The "if equals" statement in PowerShell allows you to execute a block of code conditionally based on whether two values are equal.
if ($var1 -eq $var2) {
Write-Host 'The variables are equal!'
}
Understanding the If Statement in PowerShell
What is an If Statement?
The `if` statement is a fundamental building block in PowerShell and other programming languages. It allows you to execute code conditionally, meaning that the code inside the `if` block will only run if the specified condition evaluates to true. This enables you to create logical branching in your scripts.
The syntax for an `if` statement is as follows:
if (<condition>) {
<code>
}
Comparing Values in PowerShell
Comparison is a crucial part of using conditional statements effectively. In PowerShell, you can compare values using a variety of operators. The most common are:
- `-eq`: Equal to
- `-ne`: Not equal to
- `-gt`: Greater than
- `-lt`: Less than
Understanding these operators is essential for implementing conditional logic in your PowerShell scripts.
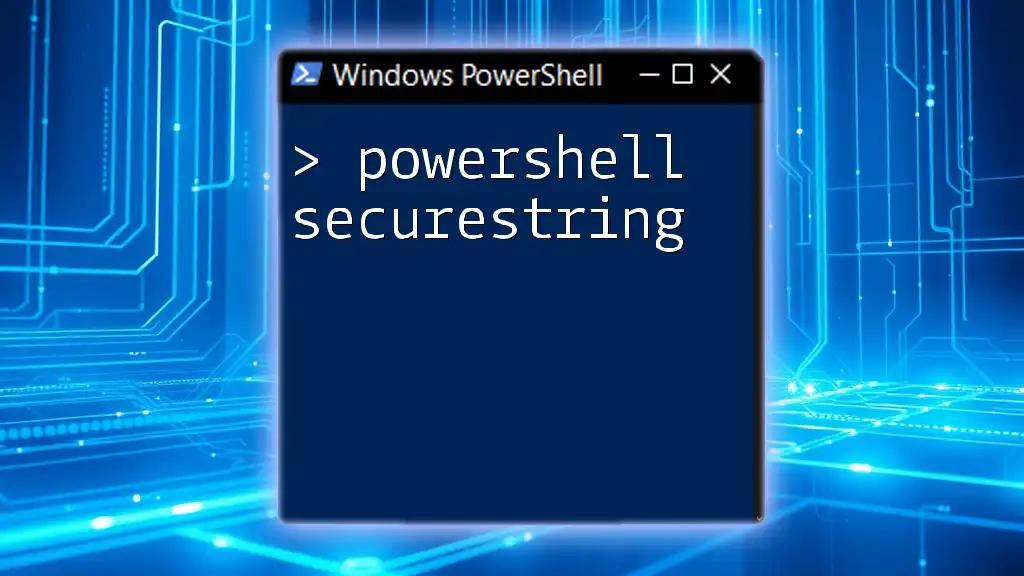
The Equality Operator: `-eq`
What is `-eq`?
The `-eq` operator is used to compare two values to determine if they are equal. When you use this operator, PowerShell evaluates whether the values on either side of the operator are the same.
Using `-eq` in PowerShell
The basic structure for using the `-eq` operator within an `if` statement is as follows:
if ($value1 -eq $value2) {
<code>
}
When using the `-eq` operator, if `$value1` equals `$value2`, the code inside the block will execute.
Example of Using `-eq`
To illustrate how the `-eq` operator works, consider the following example:
$num1 = 5
$num2 = 5
if ($num1 -eq $num2) {
Write-Host "The numbers are equal."
}
In this case, since both `$num1` and `$num2` are equal to `5`, the message "The numbers are equal." will be displayed.
Explanation: This example is straightforward, demonstrating how to evaluate two numerical variables for equality. The script shows the clear path from variable assignment to condition checking and execution of the command within the `if` block.
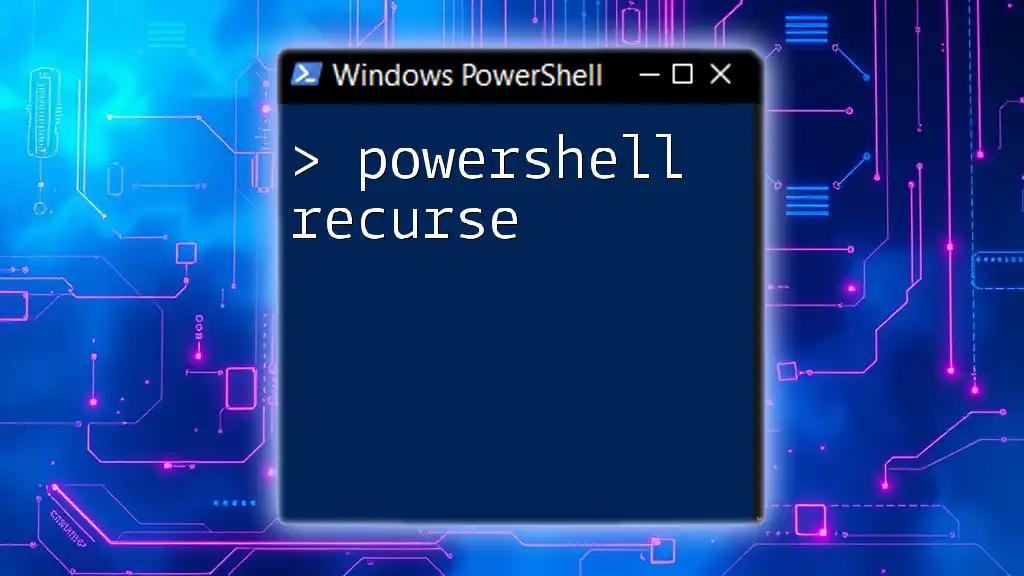
Advanced Usage of PowerShell If Equals
Multiple Conditions with If Statements
PowerShell allows you to check multiple conditions within a single `if` statement, making your scripts more versatile and powerful.
Using `-and` and `-or` operators
You can combine conditions using the `-and` and `-or` operators:
- The `-and` operator checks that all conditions are true.
- The `-or` operator checks if at least one of the conditions is true.
Example Code Snippet
$username = "admin"
$password = "1234"
if ($username -eq "admin" -and $password -eq "1234") {
Write-Host "Access Granted."
} else {
Write-Host "Access Denied."
}
Explanation: In this example, both conditions must be true for the message "Access Granted." to be displayed. If either the username is not `"admin"` or the password is not `"1234"`, the script will output "Access Denied." This demonstrates how to use combined conditional logic effectively.
Nested If Statements
Nested `if` statements allow you to evaluate further conditions within an `if` block. This is useful for more complex logic that cannot be adequately represented with a simple linear flow.
Example Code Snippet
$age = 20
if ($age -ge 18) {
if ($age -lt 21) {
Write-Host "You are an adult but not old enough to drink."
} else {
Write-Host "You are old enough to drink."
}
}
Explanation: In the above example, the first condition checks if the person is an adult (18 or older). If true, it checks if they are younger than 21. This nested condition allows for more granular control over the flow of execution, leading to different outputs based on age.
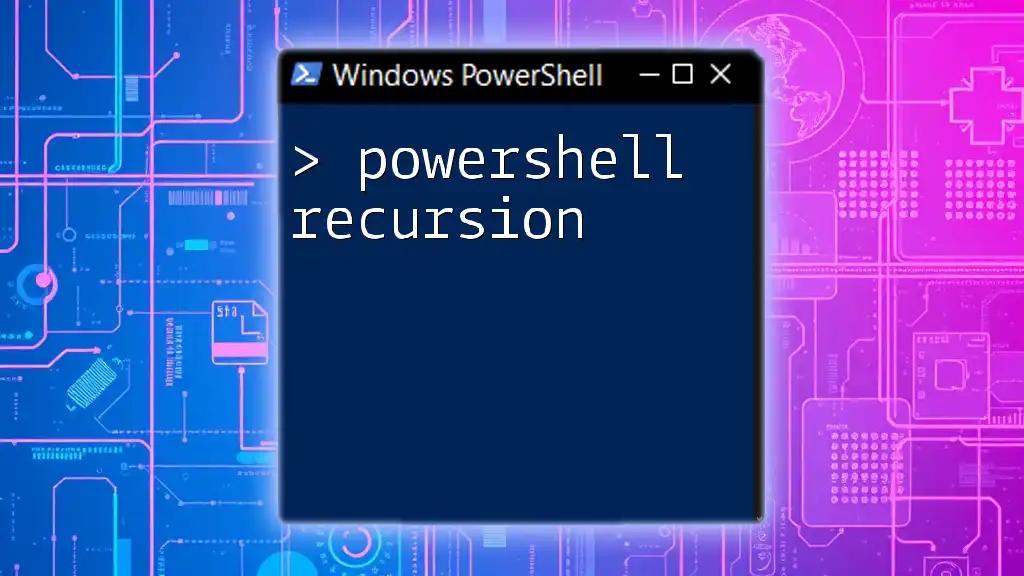
Common Mistakes and Troubleshooting
Case Sensitivity in Comparisons
When comparing strings, it’s crucial to consider that PowerShell is case-insensitive by default. However, if you want to enforce case sensitivity, you can use the `-ceq` operator, which stands for "case-sensitive equals."
Comparing Different Data Types
Be cautious when comparing values of different data types. PowerShell converts data types implicitly; for instance, comparing a string with a number can lead to unexpected outcomes. It’s best to ensure that the types match to avoid logical errors in your scripts.

Best Practices for Using If Equals in PowerShell
Writing Clean and Readable Code
Creating readable and maintainable code should always be your priority. Use clear variable names and take advantage of formatting to separate conditions. This will make your scripts easier to follow and debug.
Using Comments Effectively
Incorporating comments in your scripts can significantly enhance understanding. Use comments to explain complex logic and decisions to help both you and others who may read your code in the future.

Conclusion
Understanding how to use the `if` statement along with the `-eq` operator empowers you to write effective PowerShell scripts. This knowledge will encourage you to implement conditional logic, enhancing your automation and scripting capabilities. With practice, you will find increasing complexity in your conditions can lead to more robust and dynamic PowerShell scripts!
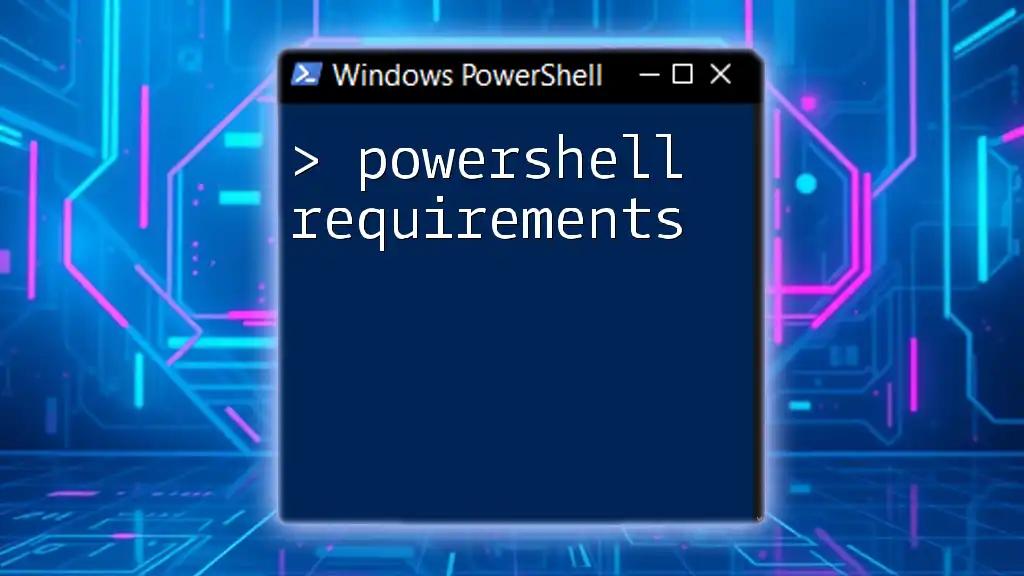
Additional Resources
For those looking to further expand their knowledge, consider checking the official PowerShell documentation, engaging in community forums, or exploring additional tutorials that align with your learning style. Learning through practice is key!