In PowerShell, the `-notin` operator checks whether a specified value is not present in a given list or array.
Here's a code snippet demonstrating this:
$fruitList = @('Apple', 'Banana', 'Cherry')
$myFruit = 'Orange'
if ($myFruit -notin $fruitList) {
Write-Host "$myFruit is not in the list."
}
Understanding PowerShell and Its "Not In List" Concept
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administrators. Built on the .NET framework, it enables users to automate tasks and manage system configurations efficiently. PowerShell has gained immense popularity due to its ability to handle both simple and complex tasks seamlessly.
Overview of Lists in PowerShell
In PowerShell, lists can be created and manipulated using arrays, which are foundational elements. An array is a data structure that can hold multiple items, such as strings, numbers, or objects. Understanding how to create and manage lists is crucial for any PowerShell user.
You can create a list in PowerShell using the `@()` syntax. For example, to create a list of integers:
$numbers = @(1, 2, 3, 4, 5)
Common commands to manipulate lists include `Add()`, `Remove()`, and you can retrieve elements using indexing, like `$numbers[0]`.
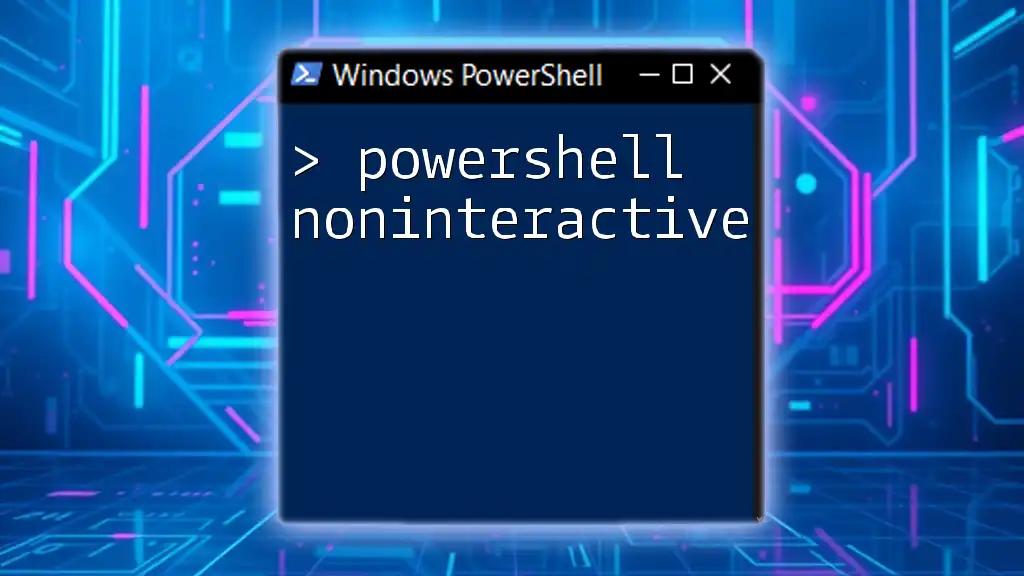
The Concept of "Not In List"
Defining "Not In List"
The phrase "not in list" refers to checking if a particular value is absent from a specified collection or array. This concept is deeply integrated into logical expressions and is crucial for decision-making processes in scripts. For instance, when filtering data, you might want to ensure certain values are excluded; using "not in list" simplifies this task.
Logic Operators in PowerShell
Understanding logical operators is essential when working with conditions in PowerShell. Logical operators include:
- `-eq` (equals)
- `-ne` (not equals)
- `-lt` (less than)
- `-gt` (greater than)
The `-notin` operator is particularly useful, allowing you to verify that a value does not exist within a given list or array.

Using "Not In List" in PowerShell Scripts
Basic Syntax of "Not In"
One of the simplest ways to implement "not in list" functionality is through the `-notcontains` operator. This operator allows you to determine whether a particular item is absent from a collection. Here’s a basic example:
$list = @(1, 2, 3, 4, 5)
$value = 6
if ($value -notin $list) {
"Value not in list"
}
In this snippet, the script checks if `6` is not in the list of numbers. Since it is indeed absent, the output would confirm that the value is not part of the array.
Advanced Examples of "Not In List"
The versatility of the "not in list" concept extends to filtering objects based on exclusion criteria. This method is particularly handy when dealing with file systems or databases.
For example, you can filter out specific items from a list of files:
$allItems = Get-ChildItem "C:\*"
$excludedItems = @("file1.txt", "file2.txt")
$filteredItems = $allItems | Where-Object { $_.Name -notin $excludedItems }
This script retrieves all items from the `C:\` directory and filters out `file1.txt` and `file2.txt`, creating a new collection containing only the files not listed in the `excludedItems`.
Using Conditional Logic with "Not In"
Conditional statements are a defining feature of scripting, enabling dynamic decision-making. You can apply the "not in" concept to check any variable against a list:
$users = @("Alice", "Bob", "Charlie")
$loggedOutUser = "Dave"
if ($loggedOutUser -notin $users) {
Write-Host "$loggedOutUser is not logged in."
}
In this code snippet, if `Dave` is not found in the `$users` array, a message is printed, indicating that he is not currently logged in.

Real-World Applications
Automating System Administration Tasks
The “not in list” operator simplifies many automation tasks. For system administrators, routinely checking and filtering user accounts or files ensures effective management of resources. For instance, when conducting user audits, this operator can help identify unused accounts quickly.
Data Validation in PowerShell
User input validation is critical to maintaining the integrity of your scripts. By using the "not in list" logic, you can verify whether the input meets predefined criteria.
Let’s assume you have a defined set of acceptable user roles:
$allowedRoles = @("Admin", "User", "Guest")
$userRole = "Visitor"
if ($userRole -notin $allowedRoles) {
Write-Host "Role not allowed: $userRole"
}
This script provides feedback if a user attempts to register with an unauthorized role.

Troubleshooting Common Issues
Common Errors When Implementing "Not In List"
When using the "not in list" functionality, one common pitfall is confusion between `-notin` and `-notcontains`. Remember that `-notin` checks for the absence of a single value within an array, while `-notcontains` typically applies to checking multiple elements.
Debugging Techniques
PowerShell offers various debugging tools that can aid in troubleshooting scripts. Use `Write-Debug` to monitor the state of your variables or condition checks, customizing your output to understand where an issue may arise effectively.
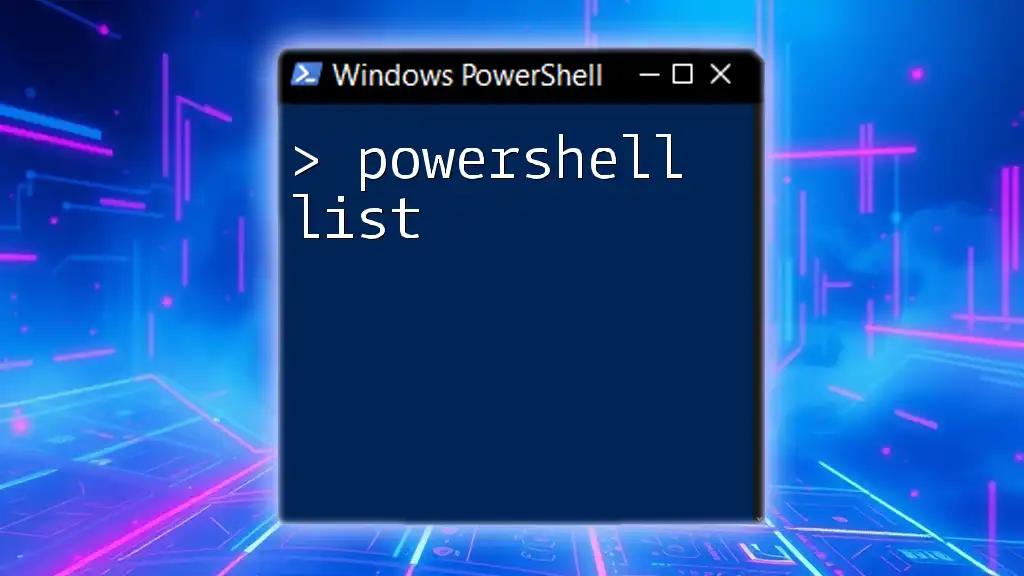
Conclusion
Recap of Key Points
Grasping the concept of "not in list" is essential for mastering PowerShell. Utilizing such logic can streamline many processes in your administration tasks. By integrating the `-notin` operator, you can efficiently handle exclusions and validations within your scripts.
Additional Resources
To further enhance your PowerShell skills, consider other resources such as official documentation, online forums, and advanced scripting tutorials. Understanding PowerShell not only boosts your productivity but also helps in automating complex tasks seamlessly, ultimately contributing to a more streamlined IT environment.