In PowerShell, you can iterate through an array using a foreach loop to perform actions on each element efficiently. Here's a simple example demonstrating this:
$array = @(1, 2, 3, 4, 5)
foreach ($item in $array) {
Write-Host "Current item: $item"
}
Understanding PowerShell Arrays
What is an Array?
In PowerShell, an array is a collection of items that can be referenced through a single variable. Arrays in PowerShell are dynamic, allowing you to store elements of different types, including integers, strings, objects, and even other arrays. One important aspect to note is that PowerShell employs zero-based indexing, which means that the first element of the array is accessed with the index 0.
Creating an Array in PowerShell
Creating an array in PowerShell is straightforward. You can declare an array using the `@()` syntax. For example:
$myArray = @(1, 2, 3, 4, 5)
This code initializes an array named `$myArray` containing five integer elements. You can also dynamically add items to the array using the `+=` operator:
$myArray += 6
This line appends the value `6` to the end of the array.

PowerShell Looping Through an Array
The Need for Looping
Looping through arrays is essential for processing multiple data items efficiently. When you have a collection of data points, it’s often necessary to iterate through each element for tasks like transformation or data analysis. Using loops, you can manipulate arrays with minimal code and maximum flexibility.
Using a For Loop in PowerShell Array
Introduction to For Loops
The for loop is one of the most commonly used control structures in PowerShell for iterating through arrays. It gives you complete control over the iteration process by allowing you to specify the initialization, condition, and incrementer all in one line.
Here’s a basic structure of a for loop:
for ($i = 0; $i -lt $myArray.Length; $i++) {
Write-Output $myArray[$i]
}
In this example, `$i` initializes at `0`, and as long as `$i` is less than the length of the array, the loop continues. The variable `$i` increments by one each time the loop runs, which allows you to access each element of the array using the `$myArray[$i]` syntax.
Example: Looping with a For Loop
Consider the following scenario where we want to print each number in an array:
$myArray = @(10, 20, 30, 40, 50)
for ($i = 0; $i -lt $myArray.Length; $i++) {
Write-Output "Element $i: $($myArray[$i])"
}
This print statement will output:
Element 0: 10
Element 1: 20
Element 2: 30
Element 3: 40
Element 4: 50
It's clear how effective and efficient a for loop can be when you need to process data in sequential order.
The ForEach Loop in PowerShell
Understanding the ForEach Loop
The foreach loop simplifies the syntax for iterating through an array. It removes the overhead of managing the index variable by directly referencing each item in the array.
Here’s a typical foreach loop structure:
foreach ($item in $myArray) {
Write-Output $item
}
The `$item` variable in this loop represents the current element in `$myArray` for each iteration.
Example: Practical Use of ForEach Loop
Let’s illustrate looping through an array using a foreach loop to format and display a list of user names:
$userNames = @("Alice", "Bob", "Charlie", "Diana")
foreach ($user in $userNames) {
Write-Output "Welcome, $user!"
}
The output would be:
Welcome, Alice!
Welcome, Bob!
Welcome, Charlie!
Welcome, Diana!
This loop is particularly useful for its readability and simplicity, especially when working with collections.
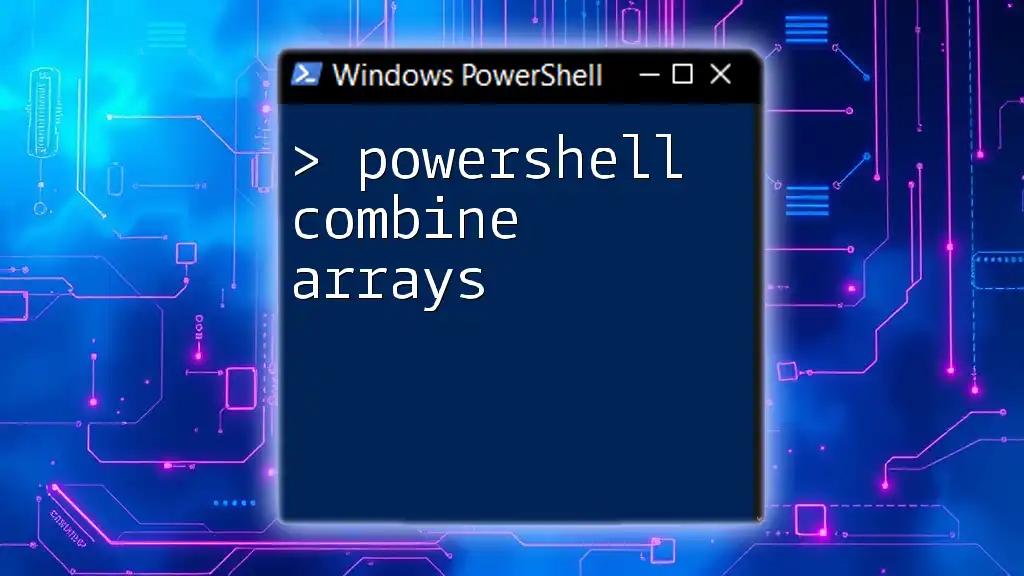
Alternative Looping Methods
Using While Loops
Another looping construct is the while loop, which continues executing as long as a specified condition remains true. It’s useful when you’re uncertain about the length of the array ahead of time.
$i = 0
while ($i -lt $myArray.Length) {
Write-Output $myArray[$i]
$i++
}
This loop starts with `$i` set to `0` and continues until `$i` is less than the length of the array, incrementing `$i` on each iteration to move to the next element.
Comparison of Loop Types
Each looping structure has its advantages and can be utilized depending on the specific requirements of your task:
- Use a for loop for precise control and when performing complex index manipulations.
- Use a foreach loop for simpler, more readable iterations over collections.
- Use a while loop when the exact number of iterations is unknown or when looping based on conditions rather than a clear index.
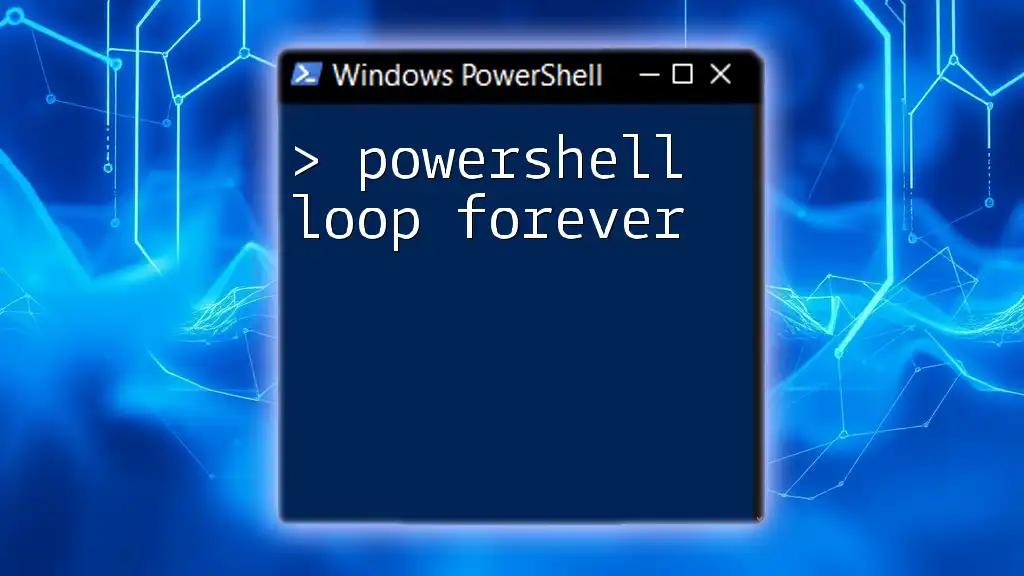
Best Practices for Looping Through Arrays
Optimization Techniques
When looping through arrays, it’s advisable to keep efficiency in mind. Avoid nested loops when possible, as they can considerably increase execution time, especially with large datasets. Instead, consider flattening your logic or using built-in array methods for data manipulation.
Readability and Maintainability
Write clear and concise looping structures. Ensure that your loops are well-documented with comments explaining their purpose. This practice significantly enhances the maintainability of your scripts, especially when revisited after some time.
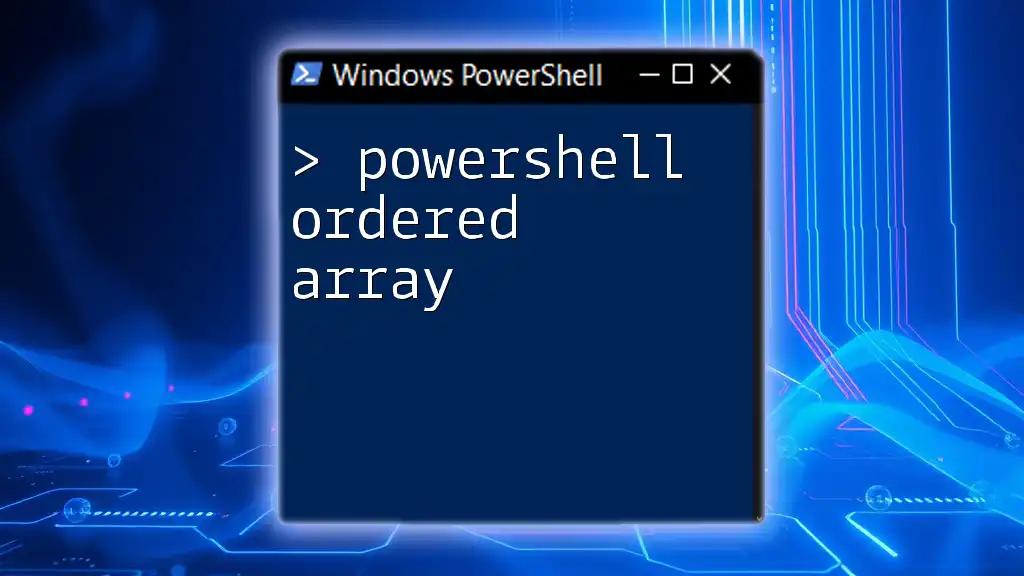
Common Errors and Troubleshooting
Common Mistakes in Looping
When working with loops, it’s easy to encounter common pitfalls such as off-by-one errors, where you either skip the first item or exceed the array length, leading to exceptions.
How to Debug Looping Issues
To debug issues, utilize PowerShell’s built-in debugging tools like `Write-Debug` and `Write-Verbose` for tracing execution. For example:
foreach ($item in $myArray) {
Write-Debug "Processing item: $item"
}
This line enables you to see what items are being processed at each iteration, making it easier to identify logical errors or inefficient loops.
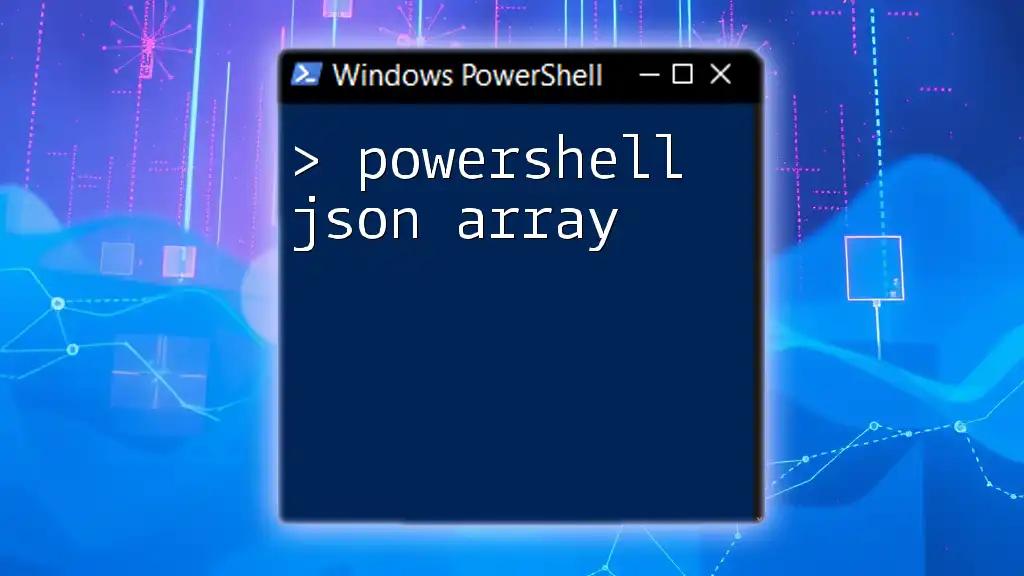
Conclusion
PowerShell’s ability to loop through arrays establishes it as a powerful tool for processing and managing data efficiently. Whether you choose a for loop, foreach loop, or while loop, understanding how to iterate through arrays will enhance your scripting capabilities and allow you to automate tasks effectively.
Equip yourself with these techniques and practice on your own arrays to become proficient in handling data through PowerShell. Engage with the PowerShell community, share your experiences, and collaborative learning will undoubtedly elevate your skills even further.