In PowerShell, the `-notlike` operator allows you to filter strings that do not match a specified pattern using wildcard characters.
# Example of using -notlike to filter out items that do not match the pattern
$items = "apple", "banana", "cherry"
$filteredItems = $items | Where-Object { $_ -notlike "a*" }
Write-Host $filteredItems # Output will be "banana", "cherry"
Understanding the Basics of PowerShell Comparison Operators
What are Comparison Operators?
In PowerShell, comparison operators allow you to compare two values to determine their relationship. They are fundamental for tasks such as filtering data, conditional execution, and much more. Comparison operators in PowerShell include `-eq`, `-ne`, `-gt`, `-lt`, `-ge`, `-le`, `-like`, and `-notlike`, among others.
Introduction to the "Like" and "Not Like" Operators
The "Like" operator is used for string matching with wildcards. For instance, if you want to find strings that start with a specific prefix or end with a particular suffix, "Like" helps achieve that easily.
Conversely, the "Not Like" operator is designed to ensure that the string does not match a specific pattern. This is particularly effective when filtering out unwanted strings from a dataset.
Example:
"apple" -like "ap*"
This command will return `True` because "apple" starts with "ap".
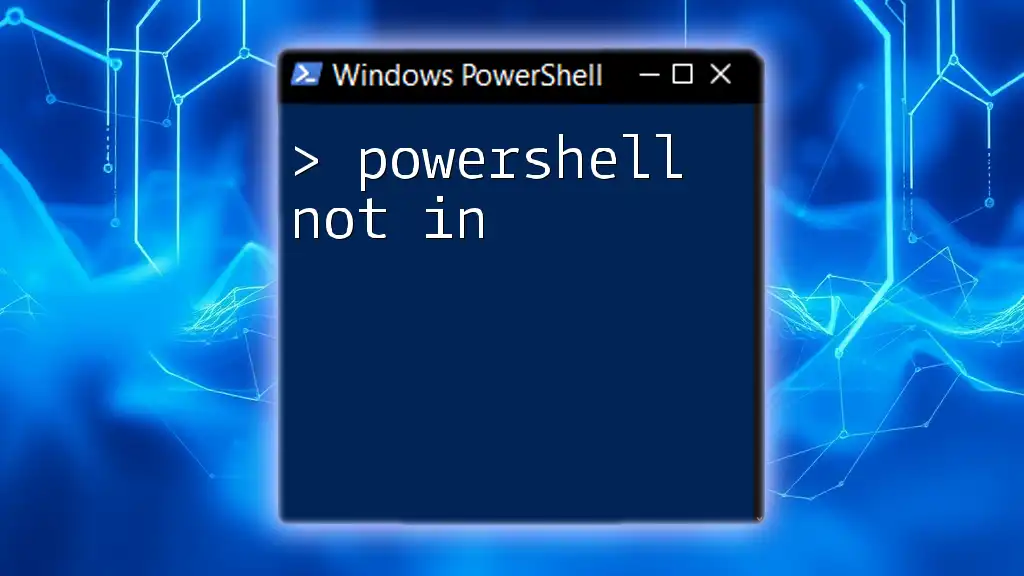
Syntax of the Not Like Operator
Basic Syntax
The basic syntax for using the "Not Like" operator is quite straightforward. It is generally structured as follows:
<value> -notlike <pattern>
Example:
"example" -notlike "ex*"
In this case, the result will return `False` since "example" does fit the pattern that begins with "ex".
Detailed Breakdown of Syntax Components
In the syntax, the left side represents the string you're evaluating, while the right side is the pattern you're comparing against. The right side often uses wildcards for flexibility—`*` represents any number of characters, and `?` represents a single character.
Understanding these components is crucial for effectively utilizing the "Not Like" operator.
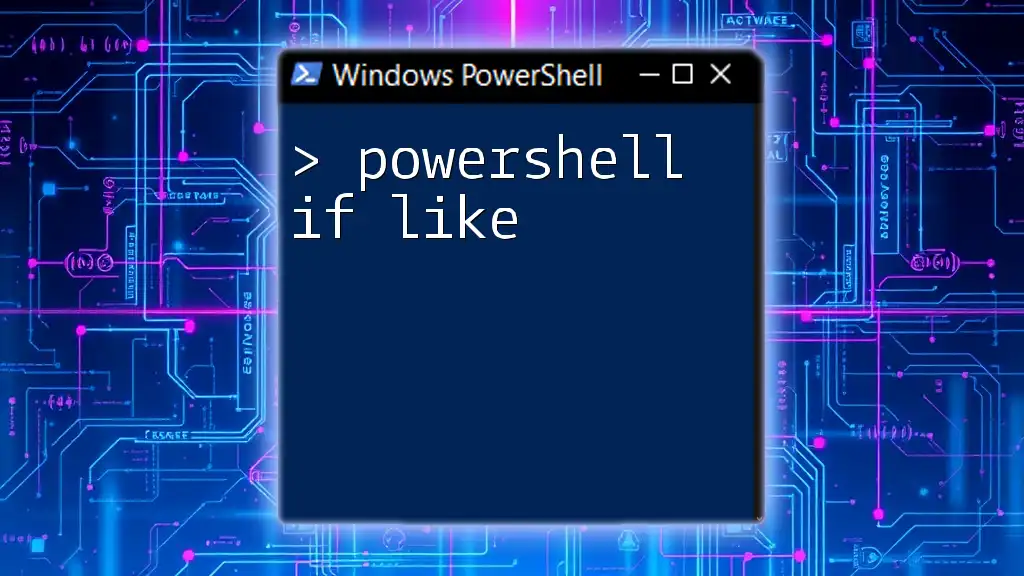
Using the Not Like Operator in PowerShell Scripts
Basic Filtering with Not Like
One of the simplest yet powerful uses of the "Not Like" operator is to filter arrays or lists based on a specific criterion.
Example Use Case: Filtering out unwanted strings from an array.
$array = @("apple", "banana", "cherry!")
$filteredArray = $array | Where-Object { $_ -notlike "cherry*" }
In this snippet, `cherry!` is excluded from `$filteredArray` because it matches the specified pattern with "cherry".
Real-World Use Cases
Filtering File Names
Another practical application is filtering file names. Imagine you want to list all files in a directory except for those with a `.txt` extension.
Get-ChildItem | Where-Object { $_.Name -notlike "*.txt" }
This command retrieves and lists all files that do not end with ".txt".
PowerShell and User Input
The "Not Like" operator can also be useful when dealing with user inputs.
Example:
$input = Read-Host "Enter a fruit name"
if ($input -notlike "apple") { "You didn't enter apple!" }
This example checks if the user did not input "apple" and responds accordingly.
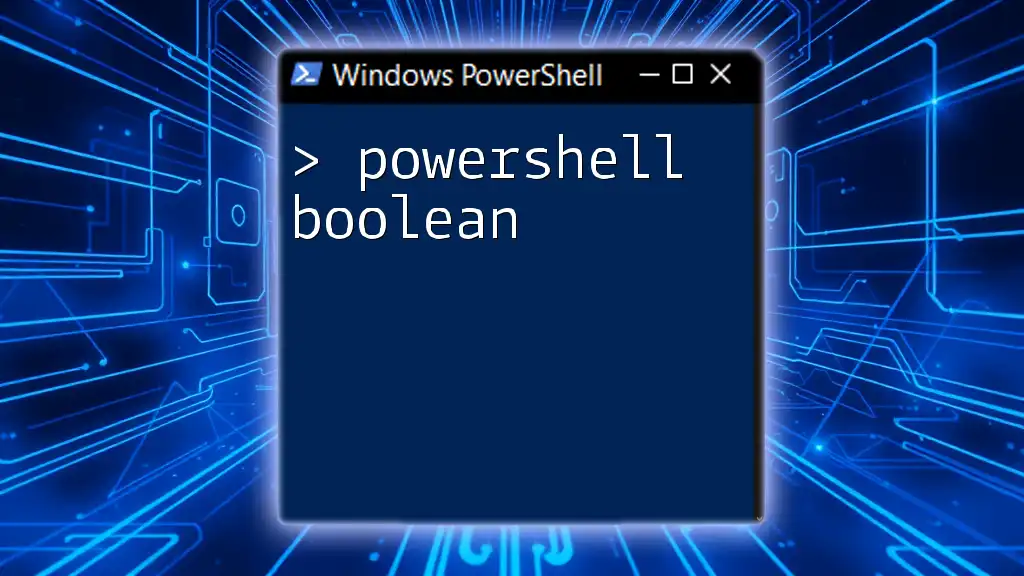
Common Patterns with Not Like
Using Wildcards Effectively
Wildcards are integral to using the "Not Like" operator effectively. The asterisk (`*`) can match any number of characters, while the question mark (`?`) matches a single character.
For example, the string "book" will return `True` when checked against the pattern "b??k".
Complex Patterns in "Not Like"
You can combine the "Not Like" operator with other conditions using logical operators like `-and` or `-or` for more refined checks.
Example:
$items | Where-Object { $_.Name -notlike "*test*" -and $_.Size -gt 100MB }
This complex filter checks that items are neither named with "test" nor below a specified file size.
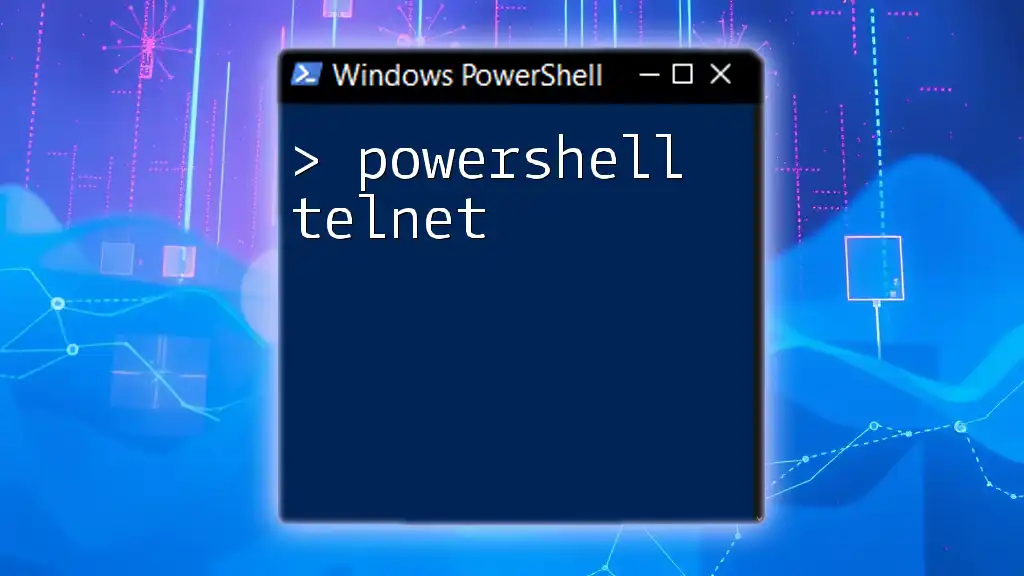
Troubleshooting Common Issues
Case Sensitivity in Comparisons
By default, PowerShell string comparisons are case-insensitive. However, if you require case sensitivity, you can use the `-clike` and `-cnotlike` operators instead.
Example:
"Example" -notlike "example" # Returns False
"Example" -clike "example" # Returns True
Identifying Errors when Using Not Like
Common pitfalls include incorrect syntax and misconfigured wildcards, which can lead to unexpected results. Always double-check your patterns and ensure the syntax is properly formatted.
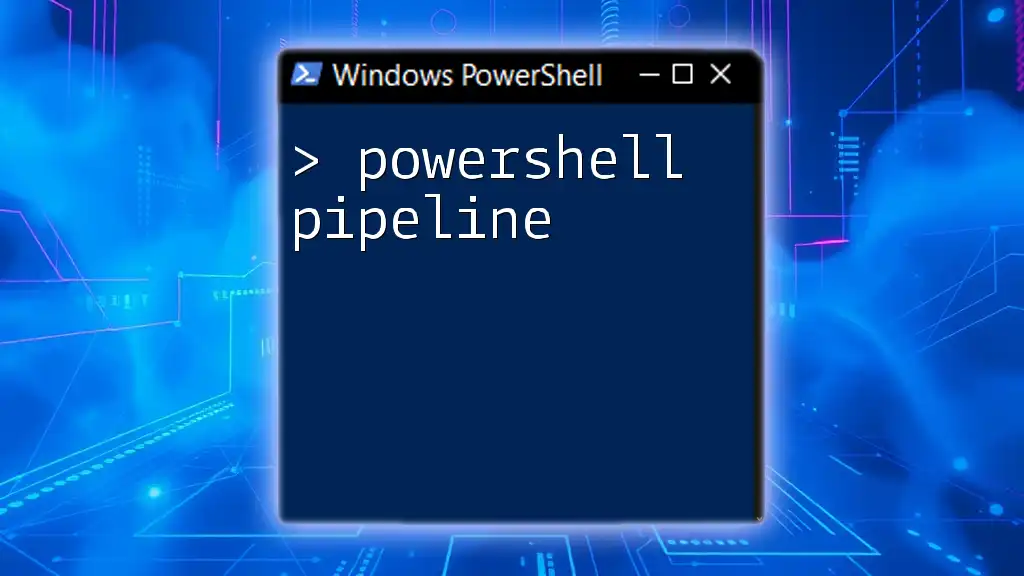
Alternatives to Not Like
Using Regular Expressions
For advanced filtering, leveraging regular expressions can provide even greater flexibility. PowerShell supports regex through the `-match` and `-notmatch` operators.
Example:
$strings | Where-Object { -not ($_ -match "^test") }
This will exclude any strings starting with "test", providing a powerful alternative to "Not Like".
Comparing with "Where-Object" and Other Operators
Using `Where-Object` to filter collections based on various criteria opens up new possibilities. For example, when deciding between "Not Like" and `Where-Object`, consider what best aligns with your filtering needs.
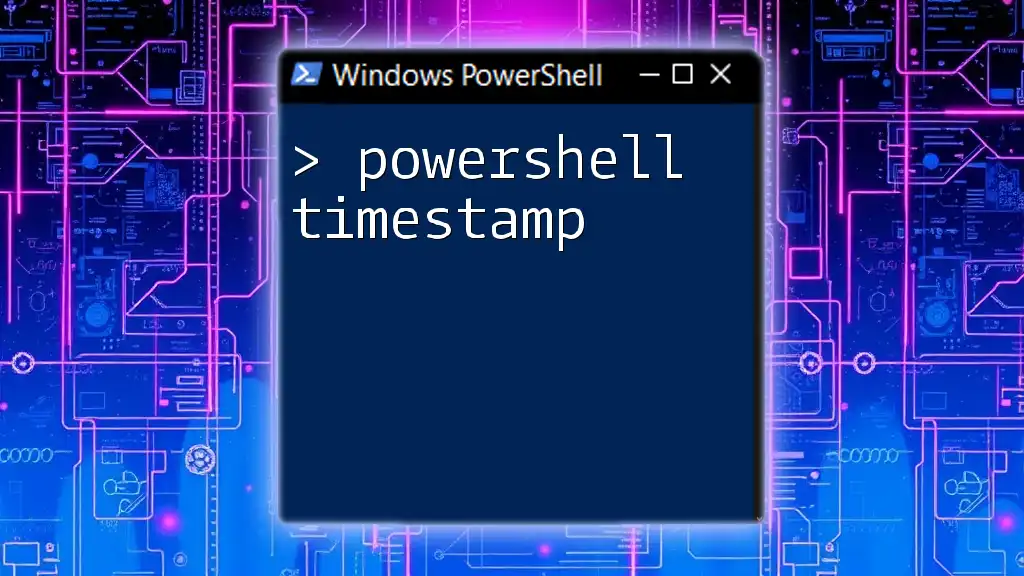
Conclusion
The "Not Like" operator in PowerShell is an invaluable tool for filtering data based on non-matching patterns. Understanding how to effectively implement this operator enhances your scripting capabilities, offering greater control over data selection processes.
Practicing the examples and scenarios outlined in this guide will empower you to leverage the full potential of the "Not Like" operator in your PowerShell scripts. Implement this knowledge in real-world applications for more efficient data handling and scripting!
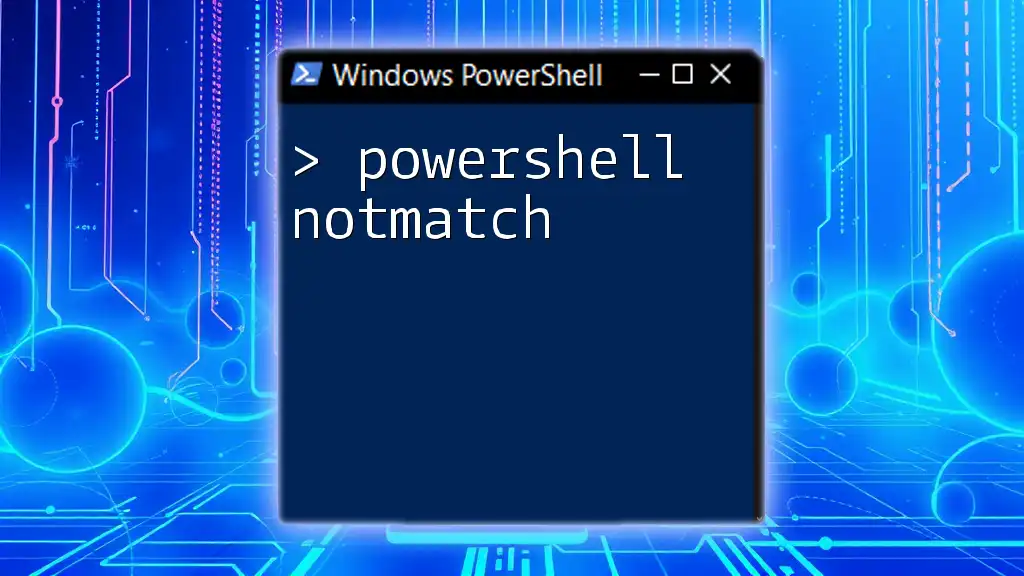
Additional Resources
- Check out the official PowerShell documentation for in-depth explanations and additional examples.
- Consider recommended PowerShell courses or books to further broaden your skills.
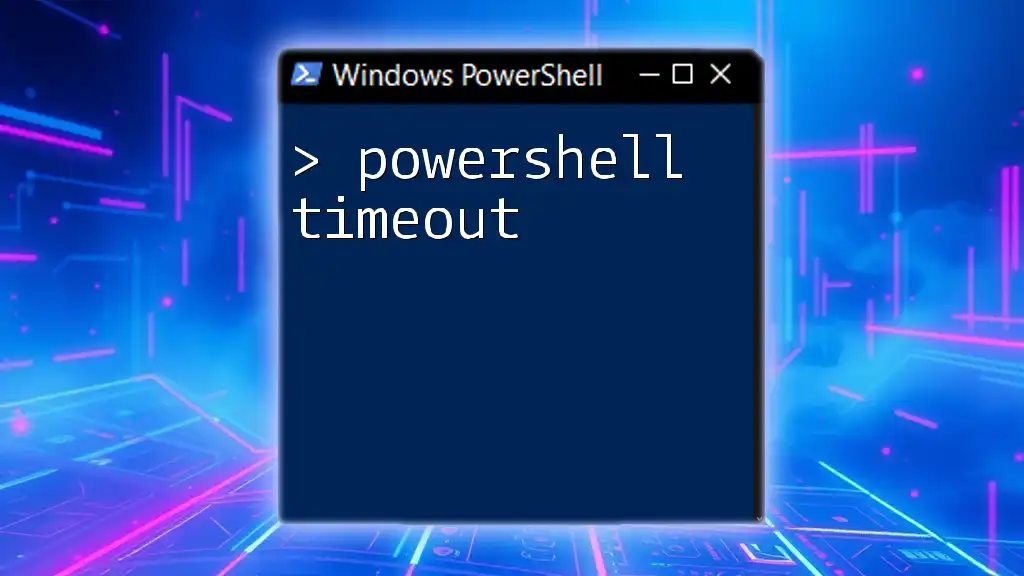
FAQs
What is the difference between Like and Not Like?
- The "Like" operator checks for a match against a specific pattern, while "Not Like" ensures that a match does not occur.
Can Not Like be used with arrays?
- Yes, you can easily apply "Not Like" to filter arrays using `Where-Object`.
How do I negate other comparison operators?
- Use the `-not` operator preceding any comparison to negate its result, similar to how "Not Like" is used with strings.