In PowerShell, you can check if a value is not in an array using the `-notcontains` operator, which returns `True` if the value is absent from the specified array.
Here's a code snippet demonstrating this:
$array = 1..5
$value = 6
if (-not ($array -contains $value)) {
Write-Host "$value is not in the array"
}
Understanding Arrays in PowerShell
What is an Array?
In PowerShell, an array is a collection of items stored together that allows you to manage multiple values efficiently. Arrays can be single-dimensional or multi-dimensional, enabling you to store lists, tables, or even complex objects. For example, to create a simple array containing fruits, you would do:
$fruits = @('Apple', 'Banana', 'Cherry')
This line initializes an array with three elements: Apple, Banana, and Cherry.
How to Populate Arrays
Populating arrays can be accomplished in various ways. You might add elements during initialization, as seen above, or leverage methods such as the `+=` operator to append items later:
$fruits += 'Dragonfruit'
This will add Dragonfruit to the existing array.
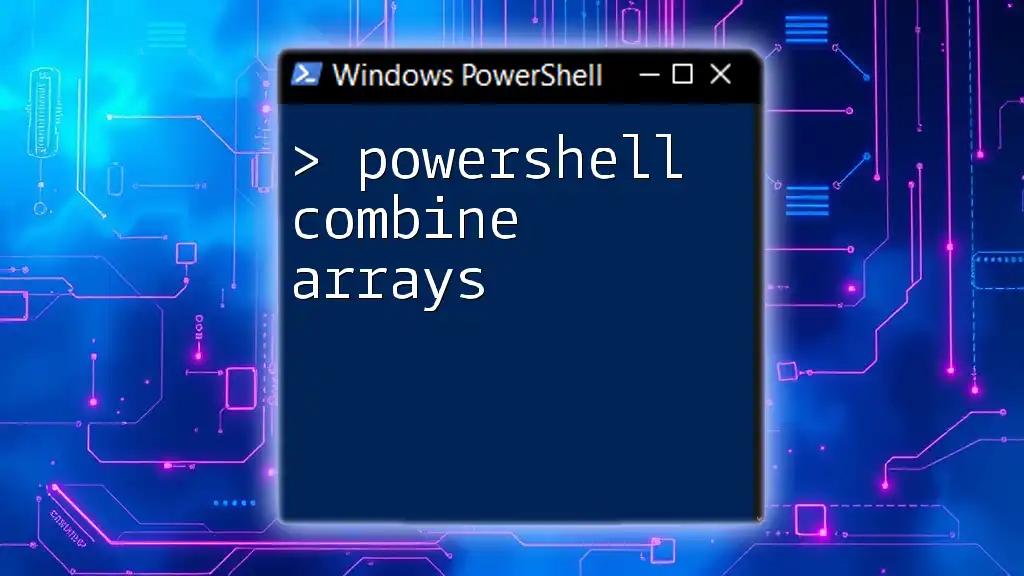
The Need for Logic: Checking Membership in Arrays
The Concept of 'In' and 'Not In'
When it comes to working with arrays, it's vital to understand how to check if a specific value exists. The `-contains` operator checks for existence, while `-notcontains` checks for non-existence. Here’s a basic usage demonstration:
if ($fruits -notcontains 'Grapes') {
Write-Output 'Grapes is not in the array.'
}
In this code snippet, the output confirms that Grapes is not part of the `$fruits` array.
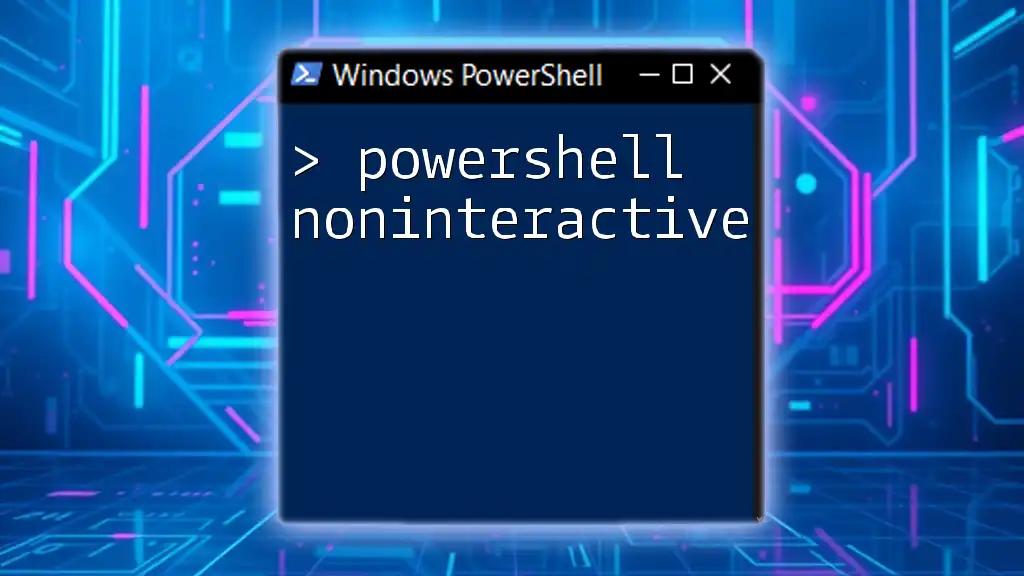
Practical Examples: Using PowerShell If in Array Logic
Checking if an Item is Not in an Array
Basic Example
Let’s dive deeper with a straightforward example. If you want to check for the presence of Orange, you can run:
$fruits = @('Apple', 'Banana', 'Cherry')
if ($fruits -notcontains 'Orange') {
Write-Output 'Orange is not found in the fruit list.'
}
The output will indicate that Orange is indeed not present in the array.
Using Variables with Conditionals
Using variables can significantly streamline your scripts. Suppose you want to confirm if a particular fruit is absent. You can assign a fruit to a variable and check as follows:
$myFruit = 'Mango'
if ($fruits -notcontains $myFruit) {
Write-Output "$myFruit is not in the array."
}
In this case, if Mango is not in the `$fruits` array, a message will be generated reflecting that.
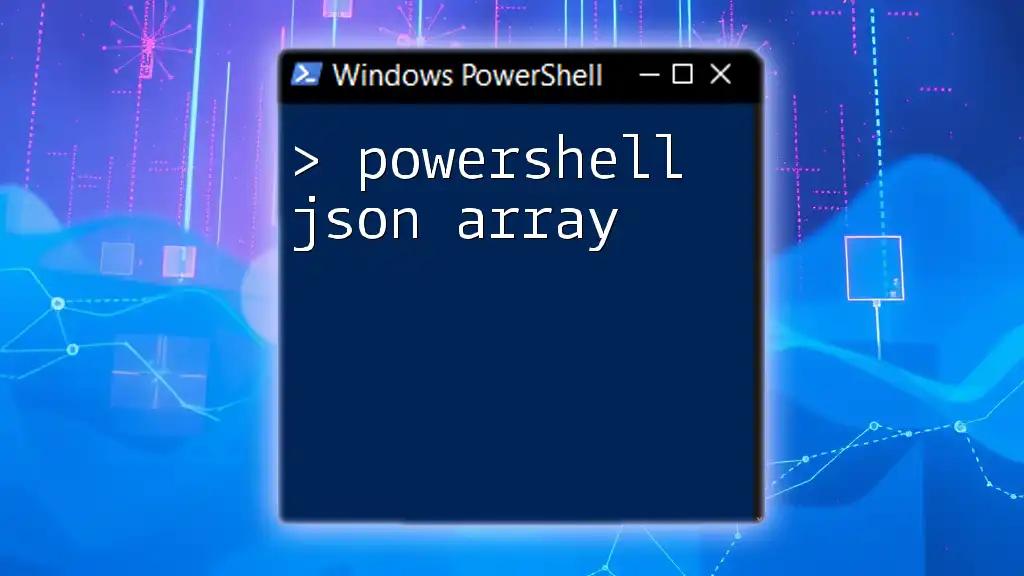
Advanced Logic with Arrays
Combining Conditions
Using Logical Operators
PowerShell allows you to combine multiple conditions to create more complex logic assessments. For example, if you want to check whether both Kiwi and Peach are absent, you can utilize logical operators:
if ($fruits -notcontains 'Kiwi' -and $fruits -notcontains 'Peach') {
Write-Output 'Neither Kiwi nor Peach is in the fruit list.'
}
This condition will evaluate to true only if both items are not present, demonstrating effective use of logical operators in PowerShell.
Checking Multiple Items
Iterating Over an Array
When checking multiple items against an array, using a loop can be incredibly effective. For instance, if you have a list of fruits to verify against the main `$fruits` array, you can do the following:
$checkItems = @('Grapes', 'Banana', 'Peach')
foreach ($item in $checkItems) {
if ($fruits -notcontains $item) {
Write-Output "$item is not in the fruit list."
}
}
In this scenario, the script will output messages for each fruit that is found to be absent from the `$fruits` array.

Common Pitfalls
Case Sensitivity
When dealing with strings in PowerShell, it’s important to remember that comparisons are case-sensitive by default. This means that checking for apple will not match Apple:
if ($fruits -notcontains 'apple') {
Write-Output 'This check is case sensitive.'
}
This snippet would output that apple is not in the array, even though Apple is present.
Mistakes in Usage
A common mistake is to confuse `-notcontains` and `-notin`. While `-notcontains` checks if an array does not include a specific value, `-notin` checks if a specific value is not in the array. Using them correctly is essential to achieving the desired functionality.

Conclusion
Understanding how to check for an item's presence or absence in an array using PowerShell is fundamental to effective scripting. Utilizing operators like `-notcontains` allows you to manage data efficiently and make decisions based on your criteria.
Whether you're a novice or an expert, mastering these commands will enhance your PowerShell expertise and ensure you can automate tasks more effectively. Engage actively with these concepts as you continue to develop your skills in scripting and automation!

Additional Resources
Documentation and References
For further reading, delve into the [official PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) for comprehensive insights and advanced syntax examples.
Community Support
The PowerShell community is vibrant and helpful. Engage on platforms like Reddit, PowerShell.org, or Stack Overflow to ask questions, share knowledge, and connect with like-minded individuals seeking to deepen their understanding of PowerShell.
Your Next Steps
Consider enrolling in specialized workshops or tutorials to further enrich your knowledge of PowerShell and automate tasks effectively. The journey of learning is ongoing, and by taking action, you'll pave the way for mastery in scripting!