A PowerShell HTTP server allows you to quickly run a simple web server that can serve files over HTTP using minimal commands.
Here's a code snippet to set up a basic HTTP server that serves files from a specified directory:
# Change to the directory you want to serve
Set-Location 'C:\Path\To\Your\Directory'
# Start the HTTP server on port 8080
Start-Process powershell -ArgumentList "-NoProfile -Command 'while ($true) { Start-BitsTransfer -Source http://localhost:8080 -Destination \"C:\Path\To\Your\LogFile.txt\"; Start-Sleep -Seconds 10 }'" -WindowStyle Hidden
Make sure to modify `C:\Path\To\Your\Directory` to the directory you wish to serve and run this command in your PowerShell terminal.
Setting Up Your PowerShell HTTP Server
Prerequisites
Before diving into creating a PowerShell HTTP Server, ensure that your system meets the necessary requirements. You need to be running Windows PowerShell 3.0 or higher. To check your current PowerShell version, you can use the following command:
$PSVersionTable.PSVersion
If your version is lower than 3.0, you will need to upgrade PowerShell to proceed.
Starting the HTTP Server
To launch your very own PowerShell HTTP Server, you can use the built-in command `Start-HttpServer`. By default, this command runs the server on port 8080. Here's how to start the server:
Start-HttpServer -Port 8080
This command initiates a simple HTTP server that listens for incoming requests on port 8080. It is crucial to understand that the default settings will serve files from the current directory unless otherwise specified.
Customizing Server Settings
Specifying the Port
Although the default port (8080) is often sufficient for development, you might want to specify a different port. For instance:
Start-HttpServer -Port 5000
Running the command this way will allow your server to listen for requests on port 5000. Make sure to use a port that's not already in use by another application to avoid conflicts.
Setting the Base Directory
You can also set a base directory for your HTTP server. The `-Path` parameter allows you to designate a root folder from which files will be served. For example:
Start-HttpServer -Port 8080 -Path "C:\MyWebsite"
This command will serve files located in `C:\MyWebsite`. Be cautious when setting this path, as it directly affects what users can access through your server.
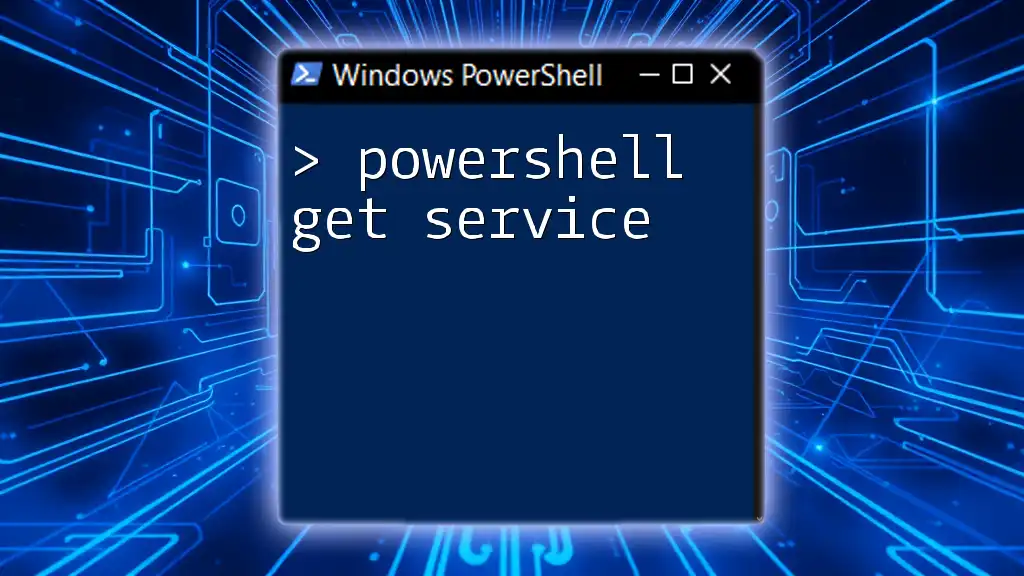
Handling Requests
GET Requests
GET requests are the most common type of HTTP request. They are used to request data from a specified resource. When your HTTP server receives a GET request, it will look to serve the requested file or data.
To test a GET request, use the following PowerShell command:
Invoke-RestMethod -Uri 'http://localhost:8080' -Method GET
If a file is served successfully, you will see its content in your PowerShell console. The response will typically be in a structured format (text, JSON, etc.), depending on the resource you accessed.
POST Requests
POST requests are used to send data to the server for processing, such as submitting forms. To demonstrate handling POST requests, use the example below:
$RequestBody = @{ name = "John Doe"; age = 30 }
Invoke-RestMethod -Uri 'http://localhost:8080' -Method POST -Body $RequestBody
This command sends a body containing a name and age to the server. The server can then process these details accordingly. Ensure that your server is set up to handle and respond to POST requests appropriately.
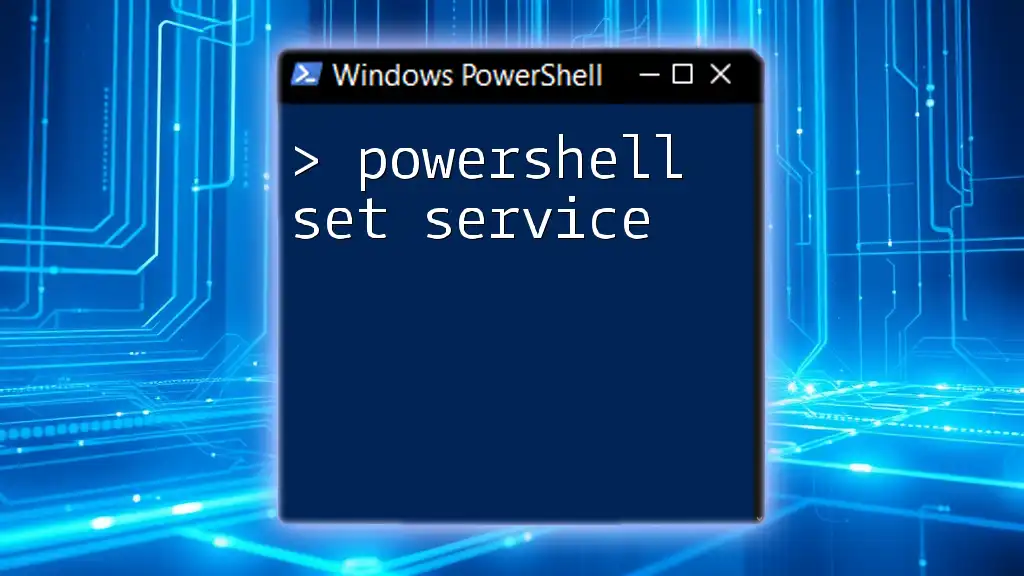
Advanced Configurations
Enabling SSL
For enhanced security, especially if you plan to transmit sensitive data, enabling SSL is advisable. You can do this by adding the `-Ssl` parameter:
Start-HttpServer -Port 443 -Ssl
This command configures your HTTP server to encrypt data transmitted over HTTPS, making your server more secure against potential eavesdropping.
Logging Requests
Logging requests is essential for monitoring traffic and debugging issues. To implement a simple logging mechanism, create a function that logs incoming requests:
function Log-Request {
param($request)
Add-Content -Path "C:\path\to\log.txt" -Value $request
}
Call this function within your HTTP handling logic to log each request to `log.txt`. This file will then serve as a record of all interactions with your server, helping you to diagnose issues or analyze usage patterns over time.
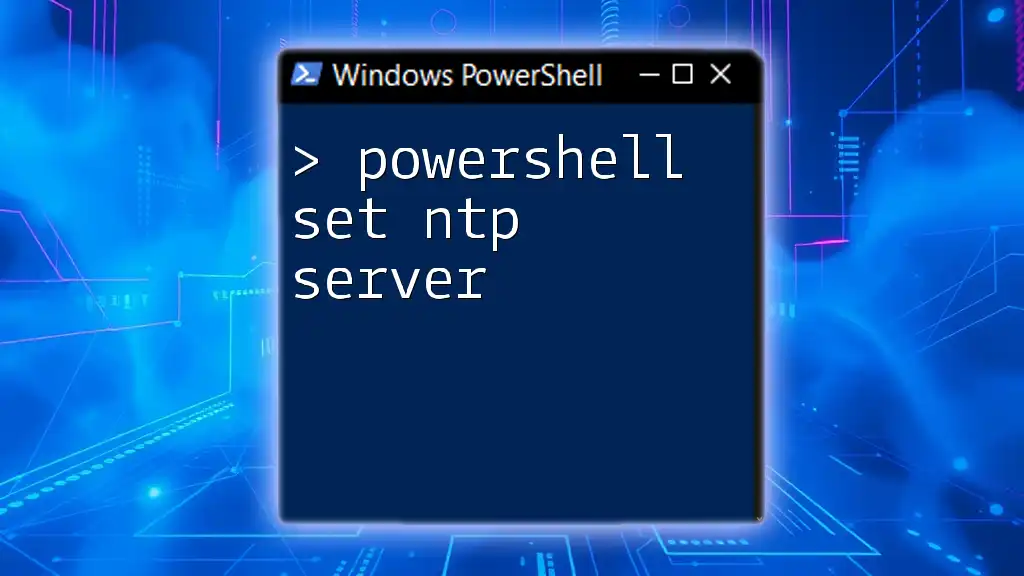
Troubleshooting Common Issues
Server Not Starting
If your PowerShell HTTP Server does not start, possible reasons could include:
- Port conflicts: Another application is already using the selected port.
- Insufficient permissions: Running PowerShell as a standard user might not grant the necessary access levels.
To resolve these issues, ensure the ports are free and try launching PowerShell with administrator privileges.
Request Timeouts
If you experience request timeouts, it may stem from:
- Network issues: Ensure your network is stable.
- Server performance: If your server is overloaded or misconfigured, it may struggle to respond in a timely manner.
Addressing these factors will enhance performance and reliability, ensuring a better user experience.
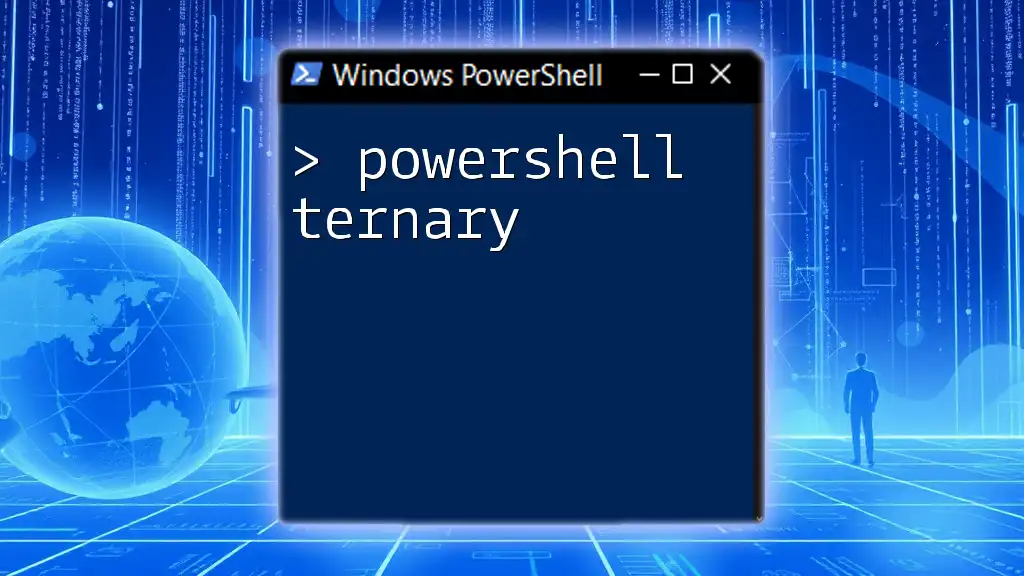
Conclusion
The PowerShell HTTP Server is a powerful yet straightforward tool for developers and testers alike. It allows for the rapid setup of an HTTP server for various scenarios, from testing APIs to serving static files. By customizing port numbers, handling different types of requests, and enabling advanced features like SSL and logging, you can create a robust development environment.
Experiment with the commands and configurations presented here to find the setup that works best for your needs. The flexibility and power of PowerShell will enable you to tailor your HTTP server to your specific requirements.
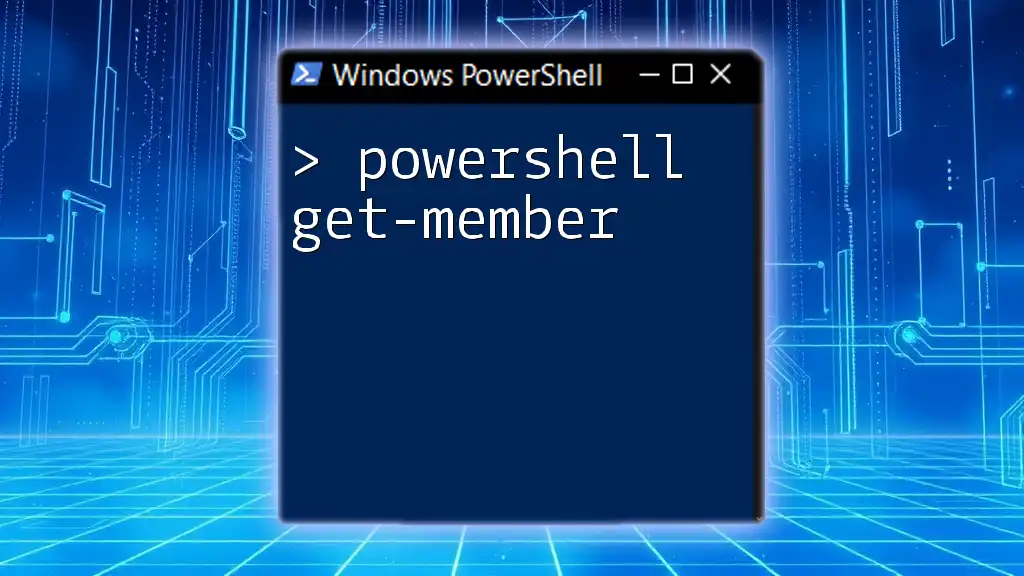
Call to Action
Do you have any experiences or questions related to setting up a PowerShell HTTP Server? Share them in the comments below! We encourage you to follow us for more useful PowerShell tips and tricks that can help elevate your scripting skills.