A PowerShell menu is a user-friendly interface allowing users to select from a list of commands or options for streamlined command execution.
Here's a simple example of a PowerShell menu:
$menuOptions = @(
'1: Display Date and Time'
'2: List Files in Current Directory'
'3: Exit'
)
foreach ($option in $menuOptions) {
Write-Host $option
}
$choice = Read-Host 'Please select an option (1-3)'
switch ($choice) {
'1' { Get-Date }
'2' { Get-ChildItem }
'3' { Write-Host 'Exiting...'; exit }
default { Write-Host 'Invalid choice. Please try again.' }
}
What is a PowerShell Menu?
A PowerShell menu is a user interface element that allows users to interact with a script or command by selecting options rather than typing them out directly. This feature is particularly useful for those who may be less familiar with PowerShell commands, providing a straightforward and user-friendly experience.
Common use cases for PowerShell menus include automating IT tasks, enhancing script usability for non-technical users, and creating guided interfaces for educational purposes.
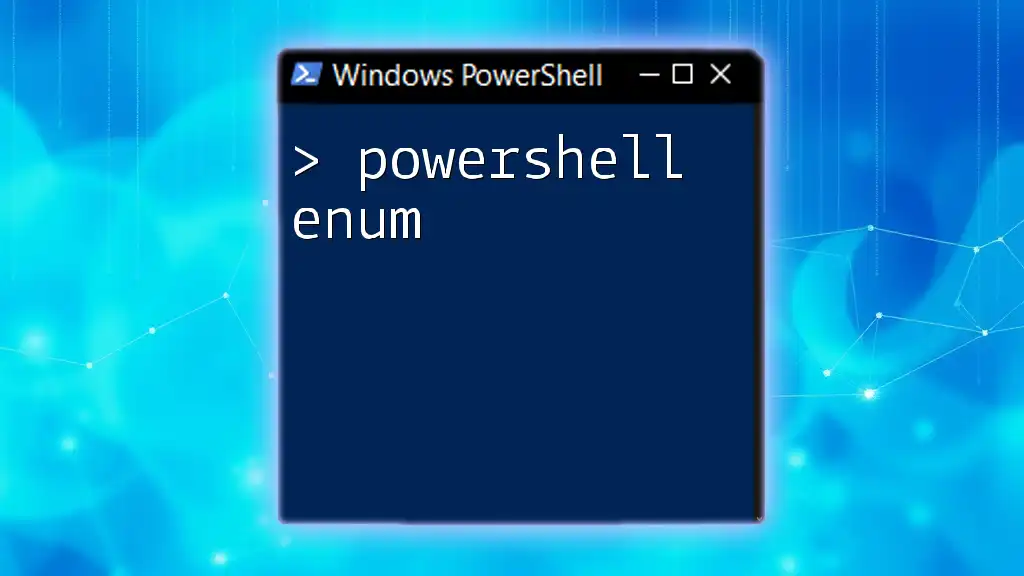
Benefits of Using Menus in PowerShell
Utilizing menus in PowerShell offers several advantages:
- Enhanced user experience: Menus simplify interaction by presenting clear choices, which can be particularly useful for complex scripts.
- User-friendly interface: By selecting options instead of typing commands, users can navigate scripts without needing extensive PowerShell knowledge.
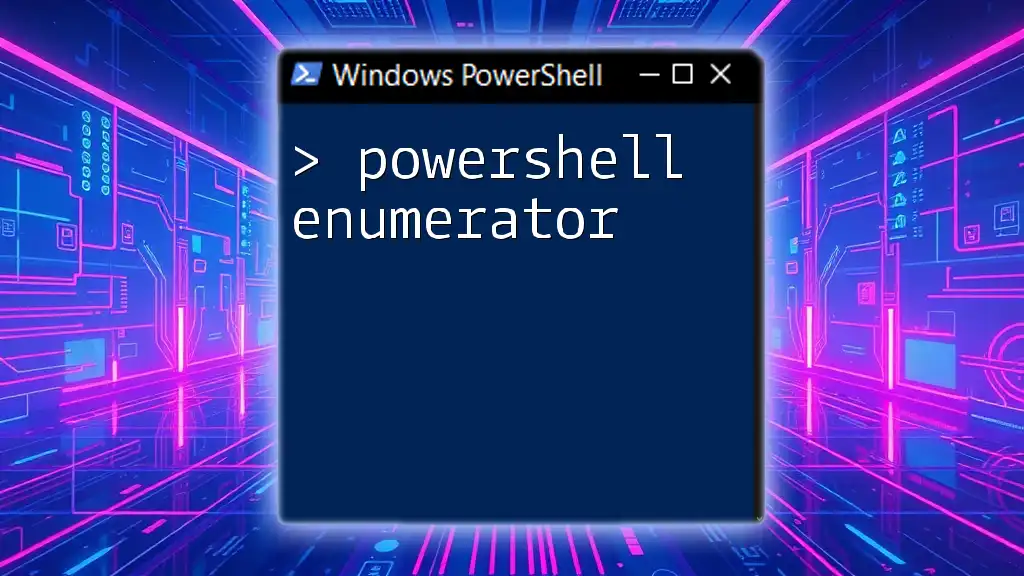
Creating a PowerShell Menu
Basic Structure of a PowerShell Menu
To begin working with PowerShell menus, it's essential to understand their basic structure. Menus function within the command-line interface (CLI), and constructing a menu involves defining options followed by handling user input.
Setting Up Your First PowerShell Menu
Start by creating a simple menu function. Here is a basic outline of how this can be accomplished:
Function Show-Menu {
param (
[string]$Title = "Menu"
)
Clear-Host
Write-Host "==== $Title ====" -ForegroundColor Green
Write-Host "1: Option One"
Write-Host "2: Option Two"
Write-Host "3: Exit"
}
Show-Menu
In this example, the function Show-Menu will clear the screen and display the menu title. The Write-Host command is used to present each option.
Example: Creating a Simple Menu
To further illustrate how a PowerShell menu works, let’s look at a step-by-step code snippet. This code creates a simple menu and invokes it:
Function Show-Menu {
param (
[string]$Title = "Menu"
)
Clear-Host
Write-Host "==== $Title ====" -ForegroundColor Green
Write-Host "1: Option One"
Write-Host "2: Option Two"
Write-Host "3: Exit"
}
Show-Menu
Explanation of the Code
- The `Clear-Host` command clears the terminal screen for a clean interface.
- The menu displays options, numbered for easy selection.
Adding Functionality to the Menu
To make the menu interactive, you must integrate user input and execute commands based on their choices.
First, prompt for user input:
$choice = Read-Host "Please choose an option"
Next, implement logic to respond to the user's choice with a Switch statement:
Switch ($choice) {
1 { Write-Host "You selected Option One" }
2 { Write-Host "You selected Option Two" }
3 { exit }
Default { Write-Host "Invalid choice, please try again!" }
}
Code Explanation
- The `Switch` statement evaluates the user's input, allowing you to provide feedback or execute specific commands for each option.
- The Default clause helps in managing unexpected input, enhancing the user experience by prompting them to try again.
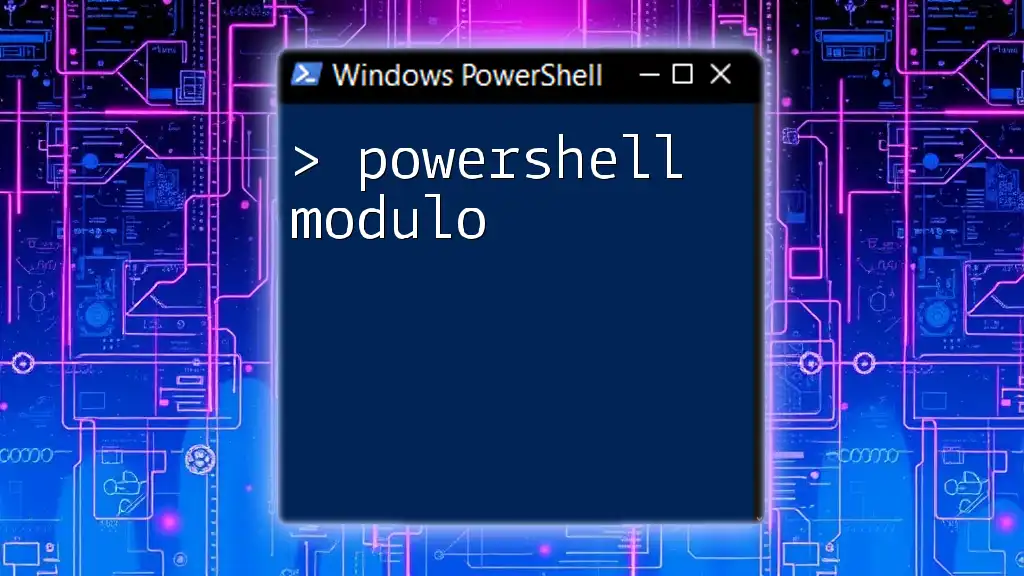
Enhancing Your PowerShell Menu
Adding Submenus
To create a more organized user experience, consider adding submenus. This allows you to group related options for easier navigation and usability.
Creating Hierarchical Menus
Here’s an example of implementing submenus:
Function Show-SubMenu {
Write-Host "1: Submenu One"
Write-Host "2: Submenu Two"
$subchoice = Read-Host "Choose a submenu option"
# Add functionality as needed
}
Improving User Experience
You can enhance your menu's appeal by adding color coding to your options. By using `Write-Host`, you can assign colors to different options, drawing users’ attention accordingly:
Write-Host "1: Option" -ForegroundColor Blue
Adding Looping Functionality
To keep users engaged until they decide to exit, employ a looping mechanism:
Do {
Show-Menu
# Handle user input and switch statements
} While ($choice -ne 3)
This loop continues to display the menu and handle choices until the user selects the exit option.
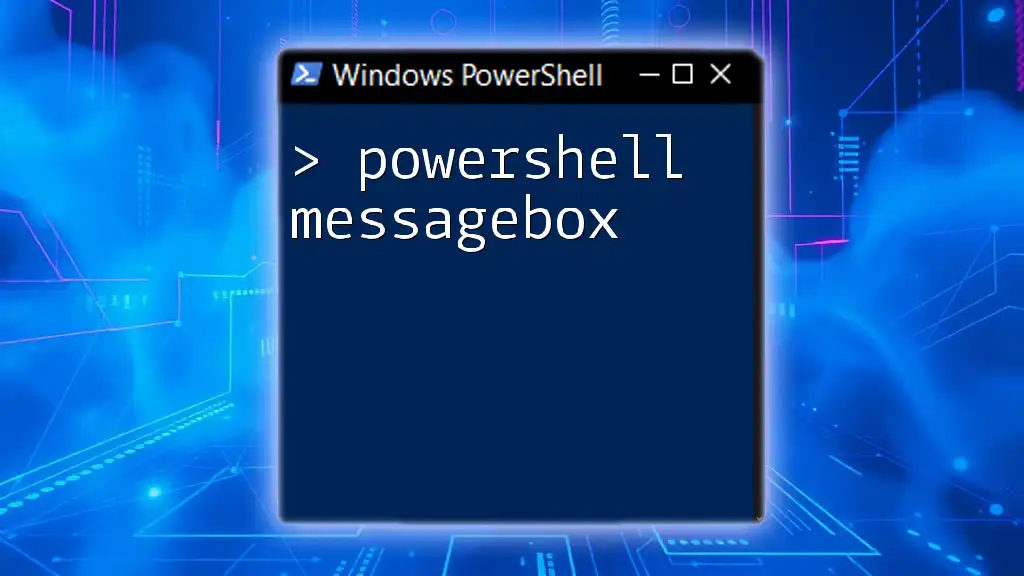
Best Practices for Designing PowerShell Menus
Clarity and Simplicity
When creating a PowerShell menu, keeping it straightforward is vital. Avoid overloading users with too many options at once. A clear structure helps users navigate more comfortably.
Error Handling
Incorporate effective feedback mechanisms for invalid selections. Always provide a way to guide users back to the valid choices, minimizing frustration and improving user experience.
Commenting Your Code
Make sure to comment your code adequately. Well-placed comments not only enhance the maintainability of your script but also help others (or future you) understand the logic more easily.
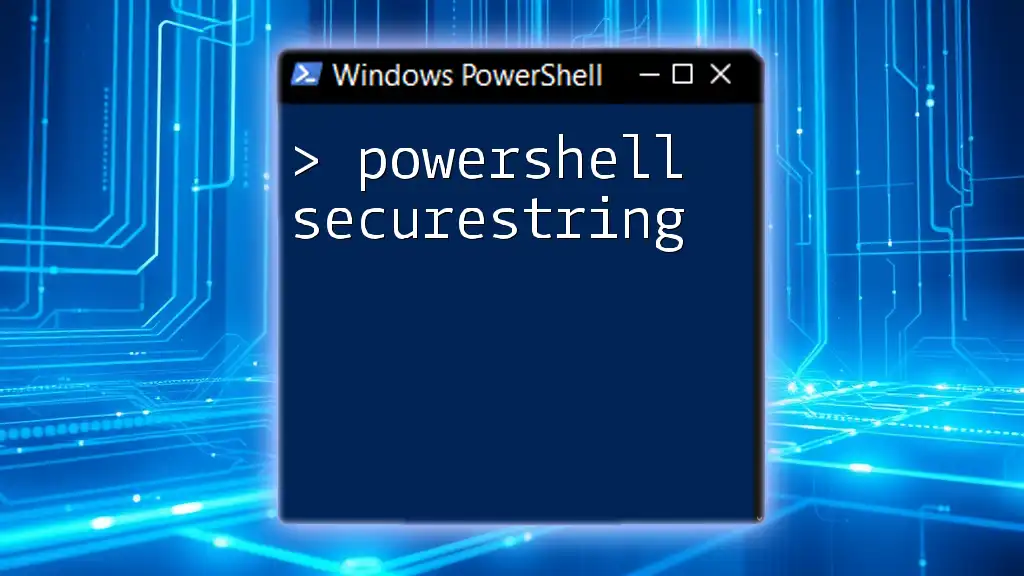
Real-World Applications of PowerShell Menus
Automating IT Tasks
PowerShell menus shine in environments where IT automation is needed. Scripts with menus can facilitate tasks such as user management, system configurations, or executing reports, ensuring that less technical staff can perform these actions with confidence.
Educational Use Cases
When teaching or conducting tutorials, scripts built around menus can provide a guided and interactive experience for learners, allowing them to focus on learning commands in a structured manner.
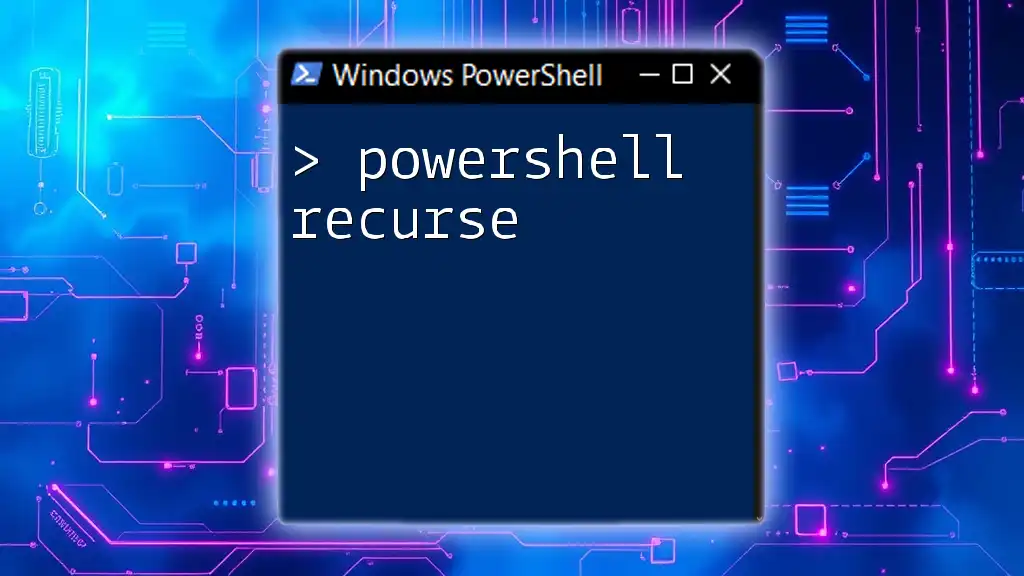
Conclusion
In conclusion, a well-designed PowerShell menu can significantly enhance user interaction and script usability. By implementing clear options, responsive input handling, and thoughtful error management, you can create an efficient and enjoyable experience for users of all skill levels. As you experiment with menus in your own scripts, don't hesitate to innovate and customize them to best suit your needs!