The `-not` operator in PowerShell is used to negate a Boolean expression, returning true if the expression is false and vice versa.
Here's a code snippet demonstrating its use:
$condition = $false
if (-not $condition) {
Write-Host 'The condition is false!'
}
What is the -Not Operator?
Definition and Purpose
The `-Not` operator is a logical negation construct used in PowerShell to reverse the condition or boolean expression that follows it. Essentially, it evaluates to `true` if the conditional expression is `false`, and vice versa. This is particularly useful when you want to exclude certain items from your results or perform actions only when specific conditions are not met.
Example: A basic use of the `-Not` operator in conditional statements can be illustrated as follows:
if (-not (Test-Path "C:\example.txt")) {
Write-Host "File does not exist!"
}
In this example, the script checks if a file does not exist and then informs the user accordingly.
Syntax Overview
The syntax for the `-Not` operator is straightforward. You simply place it before the condition you want to reverse:
condition -not condition
It is important to note how `-Not` compares to similar logical operators, such as `!`, `-eq`, and `-ne`. While they share similar functionalities—serving to create negations—their usage can influence the readability of your scripts.
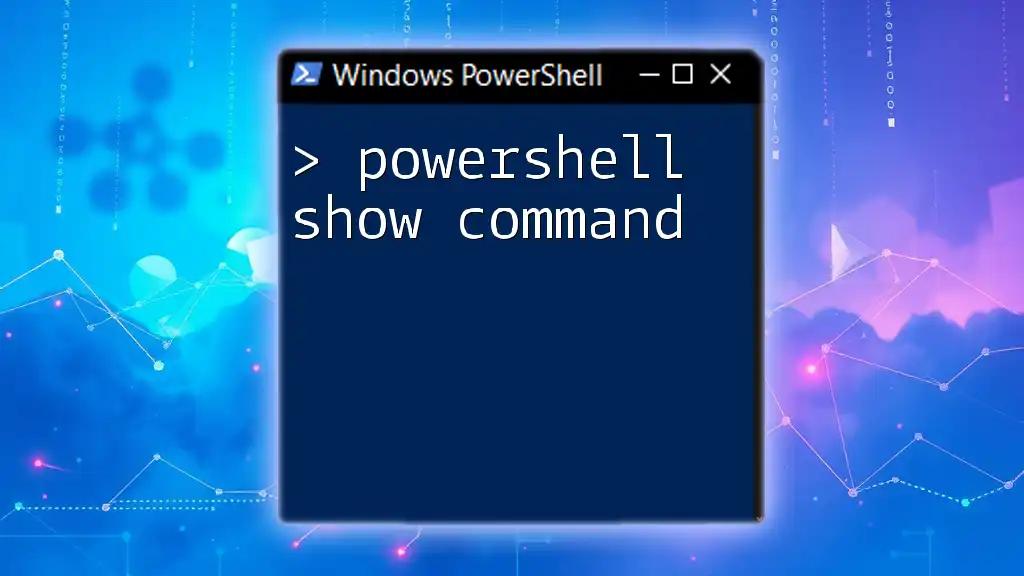
Practical Applications of -Not in PowerShell
Filtering Objects
One of the most effective applications of the `-Not` operator is in filtering objects within command pipelines. You can easily exclude items that meet certain conditions, making your scripts more efficient and focused.
Example: Here’s how you can filter out unwanted instances from the list of running processes:
Get-Process | Where-Object { $_.ProcessName -notlike "System*" }
In this command, the `-Not` operator helps filter out any system processes, allowing you to focus on user-defined processes.
Conditional Logic
The `-Not` operator can also be instrumental when employed in conditional logic. In scenarios where you want to execute a piece of code only if a specific condition is not met, `-Not` becomes quite handy.
Example:
if (-not (Test-Path "C:\example.txt")) {
Write-Host "File does not exist!"
}
In this instance, the command checks if a specific file does not exist and takes action accordingly, providing feedback to the user.
In Loop Constructs
Using `-Not` within loop constructs allows you to skip over certain actions based on defined criteria. This is particularly effective in scenarios involving arrays or collections, where you want to bypass specific entries.
Example:
foreach ($item in $items) {
if (-not ($item -eq "SkipThisItem")) {
# Process the item
Write-Host "Processing $item"
}
}
Here, the loop will process each item unless it matches the specified condition, ensuring that unwanted items are easily excluded.
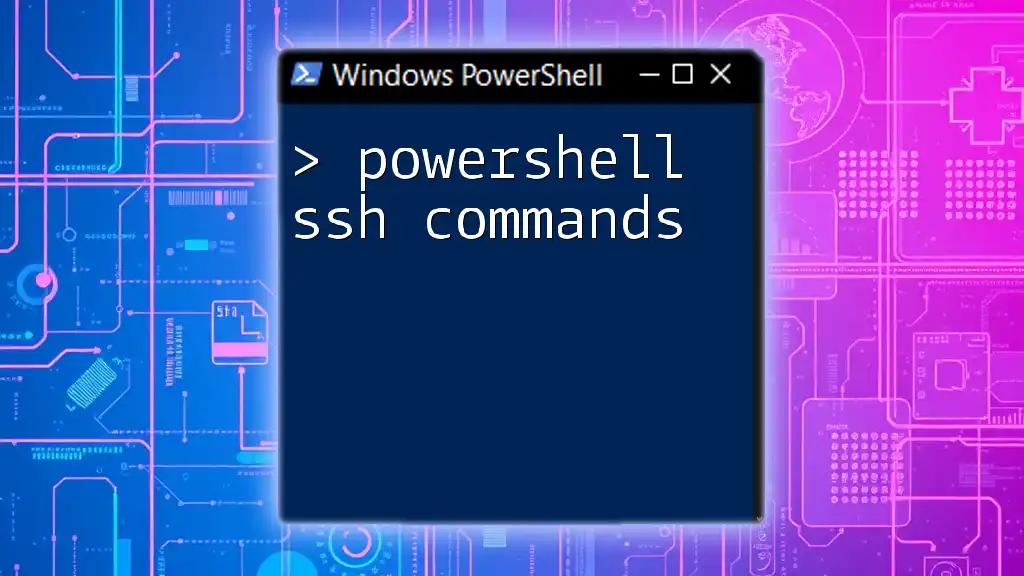
Best Practices with -Not
Readability and Maintainability
While the `-Not` operator is powerful, clarity is paramount. It's crucial to write scripts in a way that others (or you in the future) can easily read and understand. Make good use of whitespace and meaningful variable names to enhance the readability of your scripts.
Performance Considerations
Performance is also an essential consideration, particularly when using the `-Not` operator within filtering operations involving large datasets. In some cases, other approaches like direct querying or more optimized filtering methods could yield better performance results.
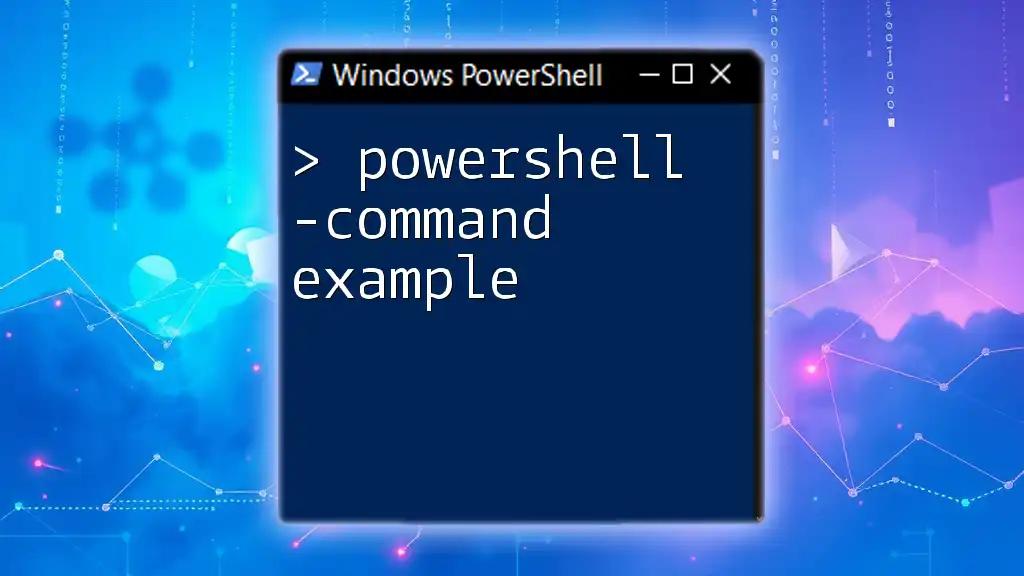
Common Mistakes to Avoid
Misunderstanding the Logic
A common pitfall when using the `-Not` operator is misunderstanding the logic it embodies. For instance, the condition `-not ($value -eq 10)` should evaluate correctly, but if misused, you could end up with unexpected results.
Example:
if (-not ($value -eq 10)) {
Write-Host "Value is not 10"
}
Here, if the `$value` evaluates to `10`, then the script will not execute the code block underneath, which can sometimes lead to confusion if not well-documented.
Overusing the Operator
It’s important to recognize that overusing the `-Not` operator may complicate your script and make it less intuitive. In some instances, opting for clearer alternatives, such as using positive conditions where applicable, can enhance code comprehension.
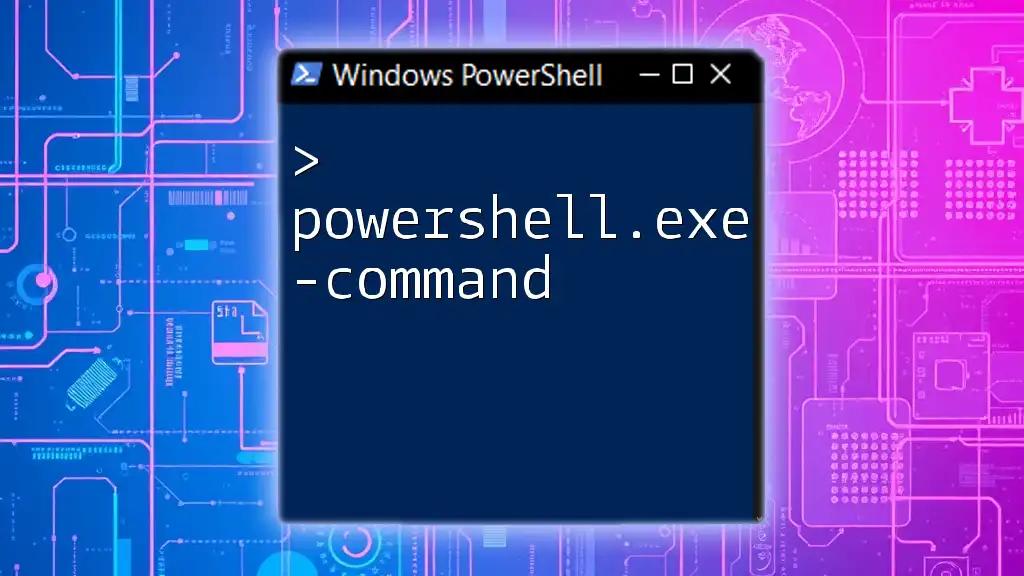
Advanced Usage of -Not
Combining with Other Logical Operators
The `-Not` operator can also be effectively combined with other logical operators, such as `-And` and `-Or`, to create complex conditions in your scripts that require multiple checks.
Example:
if (-not ($value -eq 10) -and -not ($value -eq 20)) {
Write-Host "Value is neither 10 nor 20"
}
In this example, the script checks whether a value is not equal to both 10 and 20 simultaneously, allowing for greater flexibility in logic implementations.
Using -Not with Cmdlets
The versatility of the `-Not` operator extends to many PowerShell cmdlets, enhancing their functionality. For instance, when using the `Get-Service` cmdlet, you can filter out services that aren't running:
Example:
Get-Service | Where-Object { -not ($_.Status -eq 'Running') }
This command effectively lists all services that are not currently running, making system administration tasks simpler and more efficient.
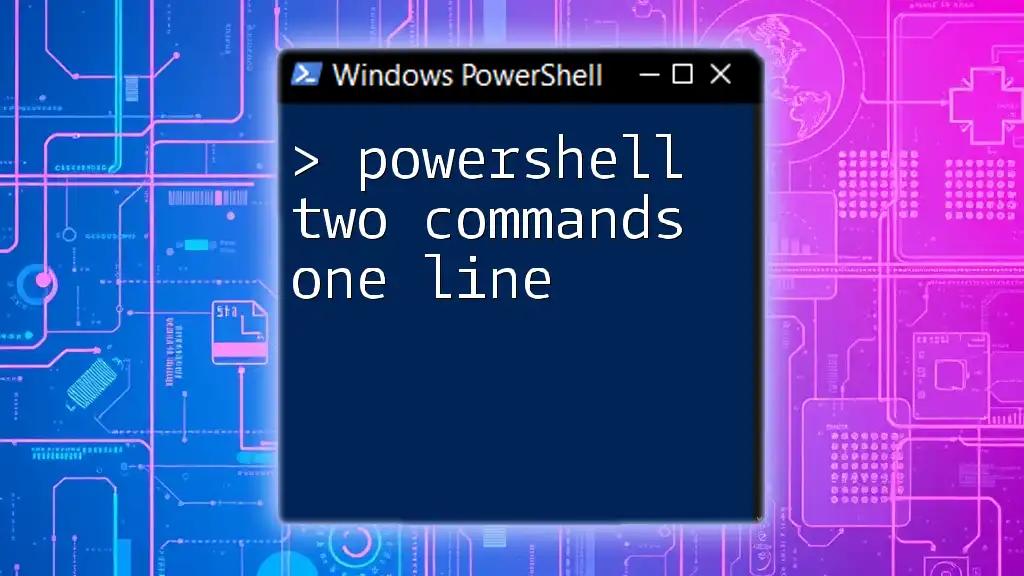
Exploring Alternatives to -Not
Logical Negation with Other Symbols
While `-Not` is a powerful operator, recognizing its alternatives can increase your scripting flexibility. The `!` symbol serves as an alternative and is often used in comparisons to achieve the same end. However, usage conventions can differ between contexts, and it's vital to select the most appropriate operator for clarity in your code.
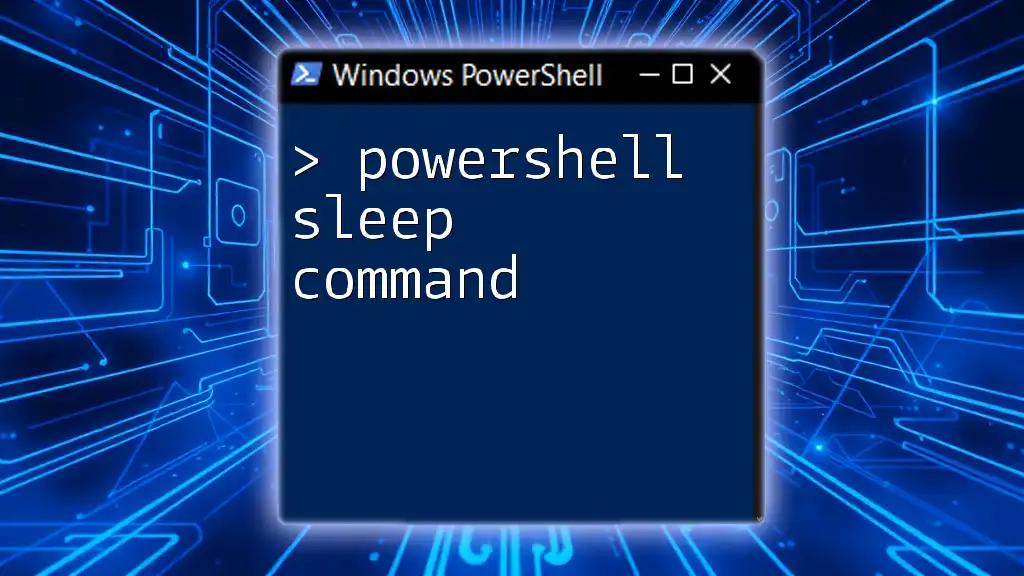
Conclusion
In conclusion, the PowerShell -Not command is an essential tool for any IT professional looking to streamline their scripting and filtering processes. Whether you're filtering out unwanted objects, implementing conditional logic, or maneuvering through complex loop constructs, the `-Not` operator can enhance your commands significantly. By understanding its applications, best practices, and potential pitfalls, you can leverage this operator more effectively in your PowerShell scripts.
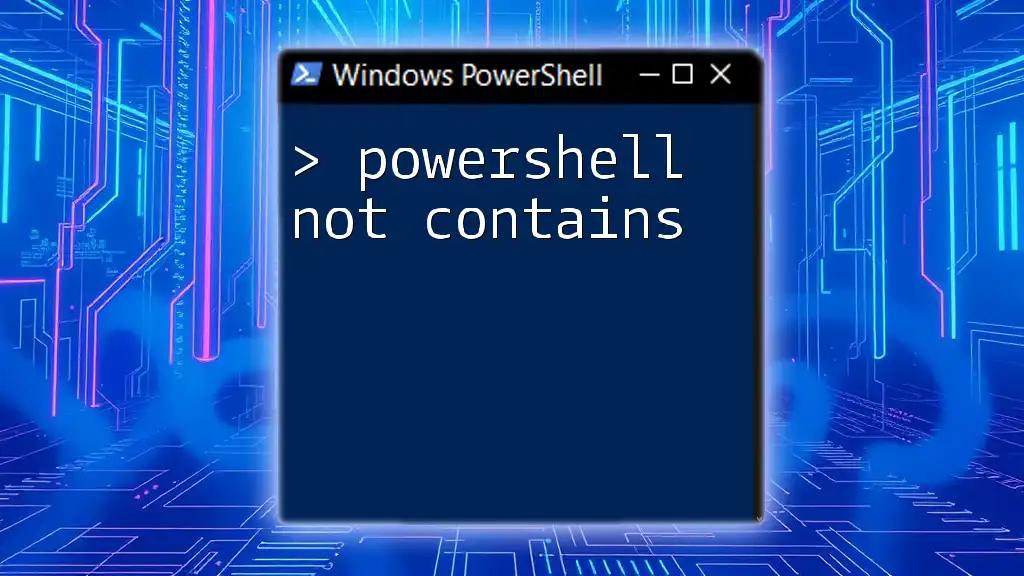
Additional Resources
For further exploration, consider delving into the official PowerShell documentation and engaging with online communities that focus on scripting and automation. These resources can provide deeper insights and opportunities to enhance your PowerShell skills.
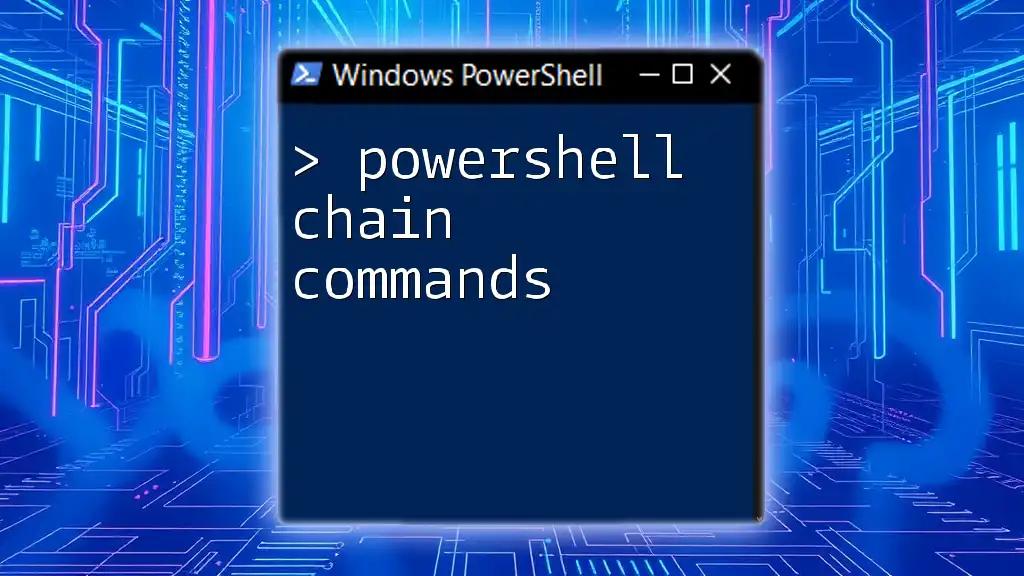
Call to Action
We encourage you to share your experiences with the `-Not` operator and how it has improved your PowerShell scripting. Don't forget to subscribe for more tips and training resources on maximizing your PowerShell efficacy!