To easily retrieve your external IP address using PowerShell, you can use the following command which queries a web service to fetch the public IP.
Invoke-RestMethod -Uri 'http://api.ipify.org'
Understanding External IP
What is an External IP Address?
An external IP address is the public address assigned to your device by your Internet Service Provider (ISP) that allows it to connect to the internet. In contrast, internal IP addresses are used within a local network and are not reachable from the outside world. Understanding the differences between external and internal IP addresses is crucial for tasks involving remote access, VPNs, and network configuration.
Why You Might Need Your External IP Address
You may need your external IP address for various reasons. For instance, if you are setting up a VPN, configuring a firewall, or troubleshooting connectivity issues, knowing your external IP can help you identify the source of problems in network communications. Additionally, if you are using cloud services such as AWS or Azure, you often need to provide your external IP to allow secure connections.

PowerShell Basics
Introduction to PowerShell
PowerShell is a powerful scripting language and automation tool that simplifies the management of system tasks and configurations. It is built on the .NET framework and provides a command-line interface that allows users to execute complex tasks with simple commands called cmdlets. With its straightforward syntax and robust features, PowerShell is an essential tool for both beginners and experienced users alike.
Setting Up PowerShell
To access PowerShell, you can easily navigate to it on various Windows versions:
- Windows 10/11: Search for "PowerShell" in the Start menu or use the Run dialog (Windows + R) and type `powershell`.
- Windows 7/8: Find it in the Start menu under Accessories.
New users can take advantage of the PowerShell Integrated Scripting Environment (ISE), which provides a graphical interface, syntax highlighting, and debugging features to make writing scripts easier.
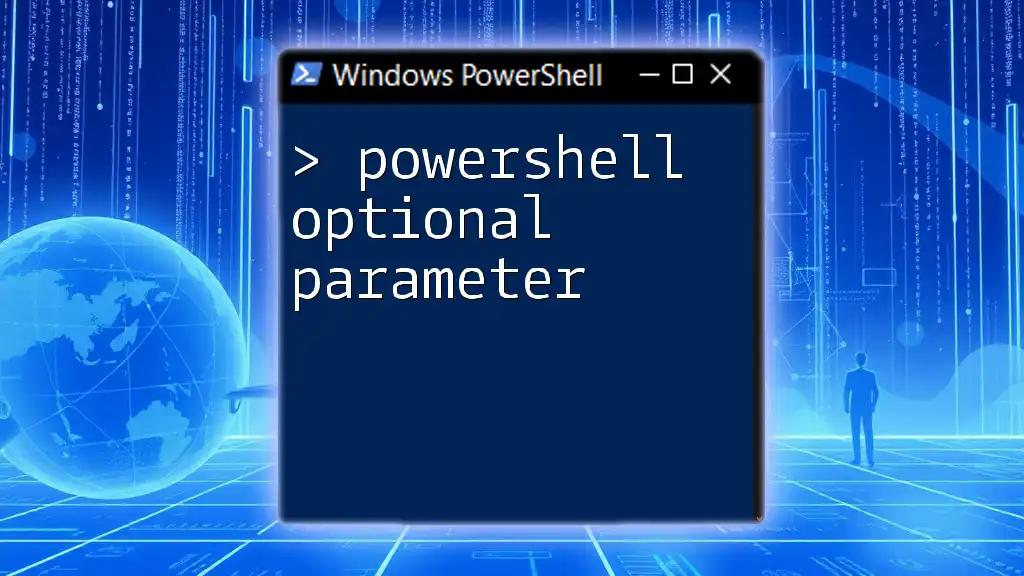
Fetching Your External IP Address with PowerShell
Using Web Requests
What Are Web Requests?
Web requests enable scripts to interact with online resources by fetching data. In the context of obtaining your external IP address, this means sending a request to a web service that can return your public IP information.
Code Snippet: Fetching IP from a Web Service
To retrieve your external IP address using PowerShell, you can use the `Invoke-RestMethod` cmdlet, which simplifies the process of sending web requests. Here’s how you can do it:
$externalIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Write-Host "Your external IP address is: $externalIP"
In this snippet, we use `api.ipify.org`, a reliable web service that returns the public IP in plain text. The `Write-Host` command is then used to display the retrieved IP.
Alternative Web Services for IP Retrieval
Besides `ipify`, there are other popular web services you can also use to fetch your external IP address. Some examples include:
Example Code Snippet: Using a Different Web Service
You can easily switch to a different service using the same method. For instance, using the AWS service:
$externalIP = Invoke-RestMethod -Uri "https://checkip.amazonaws.com/"
Write-Host "Your external IP address is: $externalIP"
Error Handling in PowerShell
Why Error Handling is Important
To enhance user experience and generate reliable scripts, incorporating error handling is integral. It allows users to understand and troubleshoot issues that may arise while executing commands.
Implementing Error Handling
You can manage errors effectively with `try-catch` blocks. Here’s how to ensure that your script can gracefully handle potential problems:
try {
$externalIP = Invoke-RestMethod -Uri "http://api.ipify.org"
Write-Host "Your external IP address is: $externalIP"
} catch {
Write-Host "Error fetching external IP. Check your internet connection."
}
In this example, if the web request fails due to connectivity issues, the catch block displays a user-friendly error message.
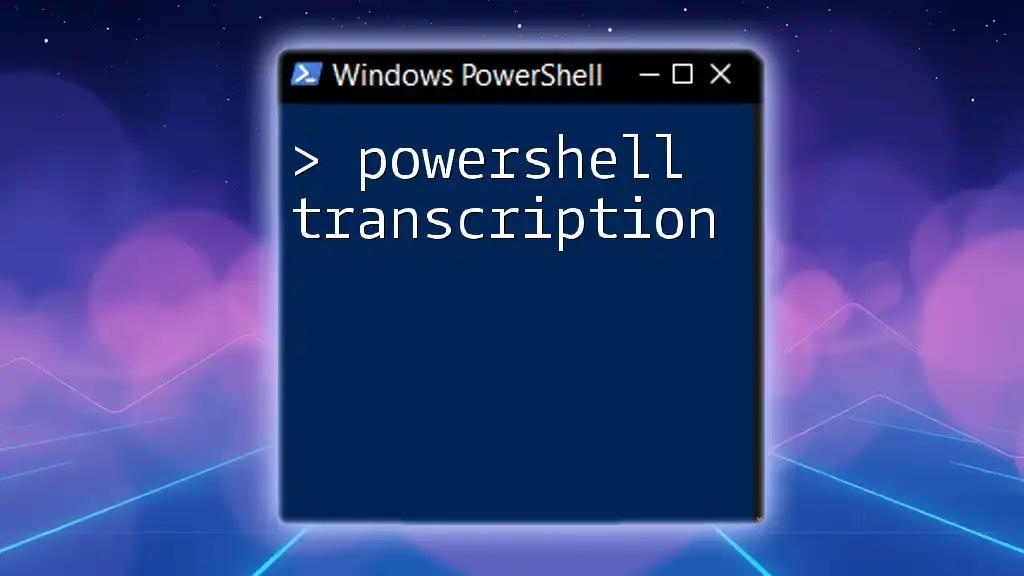
Storing and Using the External IP Address
Saving the External IP for Future Use
Once you fetch your external IP, you may want to store it for future reference. Here’s how you can save it to a file for later access:
$externalIP | Out-File -FilePath "ExternalIP.txt"
This command writes the external IP to a text file named `ExternalIP.txt`, allowing you to access it whenever needed.
Automating IP Retrieval
Scheduling Tasks
You might want to automate the retrieval of your external IP address. This can be done by creating a scheduled task that runs your PowerShell script at predetermined intervals. Use the Task Scheduler in Windows to set this up.
Basic Logging of IP Address Retrieval
For logging purposes, you can expand your script to include timestamps. Here’s a snippet that logs the IP with date and time:
$externalIP = Invoke-RestMethod -Uri "http://api.ipify.org"
$Timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"$Timestamp - $externalIP" | Add-Content -Path "IPLog.txt"
This method appends the current timestamp along with the external IP address to a log file named `IPLog.txt`, creating a history of the retrieved addresses over time.
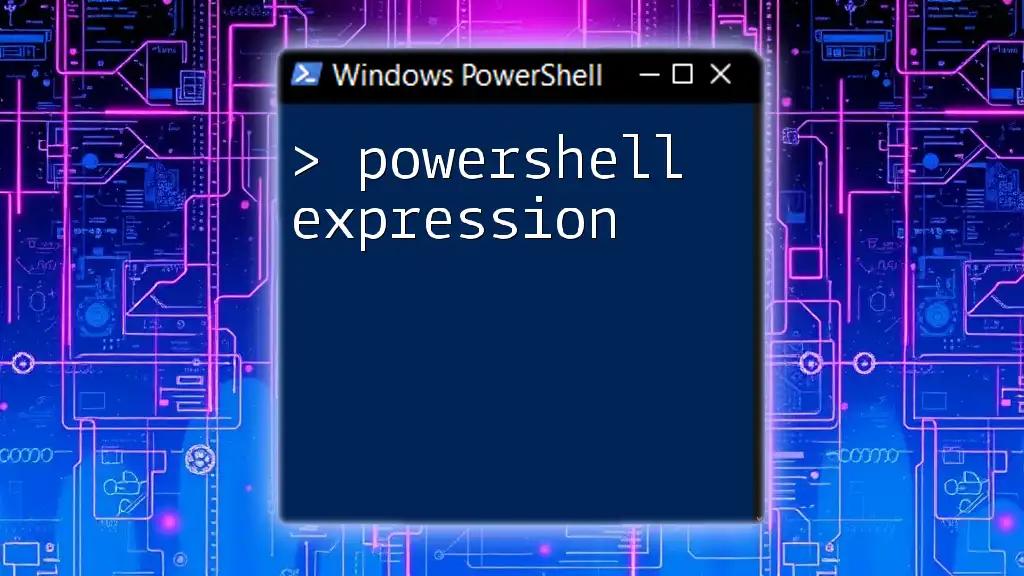
Troubleshooting Common Issues
Connectivity Problems
If you encounter issues fetching your external IP address, check your network connection. Ensure that your device is connected to the internet and that there are no disruptions on your ISP's end.
PowerShell Execution Policy
Sometimes, PowerShell scripts may not execute due to the system's execution policy settings. Adjust these settings by running the following command in an elevated PowerShell window:
Set-ExecutionPolicy RemoteSigned
This command allows scripts created on the local machine to run while still protecting against potentially harmful remote scripts.

Conclusion
Knowing how to efficiently retrieve your PowerShell external IP can greatly enhance your networking capabilities and streamline various internet-related tasks. With the commands and examples provided, you are now equipped to fetch, store, and even automate the logging of your external IP address.
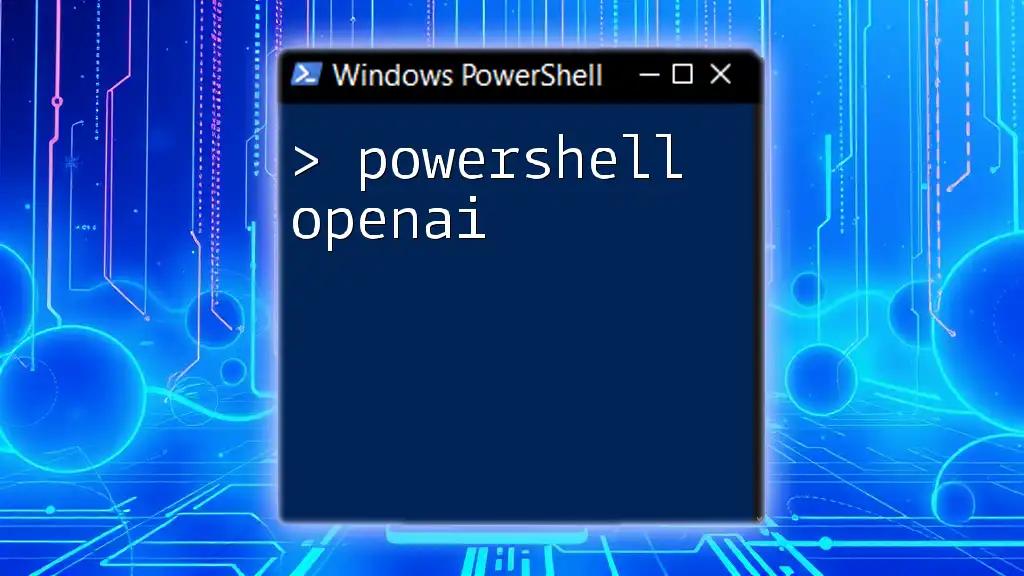
Call to Action
We invite you to explore your experiences using PowerShell to manage networking tasks. Share your stories and questions in the comments below! Additionally, check out our comprehensive resources and courses that delve deeper into PowerShell commands and automation techniques.
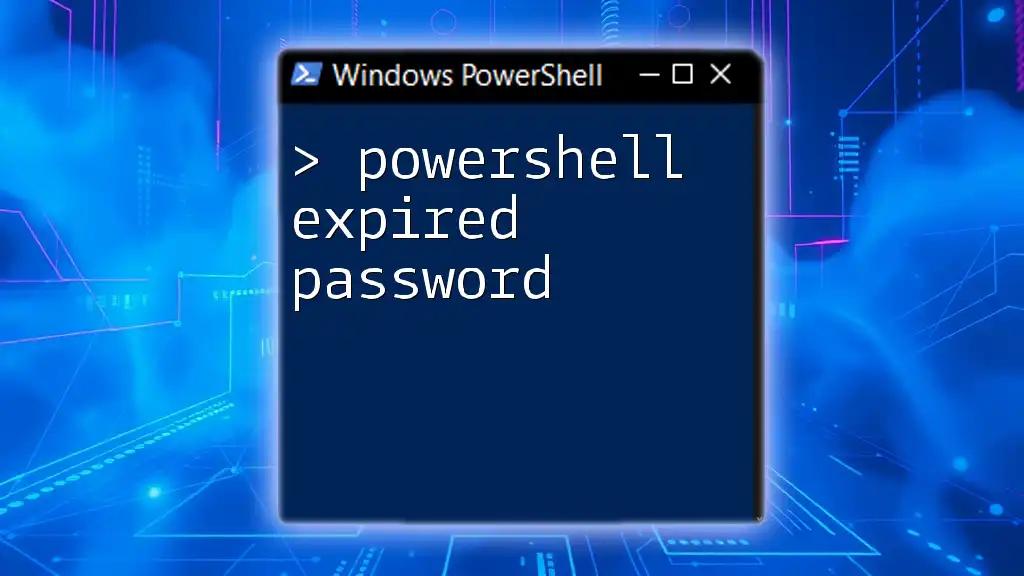
Additional Resources
For further exploration, consider reviewing the official PowerShell documentation or recommended reading materials tailored for both beginners and advanced users looking to deepen their knowledge.