In PowerShell, you can create an infinite loop using a `while` loop that continuously runs until it's manually stopped, as shown in the code snippet below:
while ($true) { Write-Host 'Hello, World!' }
Infinite Loop in PowerShell
An infinite loop is a sequence of instructions in a program that runs continually without a terminating condition. This can be incredibly useful in scenarios where you need a script to operate continuously, such as monitoring system processes or listening for events until an external condition prompts it to stop.
Common Use Cases for Infinite Loops
Infinite loops are typically employed in various situations:
- Monitoring Systems: Running a script that checks the status of a service or resource and taking action based on that status.
- User Prompts: Creating interactive scripts that repeatedly ask for user input until valid data is provided.
- Continuous Data Collection: Gathering data from sensors or APIs without having to restart the script.
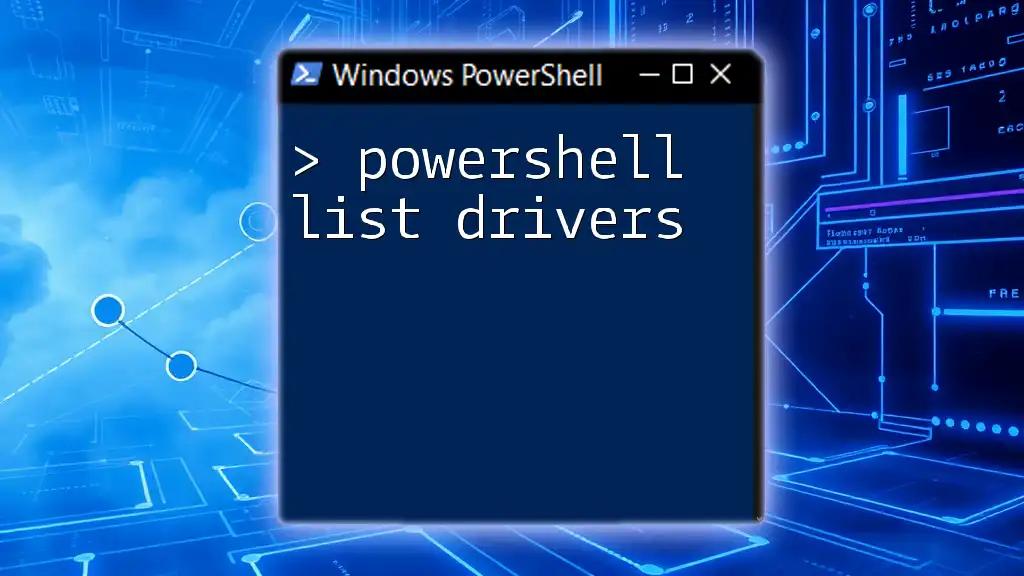
Syntax of PowerShell Infinite Loops
PowerShell provides various constructs to create infinite loops, the most common being `while`, `do-while`, and `for` loops.
While Loop
The basic structure of a `while` loop looks like this:
while ($true) {
# Code to execute forever
}
In this case, `$true` is a condition that always evaluates to true, thereby creating an infinite loop.
Example
Here is a simple PowerShell script that outputs "Running..." every 5 seconds:
while ($true) {
Write-Host "Running..."
Start-Sleep -Seconds 5
}
This loop will continue indefinitely until it is manually interrupted.
Do-While Loop
The `do-while` loop also enables the repeated execution of code perpetually as long as a condition remains true:
do {
# Code to execute forever
} while ($true)
Example
Here’s an example of a `do-while` loop that checks for system status every 10 seconds:
do {
Write-Host "Checking system status..."
Start-Sleep -Seconds 10
} while ($true)
Like the `while` loop, this structure will run until a termination condition is introduced.
For Loop
Though less common for infinite loops, a `for` loop can also be structured this way:
for ($i = 0; $true; $i++) {
# Repeat indefinitely
}
Example
This example demonstrates a `for` loop that increments a variable continuously:
for ($i = 0; $true; $i++) {
Write-Host "Loop iteration: $i"
Start-Sleep -Seconds 2
}
This will print the current iteration counter every 2 seconds indefinitely.

Best Practices for Implementing Infinite Loops
When using infinite loops, it's essential to adhere to several best practices to ensure efficient execution:
Avoiding Resource Drain
Infinite loops can consume excessive resources if not managed correctly. Always use `Start-Sleep` within your loop to pause execution, allowing the CPU to allocate resources to other processes. For example:
while ($true) {
# Perform necessary tasks
Start-Sleep -Seconds 5
}
This ensures your script doesn't overload the system.
Exit Conditions
Implementing an exit option is crucial. This could be as simple as a keyboard interrupt (Ctrl + C) that stops the loop or a specific command that breaks out of it. Always abstract the purpose of your loop to allow for a graceful termination.
Logging and Output
For any infinite loop, include logging features to track operations. This can help in troubleshooting and understanding the behavior of the script over time. For example:
while ($true) {
Write-Host "Logging event..."
Start-Sleep -Seconds 5
}
The logging becomes invaluable when monitoring tasks over extended periods.

Handling Exceptions in Infinite Loops
Including error handling within your loops is essential to prevent crashing:
Try-Catch Blocks
Using `try-catch` blocks allows you to handle exceptions gracefully without interrupting the operation of your loop:
while ($true) {
try {
# Code that may throw an exception
Write-Host "Performing a critical operation..."
} catch {
Write-Host "An error occurred: $_"
}
}
This design ensures that even if an error arises, your loop can continue running.
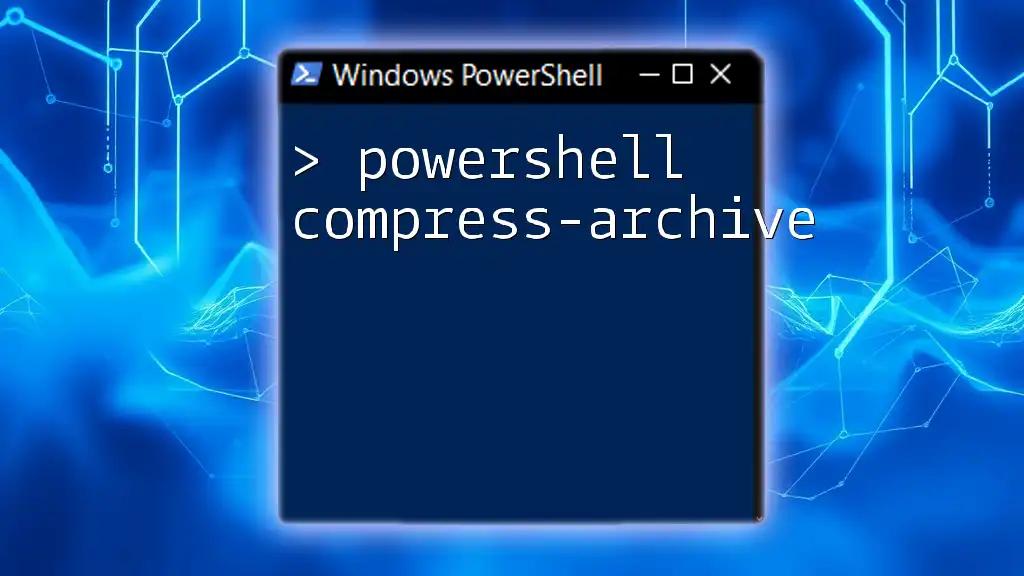
Performance Considerations
While infinite loops are beneficial, they can affect system performance. Consider the following:
Resource Management
Monitor the resource usage of your infinite loop scripts. Use PowerShell commands like `Get-Process` to ensure they are not causing unproportional memory or CPU usage.
Using Background Jobs
If a task can run independently, consider using background jobs. This allows the main script to remain responsive to other commands or user inputs without blocking:
Start-Job -ScriptBlock {
while ($true) {
Write-Host "Running background task..."
Start-Sleep -Seconds 5
}
}
This creates a separate job that runs concurrently, freeing up the main console for other activities.
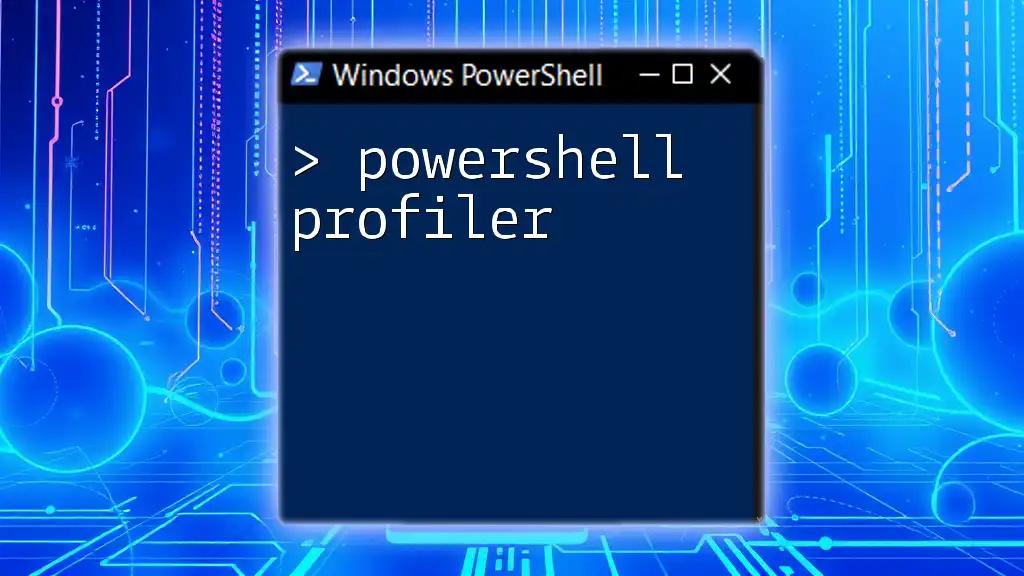
Conclusion
Utilizing a PowerShell loop forever strategy effectively opens up a variety of possibilities for automation and monitoring. However, it is paramount to understand how to manage and control these loops to maintain system integrity and performance. Experiment with the syntax and employ best practices to harness the full potential of your scripts while ensuring they remain efficient.
Explore more advanced error handling and logging methods to refine your infinite loop scripts further. The more you practice, the more adept you will become at using PowerShell for continuous automation!
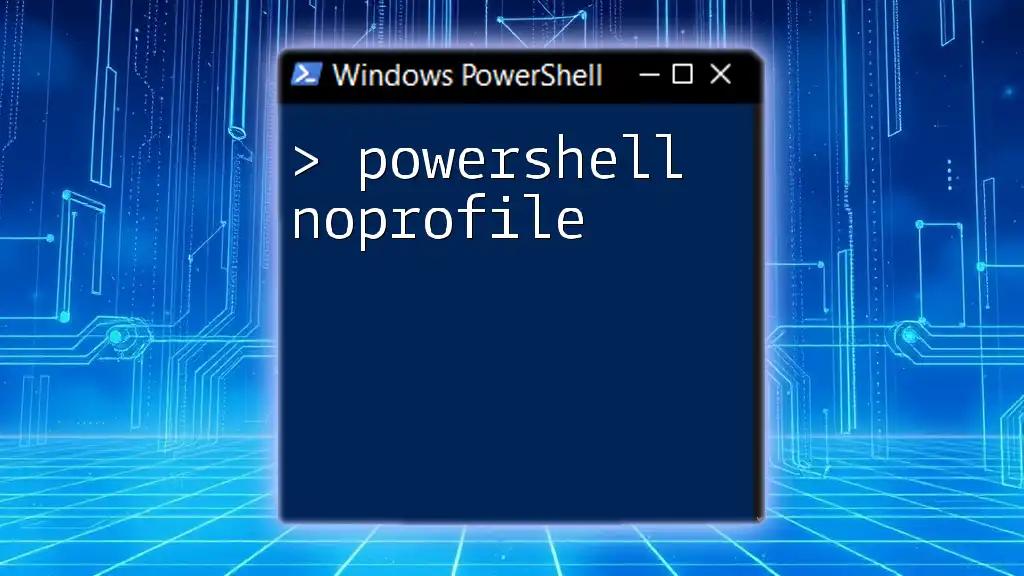
Additional Resources
To further your knowledge and skills in PowerShell, consider exploring:
- Official PowerShell documentation and community forums where you can connect with other enthusiasts.
- Tutorials and guides that delve deeper into automation techniques and PowerShell capabilities.
- PowerShell toolkits and libraries that can aid in your scripting journey.