"PowerShell and OpenAI can be integrated to leverage the power of AI-driven automation in scripting, enhancing productivity and efficiency in task execution."
# Example: Using OpenAI API to generate text with PowerShell
$apiKey = "your-api-key-here"
$prompt = "Generate a PowerShell script that lists all files in a directory."
$uri = "https://api.openai.com/v1/engines/davinci-codex/completions"
$response = Invoke-RestMethod -Uri $uri -Method Post -Headers @{ "Authorization" = "Bearer $apiKey" } -Body @{
prompt = $prompt
max_tokens = 150
temperature = 0.7
} | ConvertFrom-Json
Write-Host $response.choices.text
Understanding PowerShell
What is PowerShell?
PowerShell is a powerful scripting and automation tool designed for system administrators. It enables users to perform complex tasks with ease by combining command-line capabilities with a scripting language. Unlike traditional command-line interfaces, PowerShell utilizes an object-oriented approach, allowing users to work with .NET objects directly rather than text-based output.
Why Use PowerShell?
PowerShell offers several advantages that make it an ideal choice for automation and system management:
- Automation Capabilities: Automate repetitive tasks such as user creation, system updates, and data processing, which can save significant time and reduce errors.
- Integrated Scripting Language: It provides built-in support for handling loops, conditionals, and functions, empowering users to create sophisticated scripts.
- Enhanced Management: PowerShell seamlessly integrates with various Microsoft products and services, making it a go-to tool for managing both local and remote systems.
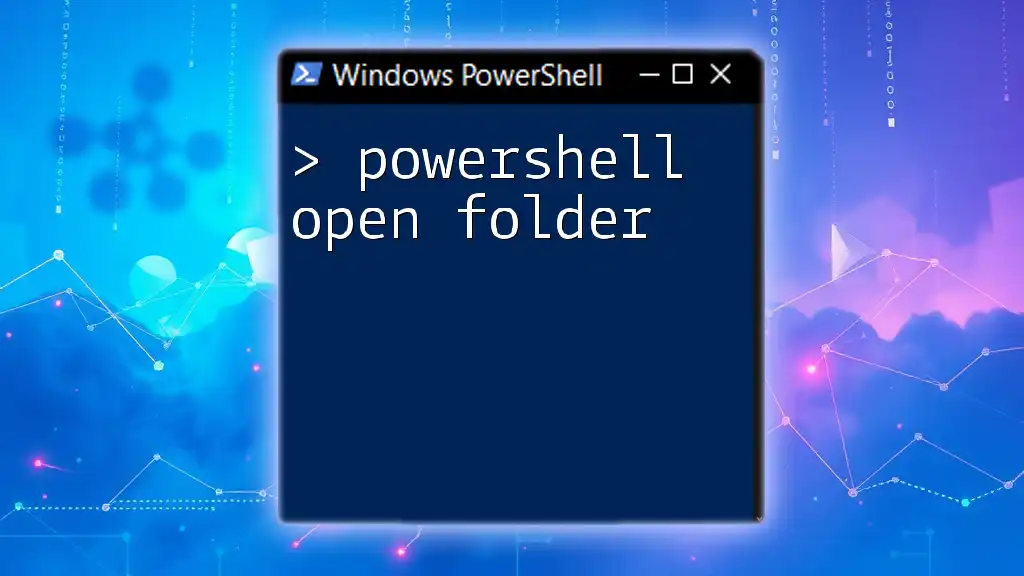
Introducing OpenAI
What is OpenAI?
OpenAI is an artificial intelligence research organization that aims to develop and promote friendly AI for the benefit of humanity. Their models, such as GPT-3, are designed to understand and generate human-like text, making them incredibly versatile across various applications—from chatbots to content creation.
Advantages of Using OpenAI
By leveraging OpenAI’s capabilities, users can benefit from:
- Natural Language Processing Abilities: OpenAI can interpret and generate text based on complex prompts, making it easier to interact with technology using human language.
- Rapid Prototyping of Applications: Developers can quickly create prototypes for applications that require advanced language understanding or generation.
- Versatility Across Industries: From customer support to data analysis, OpenAI serves diverse sectors, enhancing productivity and insights.
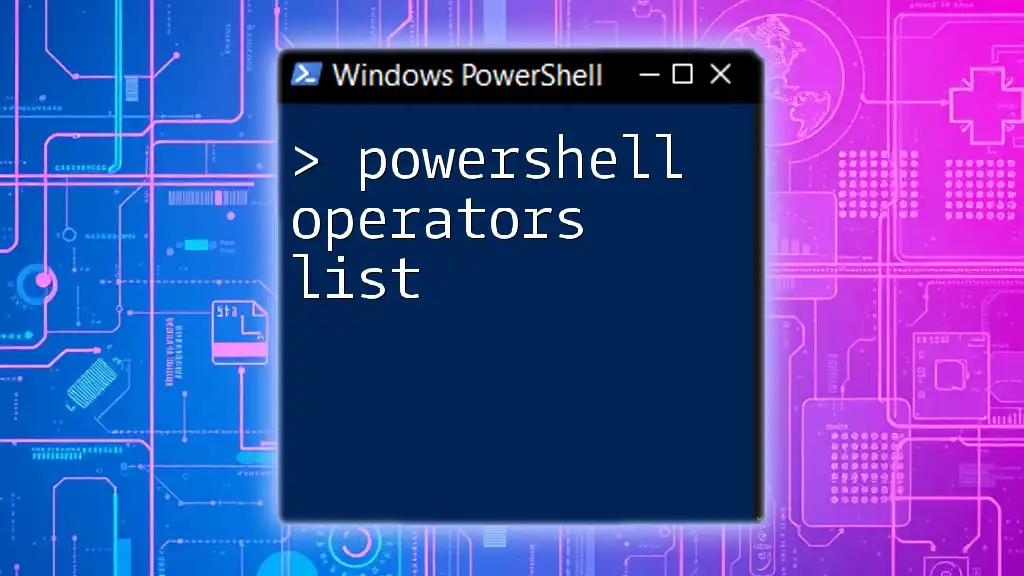
Combining PowerShell with OpenAI
The Potential of PowerShell and OpenAI
The integration of PowerShell and OpenAI opens possibilities in automation, data analysis, and system management. Imagine generating monthly reports automatically, summarizing logs in natural language, or even developing chatbots to assist users—all achievable by combining these two powerful technologies.
Requirements for Integration
Necessary Skills
To make the most of PowerShell and OpenAI, users should have:
- A basic knowledge of PowerShell scripting. Familiarity with commands, functions, and script structures is essential.
- An understanding of API usage to facilitate communications between PowerShell and OpenAI.
Tools and Libraries
To effectively use PowerShell with OpenAI, you will need:
- PowerShell Modules for HTTP Requests: Tools like `Invoke-RestMethod` to interact with web APIs.
- Libraries for JSON Data Handling: Using PowerShell's built-in capabilities to parse JSON responses from the API.
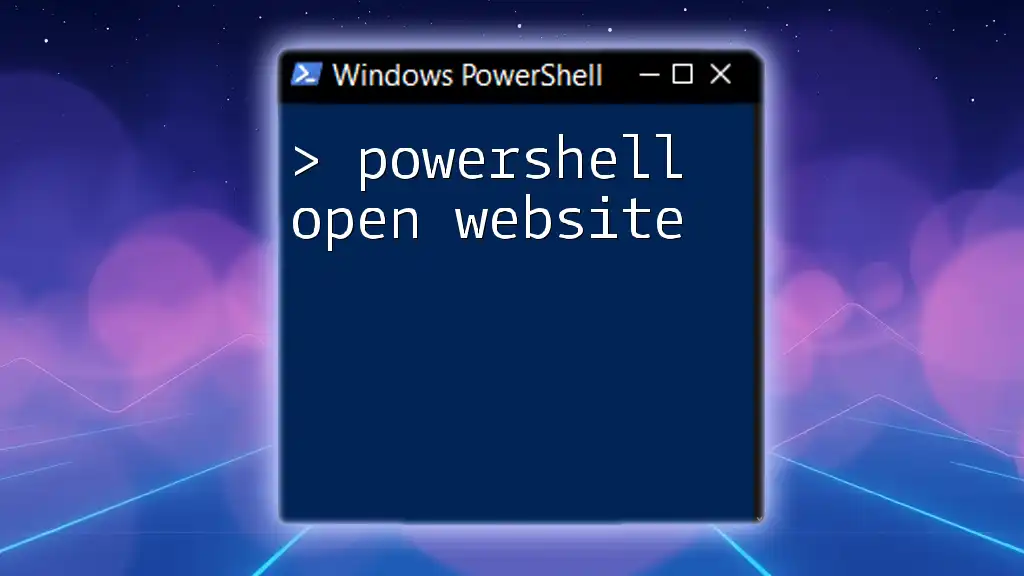
Getting Started with PowerShell and OpenAI
Setting Up the Environment
Before diving into integration, ensure you have PowerShell installed. If you're using Windows, PowerShell comes pre-installed. You can verify by running the following command in the PowerShell prompt:
$PSVersionTable.PSVersion
Creating an OpenAI Account
To use the OpenAI API, follow these steps:
- Sign Up: Visit the OpenAI website and create an account.
- Obtain API Keys: Once logged in, navigate to the API section to generate your API key. Keep this key secure, as it grants access to OpenAI’s models.
Making Your First API Call
Overview of OpenAI API
The OpenAI API has various endpoints, including endpoints for text generation, text analysis, and more. It's essential to understand the rate limits and best practices to avoid issues.
Code Snippet: Basic API Call Example
Here’s how you can make your first API call using PowerShell to generate a response from GPT-3:
# Import necessary modules
Import-Module Invoke-RestMethod
# Define OpenAI API endpoint and key
$apiUrl = "https://api.openai.com/v1/engines/davinci-codex/completions"
$apiKey = "your_openai_api_key"
# Create the headers
$headers = @{
"Content-Type" = "application/json";
"Authorization" = "Bearer $apiKey"
}
# Define the request body
$body = @{
prompt = "How to automate tasks using PowerShell?"
max_tokens = 100
} | ConvertTo-Json
# Make the API call
$response = Invoke-RestMethod -Uri $apiUrl -Method Post -Headers $headers -Body $body
$response.choices.text
Explanation of the Code Snippet: In this example, we're setting up the API URL and headers, defining a prompt, and making a call to the OpenAI API. The expected output is the generated text based on the prompt provided. Ensure you replace `"your_openai_api_key"` with your actual API key.
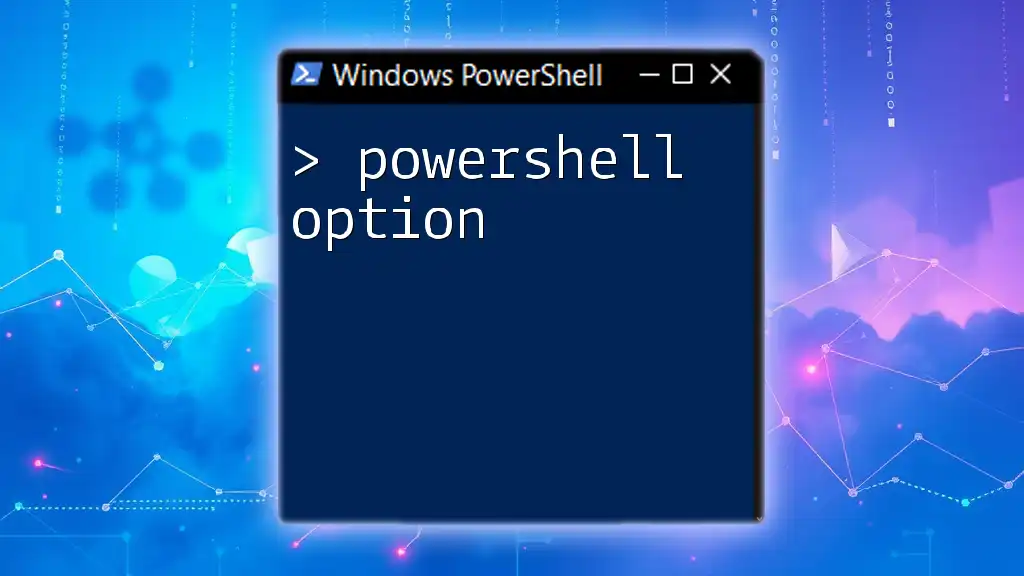
Practical Applications of PowerShell with OpenAI
Automating Reports
One compelling application is using OpenAI to generate natural language summaries from system data. For instance, you can create a PowerShell script that gathers system performance metrics and generates a report using OpenAI.
Data Analysis and Insights
OpenAI can also help analyze system logs or user data, providing insights in a human-readable format. For example, use the API to interpret and summarize trends in logs, helping teams to make informed decisions.
Enhanced Scripting
You can enhance your existing PowerShell scripts by incorporating AI capabilities. A simple chatbot can be created using PowerShell and OpenAI to assist users with basic inquiries about system status or helpdesk tickets.
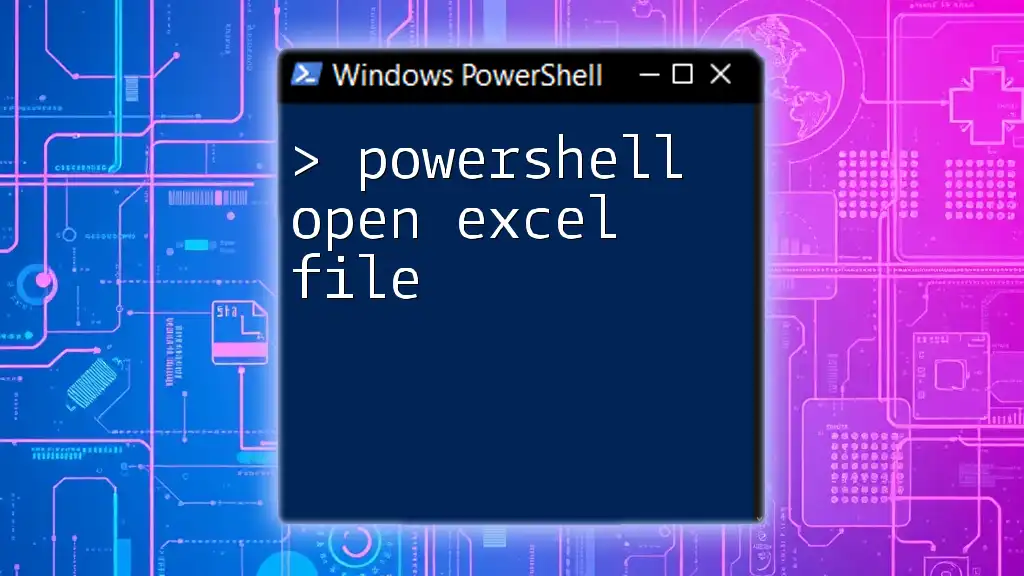
Challenges and Considerations
Common Errors and Troubleshooting
While integrating PowerShell with OpenAI, you may encounter common errors like authorization failures or incorrect prompt formats. Utilize PowerShell's debugging tools to troubleshoot. The `Try-Catch` statements can be particularly useful in such scenarios.
Ethical Considerations
While OpenAI enhances productivity, it’s critical to use this technology responsibly. Consider the impact of AI on jobs, privacy concerns, and ensure that the applications you create follow ethical guidelines.
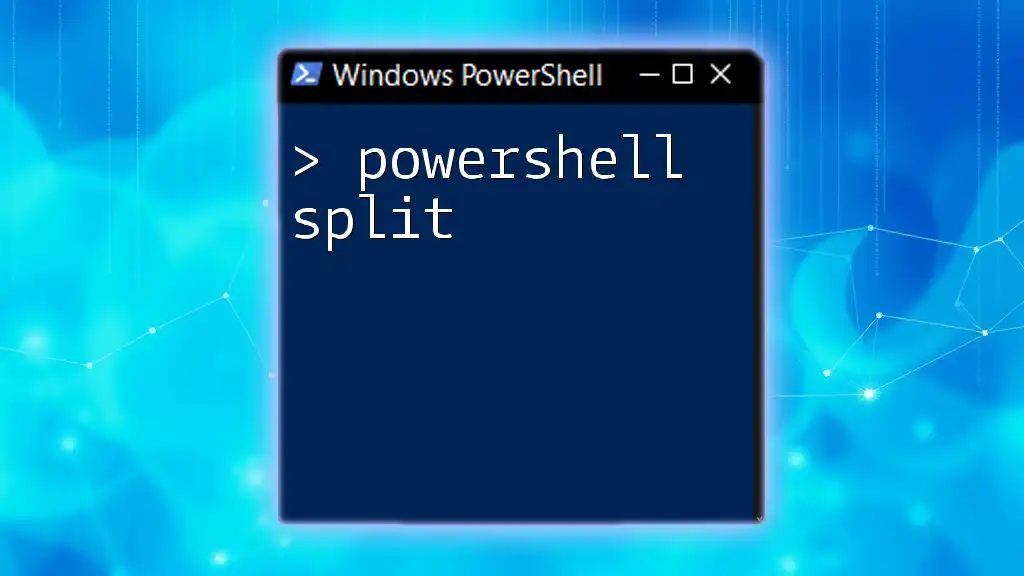
Conclusion
Recap of Key Takeaways
Integrating PowerShell and OpenAI offers immense potential for automating tasks, analyzing data, and enhancing user experiences. With the right skills and tools, anyone can leverage these technologies to improve efficiency and productivity.
Future Trends
As technology evolves, the collaboration between scripting languages like PowerShell and AI models will undoubtedly grow. Continuous learning and adaptation will be key to harnessing these advancements and staying ahead in the field.
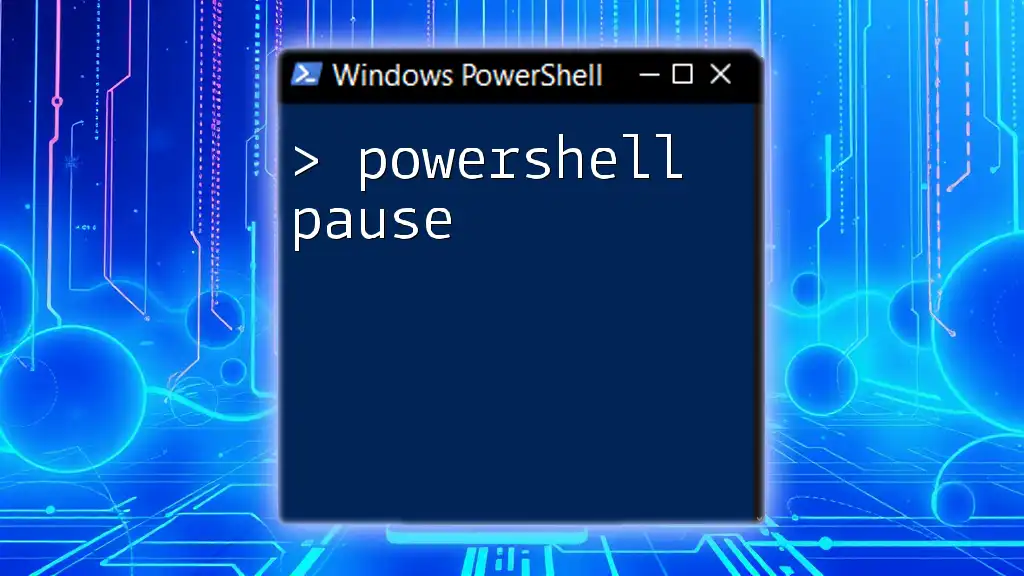
Resources
Recommended Reading
For further learning, consider exploring books on PowerShell scripting, OpenAI documentation, and relevant online courses that dive deeper into both technologies.
Community and Support
Engage with online forums, social media groups, and the PowerShell community to share knowledge, seek support, and stay informed about the latest updates in PowerShell and AI.