Powershell security best practices encompass measures such as using least privilege principles, enabling script signing, and regularly auditing commands to protect your systems from malicious activities.
# Example: Run a script with the current user's context, ensuring least privilege
Start-Process powershell.exe -ArgumentList '-File C:\Path\To\YourScript.ps1' -NoNewWindow -Verb RunAs
Understanding PowerShell Security Risks
In today’s digital landscape, the robust capabilities of PowerShell can be a double-edged sword. While it provides powerful tools for system administration and automation, it is also vital to understand the security risks associated with its use.
Common Threats
Unauthorized access is one of the primary risks when using PowerShell. Malicious actors can exploit weak access controls to gain unauthorized access to systems and sensitive data. A simple misconfiguration can lead to significant breaches that compromise entire networks.
Another critical concern is the potential for malware and ransomware attacks. PowerShell can be utilized by malicious scripts to execute harmful commands, putting an organization at risk if not properly secured.
Real-World Examples
Among notable incidents, the use of PowerShell in the WannaCry ransomware attack highlights how scripts can be deployed to conduct malicious activities automatically. Such attacks underline the urgency of implementing proper security protocols.
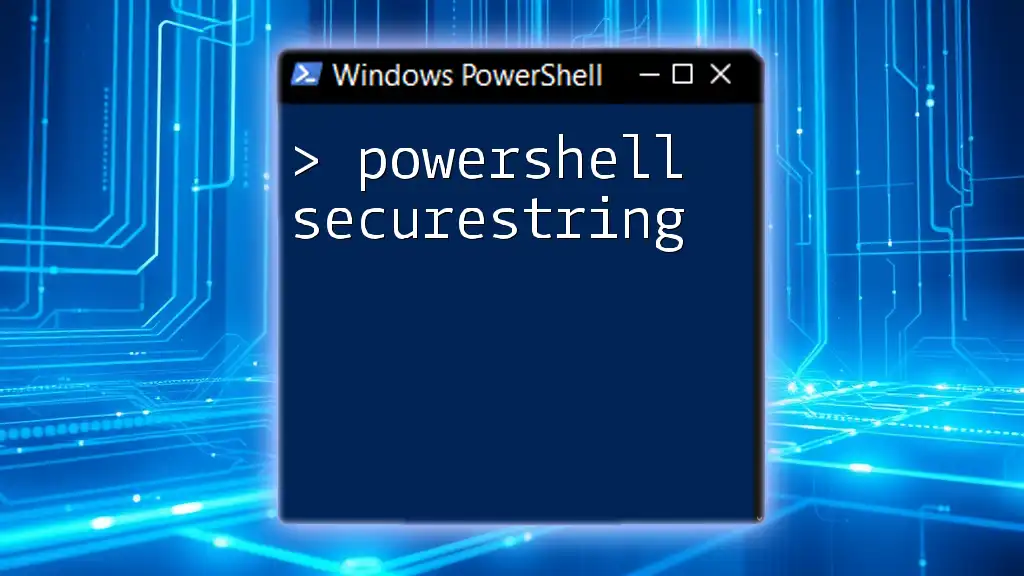
Configuring User Access Control
Least Privilege Principle
Implementing the least privilege principle is crucial in maintaining security in any IT environment, particularly when using PowerShell. This principle dictates that users should have only the permissions necessary to perform their job functions, minimizing the risk of unauthorized actions.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is another effective method for securing PowerShell commands. This control limits user permissions based on their roles within the organization.
To set up a new role with restricted access, you can use the following command:
# Example of creating a role
New-ManagementRole -Name "PowerShell Operator" -Description "Limit PowerShell actions."
By implementing RBAC, you can ensure users have controlled access to execute specific PowerShell scripts while safeguarding critical system pathways.
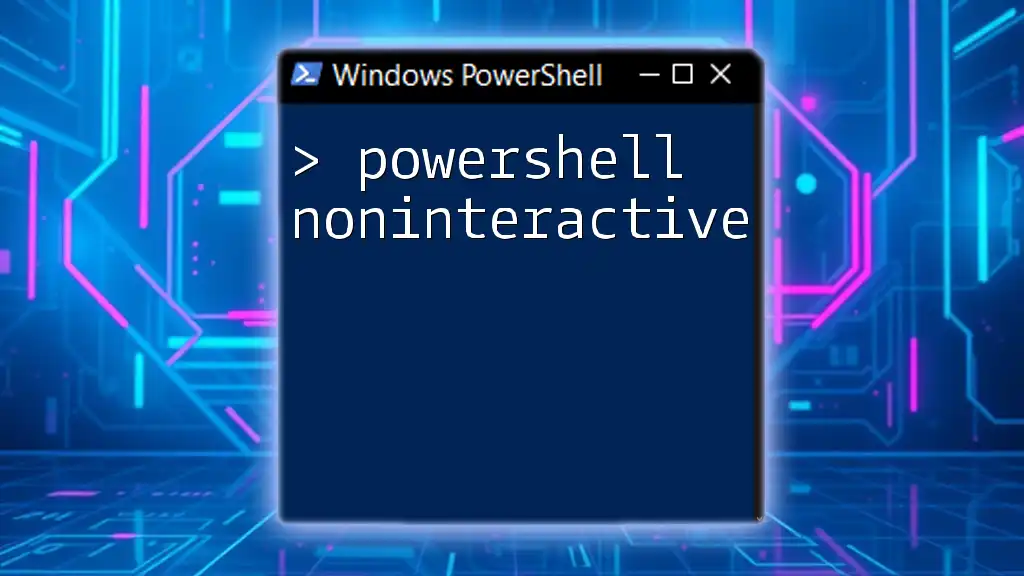
Implementing PowerShell Execution Policies
Understanding Execution Policies
PowerShell execution policies are key to controlling how scripts are run on your system. These policies can help prevent the execution of unauthorized scripts. The different types of execution policies include:
- Restricted - No scripts can be run.
- AllSigned - Only scripts signed by a trusted publisher can be run.
- RemoteSigned - Scripts created locally can run, but downloaded scripts must be signed.
- Unrestricted - All scripts can run but with warnings for downloaded scripts.
Recommended Policies for Security
For enhanced security, it's recommended to set the execution policy to RemoteSigned. This setting strikes a balance between flexibility and safety, allowing local scripts while requiring signatures for downloaded scripts.
You can configure your execution policy with the following command:
# Setting the execution policy
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
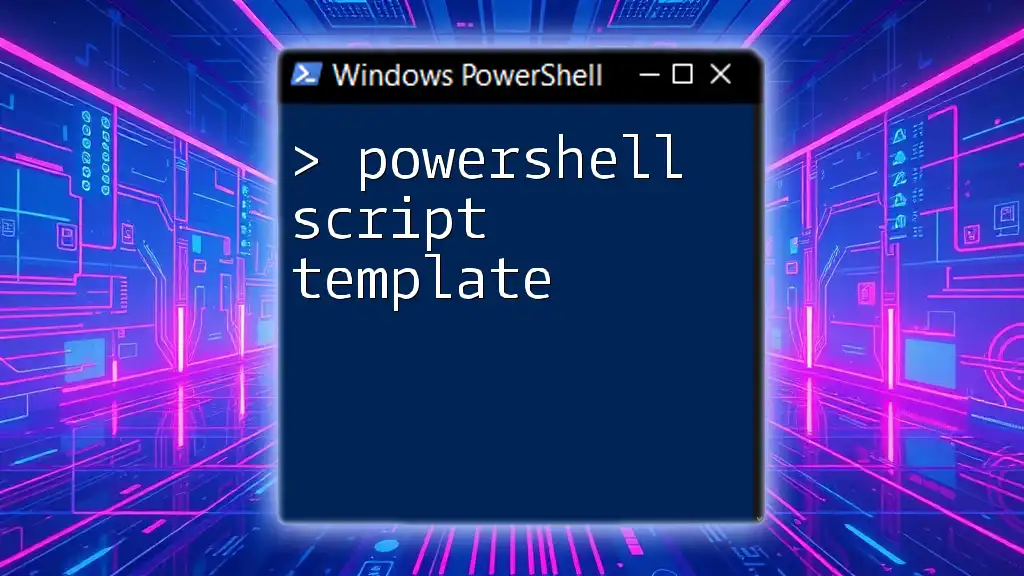
Utilizing Transcription and Logging
Enabling PowerShell Transcription
Transcription is an essential part of tracking PowerShell activity. Enabling transcription allows you to log all command inputs and outputs, which can be invaluable during audits and security reviews.
To enable transcription, you can use:
# Enable transcription
Start-Transcript -Path "C:\Logs\PowerShell_transcript.txt"
This command initiates a logging session, capturing everything executed in the PowerShell console.
Monitoring Log Files for Anomalies
Regularly monitoring log files for irregularities can help detect malicious activity. Tools and techniques for log analysis can assist in identifying patterns or anomalies that may indicate unauthorized access or scripts running outside of expected parameters.
For instance, you can analyze the transcribed logs for any errors or unauthorized actions using:
# Example to find failed commands in logs
Select-String -Path "C:\Logs\PowerShell_transcript.txt" -Pattern "Error"
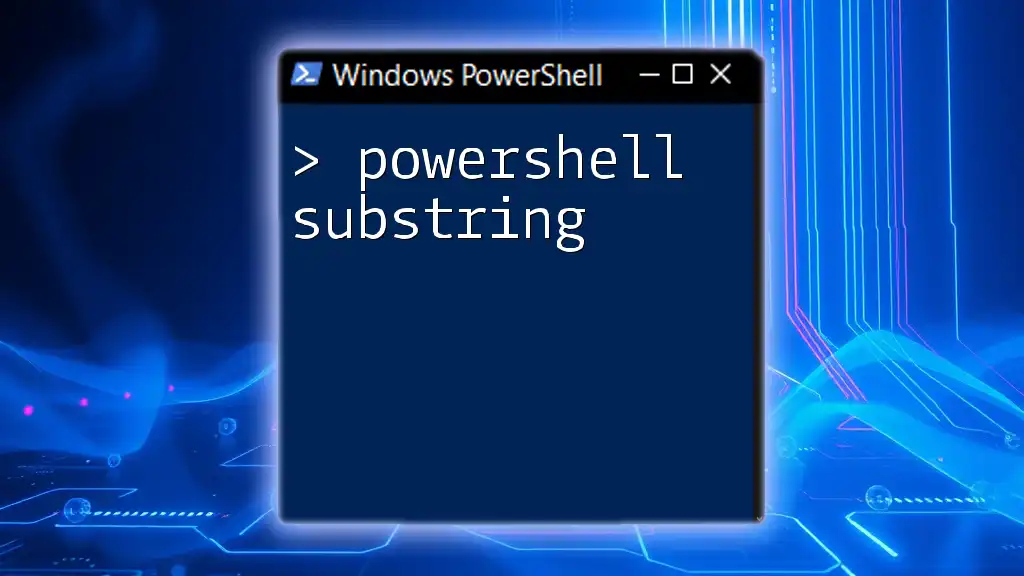
Using Secure Connections and HTTPS
Importance of Secure Connections
Ensuring that your PowerShell scripts communicate over secure connections is imperative to prevent data interception and breaches. Using HTTPS ensures that data transmitted between servers and clients is encrypted.
How to Force HTTPS in PowerShell
When dealing with remote resources, always prefer secure connections. You can enforce secure connections using code such as:
# Example of making a secure web request
Invoke-WebRequest -Uri "https://example.com" -UseBasicP -Credential $cred
This command creates a secure web request, guaranteeing that sensitive information is transmitted securely.
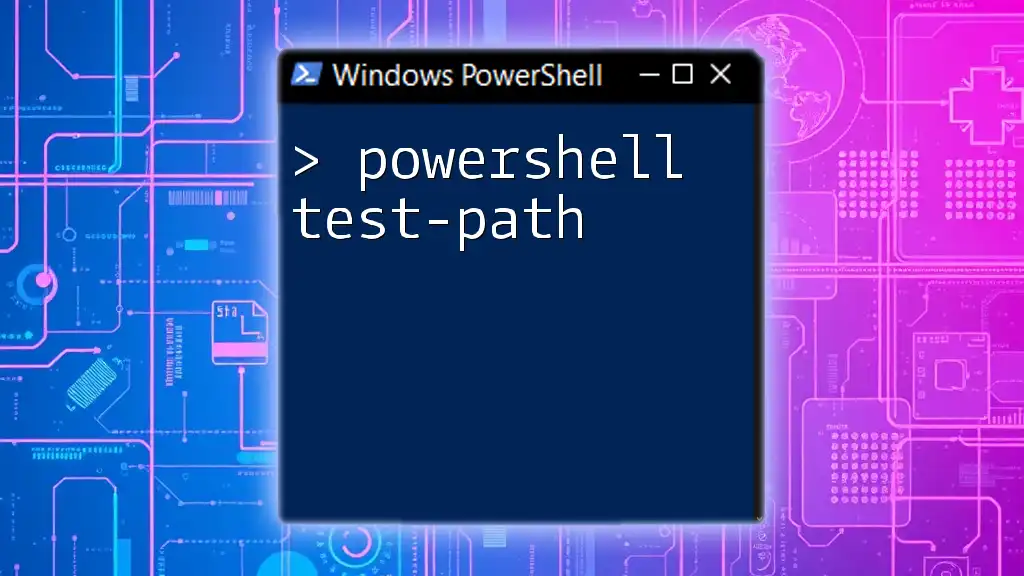
Regularly Updating PowerShell
Staying Updated with Security Patches
Keeping PowerShell updated with the latest security patches is critical. Regular updates can mitigate the risks of vulnerabilities that could be exploited by attackers.
Installing Updates
To check for and install updates, you can use the following command:
# Example command to check for PowerShell updates
Get-Package -Name PowerShell -Provider NuGet | Update-Package
Having the latest security features and fixes enhances your overall security posture when using PowerShell.
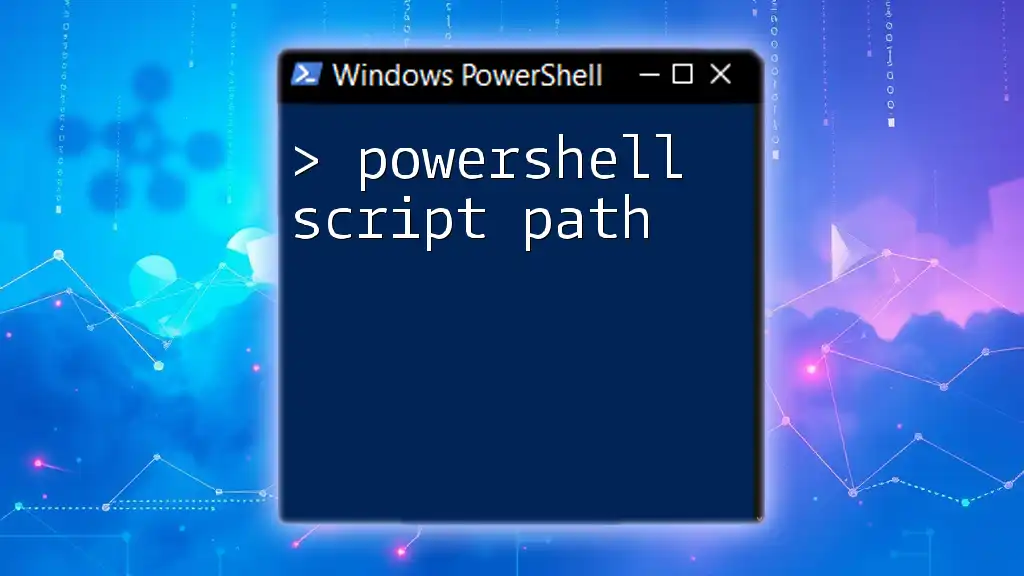
Securing PowerShell Scripts and Modules
Code Signing
Implementing code signing is essential for ensuring the integrity and authenticity of your PowerShell scripts. By signing your scripts, you can protect users from executing malicious or tampered code.
You can sign a script using:
# Example command to sign a PowerShell script
Set-AuthenticodeSignature -FilePath "C:\Scripts\myscript.ps1" -Certificate $cert
This command assigns a trusted digital signature to your script, enhancing security when executed by others.
Review Code for Security
Regular code reviews for security vulnerabilities should be an ongoing process. This can include using tools designed to analyze PowerShell scripts for risky commands or security weaknesses.
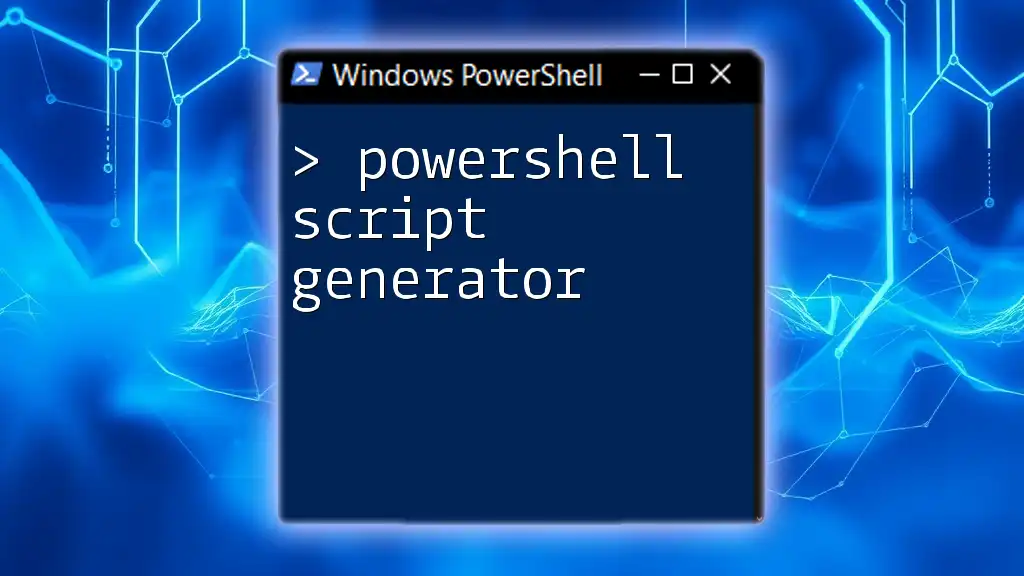
Best Practices for Script Development
Writing Secure Code
When developing PowerShell scripts, prioritize writing secure code. This includes validation of inputs, proper error handling, and avoiding hard-coded credentials.
Using Try/Catch Blocks
Implementing Try/Catch blocks is a cornerstone of effective error handling in PowerShell. They allow you to manage exceptions and respond appropriately without causing script failures.
For example:
# Example of try/catch
try {
Get-Item "nonexistentfile.txt"
} catch {
Write-Host "Error occurred: $_"
}
This code snippet attempts to get an item and gracefully handles any errors, ensuring the script can continue running as intended.
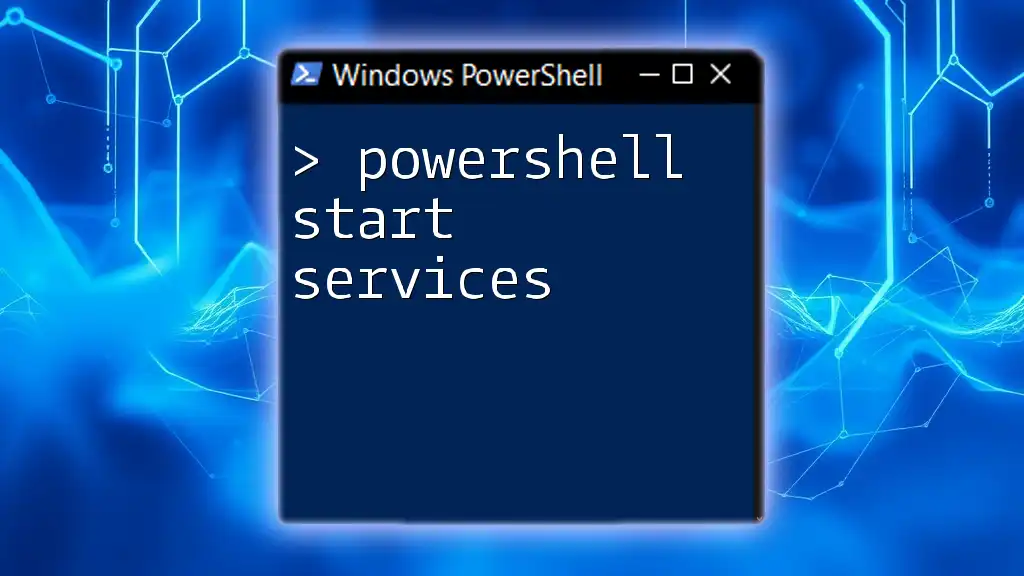
Conclusion
In an era of escalating cyber threats, implementing PowerShell security best practices is not just advisable—it is essential. By understanding the inherent risks, configuring user access controls, enabling logging, and ensuring secure connections, organizations can significantly enhance their security posture. Stay proactive, embrace these practices, and protect your environments against potential threats.