Sure! In PowerShell, "find and replace" can be achieved using the `-replace` operator to substitute specific substrings in a string or content from a file.
Here's a code snippet demonstrating how to find and replace text in a string:
# Example: Replacing 'World' with 'PowerShell'
$text = 'Hello, World!'
$updatedText = $text -replace 'World', 'PowerShell'
Write-Host $updatedText # Output: Hello, PowerShell!
Understanding PowerShell's String Manipulation Capabilities
What is String Manipulation?
String manipulation refers to the processes of creating, modifying, or analyzing strings—textual data—within programming and scripting languages. This is crucial for tasks such as data processing, reporting, and automation. In PowerShell, robust string manipulation capabilities allow users to seamlessly integrate data handling into their scripts.
The Role of PowerShell in String Manipulation
PowerShell offers a variety of cmdlets and operators specifically designed for working with strings. This includes reading, searching, and modifying text data efficiently. Among these functionalities, the ability to find and replace text is vital, as it helps users modify configurations, update content, and perform batch operations across files.
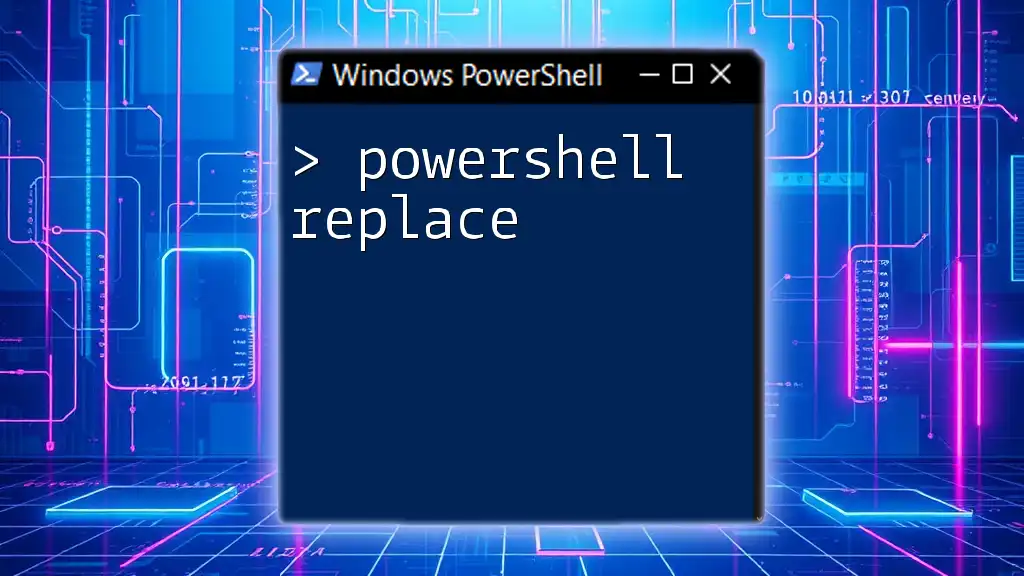
Finding Text in PowerShell
Using the `Select-String` Cmdlet
The `Select-String` cmdlet serves as PowerShell's built-in method for searching through text. Its versatility allows you to search not only through strings but also within files, making it an invaluable tool.
Syntax and Parameters Explained:
Select-String -Path <Path> -Pattern <Pattern>
- `-Path`: Specifies the files to search through.
- `-Pattern`: Defines the text pattern you are searching for.
Example: Basic Usage
To demonstrate the utility of `Select-String`, consider the following example, which searches for a term within a specified text file:
Get-Content "file.txt" | Select-String "searchTerm"
This line reads the contents of `file.txt` and pipes it to `Select-String`, which will return all instances of "searchTerm" found in the file.
Searching in Files and Directories
You can also search recursively through all files within a directory using `Get-ChildItem` in combination with `Select-String`.
Example: Search for Multiple Patterns
Get-ChildItem -Path "C:\path\to\files" -Recurse | Select-String -Pattern "term1", "term2"
This command searches for both "term1" and "term2" across all files in the specified directory and subdirectories, yielding a comprehensive search result.
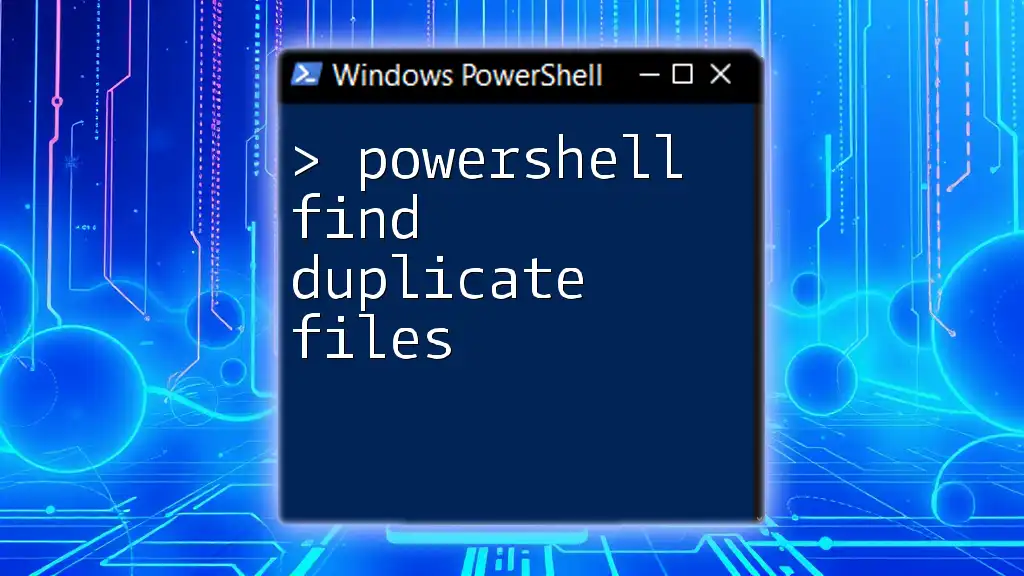
Replacing Text in PowerShell
Using the `-replace` Operator
The `-replace` operator in PowerShell is a versatile and straightforward way to replace substrings within strings. This operator is particularly useful for quickly altering content.
Syntax and Examples of Usage:
<string> -replace <pattern>, <replacement>
Example: Standard Replacement Suppose we have a string that needs modification:
$string = "Hello World"
$newString = $string -replace "World", "PowerShell"
After executing the above code, `$newString` will now hold the value "Hello PowerShell", demonstrating a basic find and replace operation.
Replacing Text in Files
You can directly manipulate the contents of a text file by reading its content, performing the replacement, and then writing back to the same file.
Example: Directly Modifying File Content
(Get-Content "file.txt") -replace "oldText", "newText" | Set-Content "file.txt"
In this example, any occurrence of "oldText" in "file.txt" will be replaced with "newText", refreshing the file with the updated content.
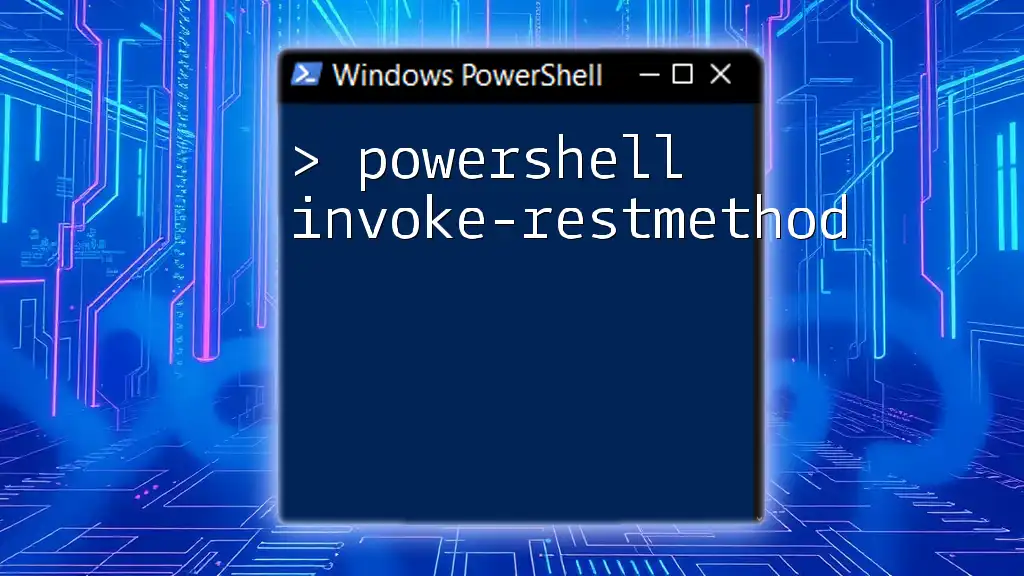
Advanced Find and Replace Techniques
Using Regular Expressions with `-replace`
Regular expressions are powerful tools for pattern matching and can greatly enhance your find-and-replace capabilities. They provide the mechanism to find complex patterns rather than simple text.
Why and When to Use Regular Expressions: Regular expressions are particularly useful when you need to find strings that meet certain patterns, such as matching phone numbers or emails.
Example: Replace Using Regex
$string = "123-456-7890"
$newString = $string -replace "\d", "X"
In this case, every digit in the string "123-456-7890" is replaced with "X", resulting in "XXX-XXX-XXXX". This illustrates how regex can be employed for advanced text manipulation.
Multiple Replacement Patterns
Sometimes you may need to replace more than one string simultaneously. This can be efficiently achieved using a hashtable.
Example: Using a Hashtable for Replacements
$patterns = @{ "apple" = "orange"; "red" = "blue" }
$text = "I have an apple and a red car."
foreach($key in $patterns.Keys) {
$text = $text -replace $key, $patterns[$key]
}
In this example, both "apple" and "red" will be replaced with "orange" and "blue", respectively, demonstrating how you can streamline multiple replacement operations.
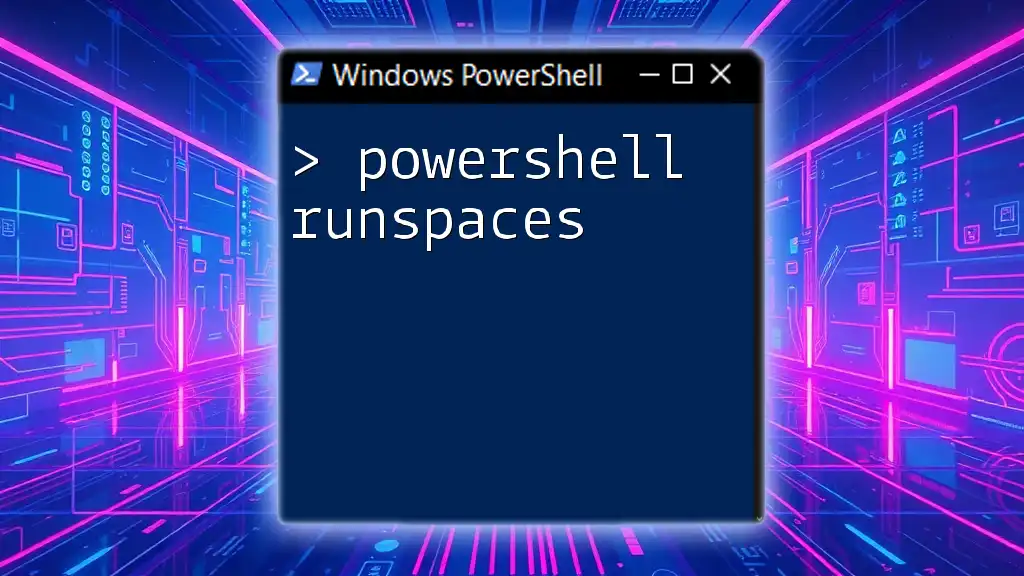
Use Cases for Find and Replace
Editing Configuration Files
The find and replace functionality is particularly salient when it comes to editing configuration files. For example, network settings, application configurations, and user settings often require updates. PowerShell's ability to automate these changes saves both time and effort.
Updating Scripts and Codebases
Software developers often find themselves needing to update specific variables or function names across a script or codebase. Utilizing PowerShell's find and replace capabilities allows for quick adjustments, significantly enhancing productivity.
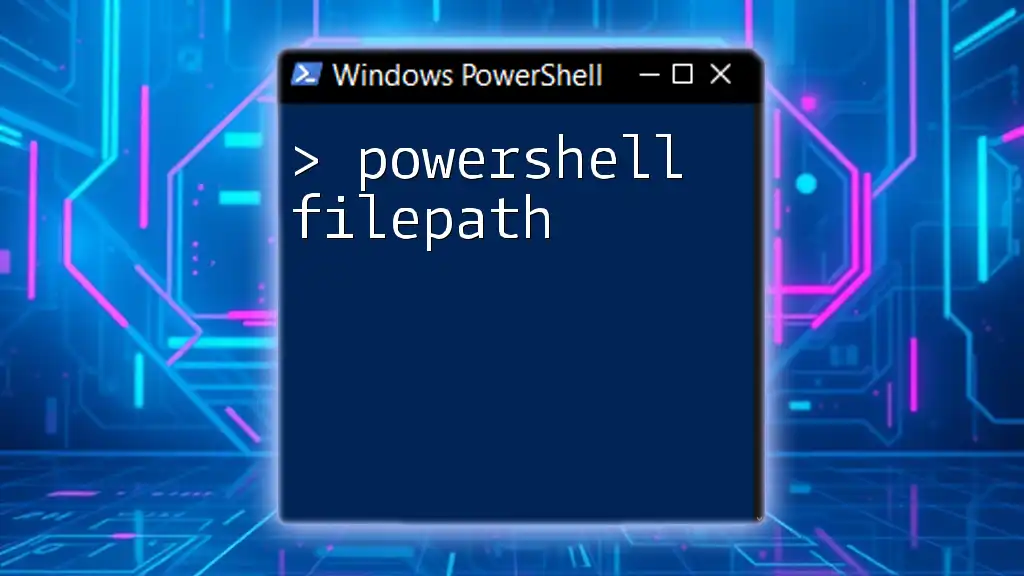
Troubleshooting Common Issues
While using PowerShell for find and replace tasks, users may encounter common pitfalls such as:
- Incorrect Pattern Matching: Ensure that the patterns you use correctly reflect the text you want to match.
- File Handling Errors: Read permissions or access issues can prevent successful file modifications.
Common Errors and Debugging Strategies
If you face an error, use the `Write-Host` cmdlet to output values at various points in your script. This can help diagnose issues by showing what your variables contain at runtime.
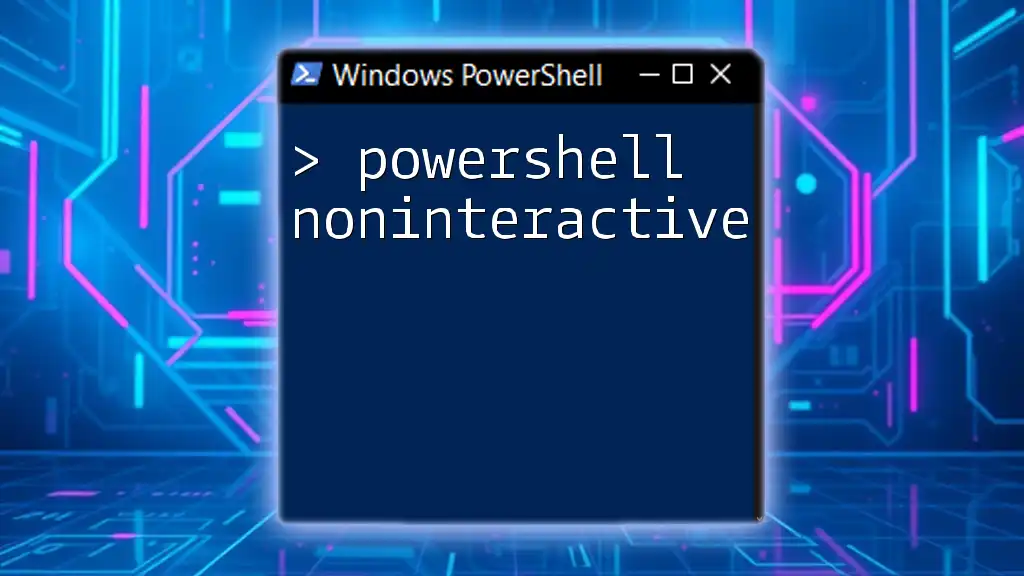
Best Practices for Using Find and Replace in PowerShell
- Keep Backups of Original Files: Before performing any replacement operations, always make a backup of your files to prevent data loss.
- Use Verbose and Debug Parameters: When executing scripts, consider using the `-Verbose` and `-Debug` parameters to receive detailed execution information, thereby helping identify issues promptly.
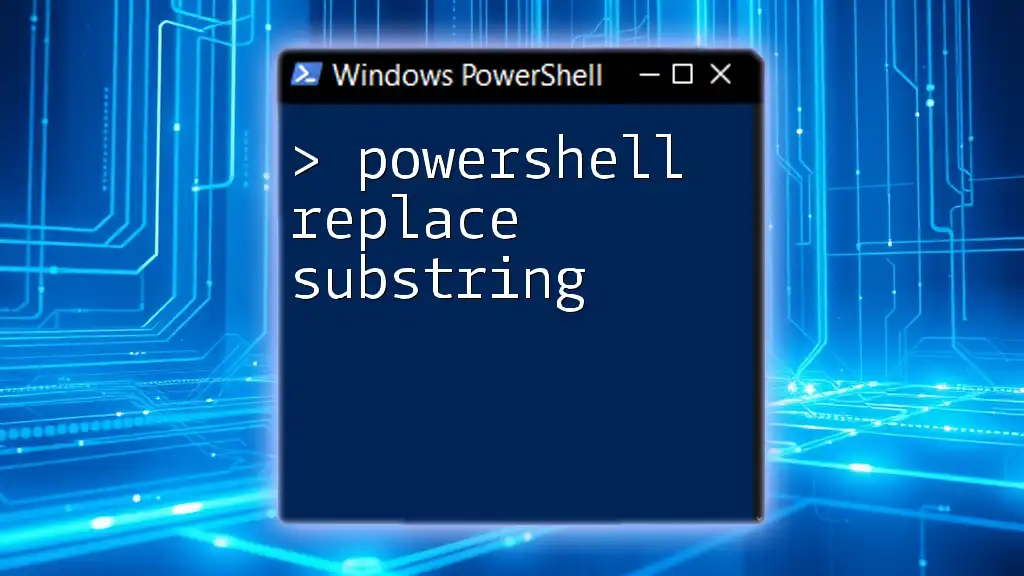
Conclusion
Mastering the PowerShell find and replace capabilities equips you with the necessary skills to efficiently manage text data. Practice with the examples provided in this guide to solidify your understanding, and remember to explore more advanced features at your own pace. For further training and resources, take advantage of community forums and official documentation to continue your PowerShell journey.
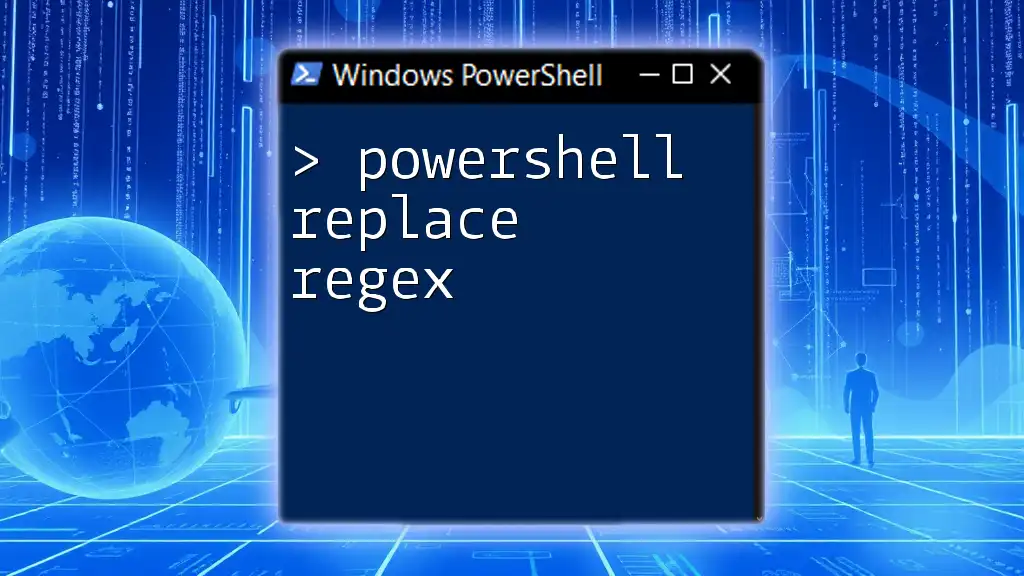
Additional Resources
- Explore the official PowerShell documentation for cmdlet reference and additional information on string manipulation.
- Join PowerShell community forums for tips, troubleshooting advice, and collaborative learning opportunities.
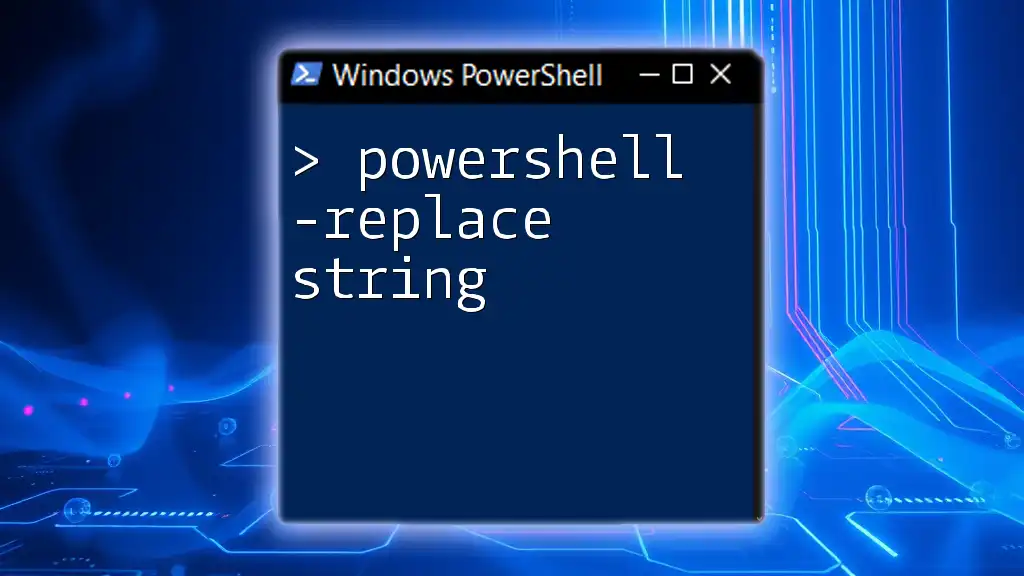
FAQs
PowerShell's find and replace features can lead to numerous questions from users. Common inquiries include:
-
How can I safely test find and replace operations? Always run a test on a duplicate of your data before applying changes to ensure desired outcomes.
-
Is it possible to preview changes before applying them? You can use `Select-String` to find occurrences before executing a replace operation, allowing for informed adjustments.
By addressing these questions, you'll not only enhance your skills but also build confidence in using PowerShell effectively.