In PowerShell, you can find an element in an array using the `-contains` operator, which checks if the specified value exists within the array.
Here's a code snippet demonstrating this:
$array = @("apple", "banana", "cherry")
$result = $array -contains "banana"
Write-Host "Found banana: $result"
Understanding Arrays in PowerShell
What is an Array?
An array is a data structure that can hold multiple items in a single variable. In programming, arrays are fundamental for organizing data efficiently. In PowerShell, arrays allow you to store lists of items ranging from strings to objects, making them extremely versatile for various tasks.
Creating Arrays in PowerShell
Creating an array in PowerShell is straightforward. You can use several methods:
- Traditional Array Creation: You can define an array using commas to separate its elements.
$myArray = "Apple", "Banana", "Cherry"
- Using the `@()` Syntax: This method is often preferred for its clarity, particularly when creating an empty array.
$myArray = @("Apple", "Banana", "Cherry")
This approach conveniently allows for both initialization and dynamic updates.
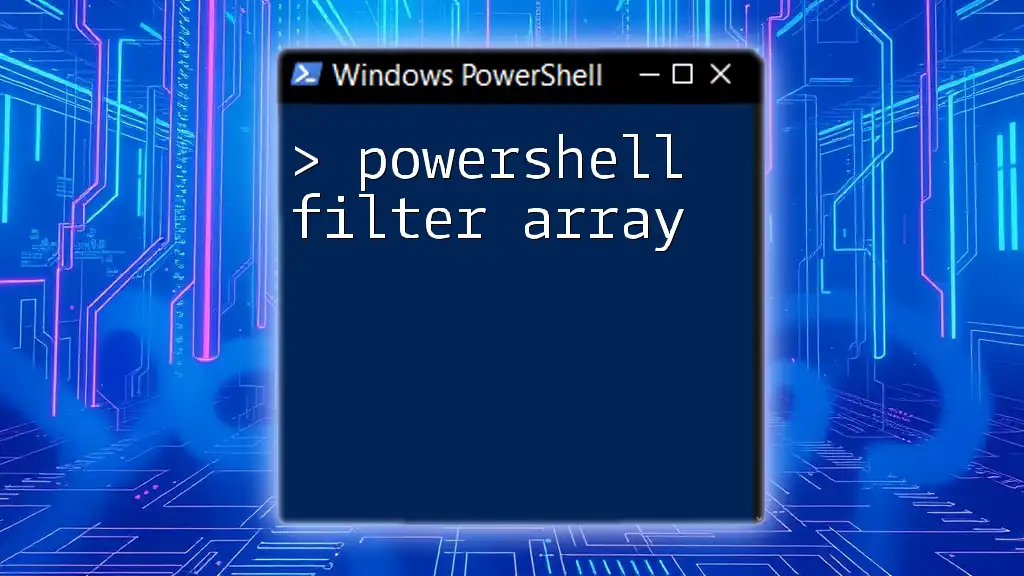
The Need for Array Searching
Why Search Arrays?
Searching for an element in an array becomes essential in real-world applications where you need to find specific data points quickly. For example, if you're managing a list of devices or user accounts, you might need to verify whether a particular entry exists.
Performance Considerations in Larger Datasets
As the size of your array increases, the search performance can degrade. Thus, knowing how to search effectively is vital to maintain efficiency.
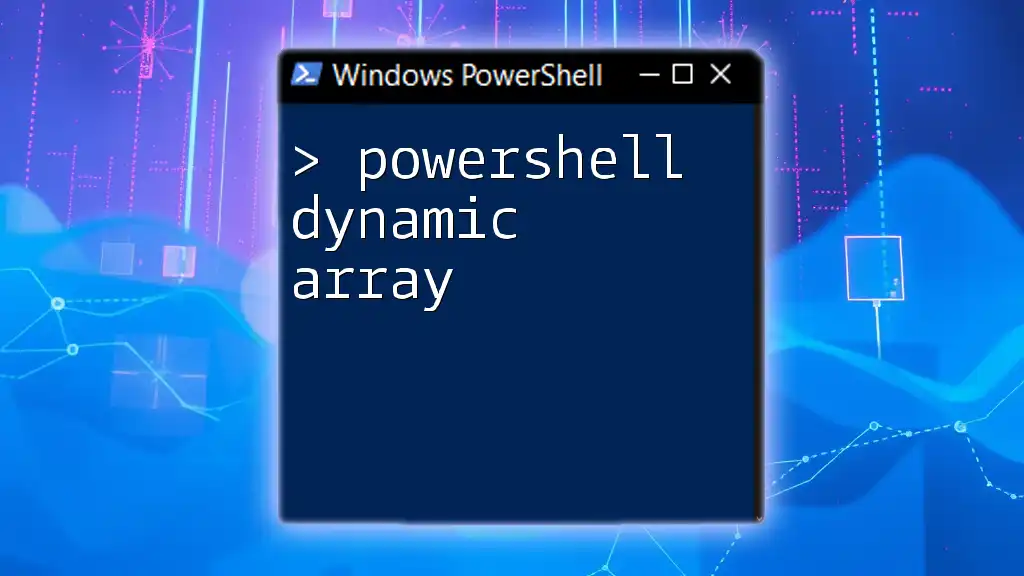
PowerShell Search Array Basics
How to Search for an Element in an Array
The most straightforward way to search for an element in an array is to use the `-contains` operator. This operator returns `True` if the specified element exists in the array.
$fruits = @("Apple", "Banana", "Cherry")
$exists = $fruits -contains "Banana"
Write-Host "Banana exists in the array: $exists"
You can also use the `-in` operator, which checks for an item in an array but with reversed syntax.
$exists = "Cherry" -in $fruits
Write-Host "Cherry exists in the array: $exists"
Searching for Multiple Elements
To search for multiple elements, you can leverage `Where-Object` to filter items in an array based on certain criteria.
$fruits = @("Apple", "Banana", "Cherry", "Avocado")
$result = $fruits | Where-Object { $_ -like "*a*" }
Write-Host "Fruits containing 'a': $result"
This snippet returns all fruits that contain the letter 'a', showcasing how to use PowerShell’s piping and filtering capabilities effectively.
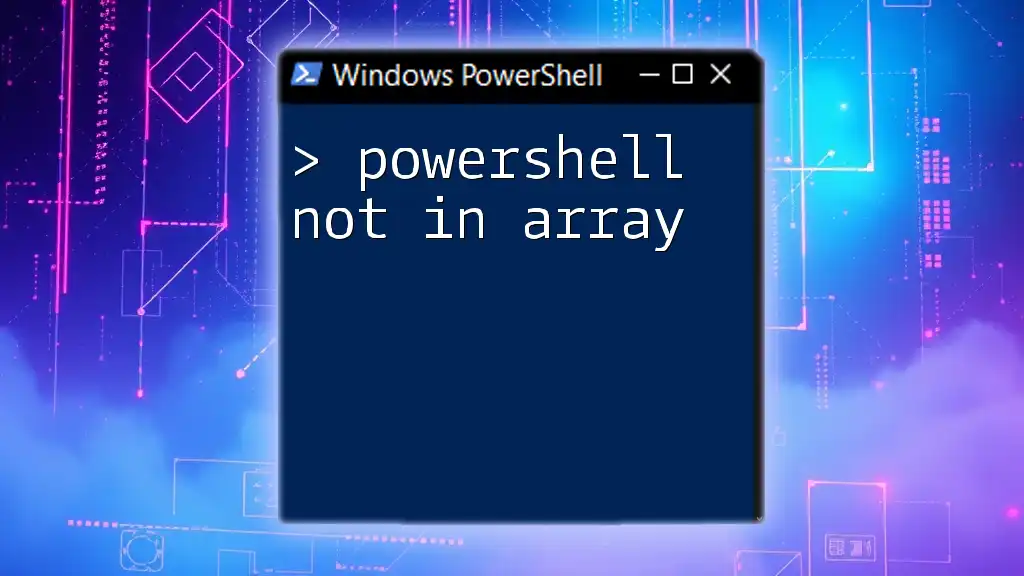
Advanced Techniques for Array Searching in PowerShell
Using `Select-String` for Pattern Matching
For more complex searches, particularly with strings, you can utilize `Select-String`. It's a powerful command in PowerShell that finds text patterns in strings or files.
$fruits = @("Apple", "Banana", "Cherry", "Avocado")
$matched = $fruits | Select-String -Pattern 'a'
Write-Host "Fruits containing 'a': $($matched.Line)"
This example returns fruits matching the pattern 'a', demonstrating the search capabilities beyond simple equality checks.
Leveraging Linq with PowerShell for Complex Searches
If you want to perform more advanced queries, you can integrate LINQ-like functionality using the `System.Linq` namespace. PowerShell allows this through .NET capabilities.
Add-Type -AssemblyName System.Core
$fruits = @("Apple", "Banana", "Cherry", "Avocado")
$results = [System.Linq.Enumerable]::Where($fruits, { $_ -like "*a*" })
Write-Host "Fruits containing 'a': $results"
This example allows for more complex filtering and can be tailored according to your specific needs.
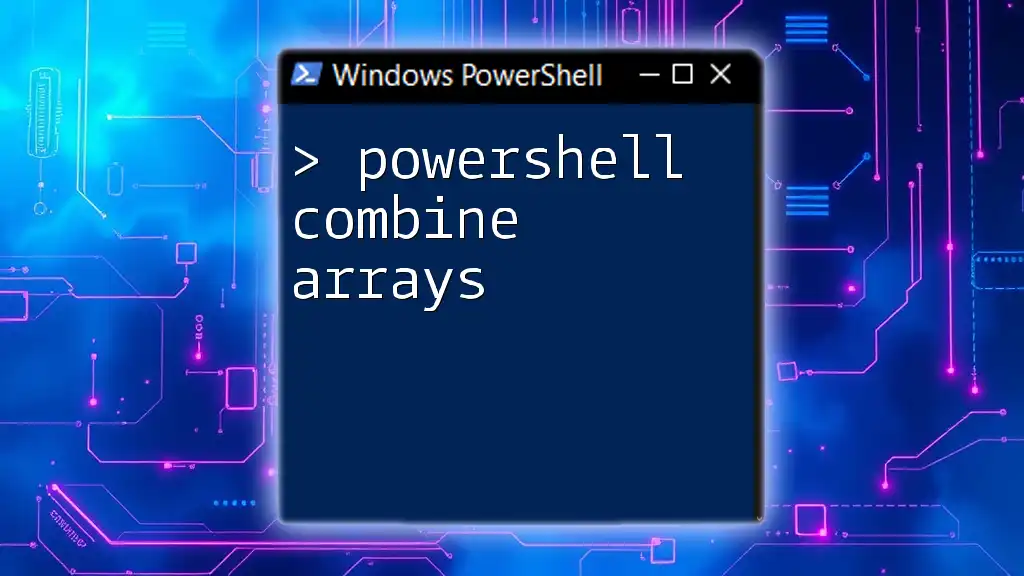
Custom Functions for Array Search
Creating Custom Search Functions
Creating custom functions for searching arrays can enhance your scripts' efficiency and readability. Here’s a simple custom function to demonstrate this.
function Search-Array {
param (
[string[]]$Array,
[string]$SearchTerm
)
return $Array -contains $SearchTerm
}
$fruits = @("Apple", "Banana", "Cherry")
$result = Search-Array -Array $fruits -SearchTerm "Banana"
Write-Host "Banana found: $result"
This function takes an array and a search term, returning a boolean indicating the presence of the term. With this function, you can easily reuse it for different data sets.
Error Handling in Custom Functions
Effective error handling is crucial for ensuring that your scripts run smoothly. Adding checks can enhance your custom functions.
function Search-Array {
param (
[string[]]$Array,
[string]$SearchTerm
)
if (-not $Array -or -not $SearchTerm) {
throw "Array and SearchTerm must be provided."
}
return $Array -contains $SearchTerm
}
This modification ensures that the function won’t try to search if the inputs are invalid, thus preventing runtime errors.
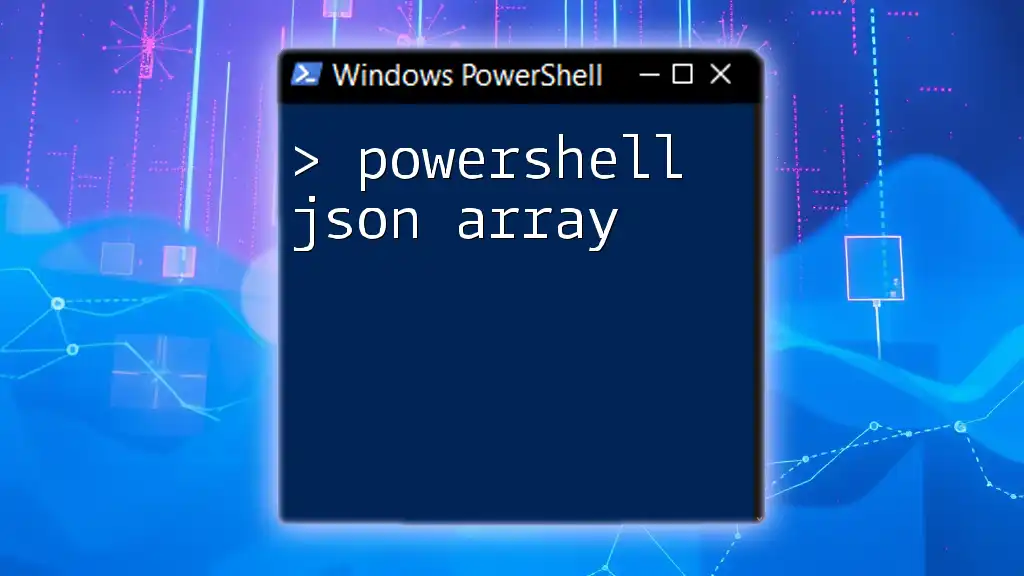
Performance Considerations
Evaluating Search Speed
Testing the performance of various array search techniques can provide insights into their efficiencies. You can use `Measure-Command` to analyze how long a command takes to execute.
$measure = Measure-Command {
$fruits = 1..10000 | ForEach-Object { "Fruit$_" }
$exists = $fruits -contains "Fruit5000"
}
Write-Host "Search took: $($measure.TotalMilliseconds) ms"
Best Practices for Efficient Array Searching
To optimize during searches, consider the method that best suits your dataset size:
- For small arrays, `-contains` is often sufficient.
- For large arrays, consider using `Where-Object` or LINQ for efficiency.
- Be mindful of casting or converting types, as unnecessary transformations can introduce overhead.
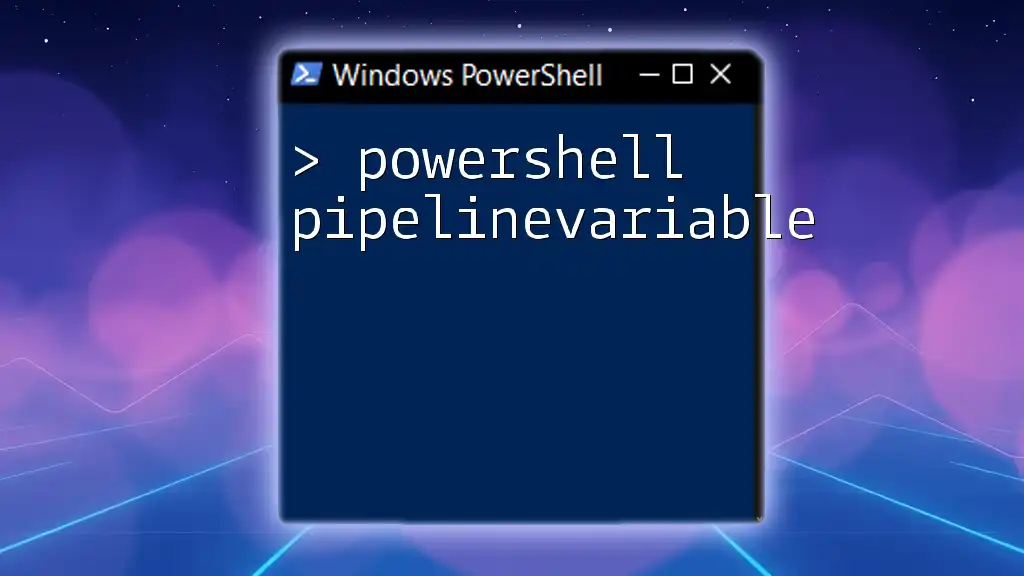
Real-World Applications
Use Case: Searching User Data
In scenarios like analyzing logs or user data, effective searches are essential. For example, if you're checking a Security log array for multiple entries to understand access patterns, use the search methods discussed.
$logEntries = @("User1 - Access", "User2 - Denied", "User3 - Access")
$filteredLogs = $logEntries | Where-Object { $_ -like "*Access*" }
Write-Host "Access logs: $filteredLogs"
Use Case: Analytics and Reporting
In reporting, searching through data arrays to generate insights is crucial. For instance, aggregate report data based on conditions can reveal patterns over time.
$reportData = @("Sales1:100", "Sales2:200", "Sales3:150")
$results = $reportData | Where-Object { $_ -like "*200*" }
Write-Host "Sales Reports with 200: $results"
This demonstration reveals how you can pull specific sales data in a concise manner to streamline reporting efforts.
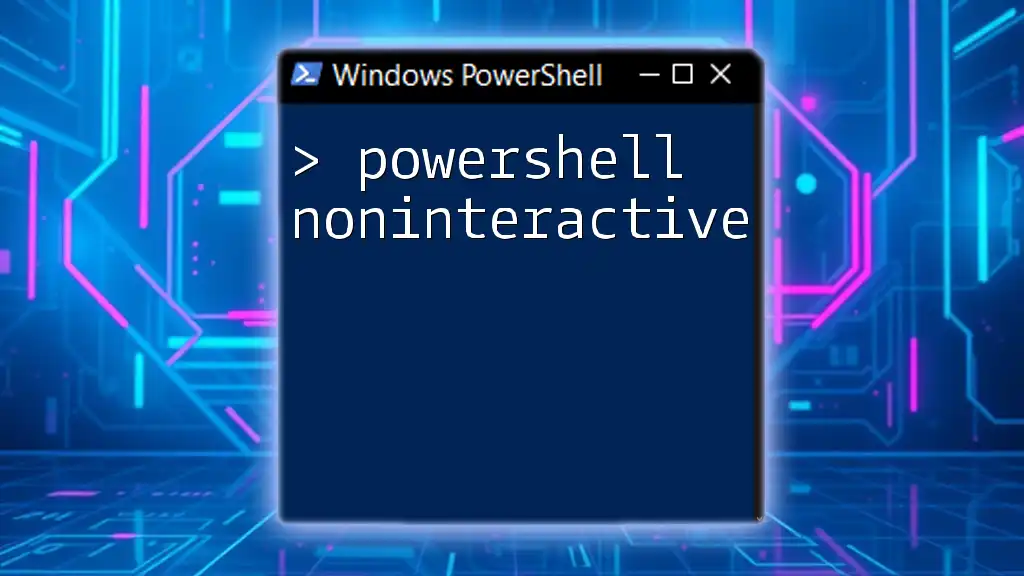
Conclusion
Exploring how to effectively perform a PowerShell find in array allows you to manage and interrogate data more efficiently in your scripts. The methods showcased provide you with versatile tools to achieve quick and reliable searches.
Whether you choose built-in operators, leverage advanced techniques, or create custom functions, the ability to search arrays in PowerShell opens up vast possibilities for data handling. Don’t hesitate to practice these methods to enhance your skills further—effortlessly navigating arrays can significantly impact your productivity.
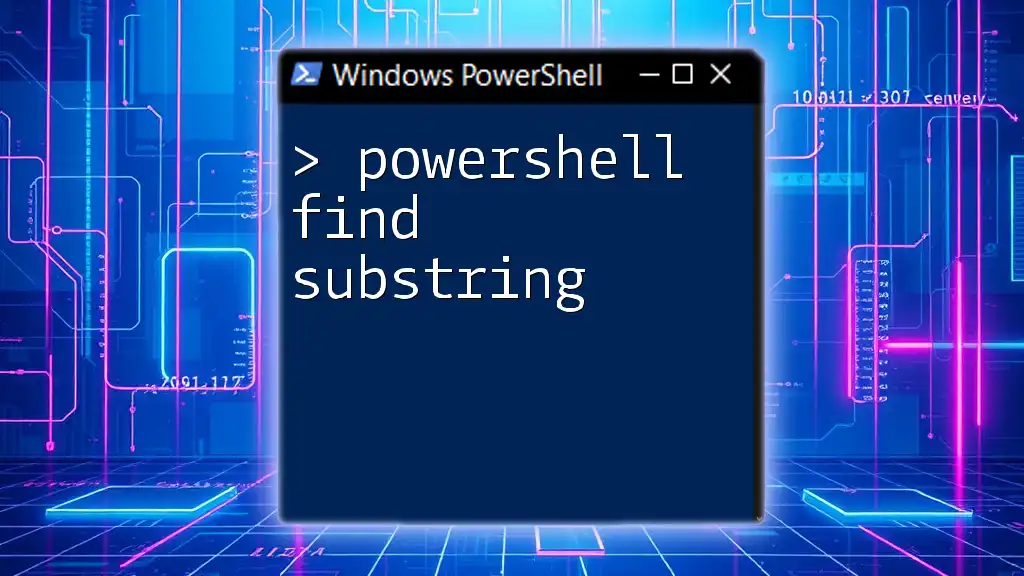
Additional Resources
Further Reading
For more in-depth information and methods, explore the [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/), which covers numerous topics around arrays and their functionalities.
Community and Support
Join PowerShell user groups and online forums to connect with experts. Follow our company for more tutorials and tips on mastering PowerShell.
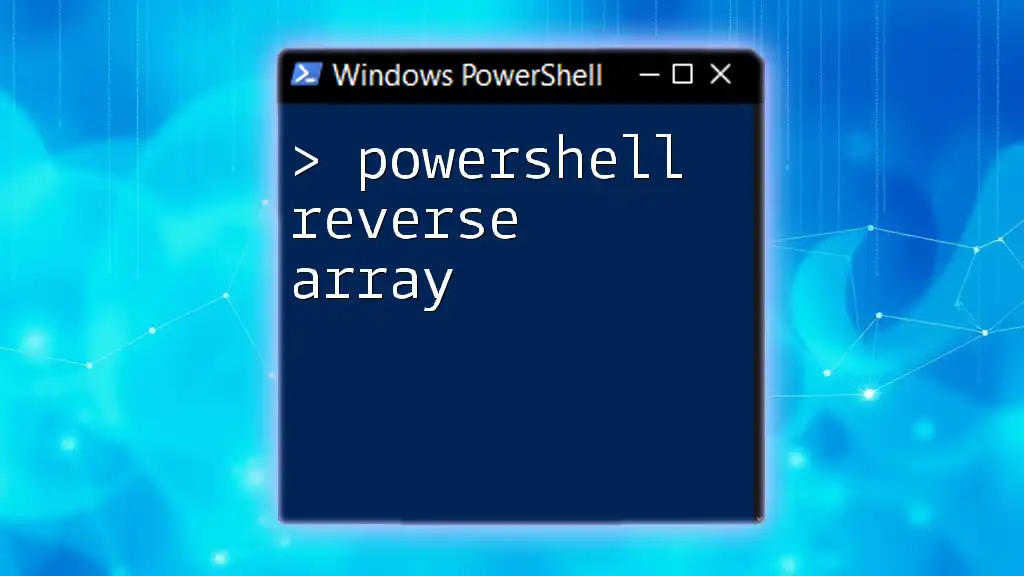
FAQ
Common Questions About PowerShell Array Searching
- What happens when I search for an element not in the array? You will receive a `False` result if the element is not present.
- Can I search multidimensional arrays? Yes, but the methods may be different, often requiring nested loops or advanced filtering.
- How do I sort arrays before searching, if needed? Use the `Sort-Object` cmdlet to organize your data before performing a search.
By applying the concepts and techniques outlined in this guide, you are well on your way to becoming proficient at searching arrays in PowerShell.