In PowerShell, the expression `-ne $null` is used to verify that a variable does not hold a null value, which is essential for ensuring that the variable has been assigned a meaningful value.
if ($variable -ne $null) {
Write-Host 'The variable is not null.'
} else {
Write-Host 'The variable is null.'
}
Understanding Null in PowerShell
What Does Null Mean in PowerShell?
In PowerShell, null is a special type that indicates the absence of a value. Unlike other data types, which can represent specific data points (such as strings, integers, and arrays), null represents a lack of data. The significance of null in PowerShell scripting cannot be overstated, as many operations and commands rely on verifying whether variables contain meaningful values or are simply uninitialized.
Why You Need to Check for Null
Understanding and checking for null values is crucial for several reasons:
- Error Prevention: If a script attempts to use a null value in an operation, it may result in runtime errors. For example, trying to call a method on a null object will generate an exception.
- Data Integrity: When processing data, null values can skew results or lead to incorrect conclusions if not handled correctly. Checking for null ensures that only valid data is processed.
- Control Flow: Many scripts use conditional logic that relies on distinguishing between valid data and null values. Proper checks can lead to more efficient and precise execution paths.
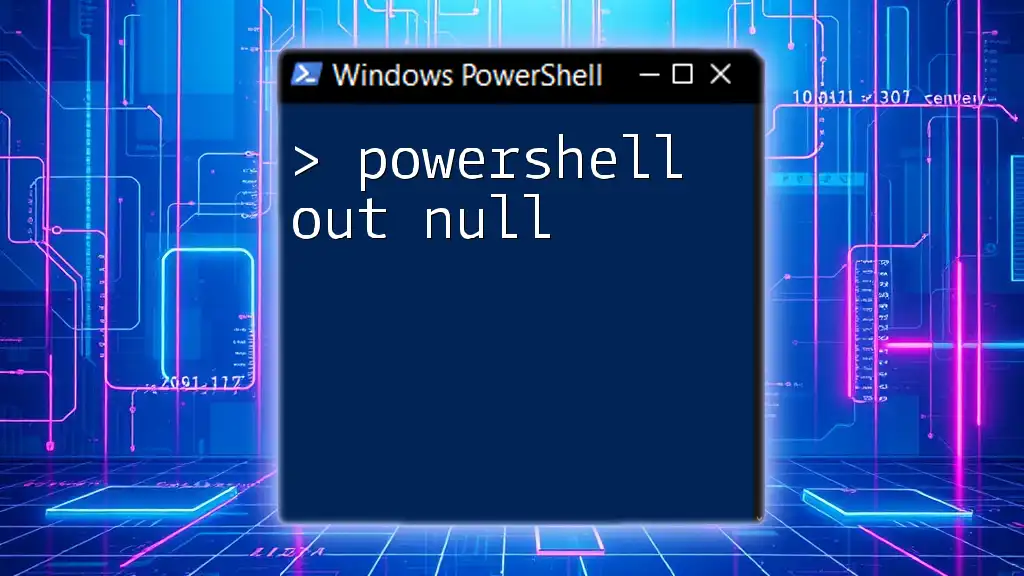
The Syntax of Null Comparison
The Not Equal Operator
In PowerShell, the not equal operator is represented by `-ne`. This operator is vital for comparing values to determine if they differ. The syntax for comparing a variable with null is straightforward:
$value -ne $null
It is important to ensure that there are no extra spaces within the command. The command should be clear and concise for optimal performance.
Code Snippet: Basic Null Comparison
Here's a simple example of how you can use the not equal operator to check for null:
$value = $null
if ($value -ne $null) {
Write-Output "Value is not null"
} else {
Write-Output "Value is null"
}
In this example, since `$value` is explicitly set to `null`, the output will be "Value is null". This simple check establishes a clear path for the subsequent logic in your scripts.
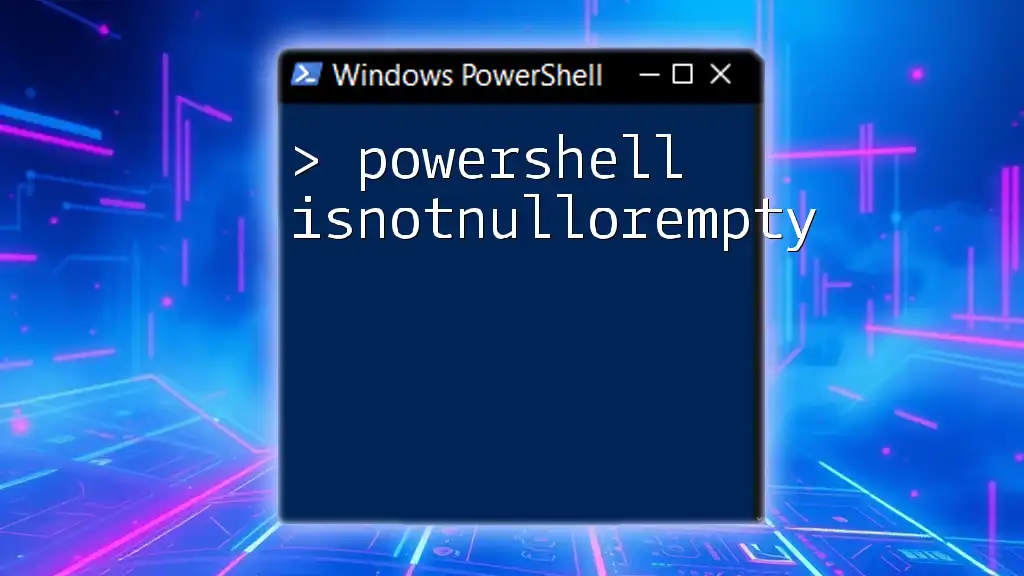
Practical Applications of Checking for Null
Conditional Statements with Null Comparison
Conditional statements in PowerShell allow you to execute specific blocks of code depending on the condition's truth. When dealing with potential null values, if statements are essential.
Code Snippet: Conditional Logic
Below is an example that illustrates the usage of an if statement:
$config = Get-Configuration
if ($config -ne $null) {
# Proceed with processing
Write-Output "Configuration loaded successfully."
} else {
Write-Output "Configuration is missing."
}
In this case, the script first attempts to retrieve a configuration. If the `$config` variable is not null, the next steps are executed; otherwise, it warns the user that the configuration is missing.
Loops and Null Values
Null values can also impact the way loops function in PowerShell. When iterating over collections, it's important to verify whether each item is null to prevent unnecessary errors.
Code Snippet: Loop Example
Here’s how you might implement a null check in a loop:
foreach ($item in $items) {
if ($item -ne $null) {
Write-Output "Processing item: $item"
}
}
In this code snippet, the loop will only process `$item` if it is not null. This practice enhances the script's robustness by avoiding potential errors when accessing null items.
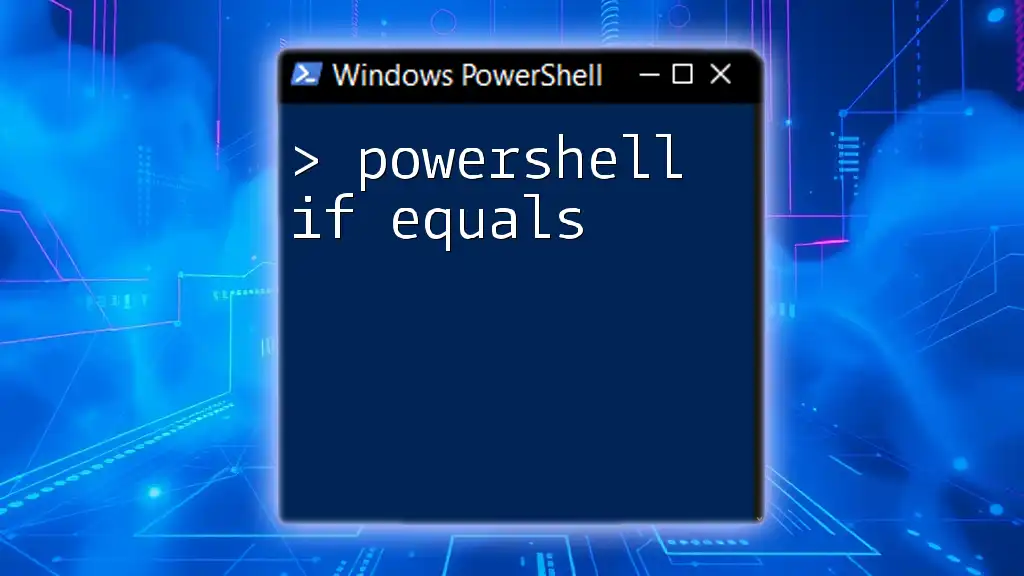
Advanced Techniques and Best Practices
Using `Where-Object` with Null Checks
The `Where-Object` cmdlet is an excellent tool when you need to filter collections based on specific criteria, including null checks. It allows you to retrieve only the objects that meet your defined conditions.
Code Snippet: Filtering Nulls
Here's an example of how to filter out null values from a dataset:
$data = Get-Data
$filteredData = $data | Where-Object { $_ -ne $null }
In this case, `$filteredData` will only include items that are not null, ensuring that further processing only involves valid data.
Functions and Null Validation
When writing functions in PowerShell, validating the parameters for null values is essential. This ensures that your function only processes valid inputs and provides appropriate feedback to the user.
Code Snippet: Function Example
Consider the following function definition:
function Process-Data {
param (
[Parameter(Mandatory=$true)]
[string]$inputData
)
if ($inputData -ne $null) {
# Process the data
Write-Output "Data processed: $inputData"
} else {
Write-Output "Input data cannot be null."
}
}
Here, the function `Process-Data` enforces that `$inputData` is mandatory. If it is null, the function responds appropriately, preventing further execution with invalid data.
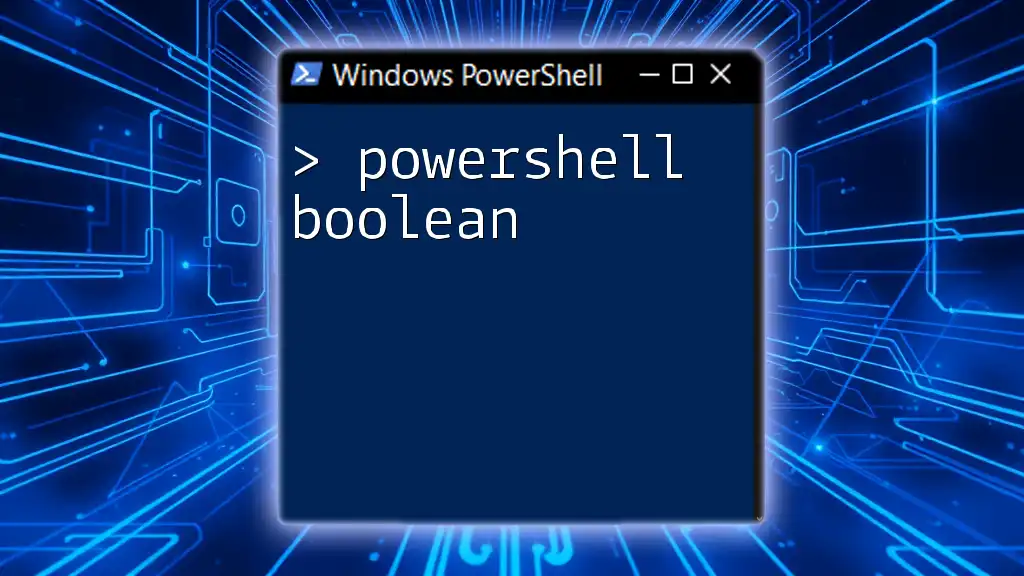
Common Mistakes to Avoid
Forgetting Null Checks
One common pitfall when programming in PowerShell is neglecting to check for null values. This can lead to script failures, particularly when the execution flow assumes the presence of valid data. Always use null checks to maintain the script's integrity.
Confusing Null with Other Values
It’s crucial to differentiate between null, an empty string `""`, and numeric zero `0`. These values can be valid yet should be treated differently in your scripts.
Best Practice: Always define clear logic for what constitutes a valid value in your specific use case to avoid misinterpretation.
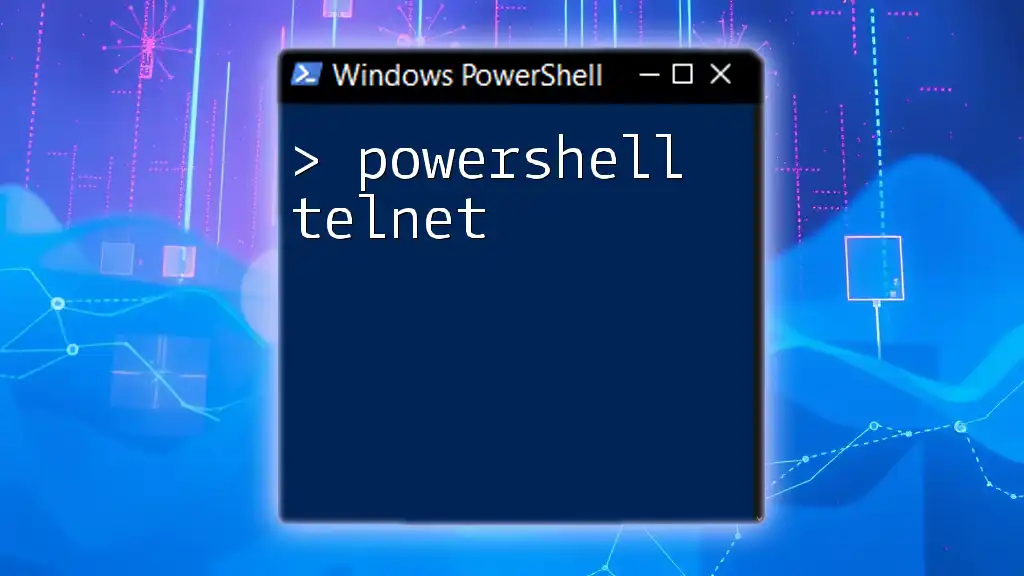
Conclusion
In summary, understanding how PowerShell not equal null works is fundamental for creating robust scripts. By mastering null comparisons, you can prevent errors, enhance data integrity, and develop more effective control flows in your PowerShell scripts. Continued practice and application of these concepts will allow you to navigate PowerShell with greater confidence.
Next Steps for Learning PowerShell
As you continue your PowerShell journey, consider experimenting with different scenarios involving null checks in your scripts. Valuable resources, such as books and online tutorials, can provide further insights and depth into mastering PowerShell scripting.
Takeaway: Always remember to check for null values; this small yet powerful practice can significantly enhance the effectiveness and reliability of your scripts.