In PowerShell, a line break can be created using the backtick character (`` ` ``) at the end of a line, allowing you to continue the command on the next line for better readability.
Here's an example:
Write-Host 'Hello, World!`
This is a new line.'
Understanding Line Breaks and Line Feeds in PowerShell
What is a Line Break?
A line break in PowerShell refers to the point at which a line of code or text ends and a new line begins. It helps improve the organization of scripts and enhances readability. This is particularly vital in programming, where clarity can significantly influence the maintainability and functionality of the code.
In PowerShell, a line break visually separates commands or segments of code, making it easier for anyone reviewing the script to understand its structure.
What is a Line Feed?
A line feed signifies a type of character used to indicate the end of a line in text files and strings. In PowerShell, it is represented by the newline character (`n`). While often used interchangeably with line breaks, understanding the distinction is key. A line break signifies a break in the line itself, while a line feed prompts the creation of a new line in the code when outputting text.
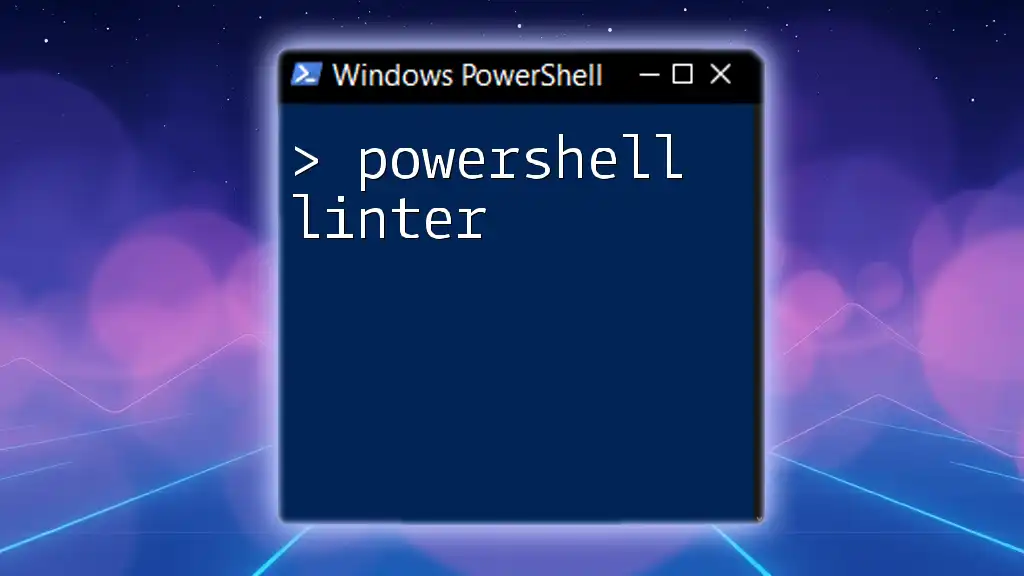
Why Use Line Breaks in PowerShell?
Enhancing Readability
One of the primary reasons to incorporate line breaks in PowerShell scripts is to enhance readability. Code that is neatly organized is easier to comprehend, maintain, and share. For example, consider the following code without line breaks:
Write-Host "This is a long command that can be difficult to read because there are no breaks."
In contrast, this version uses line breaks for clarity:
Write-Host "This is a long command that `
can be difficult to read because `
there are no breaks."
The second example allows anyone examining the code to quickly grasp its purpose.
Managing Code Length
Line breaks are also instrumental in managing the length of commands. Long commands can become unwieldy, presenting a risk of errors if one misreads or misses parts of it. By breaking a lengthy command into shorter lines, you not only create a more digestible format but also mitigate the chances of mistakes. Here's an example of a long command without breaks:
Get-ChildItem -Path "C:\Users\ExampleUser\Documents" -Recurse -Include "*.txt" -ErrorAction Stop | Out-File -FilePath "C:\Users\ExampleUser\Documents\output.txt"
With appropriate line breaks, it becomes more manageable:
Get-ChildItem -Path "C:\Users\ExampleUser\Documents" `
-Recurse `
-Include "*.txt" `
-ErrorAction Stop | `
Out-File -FilePath "C:\Users\ExampleUser\Documents\output.txt"
Formatting Output
Using Line Breaks in Output
Line breaks can also significantly improve the clarity of output data. For example, when displaying multiple lines of text, you can enhance the presentation with line breaks. Here’s an example of how line breaks can structure output into clear, distinct parts:
Write-Output "This is the first line.`nAnd this is the second line."
The output from the above command will appear as:
This is the first line.
And this is the second line.
This structured output is not only visually appealing but also enhances understanding for the end-user.
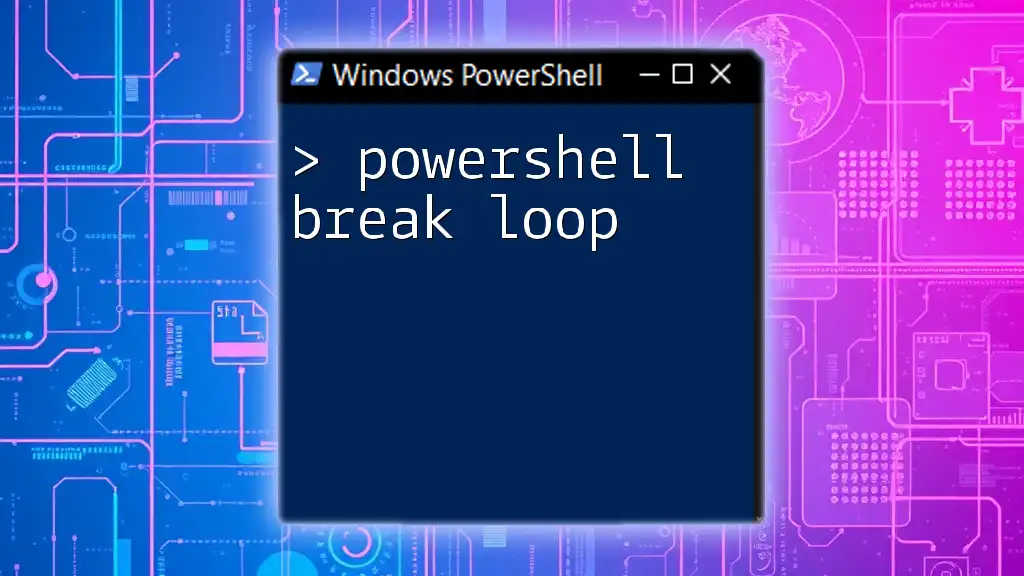
Implementing Line Breaks in PowerShell
Using Backticks for Line Breaks
PowerShell allows you to use the backtick character (`) to indicate that a command continues on the next line. This is particularly useful when you want to make lengthy commands more readable. Here's how you would use backticks effectively:
Write-Host "This is a long string that `
continues on the next line."
Using the New-Line Characters
Using `"` for New Lines
Another way to create line breaks in PowerShell is by using the newline escape sequence `n` within double quotes. This approach is practical when crafting strings that need to contain multiple lines:
$multiLineString = "Line 1`nLine 2`nLine 3"
Write-Host $multiLineString
When executed, the output will clearly depict each line separated as intended:
Line 1
Line 2
Line 3
Using the `Write-Output` Cmdlet
The `Write-Output` cmdlet also supports line breaks effectively. By including newline characters in your string, you can format the output as you wish:
Write-Output "This is the first line.`nAnd this is the second line."
This method is similar to the previous example, offering additional flexibility when generating output to the user.
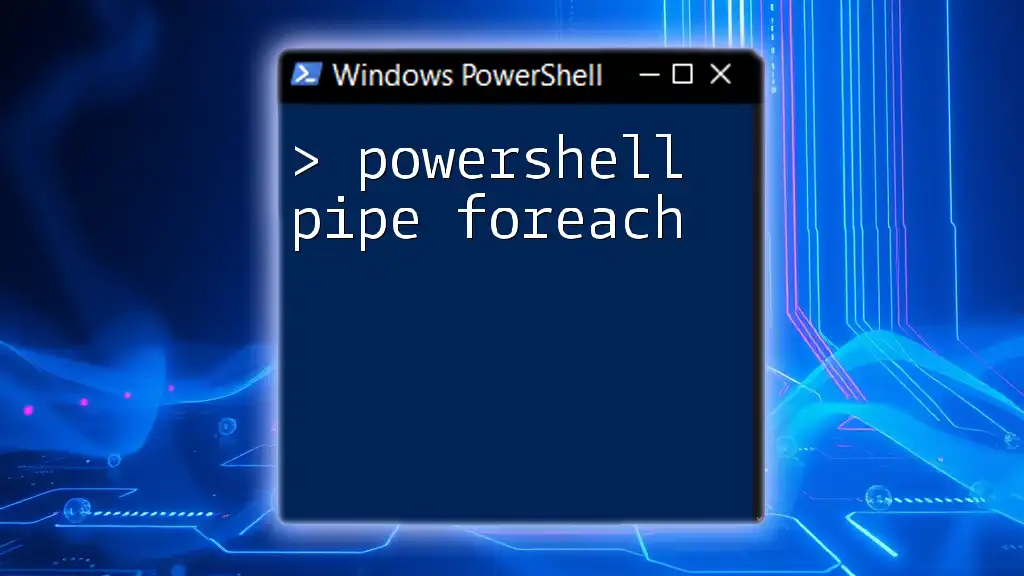
Advanced Techniques for Line Breaks
Custom Formatting with Format-Table
For displaying structured data more clearly, using `Format-Table` can help manage line breaks in output. This enables you to specify how you want your information formatted systematically. Here's an example:
Get-Process | Format-Table -Property Name, Id, @{Name='Details';Expression={"More info on `n $_.Name"}}
Here, the output not only includes the process name and ID but also a formatted string that utilizes line breaks for additional context.
Conditional Line Breaks
You can also employ line breaks based on conditional statements. By implementing this logic, you can control when line breaks are applied within your output effectively. Here’s an example:
$messages = "Hello", "World", "PowerShell"
foreach ($msg in $messages) {
if ($msg -eq "World") {
Write-Host "$msg`nenjoy PowerShell!"
} else {
Write-Host "$msg"
}
}
In this snippet, a line break is conditionally added to introduce a remark about PowerShell only when the message is "World."
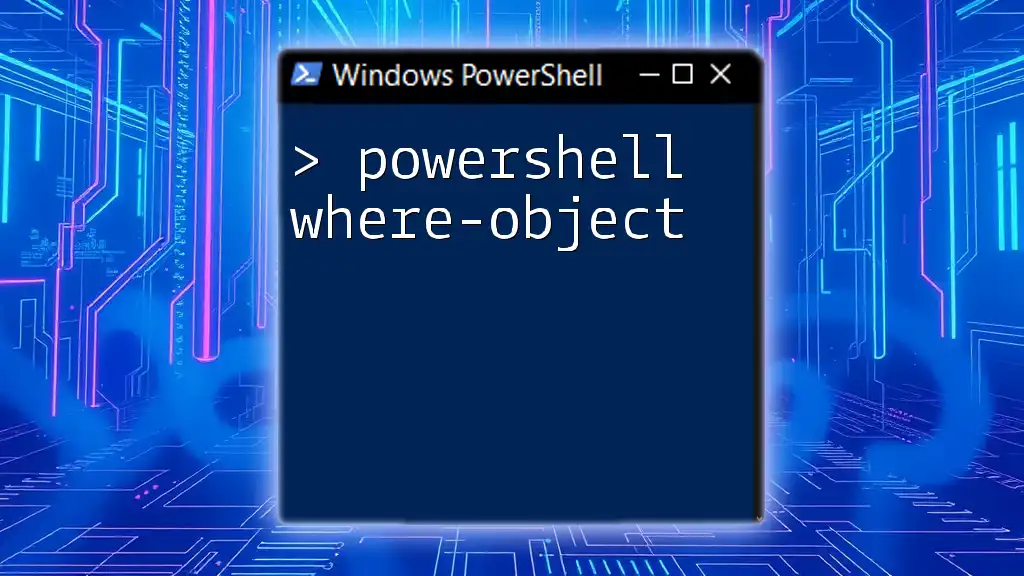
Common Mistakes to Avoid
Misusing Backticks
One common error is misusing the backtick character for line breaks. Make sure to place it at the end of the line you want to continue, and avoid adding additional spaces after it, as this may lead to unexpected behavior.
Unintended Output Format Changes
When using line feeds, inconsistencies in string formatting may arise if not applied correctly. To ensure your intended output is achieved, always double-check that you are using `n` within the correct context of strings.
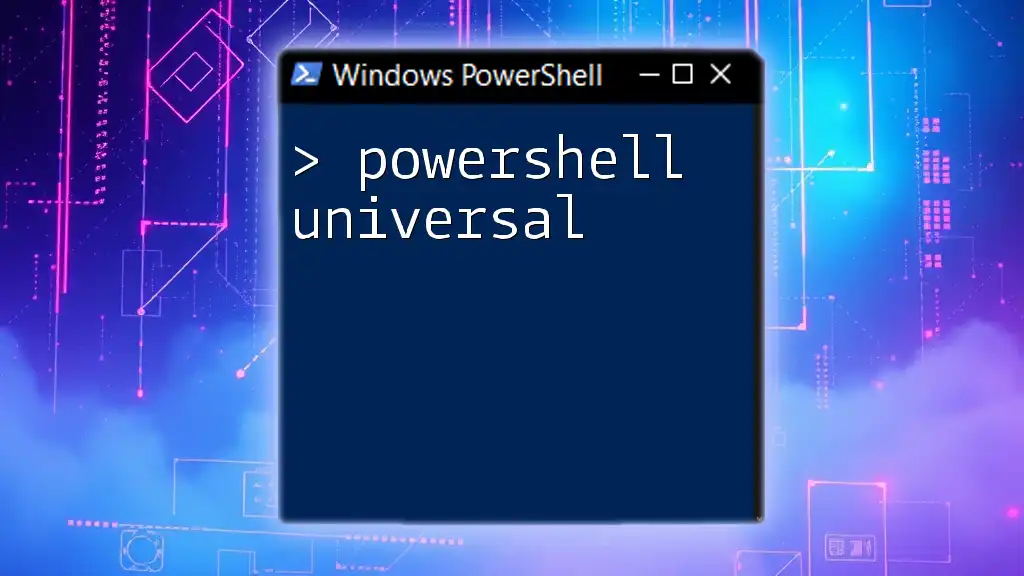
Conclusion
In summary, understanding the concept of PowerShell line breaks and their implementation is crucial for writing clean, manageable scripts. They not only enhance readability and facilitate the handling of long commands but also improve the clarity of your output.
Embrace the practice of experimenting with line breaks and line feeds to elevate your PowerShell scripting skills. Engaging with these techniques allows for clearer code, ensuring others can follow your logic or maintain your scripts with ease.