To copy and rename a file in PowerShell, you can use the `Copy-Item` cmdlet followed by the original file path and the new file path with the desired name.
Copy-Item -Path "C:\path\to\original\file.txt" -Destination "C:\path\to\new\file_renamed.txt"
Understanding PowerShell Basics
What is PowerShell?
PowerShell is a powerful scripting and automation framework developed by Microsoft. It is designed to help users automate tasks and manage systems more efficiently through command-line interface (CLI) and scripting. With its extensive range of cmdlets, PowerShell allows users to perform complex operations easily, making it an essential tool for system administrators and IT professionals.
Why Use PowerShell for File Management?
Using PowerShell for file management offers several advantages over traditional graphical user interfaces (GUIs):
- Automation: PowerShell scripts can automate repetitive tasks, saving time and minimizing errors.
- Flexibility: Users can manipulate files, folders, and system settings in a flexible manner using complex commands tailored to their needs.
- Batch Processing: Users can process multiple files and directories in a single command without needing to click through menus.
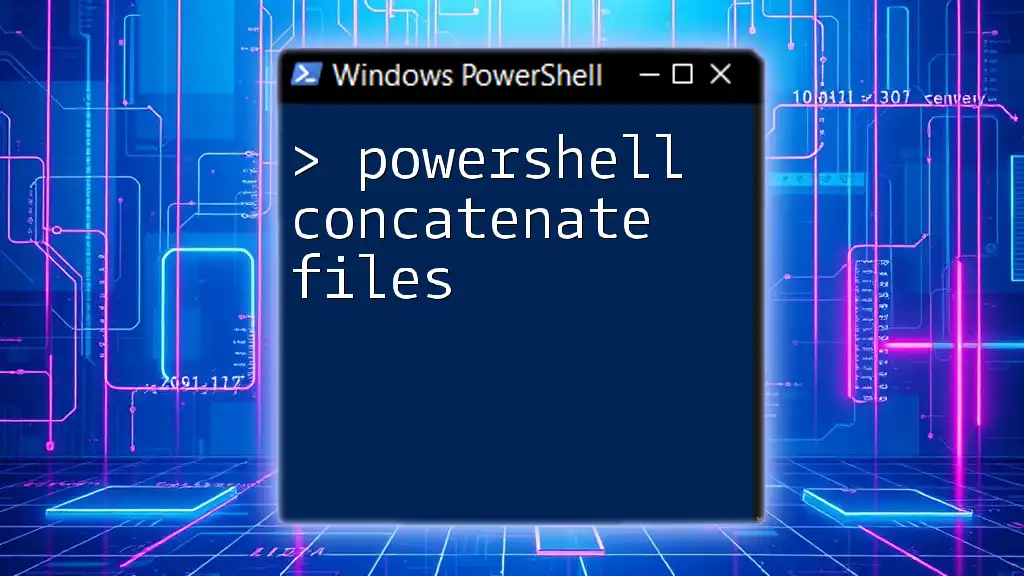
The Copy-Item Cmdlet in PowerShell
What is the Copy-Item Cmdlet?
The `Copy-Item` cmdlet is used in PowerShell to copy files and directories from one location to another. This command is essential when you need to duplicate files without altering the original.
Syntax:
Copy-Item -Path <string> -Destination <string> [-Recurse] [-Force]
Basic Usage of Copy-Item
To copy a single file, you can use the following command:
Copy-Item -Path "C:\Source\file.txt" -Destination "C:\Destination\file.txt"
In this example:
- -Path specifies the location of the file you want to copy.
- -Destination indicates where you want the new copy to be created.
By using the `-Recurse` parameter, you can copy entire directories and their contents.
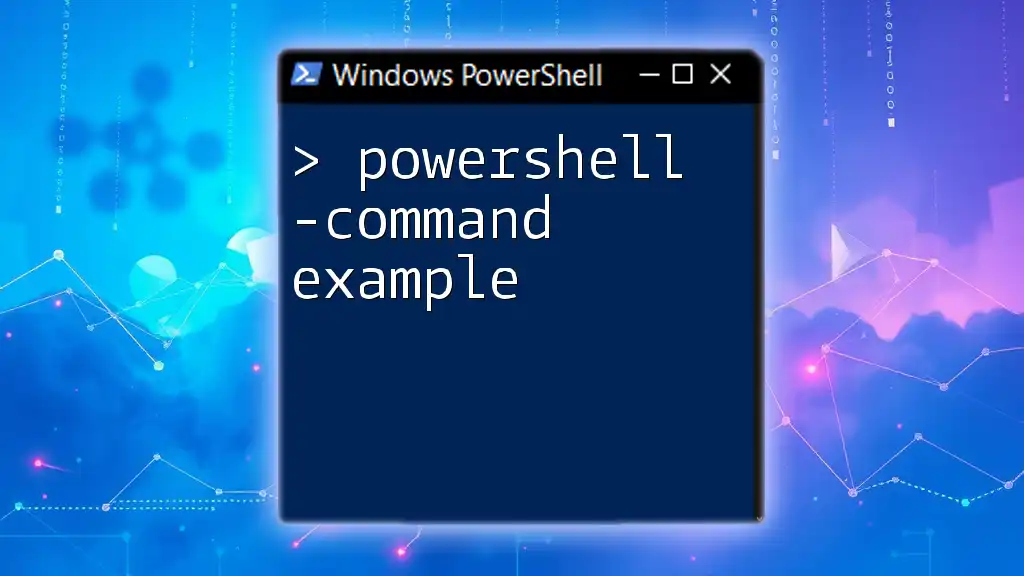
Renaming Files in PowerShell
Introduction to the Rename-Item Cmdlet
The `Rename-Item` cmdlet allows users to change the name of an existing item, such as a file or a folder. This command works seamlessly within PowerShell, empowering users to rename files without opening them or using a graphical interface.
Syntax:
Rename-Item -Path <string> -NewName <string>
Basic Usage of Rename-Item
Here’s how to rename a single file:
Rename-Item -Path "C:\Destination\file.txt" -NewName "newfile.txt"
This command effectively updates the name of `file.txt` to `newfile.txt`.
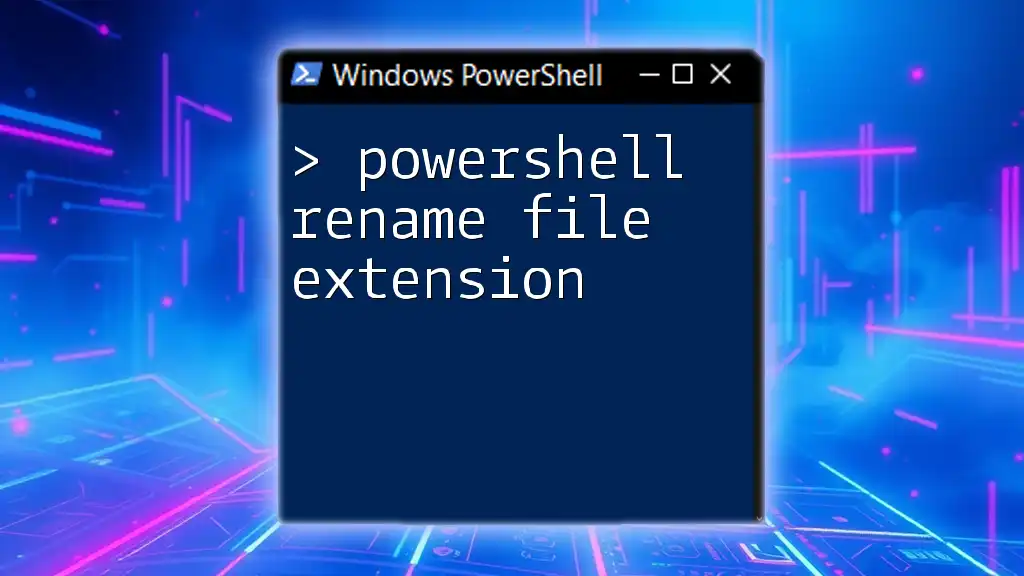
Combining Copy and Rename Operations
Step-by-step Approach
It’s possible to optimize your workflow by combining the `Copy-Item` and `Rename-Item` commands in a sequence. This is especially useful when you want to immediately rename a file after copying it.
Example of Copying and Renaming in One Go
Here’s how to perform both actions in two simple commands:
Copy-Item -Path "C:\Source\originalFile.txt" -Destination "C:\Destination\originalFile.txt"
Rename-Item -Path "C:\Destination\originalFile.txt" -NewName "renamedFile.txt"
In this example:
- The first command creates a copy of `originalFile.txt` in the `C:\Destination` directory.
- The second command renames the copied file to `renamedFile.txt`. This structure is easy to follow and ensures clarity in your script.
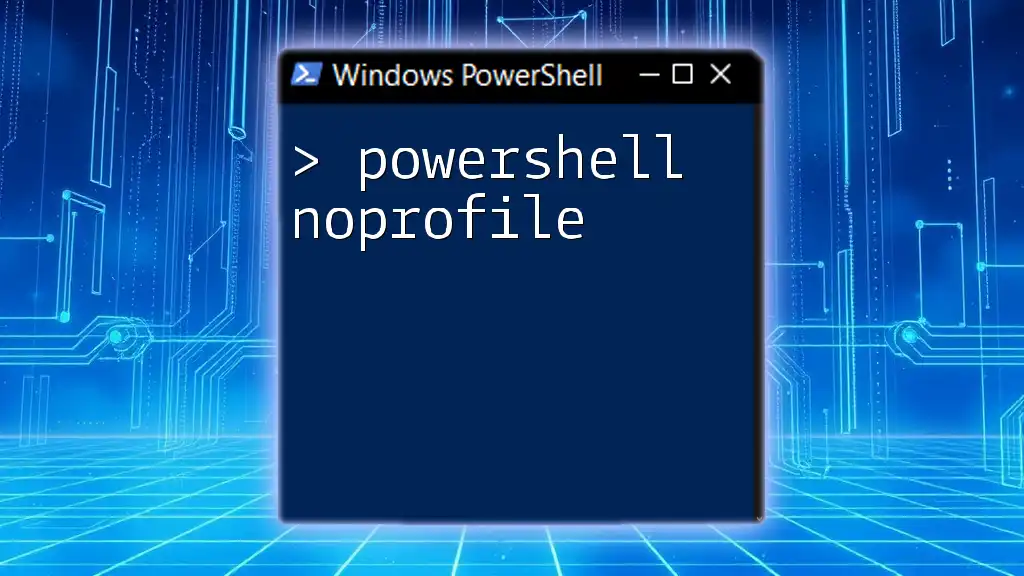
Handling Errors and Troubleshooting
Common Errors When Copying and Renaming
When working with file operations, it’s essential to be aware of potential errors. Common issues include:
- File Not Found: If the specified source file does not exist, PowerShell will throw an error.
- Permission Issues: You may encounter problems if you do not have the necessary permissions to access or modify the files.
- Path Format Problems: Always ensure that the file path is correctly formatted and that paths do not contain illegal characters.
Tips for Successful Execution
To improve reliability, consider performing error checks before executing commands. For example, you can use the `Test-Path` cmdlet to verify that the source file exists:
if (Test-Path "C:\Source\file.txt") {
Copy-Item -Path "C:\Source\file.txt" -Destination "C:\Destination\file.txt"
} else {
Write-Host "Source file does not exist."
}
For more robust error management, implement `try-catch` blocks:
try {
Copy-Item -Path "C:\Source\file.txt" -Destination "C:\Destination\file.txt"
} catch {
Write-Host "An error occurred: $_"
}
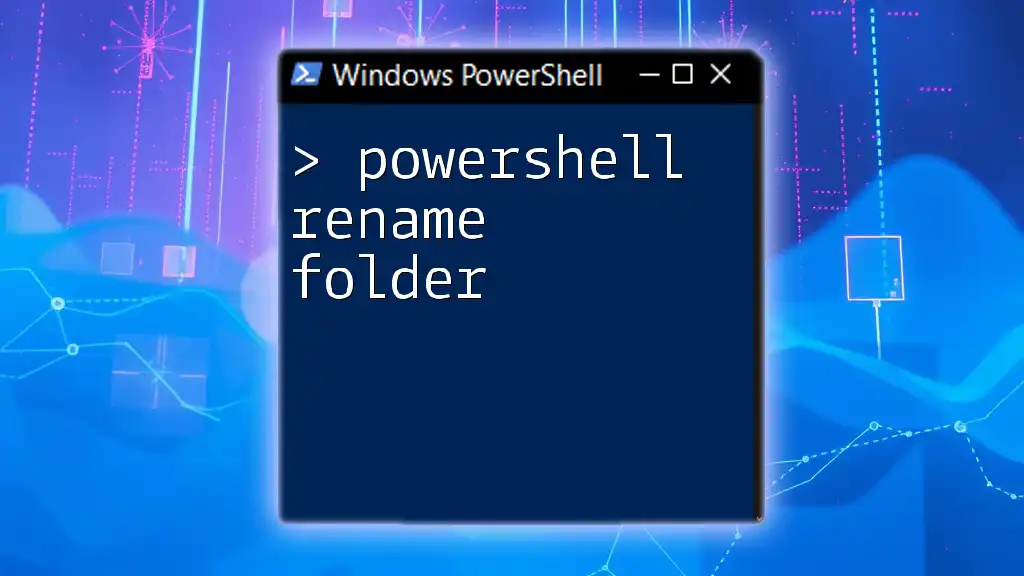
PowerShell Scripting for File Management
Creating a Script to Copy and Rename Files
Creating a script to automate the file copying and renaming process enhances efficiency, especially for tasks performed repeatedly. Here's a simple example:
$sourcePath = "C:\Source\originalFile.txt"
$destinationPath = "C:\Destination\renamedFile.txt"
Copy-Item -Path $sourcePath -Destination $destinationPath
Rename-Item -Path $destinationPath -NewName "newFile.txt"
This script defines the source path and destination path variables, then executes the copy and rename operations.
Automating File Management Tasks
To further enhance your productivity, consider scheduling your PowerShell scripts to run at specified intervals using Task Scheduler. This way, you can automate file management tasks without manual intervention, thus maintaining workflow efficiency.
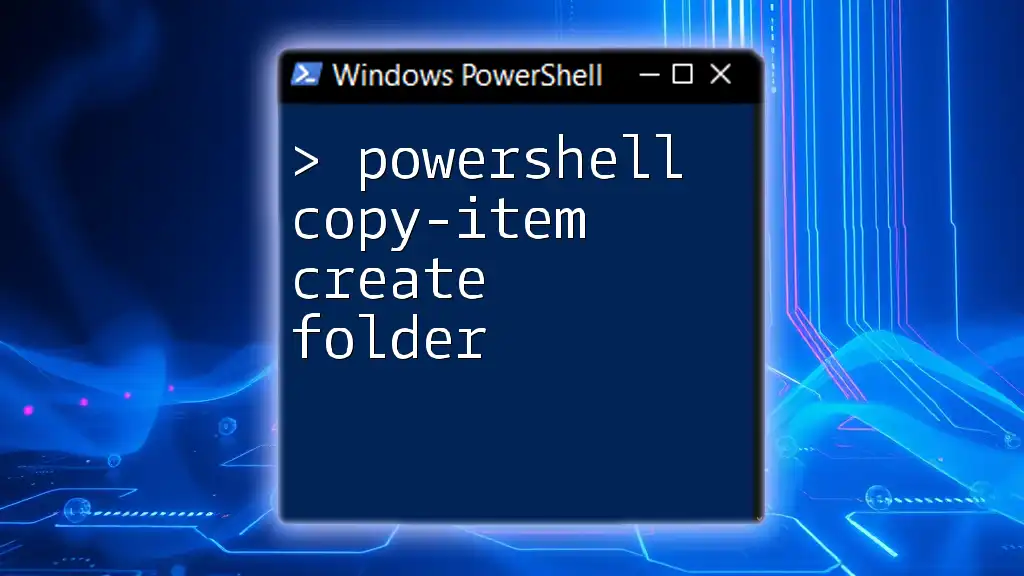
Conclusion
Mastering the PowerShell copy and rename file operations empowers users to manage their files effectively. By understanding cmdlets like `Copy-Item` and `Rename-Item`, you can navigate through your data with confidence and ease. Whether you are an IT professional or a casual user, leveraging PowerShell for file tasks can save you time and effort.
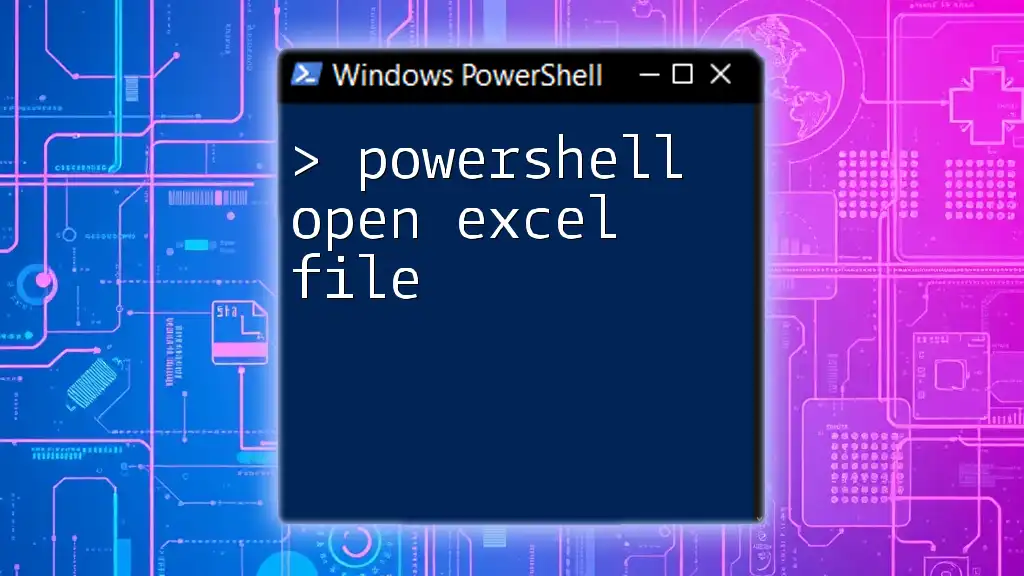
Additional Resources
To further enhance your understanding and skills in PowerShell, consider exploring Microsoft's official PowerShell documentation and taking advantage of online tutorials related to file management and scripting techniques.
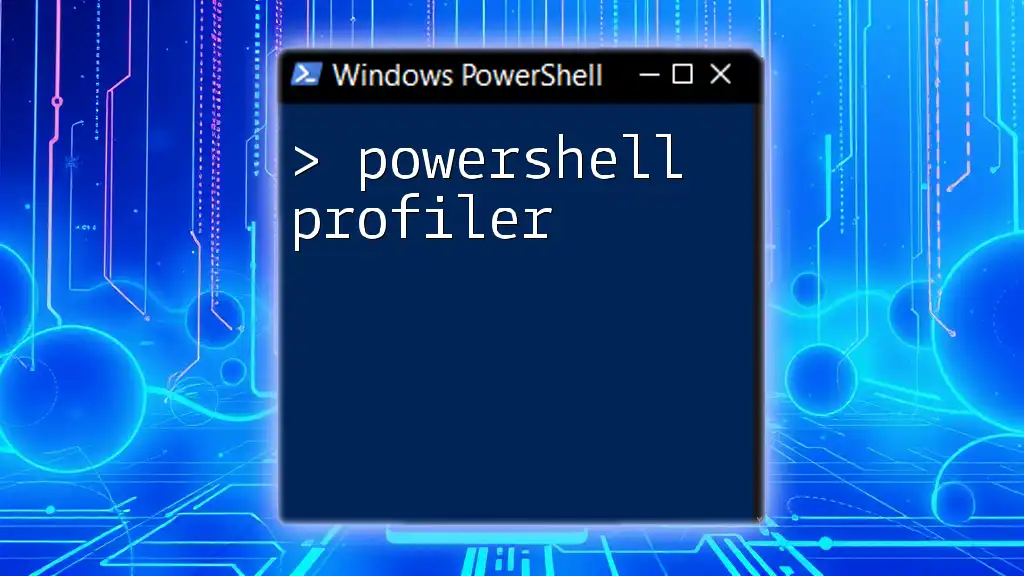
Call to Action
Engage with us for in-depth PowerShell training and resources that will help you elevate your skills and streamline your IT tasks effectively!