In PowerShell, a JSON array is a structured format that holds a collection of values, allowing you to easily work with and manipulate data using built-in cmdlets.
$jsonArray = '["Value1", "Value2", "Value3"]' | ConvertFrom-Json
Understanding JSON Arrays
What is a JSON Array?
A JSON array is a structured way to organize data using a list-like syntax that allows for storing multiple values within a single entity. It is defined by square brackets `[]` and can contain objects, strings, numbers, or even other arrays. This structure is part of JavaScript Object Notation (JSON), which is widely used for data interchange between systems.
Comparison of JSON Objects vs. Arrays
While a JSON object is a collection of key-value pairs enclosed in curly braces `{}`, a JSON array is a sequential list of values. For instance, a JSON object could look like this:
{
"name": "John",
"age": 30
}
In contrast, a JSON array could be represented as follows:
[
{"name": "John"},
{"name": "Jane"}
]
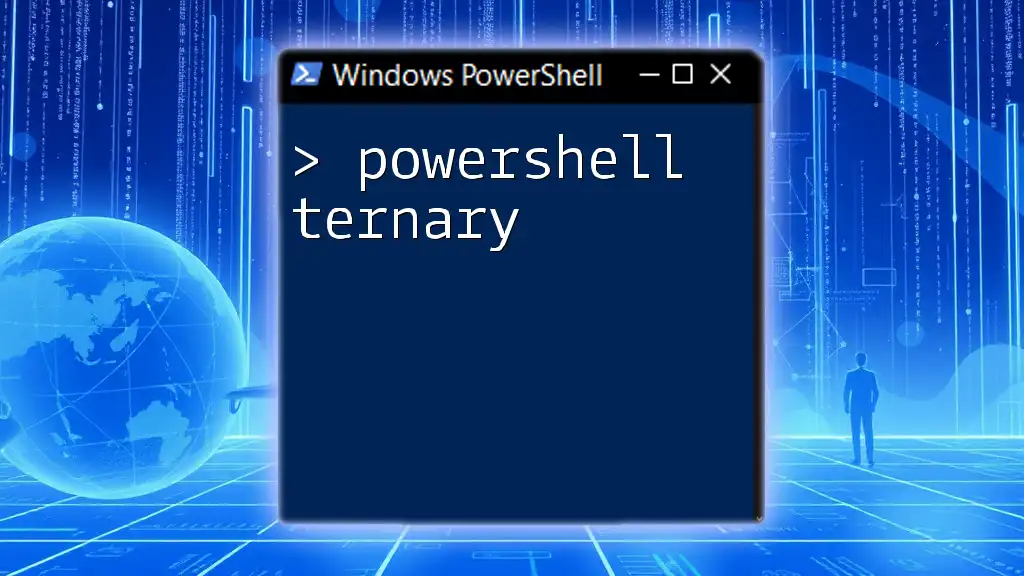
Importing JSON Arrays into PowerShell
Using `ConvertFrom-Json`
To work with JSON arrays in PowerShell, the first step is importing the data using the `ConvertFrom-Json` cmdlet. This cmdlet transforms a JSON formatted string into a PowerShell object. Here’s how you can achieve this:
$jsonArray = '[{"name":"John"}, {"name":"Jane"}]'
$powerShellArray = $jsonArray | ConvertFrom-Json
After running this code, `$powerShellArray` becomes a powerful PowerShell object containing all elements of the original JSON array.
Verifying the Imported Data
To ensure that you have successfully imported the data, you can inspect the PowerShell object using:
$powerShellArray | Format-Table
This will present the data in a tabular format, making it easy to read and verify that all expected values are included.
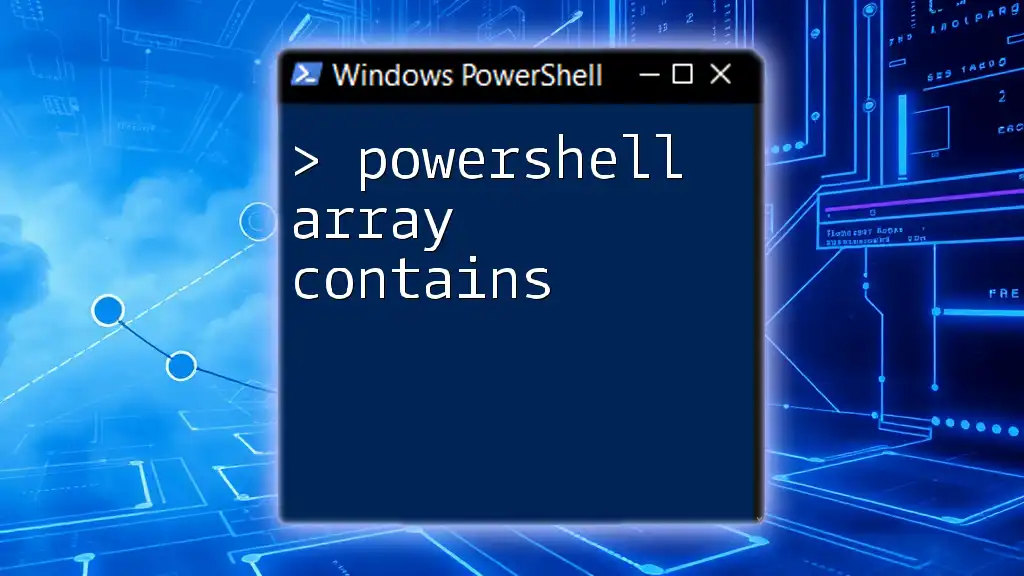
Manipulating JSON Arrays in PowerShell
Accessing Elements in a JSON Array
Once you have imported your JSON array into PowerShell, accessing individual elements is straightforward. You use the indexing method typical in arrays. For example:
$name = $powerShellArray[0].name
This retrieves the name of the first object in your array, which would evaluate to `"John"`.
Iterating Over JSON Arrays
In scenarios where you need to perform operations on each item in the array, you can use `ForEach-Object`. This cmdlet allows you to iterate over each item easily:
$powerShellArray | ForEach-Object { $_.name }
Using this code, you will retrieve a list of names from all objects in the array.
Filtering JSON Array Data
If you're interested in extracting specific elements from your JSON array based on certain criteria, `Where-Object` is your tool of choice. Here’s how to utilize it:
$filteredNames = $powerShellArray | Where-Object { $_.name -like "Ja*" }
This command filters the names that start with "Ja", returning any matching entries from the original array.
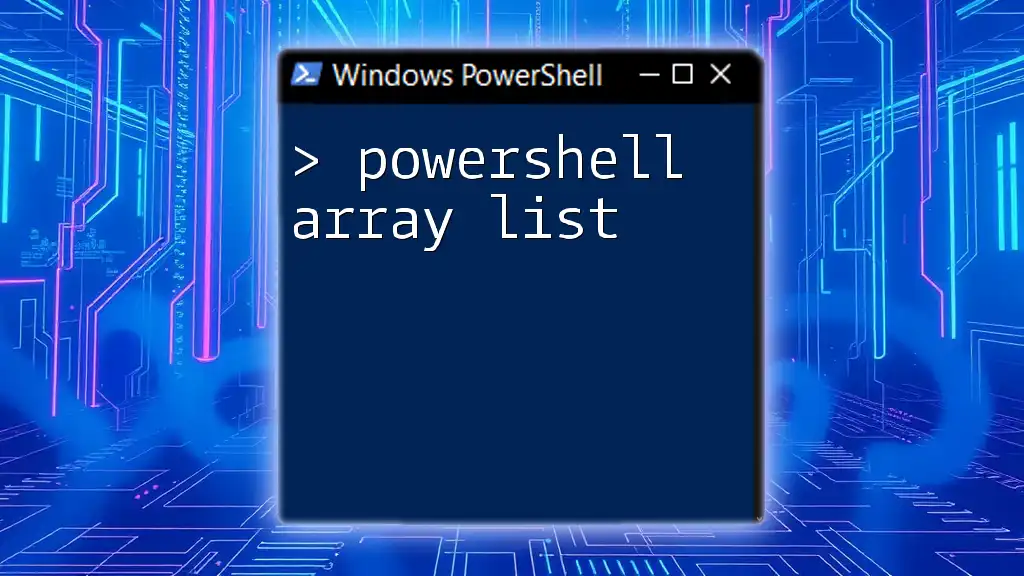
Modifying JSON Arrays
Adding New Elements
PowerShell enables you to modify JSON arrays easily. To add a new item to your array, create a new object and append it using the `+=` operator:
$newObject = New-Object PSObject -Property @{ name = "Jake" }
$powerShellArray += $newObject
This code snippet creates a new object named "Jake" and adds it to your existing array.
Removing Elements
Similarly, if you need to remove specific elements from your JSON array, you can filter them out using `Where-Object`:
$powerShellArray = $powerShellArray | Where-Object { $_.name -ne "Jane" }
Here, any objects with the name "Jane" will be excluded from the array.
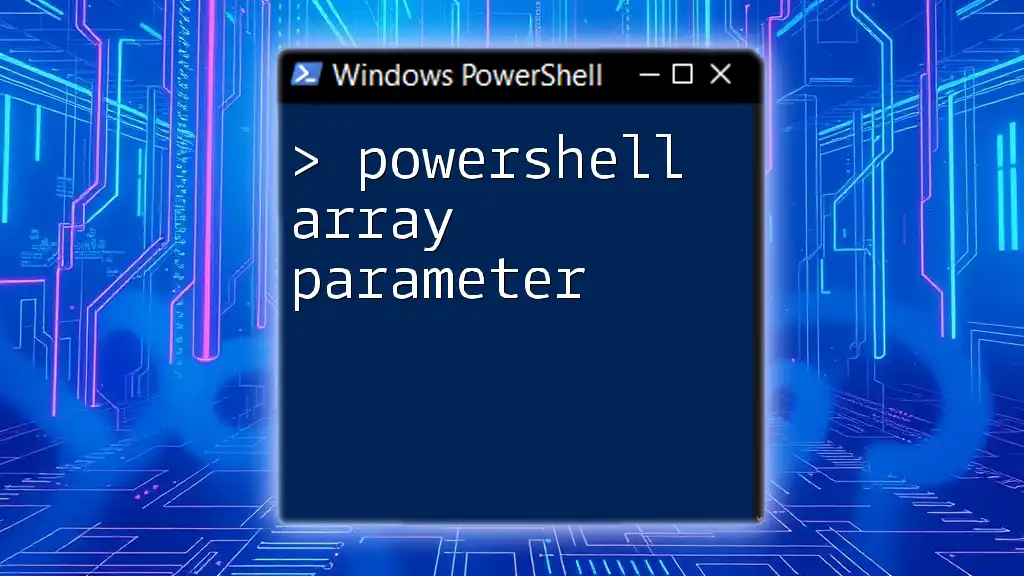
Exporting JSON Arrays from PowerShell
Using `ConvertTo-Json`
When you're ready to export your modified PowerShell object back into JSON format, the `ConvertTo-Json` cmdlet comes into play. It transforms the PowerShell object back into a JSON string:
$jsonOutput = $powerShellArray | ConvertTo-Json
Now, `$jsonOutput` holds the JSON representation of your modified array.
Saving JSON to a File
To persist this JSON data on disk, you can use the `Out-File` cmdlet:
$jsonOutput | Out-File -FilePath "output.json"
With this command, the JSON array will be saved to a file named `output.json`.
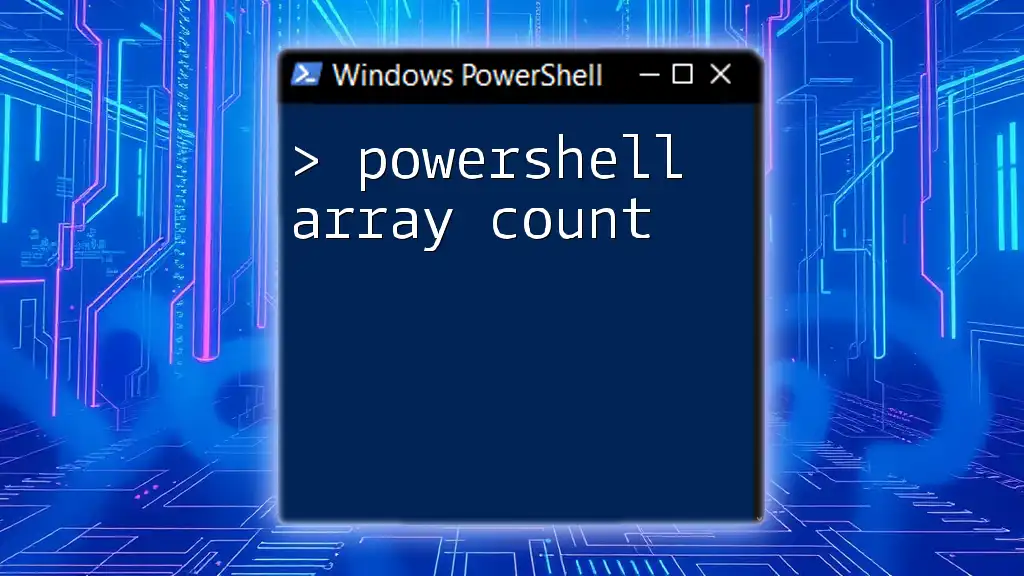
Common Use Cases for JSON Arrays in PowerShell
Automating Data Retrieval
One of the most powerful applications of JSON arrays within PowerShell is in automating data retrieval from APIs. For instance, many REST APIs return data in JSON format, including arrays. By using PowerShell, you can easily call these APIs, then process the returned JSON arrays to suit your needs.
For example, making an API request and parsing the response might look like this:
$response = Invoke-RestMethod -Uri "https://api.example.com/data"
$dataArray = $response.data | ConvertFrom-Json
Configuring Systems Programmatically
JSON arrays can also serve as a versatile configuration management tool. For scripting deployment scenarios where multiple configurations need to be applied, JSON arrays offer a structured way to define these configurations, making your scripts modular and reusable.
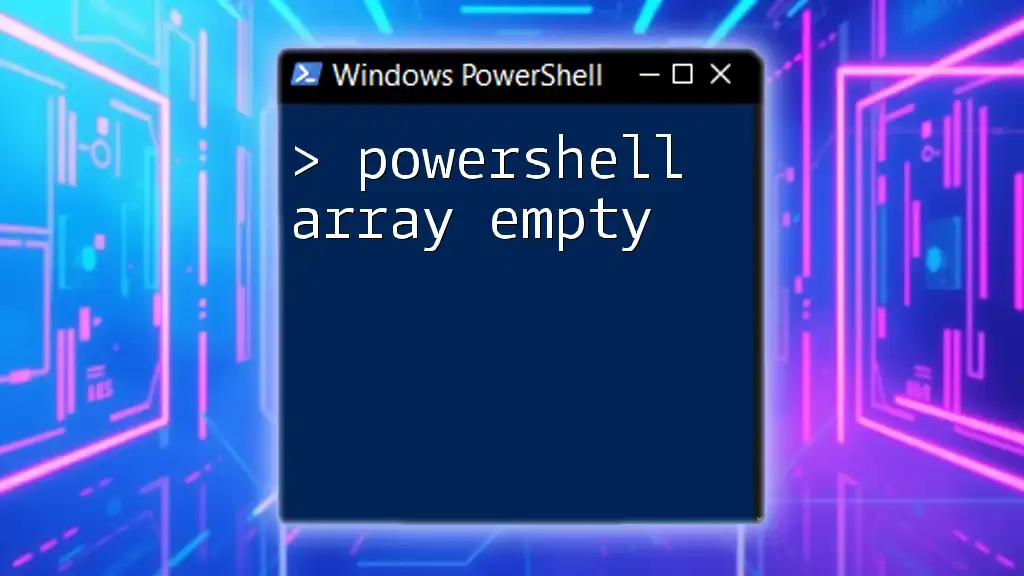
Troubleshooting Common Issues with JSON Arrays
Errors in JSON Format
One common challenge is encountering errors due to improperly formed JSON. Missing brackets, or commas can prompt errors when importing JSON data. Always validate your JSON using online tools or JSON validators to ensure that it adheres to the correct structure.
If you get an error on import, evaluate the JSON structure closely. An example of a malformed JSON error might look like this:
ConvertFrom-Json : Invalid character in JSON at line 1, position X
Debugging PowerShell Scripts with JSON Arrays
Identifying issues in your script can be streamlined by implementing debugging practices. Utilize `Write-Output` to print out variables at various stages of your script. For instance:
Write-Output "Current Items: $($powerShellArray | Out-String)"
This way, you can track changes to your JSON arrays and troubleshoot effectively.
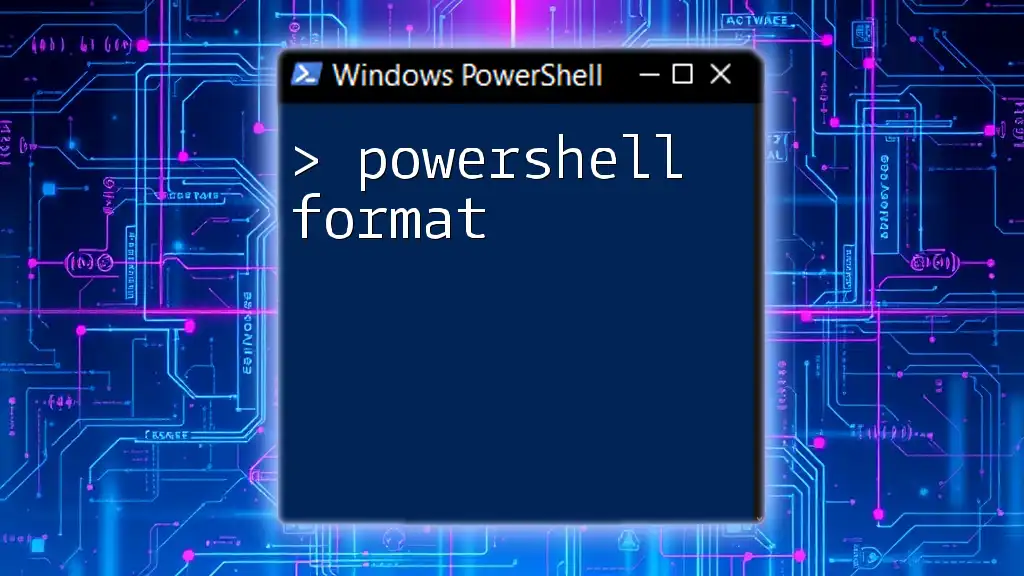
Conclusion
In this comprehensive guide, we explored the concept of PowerShell JSON arrays, from importing and manipulating them to exporting and troubleshooting. Mastering JSON arrays in PowerShell can significantly enhance your scripting capabilities, especially when managing configurations or automating data interactions. As you continue to explore PowerShell, the use of JSON arrays will become a valuable asset in ensuring efficient and effective data handling.