In PowerShell, an encoded command allows you to run commands encoded in Base64 format, making it easier to transmit complex commands without being altered by character encoding issues.
Here’s a code snippet demonstrating how to utilize an encoded command:
$command = 'Write-Host "Hello, World!"'
$bytes = [System.Text.Encoding]::Unicode.GetBytes($command)
$encodedCommand = [Convert]::ToBase64String($bytes)
powershell -EncodedCommand $encodedCommand
Understanding PowerShell Encoded Commands
What are PowerShell Encoded Commands?
PowerShell encoded commands are a mechanism used to obfuscate and execute PowerShell scripts and cmdlets. Encoded commands allow users to convert PowerShell commands into a Base64 string representation, making it more challenging for malicious actors to intercept or modify the command.
This approach is particularly useful when sharing scripts over email or within environments that may not handle raw PowerShell commands properly. Encoded commands can be particularly handy for system administrators, developers, and security professionals who want a secure and streamlined method of running PowerShell scripts.
Why Use Encoded Commands?
There are several compelling reasons to utilize PowerShell encoded commands:
- Security Benefits: Encoding commands obfuscates the script’s content, helping to conceal sensitive logic or data from being easily inspected.
- Ease of Sharing: Encoded commands can be transmitted via channels that might corrupt or interfere with normal text formats, reducing the chance of syntax errors.
- Compatibility: Encoding ensures that commands run uniformly across different PowerShell environments, enhancing reliability during execution.
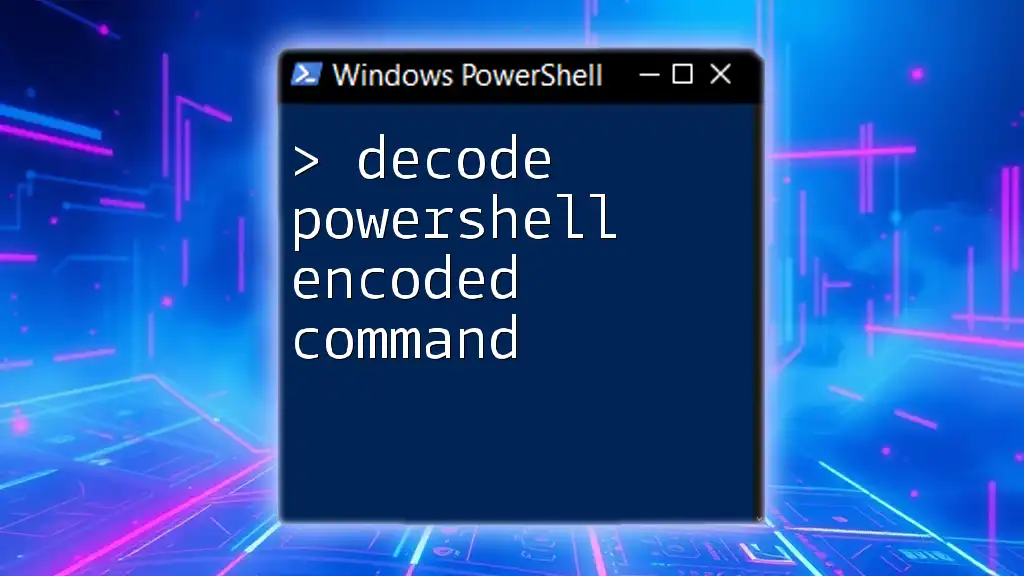
How to Create Encoded Commands
Encoding a PowerShell Command
Creating an encoded command is straightforward and can be accomplished using the `ConvertTo-Base64` method in PowerShell. Here’s how you can encode a simple command:
- Basic Syntax: Begin by defining the PowerShell command you wish to encode.
- Encode It: Use the following code snippet to achieve this:
$command = 'Get-Process'
$bytes = [System.Text.Encoding]::Unicode.GetBytes($command)
$encodedCommand = [Convert]::ToBase64String($bytes)
Write-Output $encodedCommand
In this example, `Get-Process` is transformed into its Base64 equivalent. The output will be a long string that represents the original command, making it safe for transmission.
Practical Example of Command Encoding
Let’s say you want to encode a command that lists all running processes along with their details:
$command = 'Get-Process | Format-Table -AutoSize'
$bytes = [System.Text.Encoding]::Unicode.GetBytes($command)
$encodedCommand = [Convert]::ToBase64String($bytes)
Write-Output $encodedCommand
Running this will produce a Base64 string, which can be used for execution later.
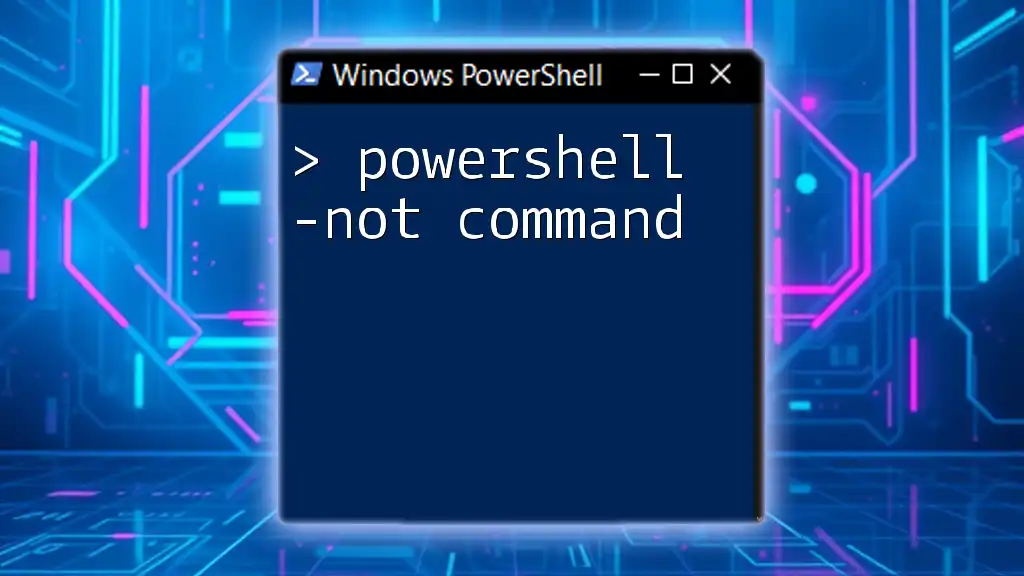
Executing Encoded Commands
How to Execute Encoded Commands
Once you have your encoded command, executing it is quite simple. PowerShell provides a built-in command for this purpose. You can use the `-EncodedCommand` parameter followed by your Base64 string like this:
powershell.exe -EncodedCommand 'YourBase64EncodedString'
Replace `'YourBase64EncodedString'` with the output from the encoding process.
Practical Example of Execution
Assuming you have encoded `Get-Process | Format-Table -AutoSize`, execute it as follows:
- First, encode your command and retrieve the Base64 string.
- Then, execute it using:
powershell.exe -EncodedCommand '[Your_Encoded_String]'
This process illustrates how to take an encoded command from creation through to execution seamlessly.
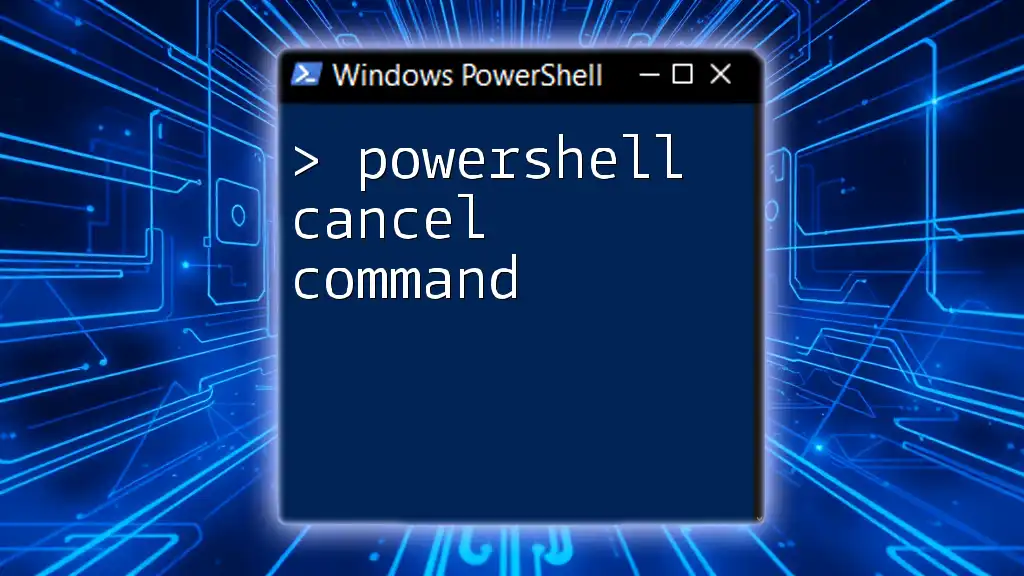
Advanced Encoding Techniques
Using Scripts with Encoded Commands
You can also encode entire PowerShell scripts, which is especially useful for complex automation tasks. First, save your script in a `.ps1` file, then read the file's contents into a variable before encoding:
$scriptContent = Get-Content -Path 'C:\Path\To\YourScript.ps1' -Raw
$bytes = [System.Text.Encoding]::Unicode.GetBytes($scriptContent)
$encodedScript = [Convert]::ToBase64String($bytes)
Write-Output $encodedScript
Understanding Execution Policies
PowerShell's execution policies control the conditions under which scripts are allowed to run. This is significant when dealing with encoded commands. For instance, if your execution policy does not permit script execution, your encoded commands might fail.
To modify the execution policy temporarily or for the session, use:
Set-ExecutionPolicy -Scope Process -ExecutionPolicy Bypass
This command allows you to run scripts without altering the system-wide policy, which can help avoid complications when executing encoded commands.
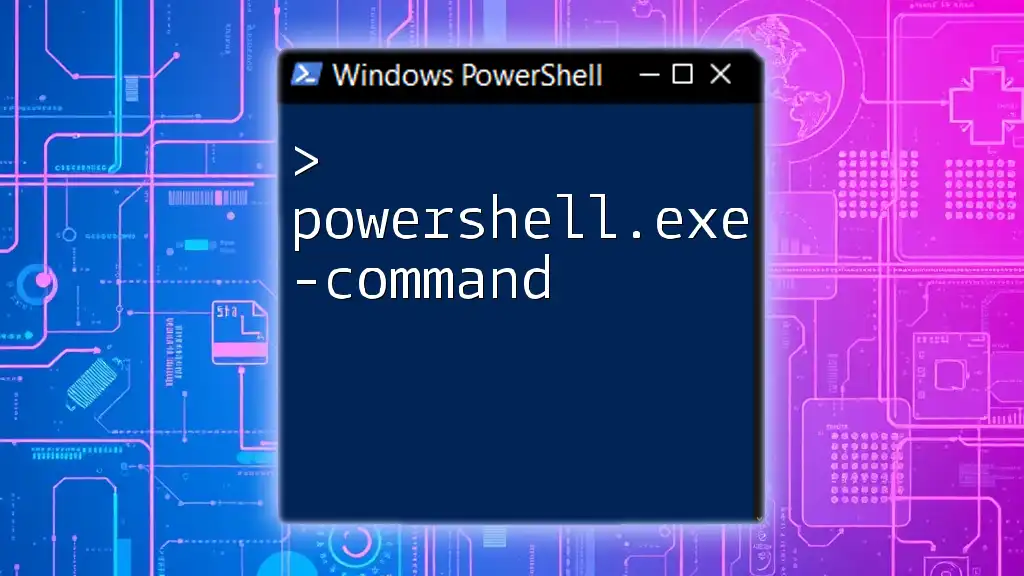
Troubleshooting Common Issues
Problems When Executing Encoded Commands
Occasionally, you may encounter errors when executing encoded commands. Some common issues include:
- Invalid Base64 strings: Ensure that the complete encoded string was copied correctly.
- Execution policies: Confirm that your execution policy permits script execution.
- Syntax errors: Although encoded, if your command had syntax errors before encoding, those will persist.
Best Practices for Using Encoded Commands
- Avoid storing sensitive data in scripts: Even with encoding, it’s wise not to rely solely on this method for securing sensitive information.
- Limit the use of encoded commands: Use them only when necessary, especially in development environments.
- Comment and document your scripts: Encoded commands can be harder to debug, so having thorough documentation is advantageous.
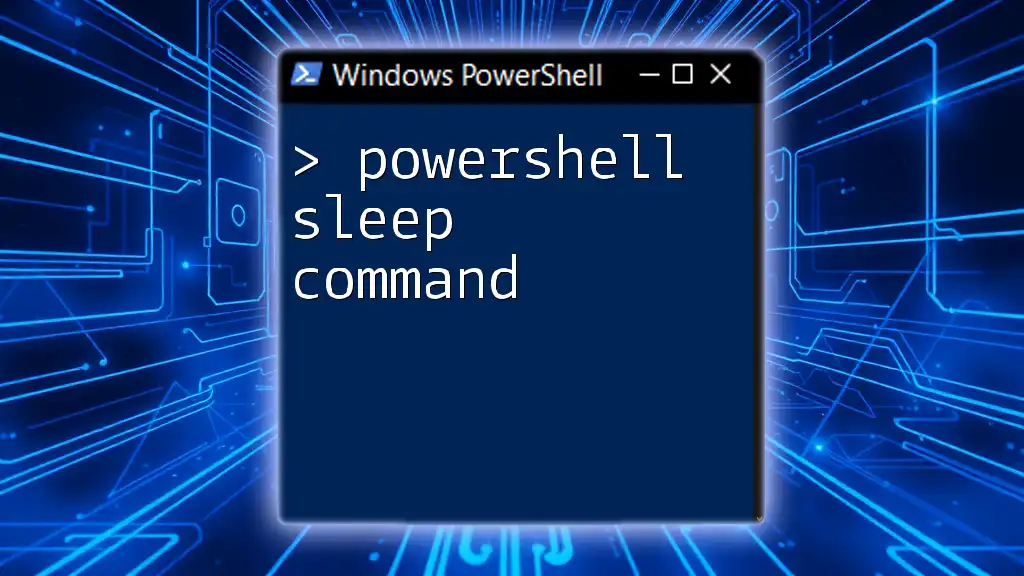
Conclusion
Summary of Key Points
In summary, PowerShell encoded commands are a powerful tool for enhancing script security and compatibility. Understanding how to create, execute, and troubleshoot these commands equips users with a robust method for automating tasks while protecting sensitive information.
Call to Action
Now that you're familiar with the process of encoding PowerShell commands, why not give it a try? Start experimenting with your own commands to understand the benefits of this powerful feature. For further exploration, consider diving into additional PowerShell resources to expand your knowledge and capabilities.
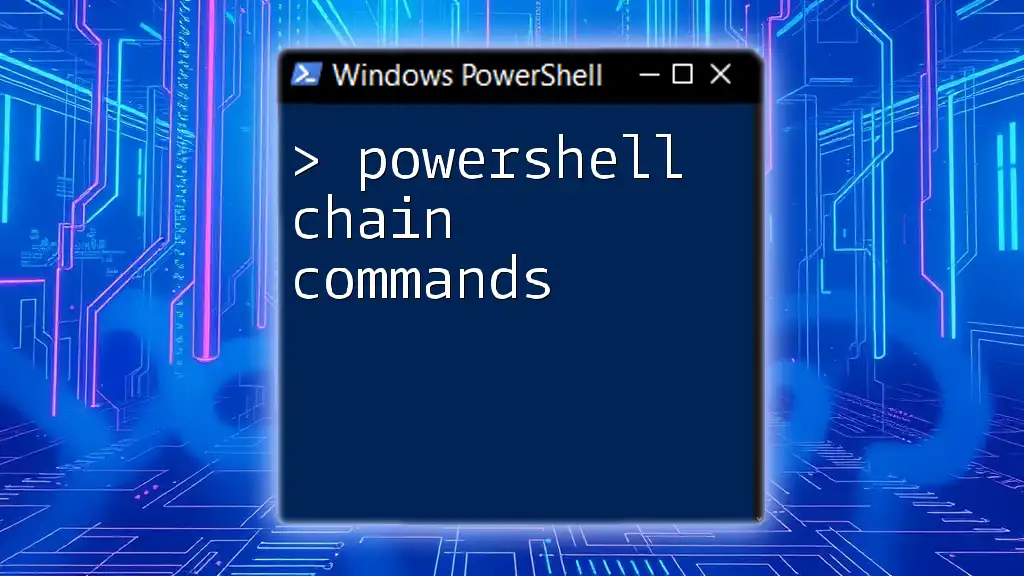
Additional Resources
Recommended Reading and Tools
- Official PowerShell Documentation: A comprehensive resource for everything PowerShell-related.
- Base64 Encoding Tools: Utilities to help with manual encoding and decoding.
- Online PowerShell Communities and Forums: These platforms offer peer support and extended learning opportunities.