In PowerShell, you can combine arrays using the `+=` operator or by using the `@()` array subexpression syntax for more complex combinations.
Here’s a code snippet illustrating both methods:
# Method 1: Using += operator
$array1 = @(1, 2, 3)
$array2 = @(4, 5, 6)
$combinedArray1 = $array1 + $array2
# Method 2: Using array subexpression
$combinedArray2 = @($array1) + @($array2)
Write-Host "Combined Array using Method 1: $combinedArray1"
Write-Host "Combined Array using Method 2: $combinedArray2"
What are Arrays?
Arrays are a fundamental data structure widely used in programming, including PowerShell. They provide a way to store multiple values in a single variable, enabling efficient data handling and manipulation. In PowerShell, arrays can hold various data types, including strings, numbers, and even complex objects, making them incredibly versatile and essential for scripting tasks.
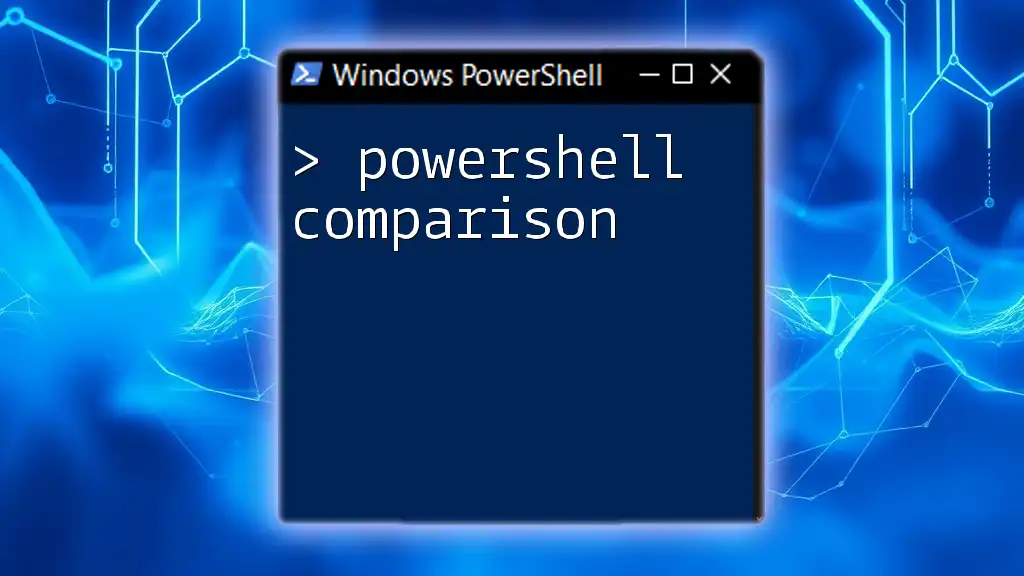
Why Combine Arrays?
Combining arrays in PowerShell is crucial for scenarios where you need to aggregate data from multiple sources or datasets. For example, merging lists of users, file paths, or configuration settings enhances your scripts' effectiveness and readability. By utilizing array combination techniques, you can also optimize performance, ensuring that your scripts run smoothly and efficiently.
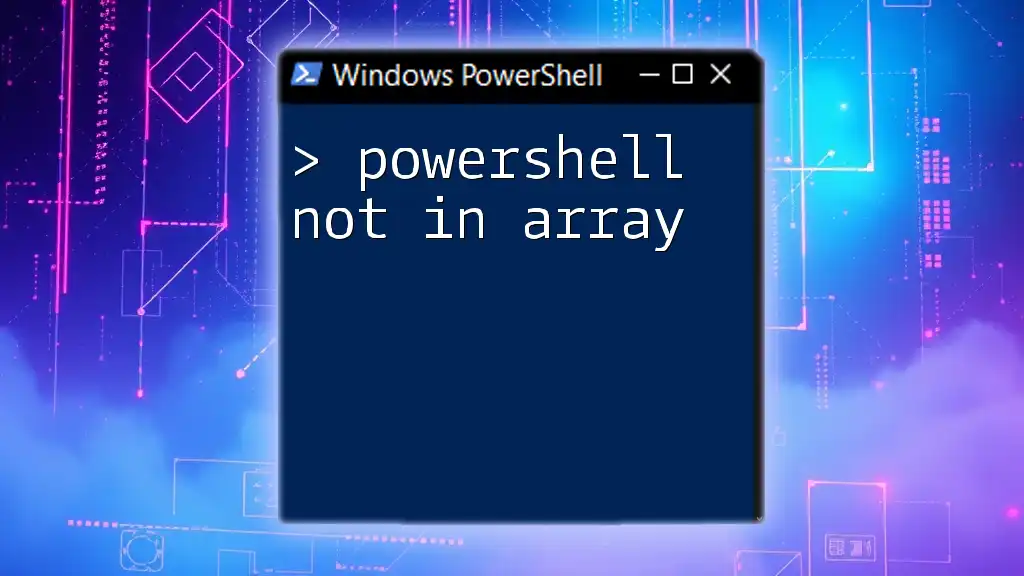
Creating Arrays in PowerShell
Creating Arrays in PowerShell
Creating arrays in PowerShell is straightforward. You can declare an array using the `@()` syntax. Here’s how you can create simple arrays:
# Single-dimensional array
$singleArray = @(1, 2, 3, 4, 5)
# Multi-dimensional array
$multiArray = @(@('a', 'b'), @(1, 2, 3))
Accessing Array Elements
Accessing elements within an array is done using zero-based indexing. You can reference an element by its index:
# Accessing elements
$secondElement = $singleArray[1] # This will return '2'
You can also modify an element:
# Modifying an element
$singleArray[2] = 10 # Changes the third element from 3 to 10
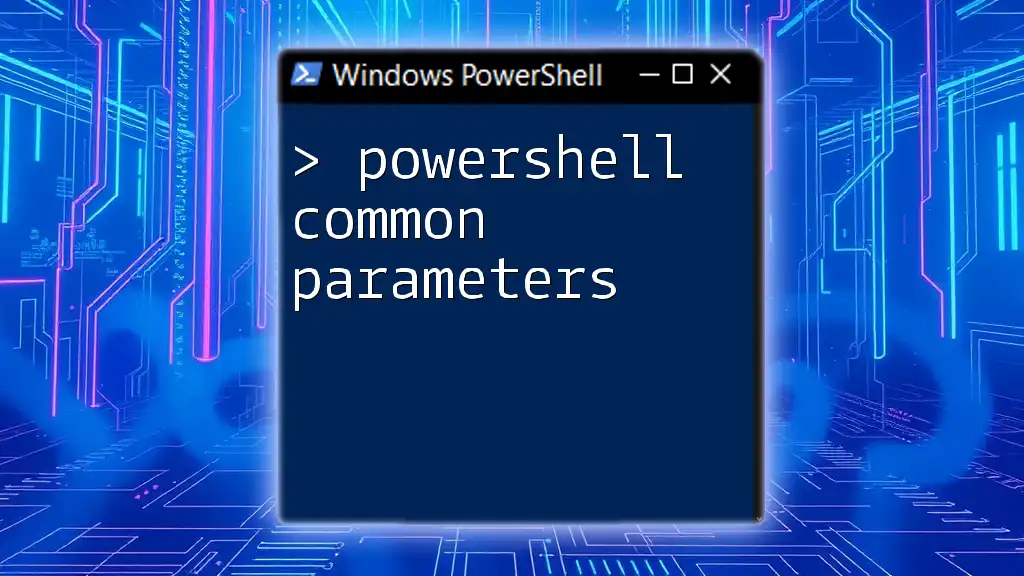
Methods to Combine Arrays in PowerShell
Using the `+=` Operator
One of the simplest ways to combine arrays in PowerShell is by using the `+=` operator. This operator appends elements to an existing array. Although easy to use, keep in mind that this method can become inefficient with larger datasets due to the way it operates internally, potentially creating a new array each time:
# Combine arrays using the += operator
$array1 = @(1, 2, 3)
$array2 = @(4, 5)
$array1 += $array2 # $array1 now contains 1, 2, 3, 4, 5
Using the `ArrayList` Class for Merging
The `ArrayList` class can be an optimal solution when you want to combine arrays without the overhead of using `+=`. An `ArrayList` is a dynamic array which allows you to add elements efficiently:
# Using ArrayList to combine arrays
$arrayList = New-Object System.Collections.ArrayList
$arrayList.AddRange($array1) # Adds elements of array1
$arrayList.AddRange($array2) # Adds elements of array2
# Convert back to array if needed
$combinedArray = $arrayList.ToArray()
Utilizing the `+` Operator
Another straightforward approach to merging arrays is by using the `+` operator, which concatenates two arrays into a new array. This method does not modify the original arrays:
# Merging arrays using the + operator
$combinedArray = $array1 + $array2 # Creates a new array containing elements from both arrays
Using `Join-Path` for Array Elements
When working with file paths, the `Join-Path` cmdlet is an excellent choice for combining string elements within arrays. It ensures that path formats are correct and is especially useful when merging directory paths:
# Example of using Join-Path
$basePath = 'C:\Users\Example'
$subFolders = @('Documents', 'Pictures')
$fullPaths = foreach ($folder in $subFolders) {
Join-Path -Path $basePath -ChildPath $folder
}
Exploring the `ForEach` Loop for Custom Combinations
For situations requiring more complex combinations, utilizing the `ForEach` loop can provide flexibility. You can iterate through each array and compose the combinations as needed:
# Combines arrays using ForEach
$additionalArray = @(7, 8)
$combinedArray = @()
foreach ($element in $array1) {
$combinedArray += $element
}
foreach ($element in $additionalArray) {
$combinedArray += $element
}
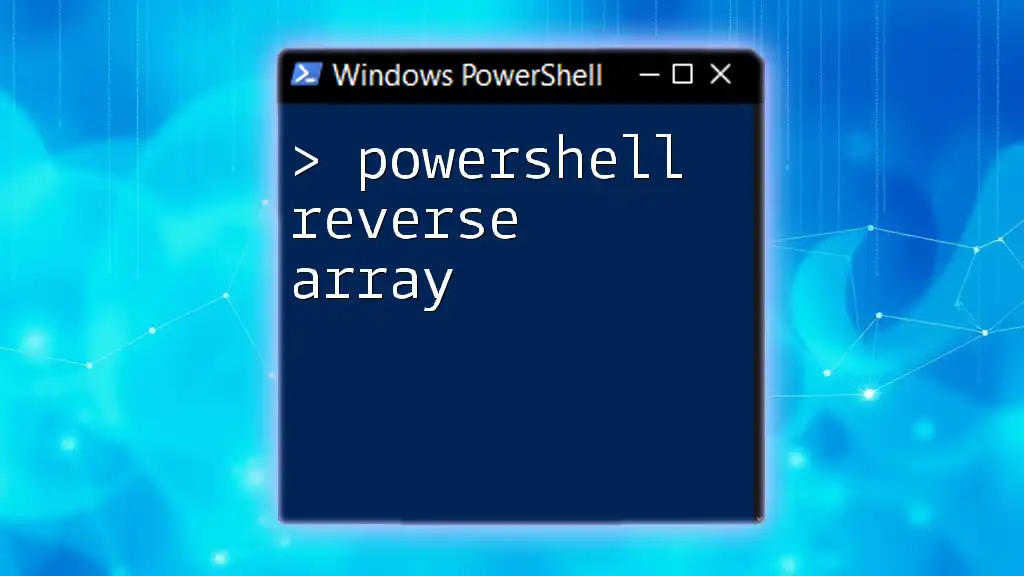
Advanced Techniques for Array Manipulation
Creating a Function to Merge Arrays
Creating a reusable function for merging arrays can streamline your scripts. Here’s how you can define a simple function:
function Combine-Arrays {
param (
[Array]$arr1,
[Array]$arr2
)
return $arr1 + $arr2
}
# Example usage
$newArray = Combine-Arrays -arr1 $array1 -arr2 $array2
Handling Duplicate Values when Combining Arrays
In some cases, duplicates may arise during the merging process. You can manage duplicates using `Where-Object`, filtering out any repeated values:
$combinedArray = $array1 + $array2
$distinctArray = $combinedArray | Select-Object -Unique # Removes duplicates
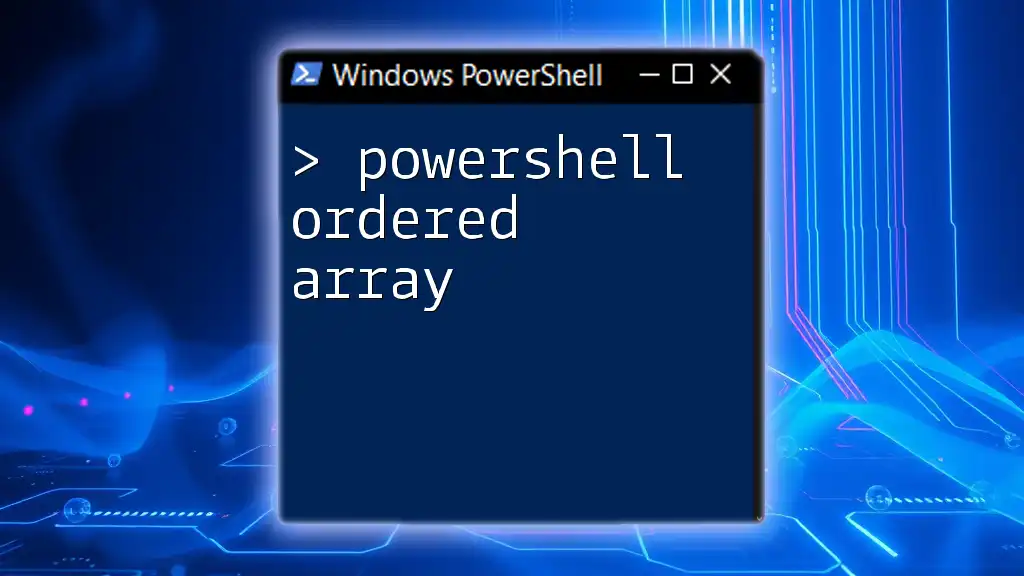
Practical Examples of Combining Arrays
Combining Two User-Defined Arrays
Let’s consider a practical example where you combine two arrays defined by user input:
# Collecting user input for two arrays
$array1 = Read-Host 'Enter comma-separated values for the first array' -split ','
$array2 = Read-Host 'Enter comma-separated values for the second array' -split ','
$combinedArray = $array1 + $array2
Write-Host "Combined Array: $($combinedArray -join ', ')"
Using Arrays to Create Complete File Paths
This example showcases how to effectively utilize arrays when generating complete file paths:
$basePath = 'C:\Projects'
$projectFolders = @('ProjectA', 'ProjectB')
$fullPaths = foreach ($folder in $projectFolders) {
Join-Path -Path $basePath -ChildPath $folder
}
Write-Host "Combined Paths: $($fullPaths -join ', ')"
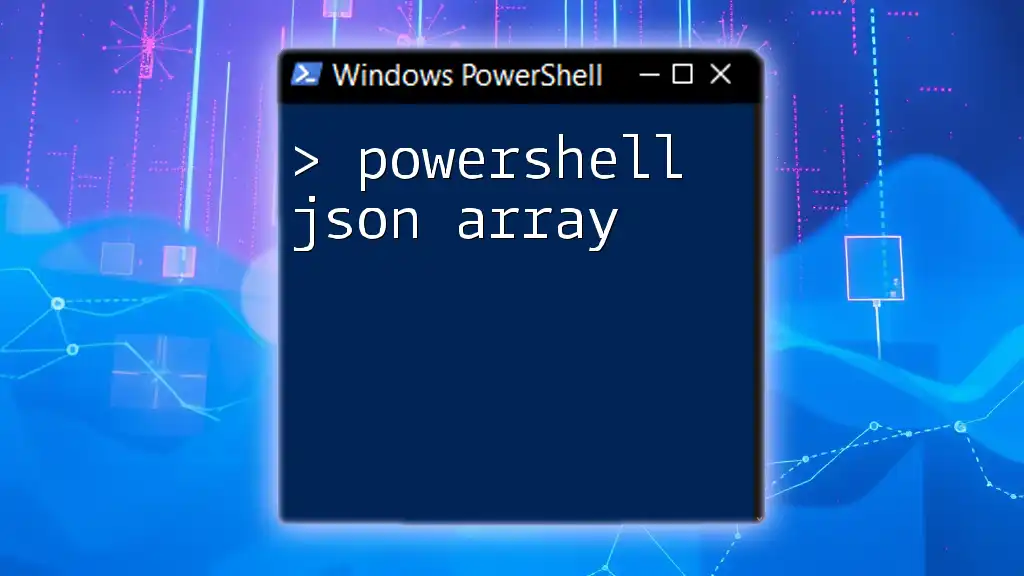
Performance Considerations
When combining arrays in PowerShell, it's essential to consider the efficiency of different methods. The `+=` operator, while helpful for smaller arrays, can lead to performance issues with larger datasets due to its underlying mechanics. In contrast, using `ArrayList` tends to be more efficient as it allows for dynamic resizing. If you're working with large amounts of data, opt for methods like `+` or `AddRange` with `ArrayList` to minimize resource consumption.
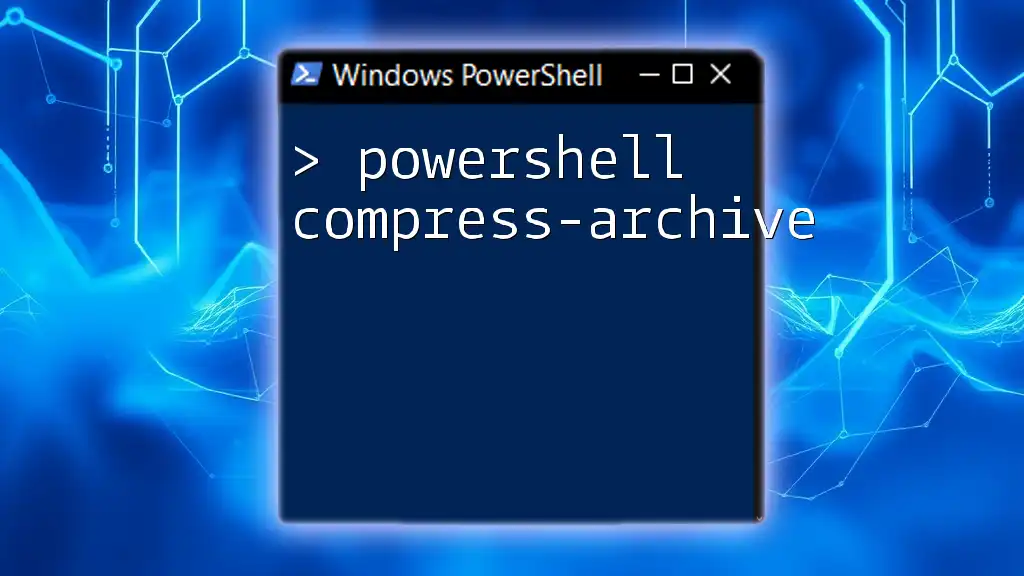
Summary of Key Points
In this article, we explored the various techniques and methods to PowerShell combine arrays efficiently. Whether you choose to use simple concatenation operators, the `ArrayList` class, or even custom functions, understanding how to manipulate arrays can significantly enhance your scripting skills.
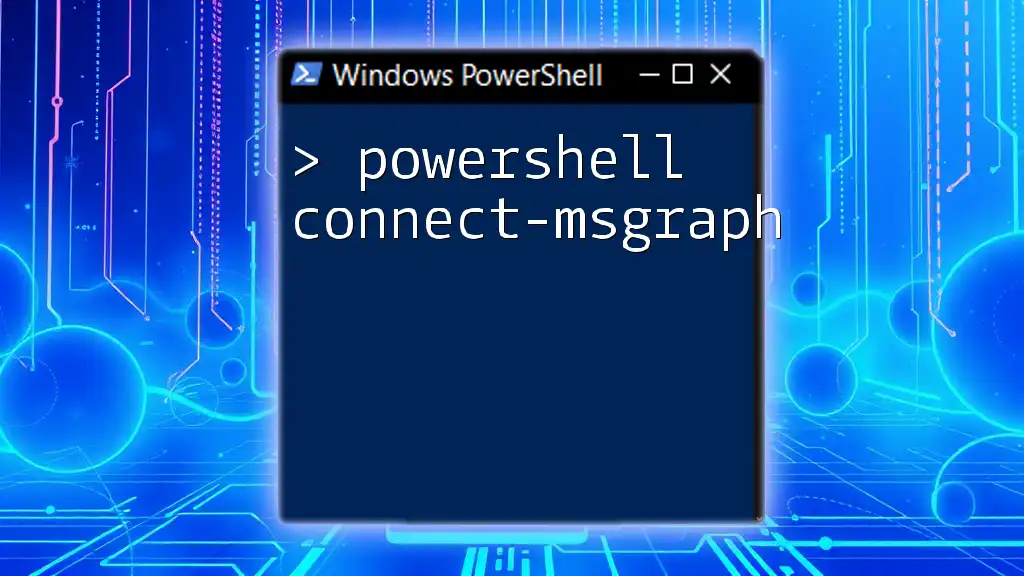
Join Us to Learn More
If you're eager to dive deeper into the world of PowerShell and enhance your skills further, join us for more in-depth tutorials and workshops tailored for all levels of learners.