In PowerShell, you can compare the contents of two directories to identify differences in files and subfolders using the `Compare-Object` cmdlet along with `Get-ChildItem`.
Compare-Object (Get-ChildItem 'C:\Path\To\Directory1') (Get-ChildItem 'C:\Path\To\Directory2')
Why Use PowerShell for Directory Comparison?
Using PowerShell for directory comparison provides significant speed and efficiency compared to graphical user interface (GUI) methods. When working with directories that contain a large number of files, navigating and comparing them in a GUI can be cumbersome and slow. In contrast, using commands directly in PowerShell allows you to quickly initiate comparisons and obtain results with minimal fuss.
PowerShell enables automation, meaning you can set up scripts that can be reused and executed without manual intervention each time you need to compare directories. This is especially beneficial in scenarios where directory content is constantly changing or requires regular monitoring.
The flexibility of PowerShell cannot be overlooked. With a diverse range of cmdlets and the capability to pipe commands together, you have the power to customize your directory comparisons according to your unique requirements.
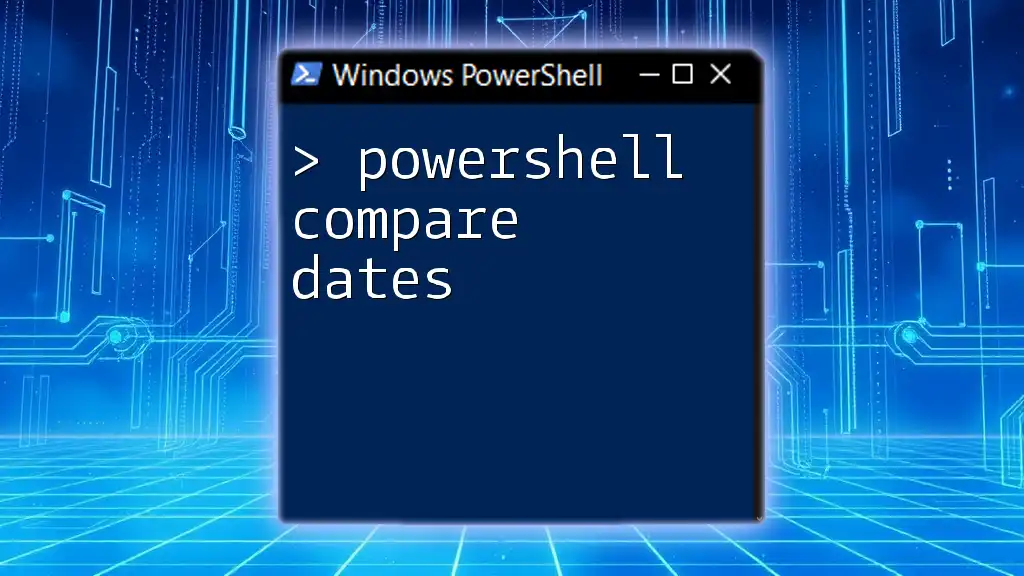
Getting Started with PowerShell
Setting Up PowerShell
Before embarking on your PowerShell journey, you may need to set it up. On Windows, PowerShell is typically pre-installed. For Mac and Linux users, you can download PowerShell Core from the official Microsoft documentation. To open PowerShell, simply search for "PowerShell" in the start menu on Windows, or use the terminal on macOS or Linux with the command `pwsh`.
Basic PowerShell Commands
Understanding the cmdlets in PowerShell is crucial. Cmdlets are built-in functions and can be invoked with specific syntax to perform a variety of tasks related to file and directory management. Utilize `Get-Help` to learn more about a specific cmdlet:
Get-Help Compare-Object
This command provides detailed information about the `Compare-Object` cmdlet, including syntax, parameters, and usage examples.
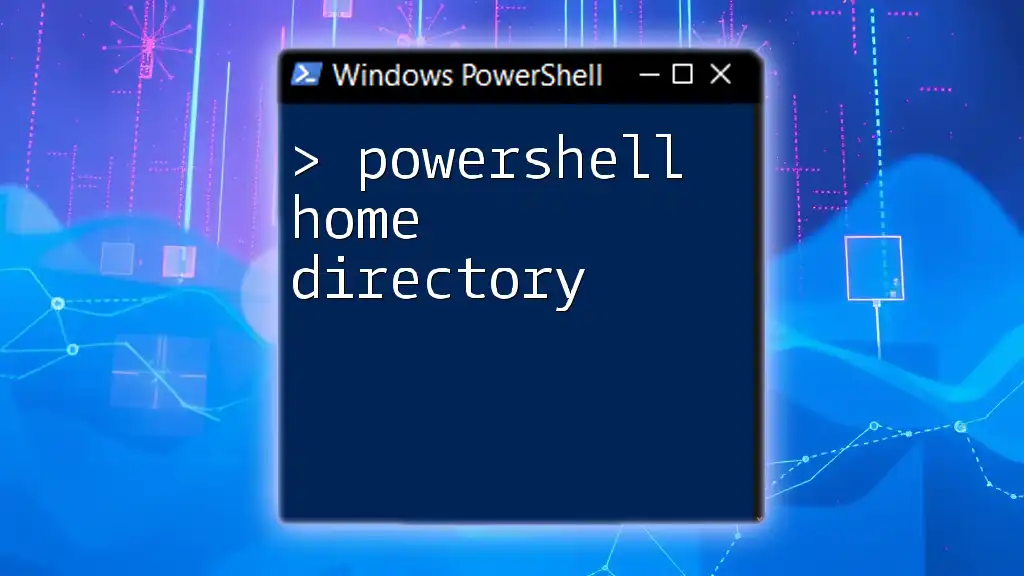
Comparing Directories
Using `Compare-Object`
A powerful tool for directory comparisons in PowerShell is the `Compare-Object` cmdlet. This cmdlet takes two objects and identifies differences between them. The basic syntax appears as follows:
Compare-Object -ReferenceObject $dir1 -DifferenceObject $dir2
Example: Basic Comparison
To compare two directories, you first need to gather their contents. The `Get-ChildItem` cmdlet retrieves the files and directories within a specified folder. Here is an example:
$dir1 = Get-ChildItem "C:\Path\To\Directory1"
$dir2 = Get-ChildItem "C:\Path\To\Directory2"
Compare-Object -ReferenceObject $dir1 -DifferenceObject $dir2
Understanding the Output
When you execute the above command, PowerShell will produce an output indicating which items are unique to each directory. The symbols `=>` and `<=` denote differences, with `=>` showing items unique to the second directory and `<=` indicating items unique to the first.
Comparing Files in Two Directories
Comparing files specifically can provide more refined insights, especially when checking for missing or modified files.
Example: File-Specific Comparison
You can adjust your comparison to focus on files within the directories as follows:
$filesDir1 = Get-ChildItem "C:\Path\To\Directory1" -File
$filesDir2 = Get-ChildItem "C:\Path\To\Directory2" -File
Compare-Object -ReferenceObject $filesDir1 -DifferenceObject $filesDir2
This command filters the output to only include files, omitting directories. You can also include specific file attributes for more tailored comparisons.
Matching Files by Name, Size, and Date
To compare files based on name, size, and last modified date, utilize the `-Property` parameter. Here's an advanced example:
Compare-Object -ReferenceObject $filesDir1 -DifferenceObject $filesDir2 -Property Name, Length, LastWriteTime
The output will reveal discrepancies between the selected properties, aiding in tracking file changes across directories.
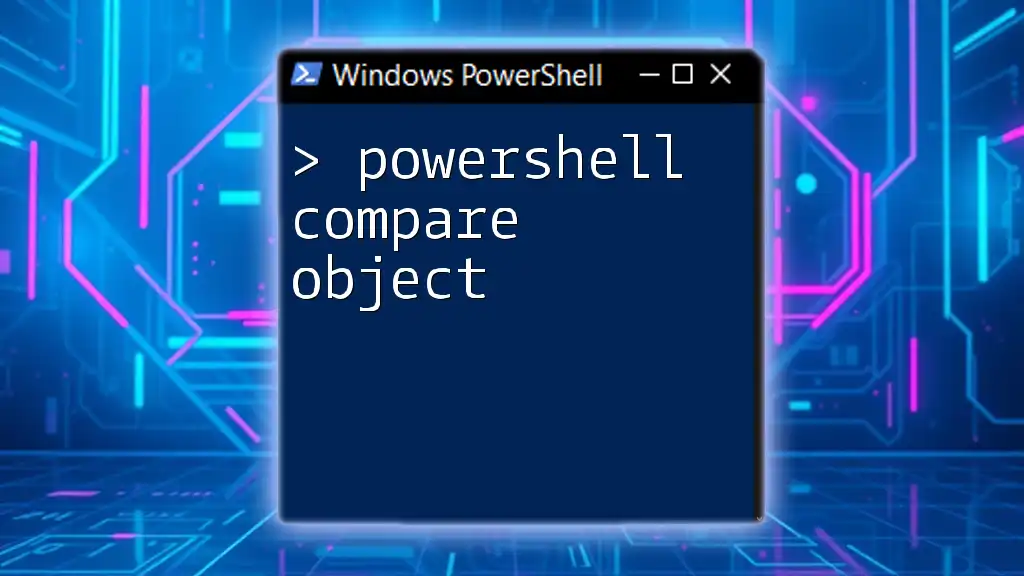
Additional Comparison Methods
Using `Get-ChildItem` and `Where-Object`
To refine your comparisons further, consider filtering files through `Where-Object`. This is particularly useful when you want to limit the comparison to specific criteria, such as file size or modification date.
$dir1Files = Get-ChildItem "C:\Path\To\Directory1" | Where-Object { $_.Length -gt 1MB }
$dir2Files = Get-ChildItem "C:\Path\To\Directory2" | Where-Object { $_.Length -gt 1MB }
Compare-Object -ReferenceObject $dir1Files -DifferenceObject $dir2Files
This command will only compare files larger than 1MB, allowing for a more targeted analysis of your directories.
Utilizing Checksum for File Comparison
For an in-depth file comparison, consider using checksums. A checksum is a computed value that can confirm whether files are identical, as it changes when the content of the file changes.
Examples of Using MD5 or SHA256
Here’s how you can calculate and compare the checksums for two files:
$hash1 = Get-FileHash "C:\Path\To\Directory1\file.txt" -Algorithm SHA256
$hash2 = Get-FileHash "C:\Path\To\Directory2\file.txt" -Algorithm SHA256
if ($hash1.Hash -eq $hash2.Hash) {
"Files are identical."
} else {
"Files are different."
}
Using checksums is especially useful for verifying integrity after file transfers or backups, providing an extra layer of assurance that your files are consistent.
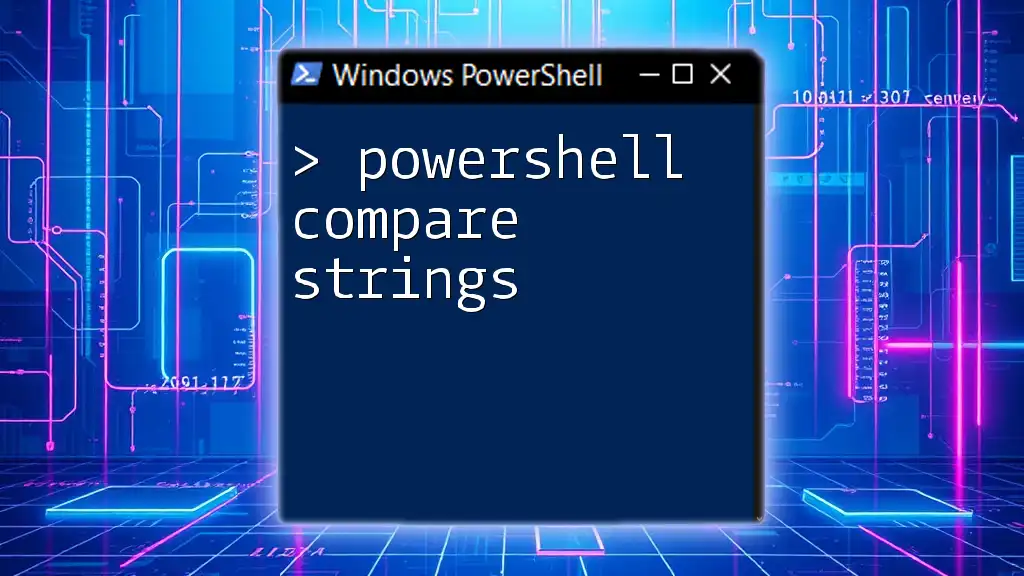
Automating Directory Comparisons
Creating a PowerShell Script
To improve efficiency, you can package your comparison commands into a reusable PowerShell script. A basic structure for a comparison script would look like this:
param (
[string]$Dir1,
[string]$Dir2
)
$dir1Files = Get-ChildItem $Dir1
$dir2Files = Get-ChildItem $Dir2
Compare-Object -ReferenceObject $dir1Files -DifferenceObject $dir2Files
This script takes two directories as parameters, compares their contents, and outputs the differences.
Scheduling the Script
Automation doesn’t stop at script creation. You can schedule your PowerShell script to run at designated intervals using Windows Task Scheduler. This includes setting the conditions for when to execute, ensuring your directory comparisons occur without manual prompting.
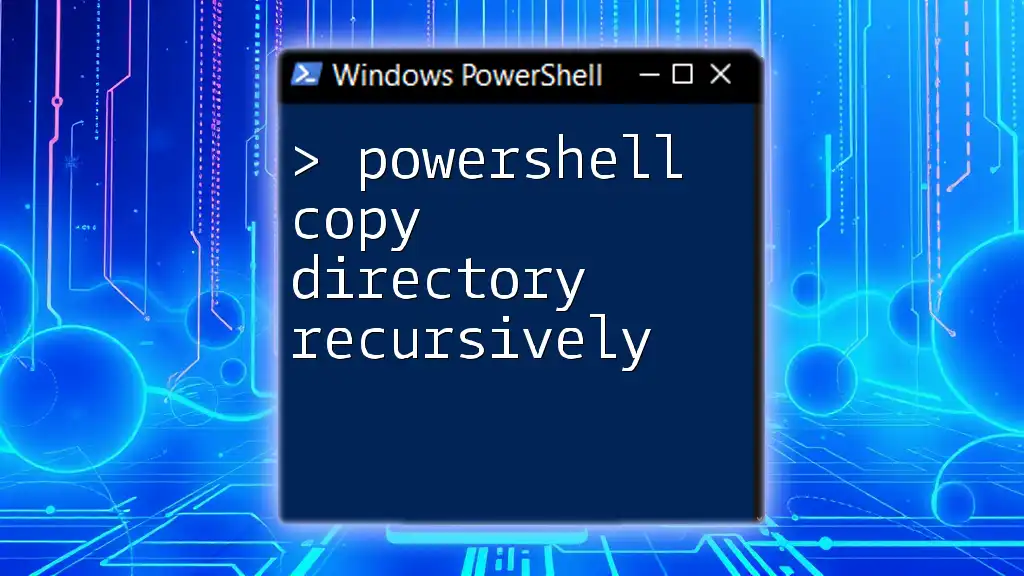
Troubleshooting Common Issues
While PowerShell is a robust tool, users may encounter common issues. File permissions can often prevent access to certain directories. Be sure to run your scripts in an elevated PowerShell session by right-clicking and selecting "Run as Administrator."
Utilizing `Try...Catch` blocks in your scripts can enhance error handling. This structure allows you to manage errors gracefully and respond accordingly:
try {
Compare-Object -ReferenceObject $dir1 -DifferenceObject $dir2
} catch {
Write-Host "An error occurred: $_"
}
This way, you can track and resolve issues as they arise.
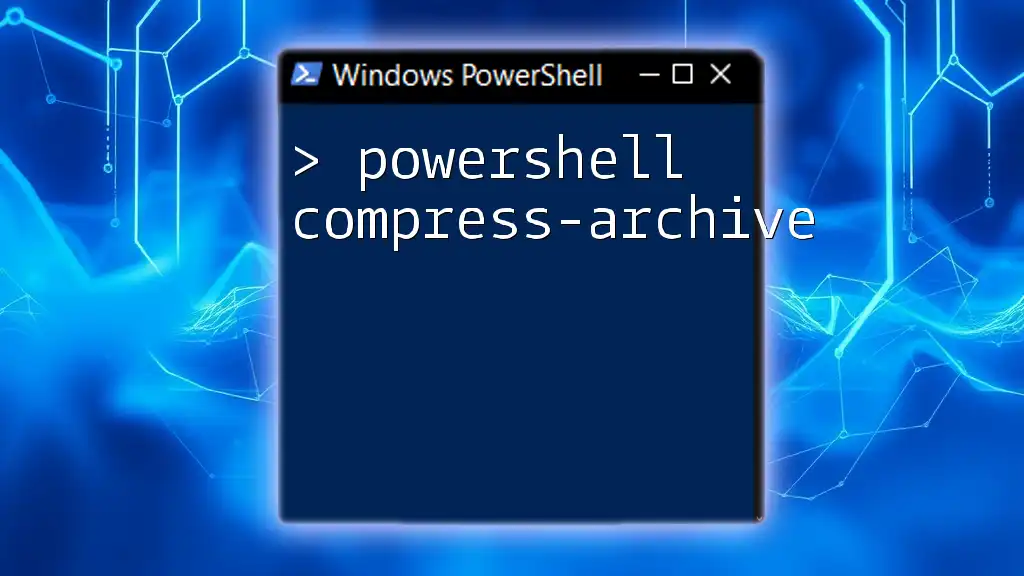
Conclusion
In this guide, you've explored how to use PowerShell to compare directories effectively. You've learned about the various cmdlets, filtering options, and checksum verifications. The versatility of PowerShell makes it an invaluable tool for IT professionals and enthusiasts alike who need to manage and monitor filesystem changes efficiently.
By mastering these techniques, you're not just executing commands; you're building a foundational skill set that enhances your overall scripting proficiency. Embrace the power of PowerShell for your directory comparisons and automate recurring tasks to save time and effort in your workflows.