsJob` parameter to execute a script in the background:
Invoke-Command -ComputerName (Get-Content machines.txt) -FilePath C:\Scripts\BatchScript.ps1 -AsJob
This command reads from a text file containing a list of machine names and runs the specified script on each.
Automation with Scheduled Tasks
You can also manage scheduled tasks remotely. To create a new scheduled task that executes a PowerShell script at a specific time on a remote computer, use:
$Action = New-ScheduledTaskAction -Execute 'PowerShell.exe' -Argument '-File C:\Scripts\MyScript.ps1'
Register-ScheduledTask -Action $Action -Trigger (New-ScheduledTaskTrigger -At 12:00PM) -TaskName 'RunMyScript' -Computer 'RemotePC'
This example sets up a scheduled task named "RunMyScript" that triggers daily at noon.
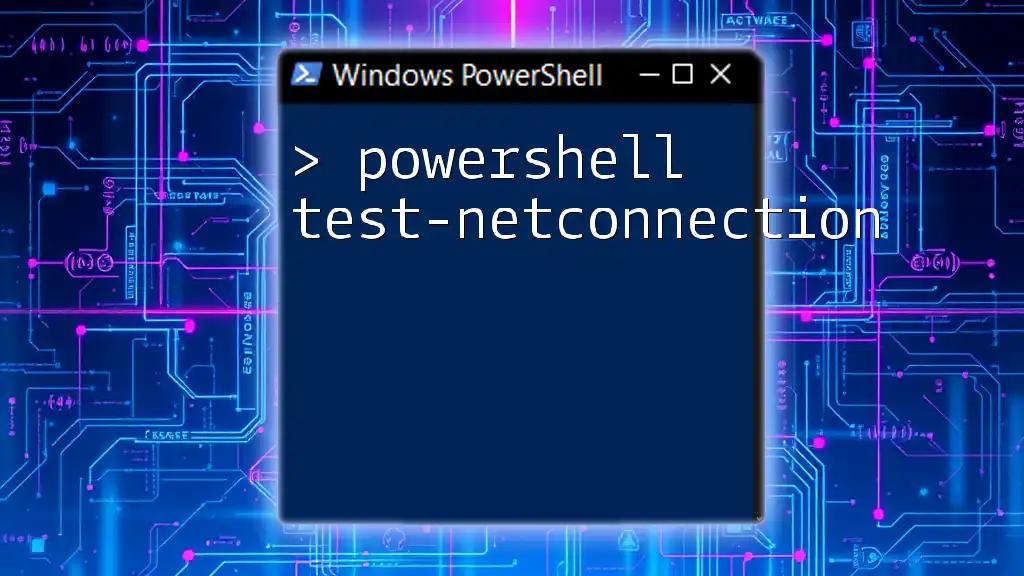
Conclusion
PowerShell script remote execution is a powerful feature that offers profound efficiencies in system administration. By following the guidelines laid out in this article, such as proper configuration, secure practices, and best execution methods, you can greatly enhance your remote management capabilities.
Start developing your skills with PowerShell remote execution today, automating processes and optimizing your workflow. Embrace these practices, and unlock the full potential of PowerShell for your needs.