In PowerShell, you can start a service using the `Start-Service` cmdlet, which allows you to quickly activate any stopped service on your system.
Here's a code snippet to exemplify:
Start-Service -Name "YourServiceName"
Replace `"YourServiceName"` with the actual name of the service you wish to start.
What are Windows Services?
Windows Services are essential programs that run in the background on a Windows operating system. They are designed to perform specific functions without requiring direct user interaction. For instance, services can facilitate network communications, manage security protocols, or run scheduled tasks, ensuring that critical operations continue running smoothly even when a user is not logged in.
The ability to manage these services effectively can greatly enhance system performance and stability. Therefore, understanding how to use PowerShell to start services can significantly impact the way administrators maintain their systems.
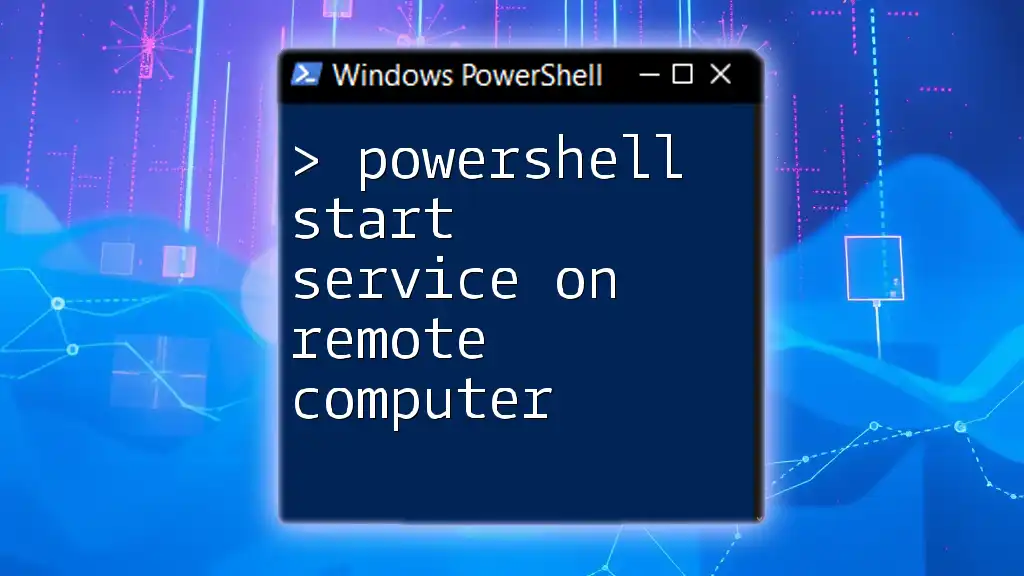
Understanding PowerShell
PowerShell is a powerful task automation and configuration management framework from Microsoft. It combines the functionality of the command line with a scripting language, allowing users to manage configurations, automate tasks, and handle system administration tasks efficiently. When it comes to service management, PowerShell makes it easy to start, stop, and check the status of Windows services.
PowerShell's Role in Service Management
PowerShell allows system administrators to interact with services through simple commands. This automation capability simplifies the management of services, particularly when dealing with many services or when ensuring certain services are running for applications to function correctly. Learning how to use PowerShell to start services is vital for any IT professional.
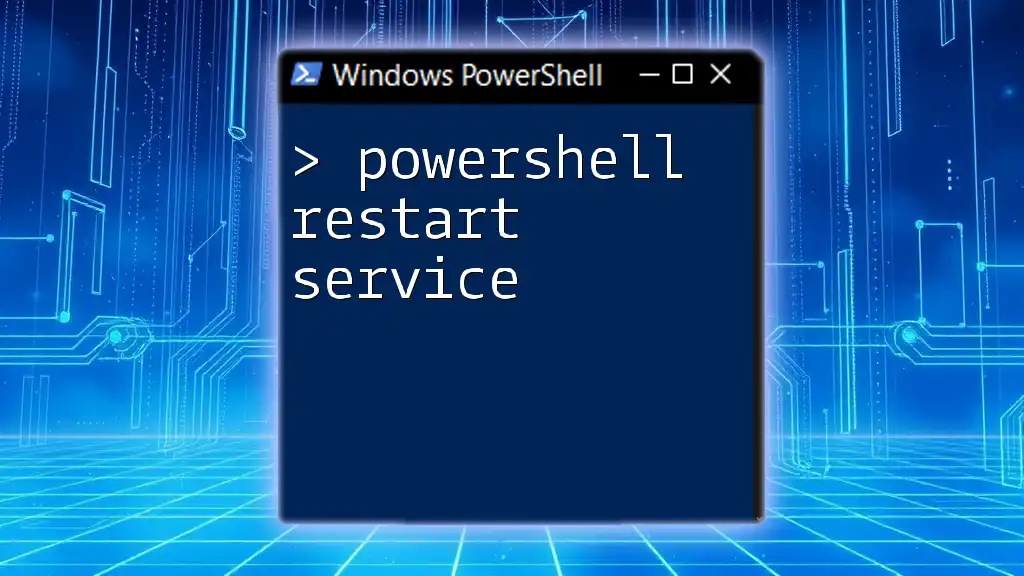
Starting Services with PowerShell
Basic Syntax for Starting Services
To start a service using PowerShell, you will primarily use the `Start-Service` cmdlet. This command is straightforward and allows you to specify the service you wish to start by name.
For example, if you want to start a service named "YourServiceName," you can use the following command:
Start-Service -Name "YourServiceName"
This single line of code initiates the service, provided that you have the necessary permissions to do so.
How to Identify the Service Name
Knowing the exact name of the service is crucial to successfully starting it. PowerShell provides the `Get-Service` cmdlet, which displays a list of all services and their statuses.
To see all running and stopped services, you can simply run:
Get-Service
To filter and find only those services that are stopped, use the `Where-Object` cmdlet:
Get-Service | Where-Object {$_.Status -eq 'Stopped'}
This command will give you a clean list of the services that are currently not running, making it easy to determine which ones you may want to start.
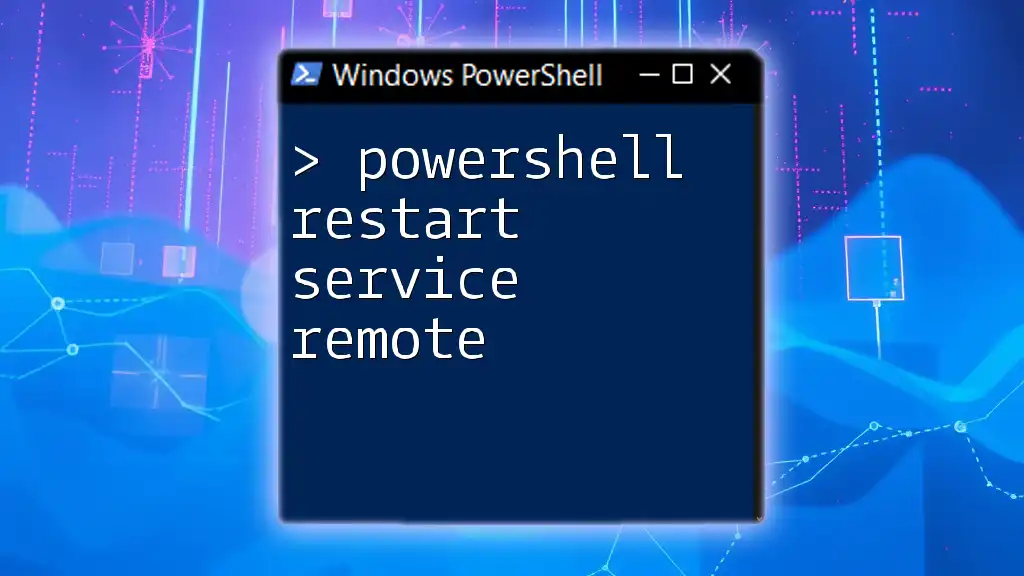
Starting Multiple Services
If you need to start multiple services quickly, you can utilize a PowerShell array to streamline the process. Define an array of services and iterate through them using `ForEach-Object` to start each one.
Here’s an example of how to accomplish this:
$services = "Service1", "Service2", "Service3"
$services | ForEach-Object { Start-Service -Name $_ }
In this example, replace "Service1," "Service2," and "Service3" with the names of the services you wish to start. This method allows you to manage multiple services efficiently in one command.
Handling Errors
When working with any script or commands, it’s essential to anticipate errors. You can use PowerShell’s `Try-Catch` structure to manage potential errors gracefully. This way, if the service fails to start, your script will handle it appropriately without crashing.
Here’s how you can implement error handling:
Try {
Start-Service -Name "YourServiceName"
}
Catch {
Write-Host "Failed to start service: $_"
}
This structure allows you to display a meaningful error message if the service does not start as expected, enhancing the robustness of your scripts.
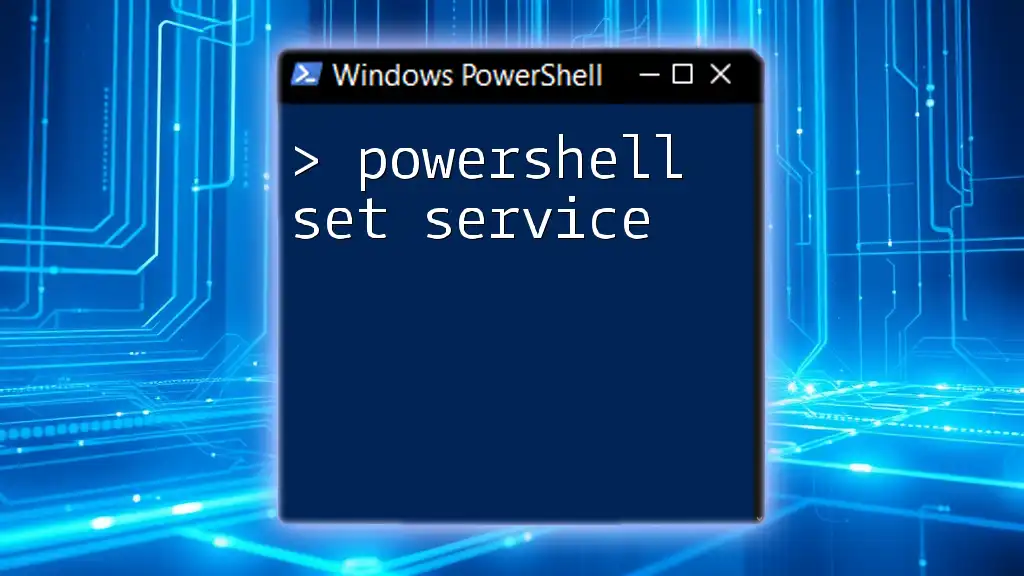
Confirming Service Start Status
After attempting to start a service, you should confirm that the service has started successfully. You can do this by using the `Get-Service` cmdlet again to check the status:
Get-Service -Name "YourServiceName" | Select-Object Status
This command will return the current status of the specified service, allowing you to verify if it is indeed running.
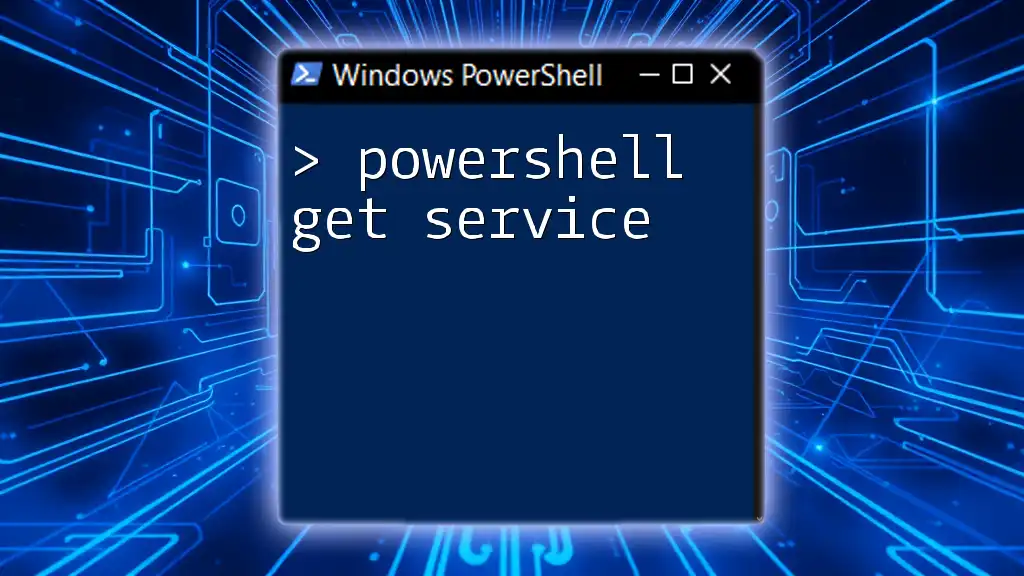
Automating the Process
To further increase efficiency, consider creating a PowerShell script for starting services. Automation allows you to perform routine tasks without manual input, saving valuable time in a busy operational environment.
Here’s a simple example of a script that starts multiple services:
# StartServices.ps1
$services = "Service1", "Service2"
foreach ($service in $services) {
Start-Service -Name $service -ErrorAction Stop
}
You can run this script whenever you need to start these services, making your workflow smoother.
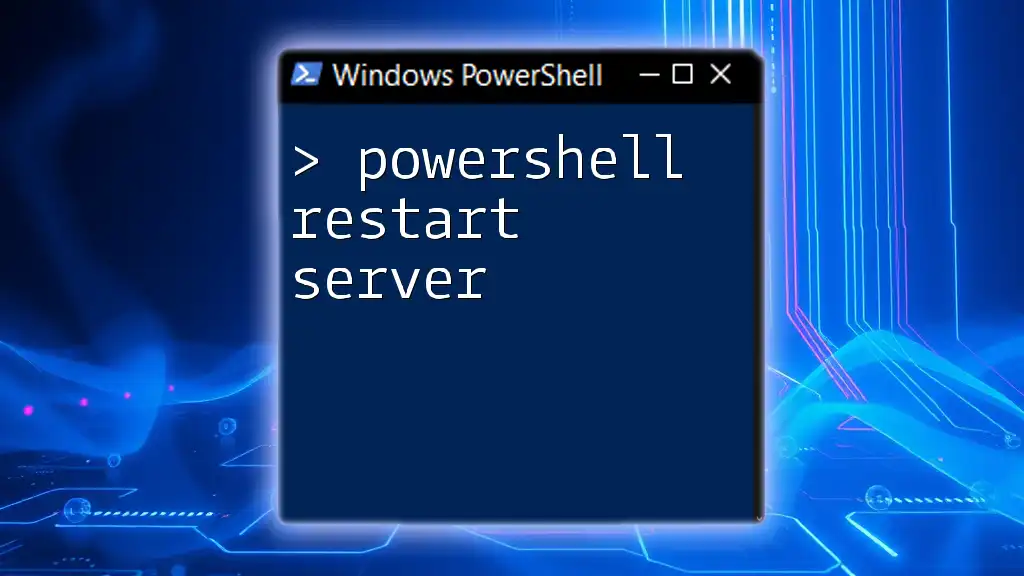
Best Practices for Managing Services
To maximize productivity and minimize issues related to service management, here are a few best practices:
-
Documenting Service Dependencies: Understanding which services depend on others is crucial for preventing system failures and ensuring that all necessary components are running.
-
Testing in Development Environments: Before applying changes or commands in production, always test them in a safe development environment. This practice allows you to catch and troubleshoot any issues that may arise without affecting live systems.
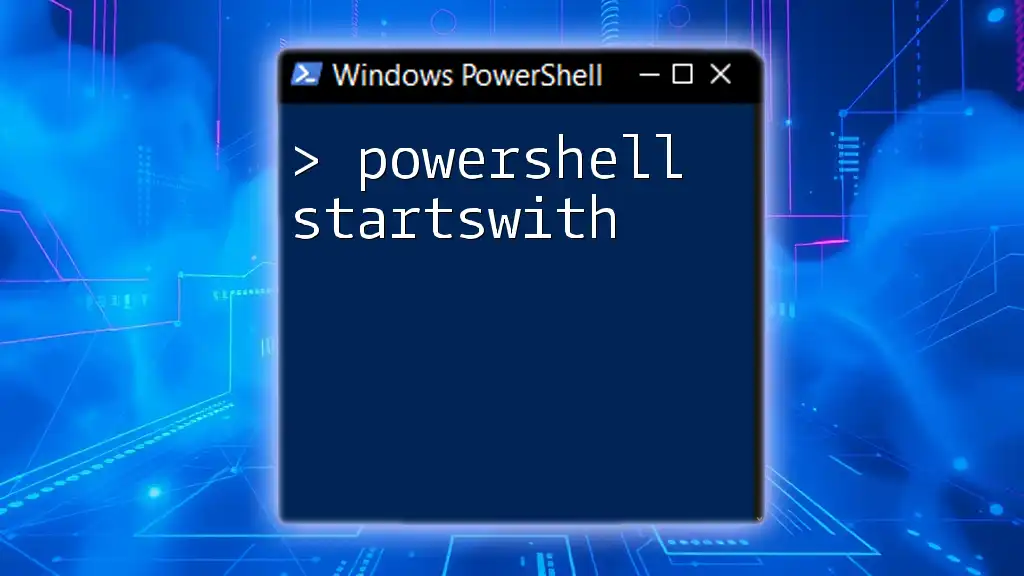
Conclusion
Mastering PowerShell start services commands not only enhances your system administration skills but also ensures that you can manage services effectively across different environments. As a PowerShell user, take time to practice these commands, explore their functionalities, and incorporate them into your daily tasks. As you become familiar with PowerShell, you’ll find many advanced capabilities that can streamline your workflow even further.
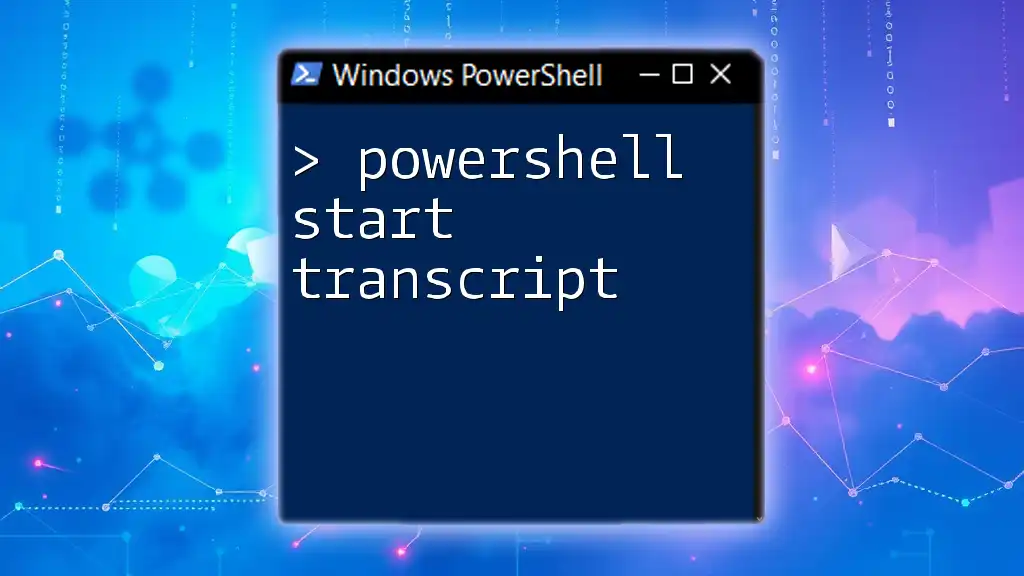
Additional Resources
For deeper insights and expand your PowerShell knowledge, consider reviewing the official Microsoft documentation or join PowerShell communities where you can learn from experienced users and professionals.
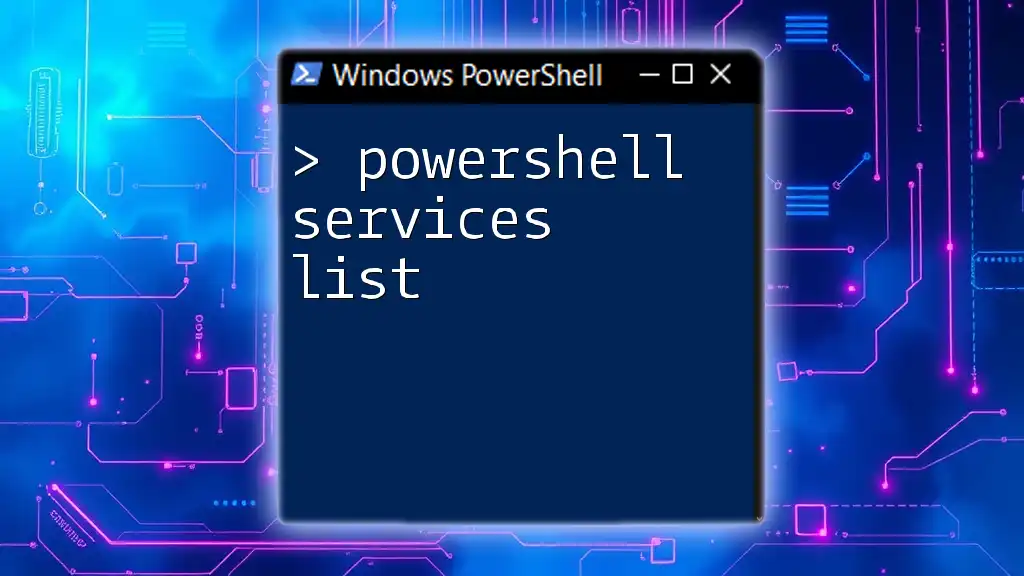
Call to Action
Interested in learning more about PowerShell? Sign up for our newsletters to receive tips, tricks, and exclusive resources. Don’t forget to download our free cheat sheet of commonly used PowerShell commands related to service management!