Certainly! The "PowerShell Enum" refers to a way to define a set of named constants in PowerShell, which helps in creating more readable and maintainable code.
Here's a simple code snippet to illustrate how to define and use an enum in PowerShell:
enum DaysOfWeek { Sunday = 0; Monday = 1; Tuesday = 2; Wednesday = 3; Thursday = 4; Friday = 5; Saturday = 6 }
# Example of using the enum
$today = [DaysOfWeek]::Friday
Write-Host "Today is: $today"
What is an Enum in PowerShell?
Definition of Enum
An enum (short for enumeration) in PowerShell is a distinct data type that consists of a set of named constants. Enums enable you to define variables that can hold a limited set of predefined values, which improves type safety and ensures that your scripts are both readable and maintainable. Unlike traditional data types, enums provide an efficient way to define and manage these sets of related constants.
Key Characteristics of PowerShell Enums
One of the key characteristics of PowerShell enums is their type safety. Instead of relying on plain integers or strings, an enum restricts the values a variable can assume, minimizing errors. Additionally, using enums enhances the readability of your scripts. For example, rather than using arbitrary numbers or strings to represent statuses, you can use descriptive names that clearly signify their meaning.
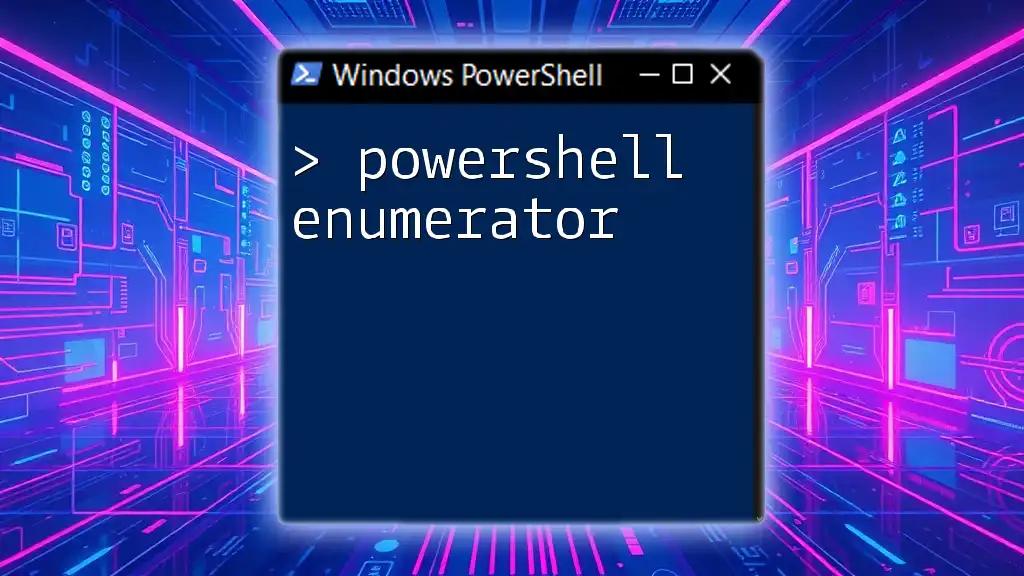
Creating and Using Enums in PowerShell
How to Create Your Own Enum
Creating your own enum in PowerShell is straightforward. You simply use the `enum` keyword followed by the name of the enumeration and its members enclosed in braces. Here's a simple example:
enum Color {
Red
Green
Blue
}
In this case, we've created an enum called `Color`, which has three members: `Red`, `Green`, and `Blue`. You can now use this enum to define variables that can only take these three values.
Accessing Enum Values
Listing Enum Members
You may want to retrieve a list of the members defined in your enums. PowerShell makes this easy with the `GetNames` method, as shown below:
[Color]::GetNames([Color])
This command will output an array of member names: `Red`, `Green`, and `Blue`.
Working with Enum Variables
Once you've defined an enum, you can create variables of that type. For example:
$myColor = [Color]::Red
In this script, `$myColor` is assigned the value `Red`, one of the defined enum members. When you examine `$myColor`, PowerShell identifies it as an instance of the `Color` enum.
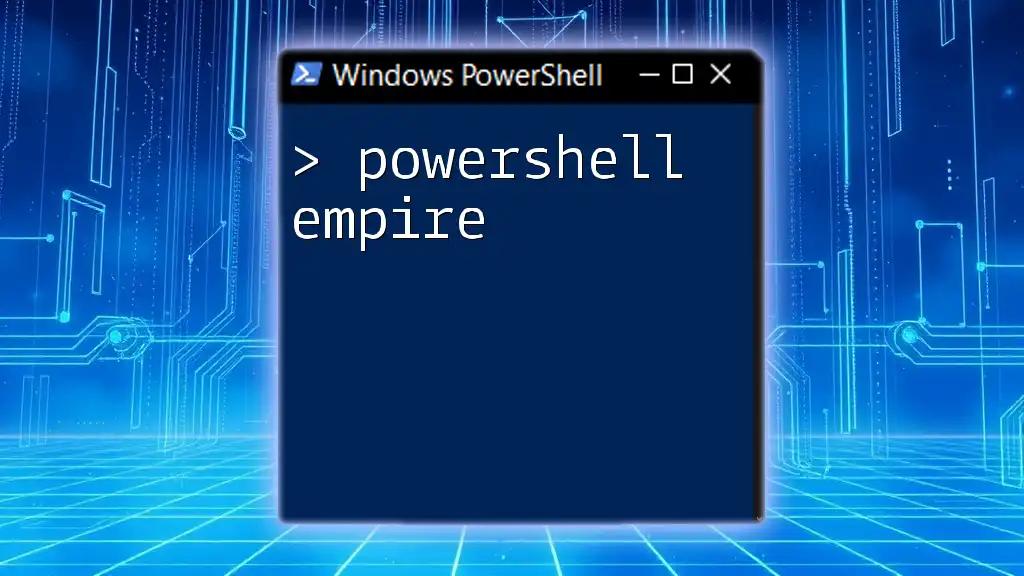
PowerShell Enumeration Techniques
Understanding the Enum's Underlying Integer Value
Each enum member in PowerShell is associated with an implicit integer value, starting at 0 for the first member and increasing by one for each subsequent member. If you want to extract the integer representation of an enum member, you can do so like this:
$value = [int][Color]::Green
In this case, `$value` will hold the integer `1`, given that `Green` is the second member of the `Color` enum.
Comparing Enum Members
Enums can also facilitate comparisons. You can compare different enum values to control logic flow within your scripts. Here’s an example to demonstrate:
if ($myColor -eq [Color]::Red) {
"It's Red!"
}
In this script, the condition checks if `$myColor` is equal to `Red`, and it outputs the string “It’s Red!” if the condition is true.

Advanced Enum Features
Associating Enums with Descriptive Text
For scenarios where you want to provide more context or descriptions for enum members, you can use the `[System.ComponentModel.Description]` attribute. This feature allows you to enhance the usability of your enums, making them more descriptive. A typical implementation may resemble:
enum Color {
[Description("Bright Red")]
Red
[Description("Lush Green")]
Green
[Description("Cool Blue")]
Blue
}
In this example, each color is associated with its description, making it easier for anyone reading the code to understand its purpose.
Extending Enums with Methods
PowerShell also allows you to add methods to enums, enhancing their functionality. This can be particularly useful for encapsulating behavior directly associated with enum members. Consider the following example:
enum Color {
Red
Green
Blue
[string] GetDescription() {
switch ($this) {
[Color]::Red { return "Bright Red" }
[Color]::Green { return "Lush Green" }
[Color]::Blue { return "Cool Blue" }
}
}
}
In this extended `Color` enum, we added a method called `GetDescription()` that returns a descriptive string for each color when invoked.
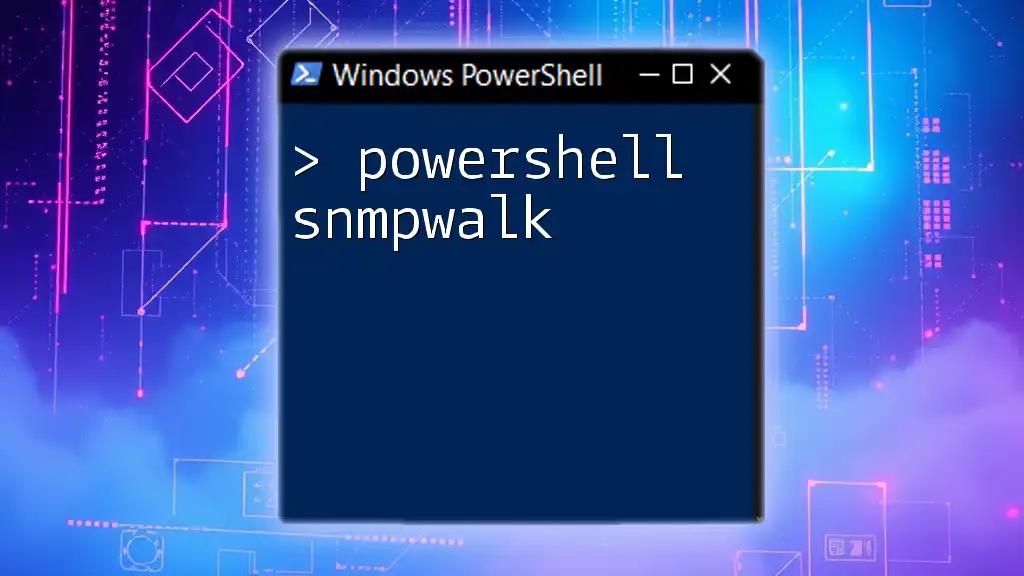
Real-World Applications of PowerShell Enums
Using Enums in Scripts
Enums find practical applications in PowerShell scripts, particularly for defining states or modes. For instance, consider a script that defines the status of various processes. By using enums, you can make the script easier to manage and understand:
enum ProcessStatus {
NotStarted
InProgress
Completed
Failed
}
With this enum, you can manage the states of your processes in a clear manner, enhancing both functionality and readability.
Enums in Workflow Automation
In automation scripts, enums can play a vital role in streamlining processes. They allow you to set and check statuses, executing certain blocks of code based on the current enum state. For instance, you could use this enum to check whether a process has completed successfully before proceeding with further steps.
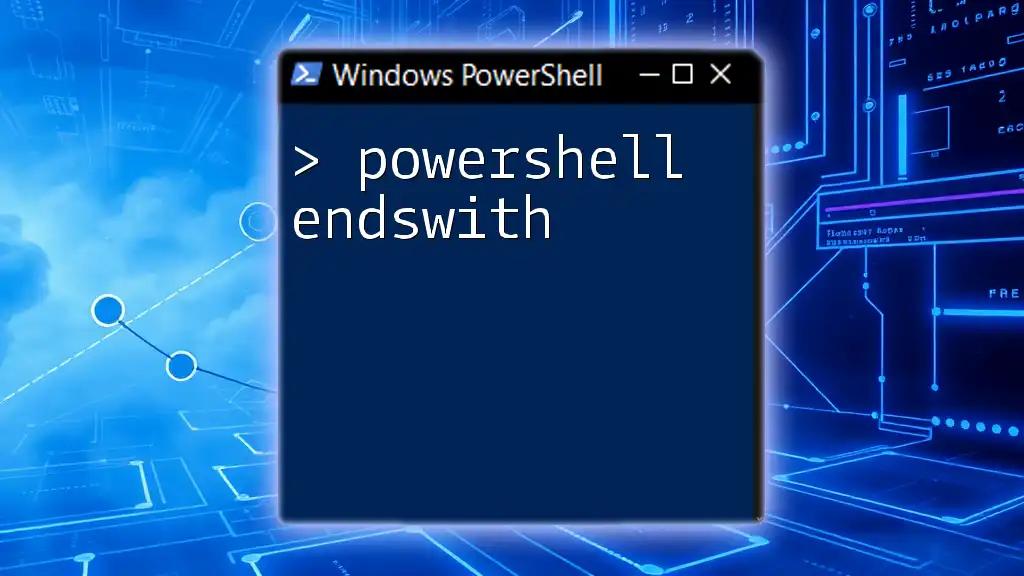
Conclusion: The Power of PowerShell Enums
Recap of Key Points
To summarize, PowerShell enums provide a powerful and type-safe way to work with a set of related constant values. They enhance readability and maintainability in your scripts while facilitating easy comparisons and logic flow control. By creating your own enums, you can tailor them to meet the specific needs of your PowerShell projects.
Additional Resources
For further learning, consider exploring the official PowerShell documentation, which contains extensive information on enums and other powerful features.
Call to Action
Now, it's time for you to experiment. Start creating your own enums in PowerShell scripts. You'll find that using enums not only makes your scripting more efficient but also improves the overall clarity of your code. Happy scripting!