In PowerShell, you can compare two arrays to find the differences between them using the `Compare-Object` cmdlet, which returns the unique elements from each array.
$array1 = 1, 2, 3, 4
$array2 = 3, 4, 5, 6
Compare-Object -ReferenceObject $array1 -DifferenceObject $array2
Understanding PowerShell Arrays
Creating Arrays in PowerShell
In PowerShell, arrays are a collection of items that can be of any data type. Creating an array is straightforward. Here are two common methods:
- Using Comma-Separated Values: You can create an array by enclosing values in parentheses and separating them with commas.
$fruits = "Apple", "Banana", "Cherry"
- Using the `@()` Syntax: This is particularly useful when defining an empty array or when you want to ensure that a single object is treated as an array.
$emptyArray = @()
$numbers = @(1, 2, 3, 4)
In this method, the `@` symbol indicates that you're working with an array.
Accessing Array Elements
Accessing elements in an array is done using indexes, starting from zero. For example:
$fruits[1] # Outputs "Banana"
You can also iterate through an entire array using a `foreach` loop:
foreach ($fruit in $fruits) {
Write-Host $fruit
}
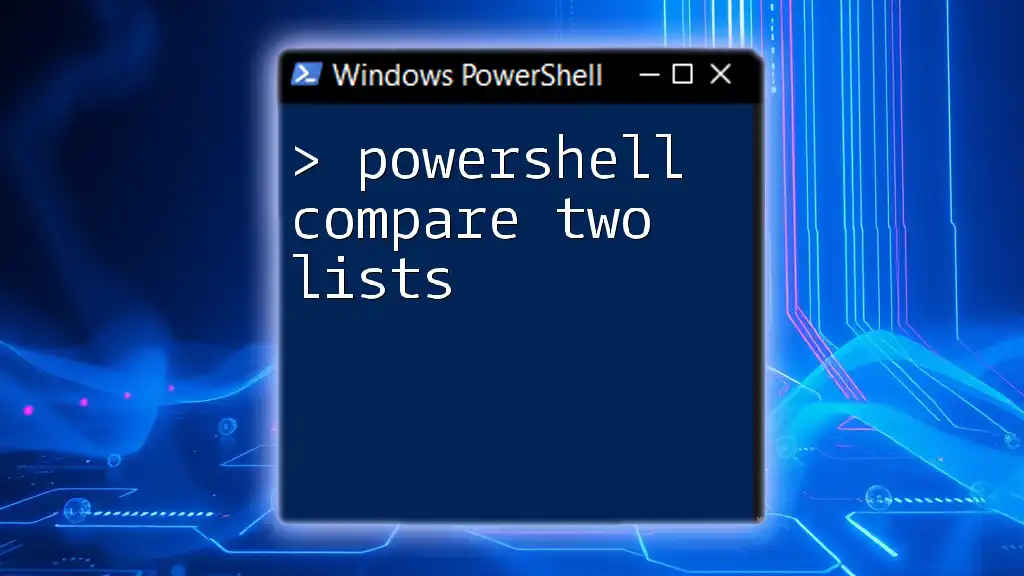
Why Compare Arrays?
Array comparison comes into play in numerous scenarios, such as:
- Validating Data: Ensures that two data sets match, such as checking database records.
- Finding Differences: Useful for identifying discrepancies, such as comparing logs or configurations.
- Synchronizing Data: Necessary when ensuring two systems have the same resources.
These functions streamline processes and improve accuracy, making array comparisons vital in scripting.
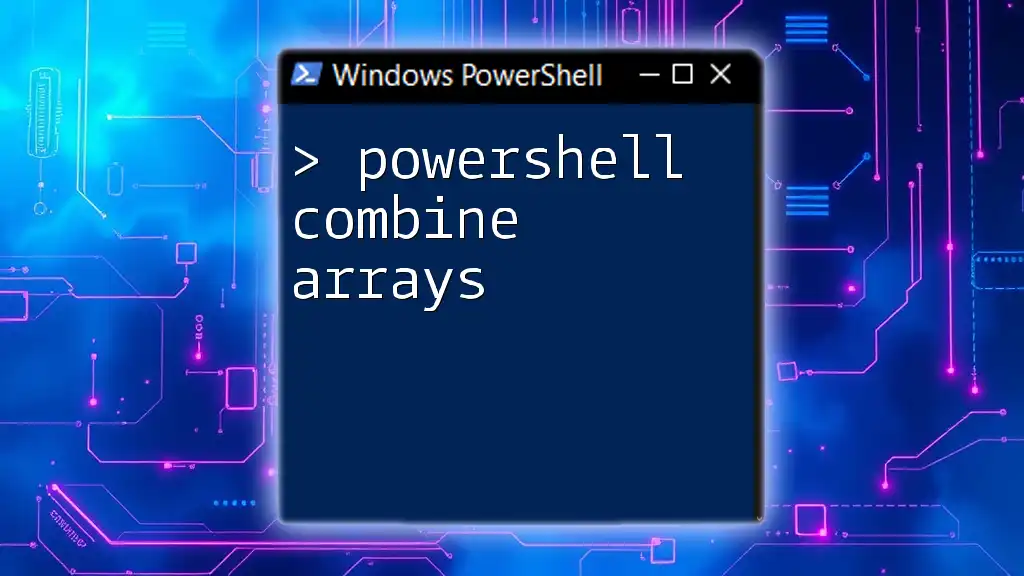
Comparing Arrays in PowerShell
Using the `-eq` Operator
The equality operator `-eq` allows for straightforward comparisons between two arrays. However, note that using `-eq` can return `True` if the arrays are identical objects in memory, which might not be what you want.
Example:
$array1 = @(1, 2, 3)
$array2 = @(1, 2, 3)
$array1 -eq $array2 # This will return 'False' because they are different instances
Using the `Compare-Object` Cmdlet
For a more comprehensive comparison, PowerShell provides the `Compare-Object` cmdlet. This cmdlet compares two sets of objects and returns the differences.
Basic Usage
Here’s how you can use `Compare-Object` to see differences between two arrays:
$array1 = @(1, 2, 3)
$array2 = @(2, 3, 4)
$comparison = Compare-Object -ReferenceObject $array1 -DifferenceObject $array2
$comparison
This outputs:
InputObject SideIndicator
----------- -------------
1 <=
4 =>
The `SideIndicator` shows which side the element belongs to, where `<=` indicates the element is only found in the reference array, and `=>` indicates it exists only in the difference array.
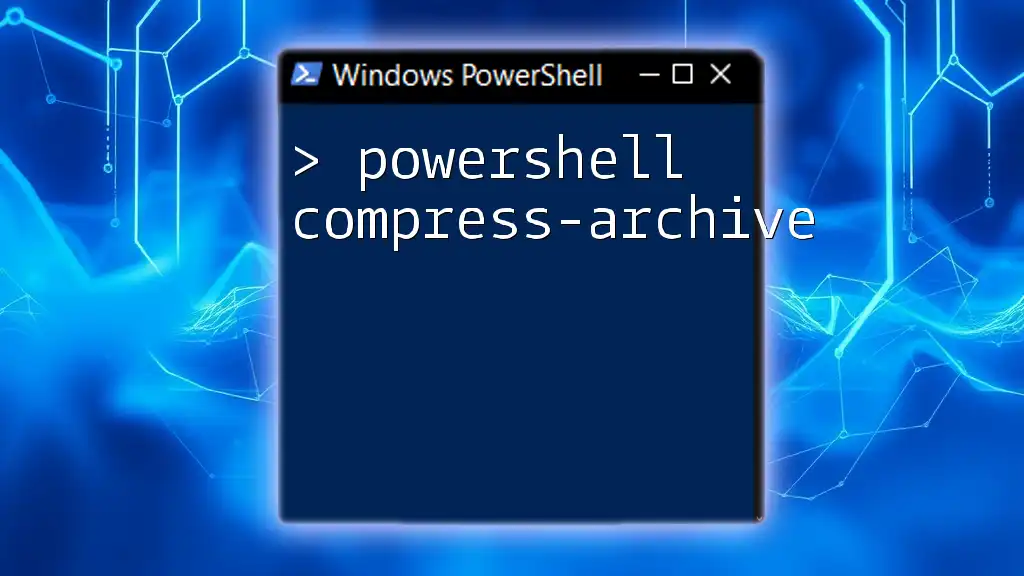
Advanced Array Comparison Techniques
Finding Differences between Two Arrays
Using `Compare-Object`, you can isolate items in one array that are not found in the other. This is crucial for identifying missing data or discrepancies.
Example:
$arrayA = @("A", "B", "C")
$arrayB = @("B", "C", "D")
$difference = Compare-Object -ReferenceObject $arrayA -DifferenceObject $arrayB
$difference
You will find elements "A" and "D" indicated, allowing you to see items unique to each array.
Identifying Common Elements
To find items present in both arrays, adjusting the output of `Compare-Object` is essential. You can filter the results to show only common elements:
$common = Compare-Object -ReferenceObject $arrayA -DifferenceObject $arrayB -IncludeEqual
$common | Where-Object { $_.SideIndicator -eq "==" }
Custom Comparison Logic
For nuanced requirements, implementing custom comparison logic enhances flexibility. You can use script blocks for tailored criteria.
Example using case sensitivity:
$arrayC = @("apple", "Banana", "CHERRY")
$arrayD = @("Apple", "banana", "Cherry")
$caseSensitiveComparison = Compare-Object -ReferenceObject $arrayC -DifferenceObject $arrayD -CaseSensitive
$caseSensitiveComparison
This highlights how case sensitivity can impact comparisons.
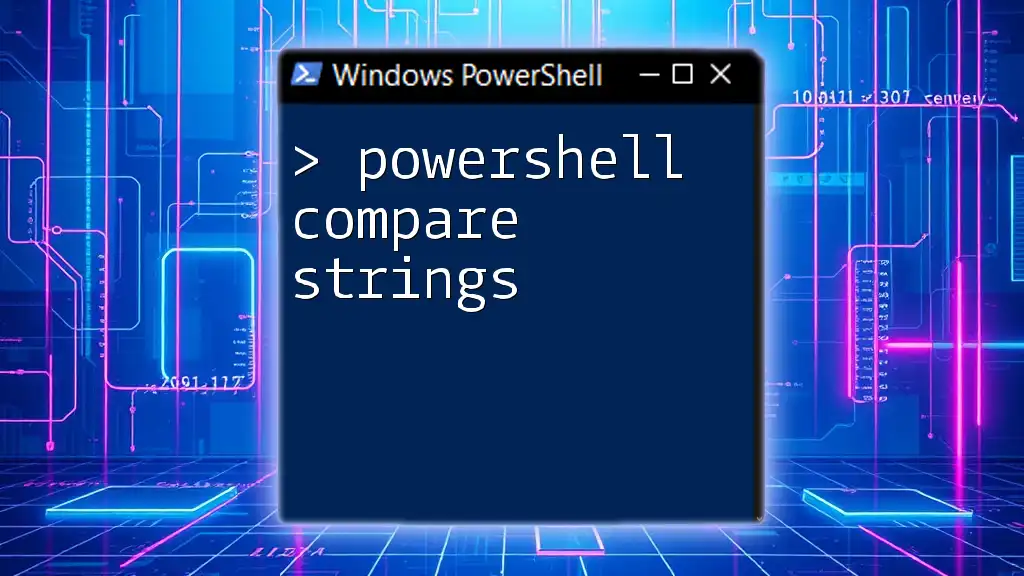
Performance Considerations
Time Complexity of Array Comparison
When dealing with large arrays, understanding performance metrics is crucial. The time complexity of comparing two arrays can increase linearly with their size. For small arrays, performance is usually not a concern; however, consider optimizations for massive datasets, such as:
- Reducing Array Size: Filter out irrelevant data before comparisons.
- Multi-threading: If applicable, use asynchronous operations.
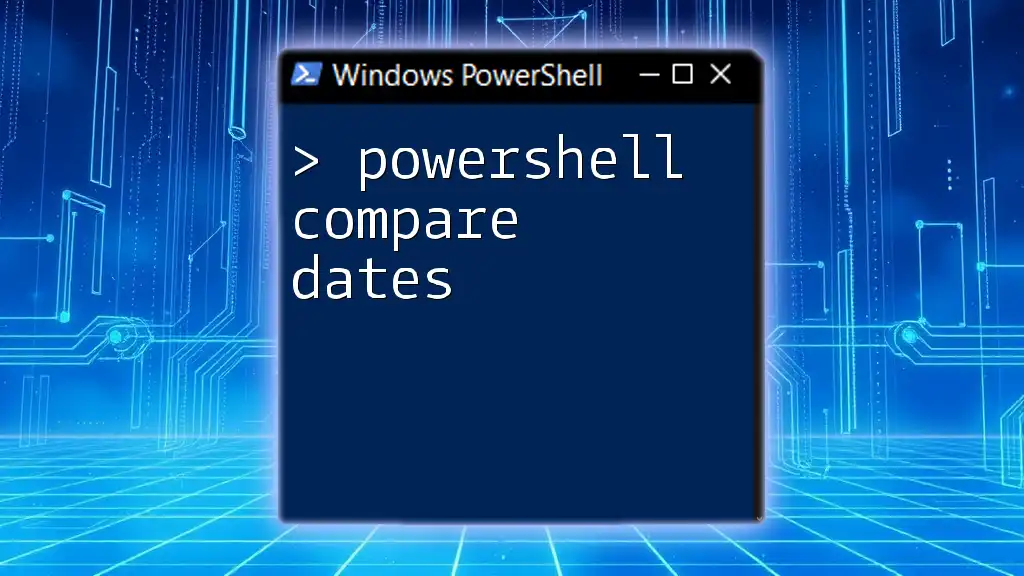
Troubleshooting Common Issues
Common Errors and How to Fix Them
While performing array comparisons, you might encounter:
- Null or Undefined Arrays: Ensure that your arrays are initialized before performing comparisons.
- Mismatched Data Types: Type mismatches can result in unexpected comparison failures.
Example of handling nulls:
if ($null -eq $array1 -or $null -eq $array2) {
Write-Host "One or both arrays are null."
}
Best Practices for Array Comparison
Adhering to best practices can minimize errors and improve readability:
- Use Meaningful Variable Names: This helps in understanding the content of arrays.
- Test with Sample Data: Validate your comparisons using known datasets before applying to live data.
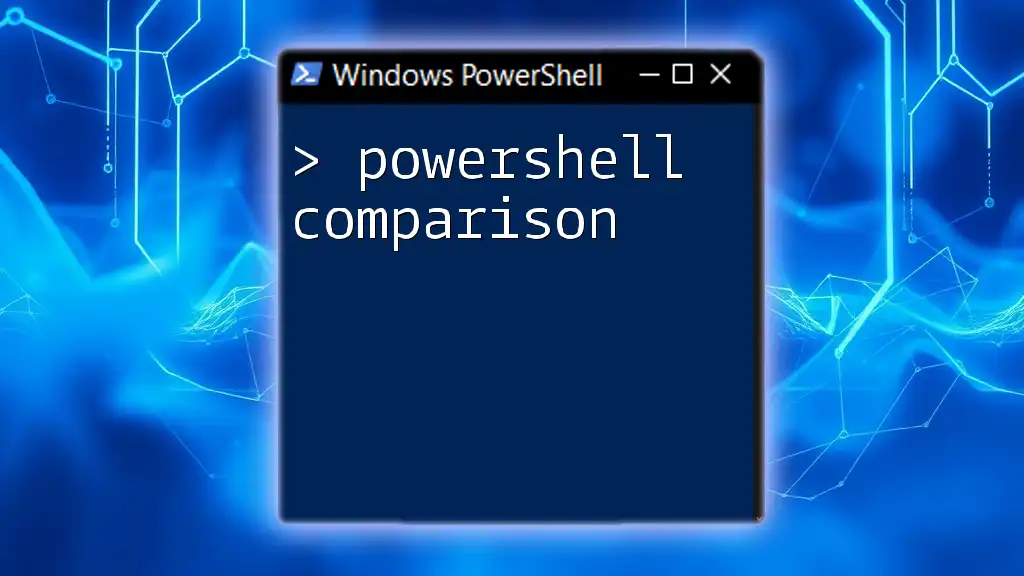
Conclusion
PowerShell provides robust tools for effectively comparing two arrays through various methods, such as the `Compare-Object` cmdlet and the equality operator. Utilizing these comparisons can enhance data validation and manipulation scripts, paving the way for efficient automation solutions.
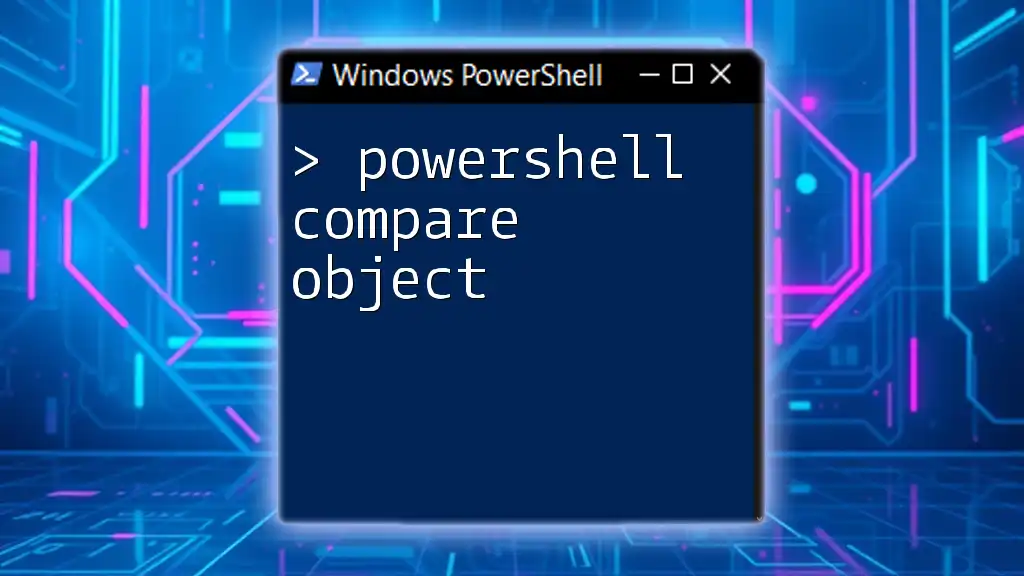
Additional Resources
For those seeking to dive deeper into PowerShell and array operations, consider exploring relevant articles, tutorials, and forums dedicated to PowerShell scripting.
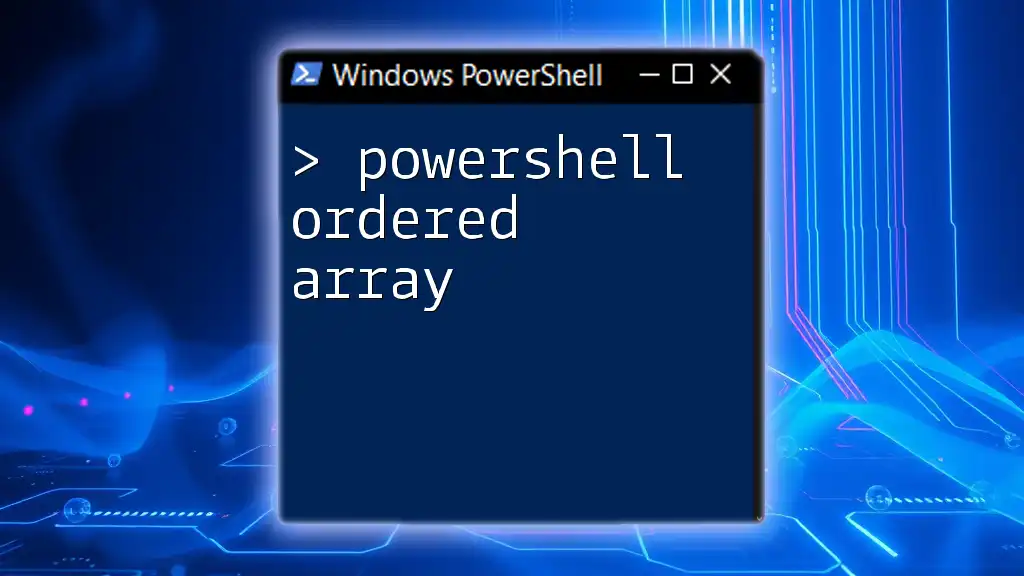
Call to Action
Share your experiences or unique challenges regarding array comparisons in PowerShell. Your insights can enrich our community and help others on their scripting journey!