To securely encrypt a password using PowerShell, you can utilize the `ConvertTo-SecureString` cmdlet along with the `-AsPlainText` and `-Force` parameters, as shown in the following code snippet.
$encryptedPassword = ConvertTo-SecureString "YourPlainTextPassword" -AsPlainText -Force
Understanding Password Encryption
What is Password Encryption?
Password encryption refers to the process of converting plain text passwords into a coded format that cannot be easily read or understood by unauthorized individuals. Unlike hashing, which is a one-way function, encryption is designed to be reversible, allowing authorized users to decrypt the information when needed.
Why Encrypt Passwords in PowerShell?
Encrypting passwords in PowerShell is essential for protecting sensitive information that may be used in scripts or automation tasks. Without encryption, plain text passwords can be exposed, leading to serious security vulnerabilities. Use cases for encrypted passwords in PowerShell include:
- Automating repetitive tasks without requiring user intervention.
- Protecting user credentials when accessing APIs or databases.
- Storing sensitive information securely within scripts.
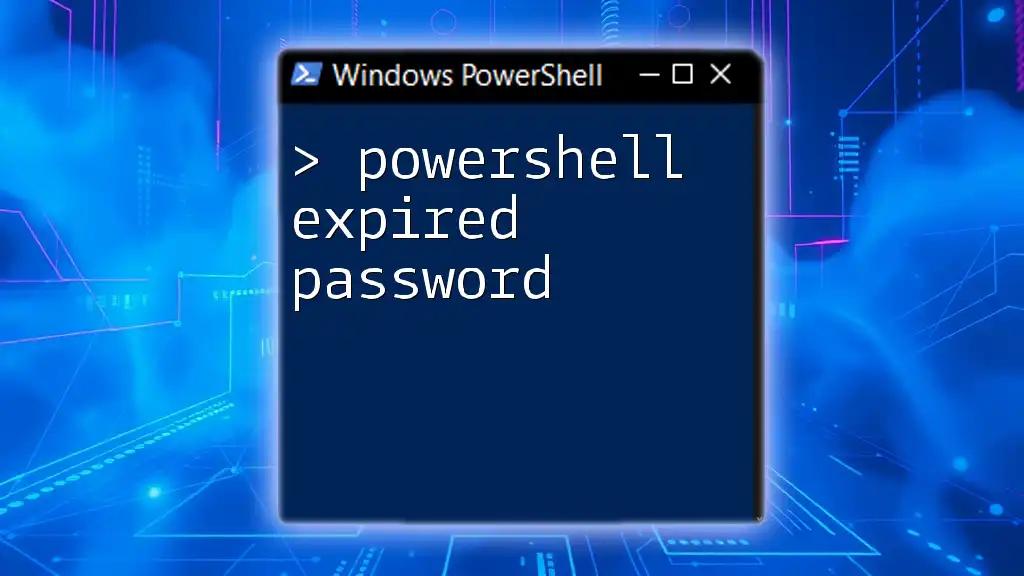
Powershell Encrypt Password: Getting Started
Prerequisites
Before diving into password encryption, it's crucial to have a basic understanding of PowerShell. Familiarity with cmdlets, scripting conventions, and the PowerShell environment will help you grasp the concepts more effectively. Ensure that your PowerShell installation is up to date to access the latest features.
Setting Up Your Environment
For optimal security, run PowerShell as an administrator. This ensures that you have the necessary permissions to execute commands that involve sensitive data. Check your execution policy by running:
Get-ExecutionPolicy
If it's not set to `RemoteSigned` or `Unrestricted`, consider changing it temporarily for your session with:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope Process
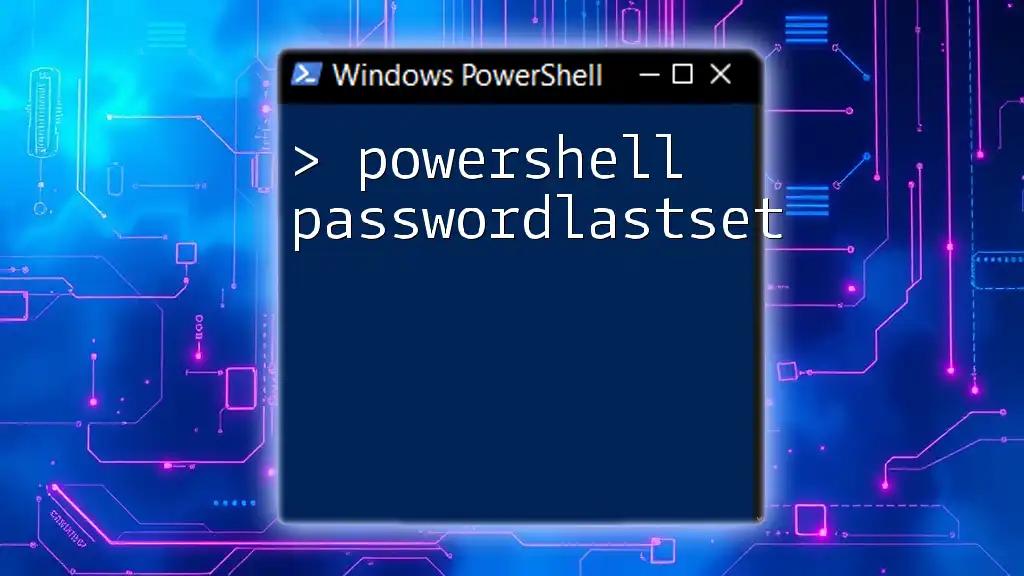
Methods to Encrypt Passwords in PowerShell
Using ConvertTo-SecureString
The `ConvertTo-SecureString` cmdlet is a powerful tool that allows you to convert plain text passwords into a secure format. Here’s how you can use it:
$securePassword = ConvertTo-SecureString "YourPlainTextPassword" -AsPlainText -Force
- `-AsPlainText`: This parameter indicates that the password you are providing is in plain text.
- `-Force`: This force prompts PowerShell to accept the plain text input without further confirmation.
This command will create a secure string representation of the provided password. At this stage, it's stored in memory in an encrypted format.
Storing Encrypted Passwords
You may choose to store the encrypted password for later use. To save the encrypted password to a file, use the following command:
$securePassword | ConvertFrom-SecureString | Set-Content "encryptedPassword.txt"
This command encrypts the secure string again into a format that can be easily saved to a file, enabling you to retrieve it when necessary.
Retrieving Encrypted Passwords
Accessing the encrypted password is straightforward. Use the following code to read and convert the stored password back into a secure format:
$encryptedPassword = Get-Content "encryptedPassword.txt"
$securePassword = $encryptedPassword | ConvertTo-SecureString
This retrieves the encrypted password from the text file and reverts it back to a secure string.
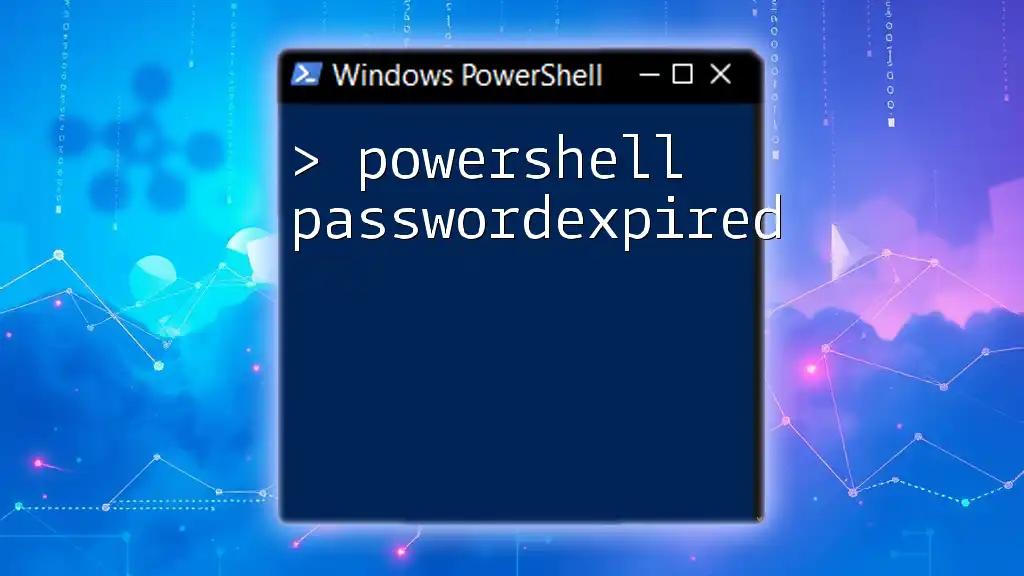
Powershell Encrypt Credentials
Creating a Credential Object
To manage credentials more effectively, you can create a `PSCredential` object, which encapsulates the username and the secure password. Here’s an example:
$username = "YourUsername"
$credential = New-Object System.Management.Automation.PSCredential($username, $securePassword)
This encapsulation enables you to use the credentials in various contexts, such as running scripts that require authentication.
Using the Credential Object
The `PSCredential` object can be employed for secure operations in PowerShell. For instance, if you want to start a process with specific credentials, you could use:
Start-Process "YourApplication.exe" -Credential $credential
This command ensures that the application runs under the context of the provided user, enhancing security by limiting the exposure of plain text credentials.
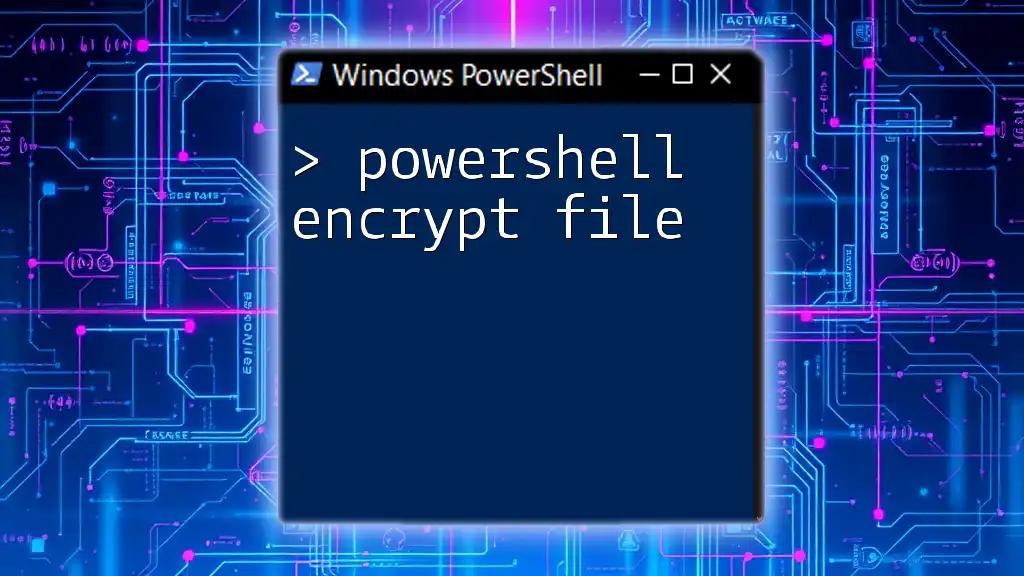
Best Practices for Password Encryption in PowerShell
Avoid Hardcoding Passwords
One of the critical mistakes in script writing is hardcoding passwords directly within the code. This exposes sensitive information and increases the risk of breaches. Instead, use encrypted storage solutions and retrieve passwords dynamically.
Regularly Update Encryption Keys
It's advisable to regularly update the encryption method and keys you use. Over time, encryption methods can become less secure due to advancements in computing power. Rotating keys helps ensure that even if passwords are compromised, they remain protected through modern encryption standards.
Use of Secure Password Storage Options
For further improving security, consider using specialized secure vault solutions. Options such as Azure Key Vault or Windows Credential Manager provide additional layers of security for storing sensitive information, integrating seamlessly with PowerShell for automated access.
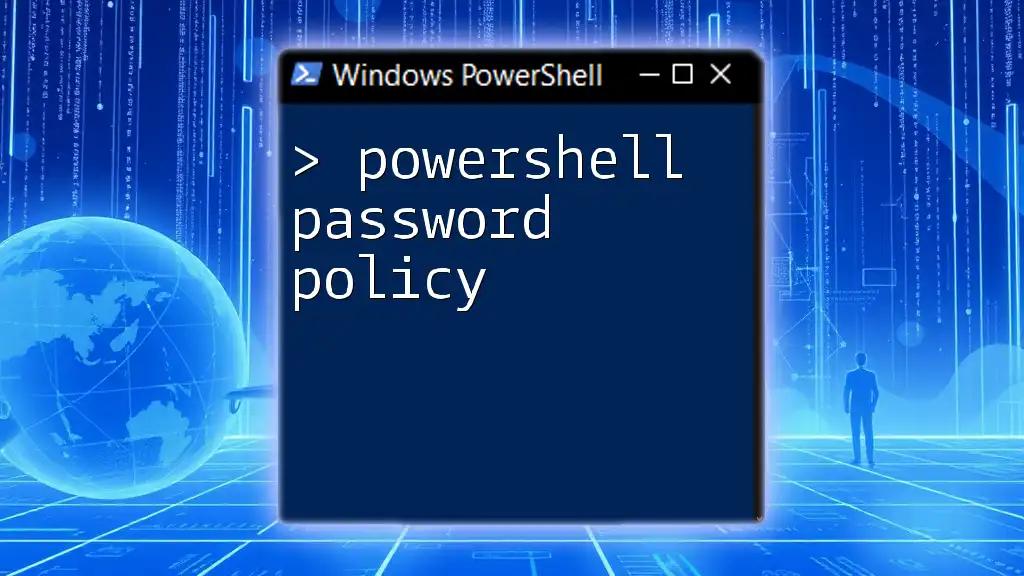
Debugging Common Issues
Troubleshooting SecureString Errors
Though PowerShell is intuitive, users may encounter errors while working with secure strings. Common errors often involve incompatible formats when attempting to convert between secure strings and plain text. Always verify the secure string before attempting to use it in commands.
Permissions Issues
If you face permission-related issues while executing commands that involve encryption or credential management, check the user account control settings and ensure adequate permissions for your scripts.
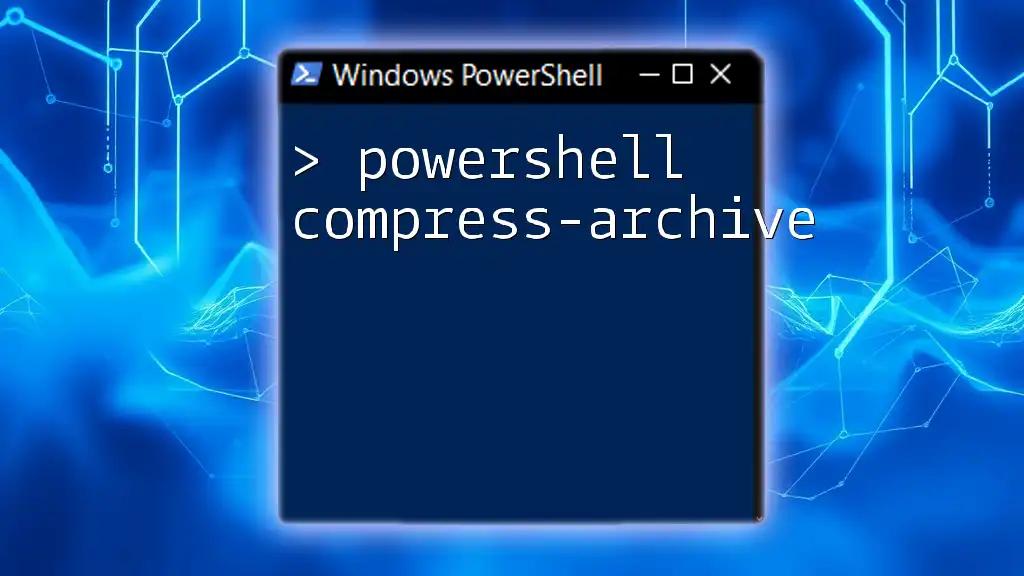
Conclusion
Incorporating the practice of password encryption within your PowerShell scripts is vital for maintaining the integrity and security of sensitive information. By following the guidelines and methods presented in this article, you can effectively utilize PowerShell to encrypt passwords and enhance confidentiality in your automation tasks. Implementing these strategies will not only protect sensitive data but also instill a stronger security posture in your operations. Explore further training opportunities to continually enhance your skills in PowerShell and cybersecurity.