To find a character in a string using PowerShell, you can utilize the `-contains` operator or the `.IndexOf()` method to check if the character exists and retrieve its position. Here's a code snippet demonstrating this concept:
$string = "Hello, World!"
$charToFind = 'W'
$index = $string.IndexOf($charToFind)
if ($index -ge 0) {
Write-Host "Character '$charToFind' found at index $index."
} else {
Write-Host "Character '$charToFind' not found."
}
Understanding Strings in PowerShell
What is a String?
A string is a sequence of characters used to represent text in programming. In PowerShell, strings play a crucial role, as they are fundamental to operations such as data manipulation, user input handling, and output formatting. Strings can contain letters, numbers, symbols, and spaces, making them versatile for various tasks.
Working with PowerShell Strings
To create a string in PowerShell, you can use either single (`'`) or double (`"`) quotes. The key difference is that double quotes allow for variable interpolation, meaning you can include variable values directly within the string.
For example, a simple string can be created as follows:
$greeting = "Hello, World!"
In this example, the variable `$greeting` now holds the string "Hello, World!".
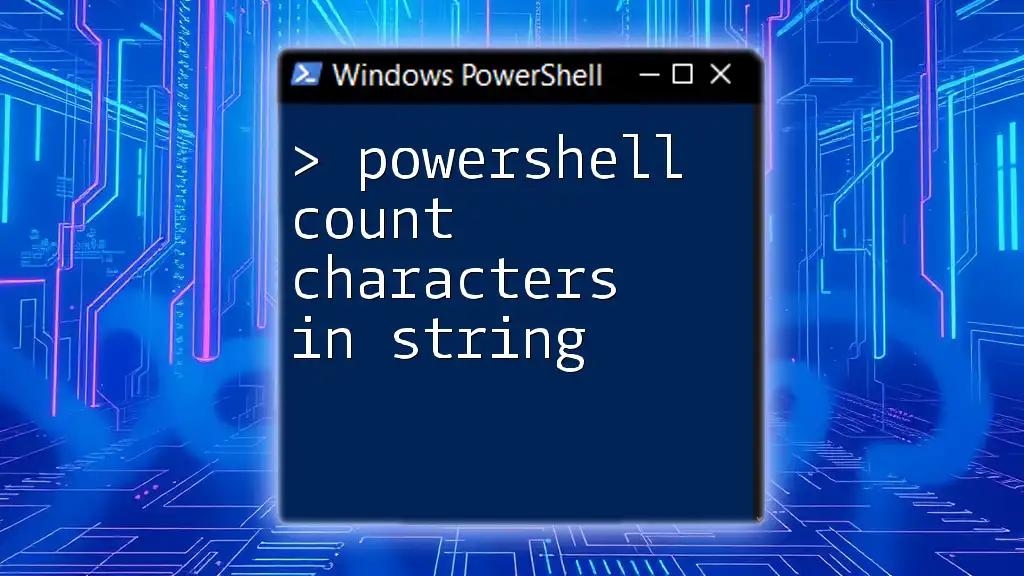
Finding Characters within a String
Using the IndexOf Method
One of the simplest ways to find a character within a string in PowerShell is to use the `IndexOf` method. This method returns the zero-based index of the first occurrence of the specified character within the string. If the character is not found, it returns `-1`.
The syntax is as follows:
String.IndexOf(Char)
Example
To illustrate, let's find the character "S" in the string "PowerShell":
$text = "PowerShell"
$position = $text.IndexOf("S")
Write-Host "The character 'S' is found at position: $position"
This code snippet will output:
The character 'S' is found at position: 5
This example demonstrates how the `IndexOf` method works, showing that indexing starts at 0, where "P" is at position 0, "o" at 1, and so forth.
Using the Contains Method
Another useful method for finding characters in a string is `Contains`. This method checks if the specified character exists within the string and returns a boolean value: `True` if it exists and `False` if it does not.
The syntax is:
String.Contains(Char)
Example
Here’s how to use the `Contains` method to check for the presence of the letter "P":
$text = "PowerShell"
$exists = $text.Contains("P")
Write-Host "Is 'P' present in the string? $exists"
The resulting output will be:
Is 'P' present in the string? True
This boolean response is helpful for control structures, where you might want different actions based on whether a specific character exists.

Case Sensitivity in Character Searches
Understanding Case Sensitivity
It's essential to consider that character searches in PowerShell are case-sensitive by default. This means that `"P"` is different from `"p"`. A search for the lowercase "p" in the string "PowerShell" will yield different results than a search for the uppercase "P".
To illustrate:
$text = "PowerShell"
$positionLower = $text.IndexOf("p") # Should return -1
$positionUpper = $text.IndexOf("P") # Should return 0
In this example, the first command returns `-1` because "p" does not appear in "PowerShell," while the second successfully finds "P" at position 0.

Finding All Instances of a Character in a String
Using a Loop
If you want to find all instances of a character in a string, you can use a `for` loop to iterate through each character of the string. This approach allows you to check every position systematically.
Here’s a code example that finds all occurrences of the letter "e":
$text = "PowerShell"
for ($i = 0; $i -lt $text.Length; $i++) {
if ($text[$i] -eq "e") {
Write-Host "Found 'e' at position: $i"
}
}
This code will output each position where the character "e" appears within the string. Loops are particularly useful for more complex string analyses.
Using Regular Expressions
Regular expressions (regex) are another powerful tool for finding characters in strings. They allow you to define complex search patterns and are particularly handy for checking the presence of characters.
To use regex to find a character, you can use the `-match` operator:
$text = "PowerShell"
if ($text -match "e") {
Write-Host "'e' found in the string."
}
This example checks for the character "e" and will output the message if found.

Practical Applications of Finding Characters in Strings
Data Validation
Finding characters in strings is vital for data validation—ensuring that input adheres to certain formats. For instance, checking whether user input contains valid characters can help prevent errors and ensure data integrity.
File Parsing and Data Extraction
Searching for specific characters becomes crucial when parsing files, especially when you need to extract meaningful data. For example, if you’re reading CSV files, you may need to find delimiters or specific indicators within the data.
String Manipulation in Automated Scripts
In automated scripts, being able to quickly find and manipulate characters can significantly enhance task efficiency. For instance, examining log files for specific error codes can help automate responses to issues.

Troubleshooting Common Issues
Index Out of Range Errors
When using character indices, be aware of potential Index Out of Range errors. These occur if you attempt to access a position that doesn’t exist within the string. Always ensure that you provide a valid index based on the string's length.
Performance Considerations
Choosing between simple searches (like `IndexOf` and `Contains`) versus regex can impact performance. For basic searches, the simpler methods are often faster and easier to read. Use regex methods when dealing with complex search requirements.
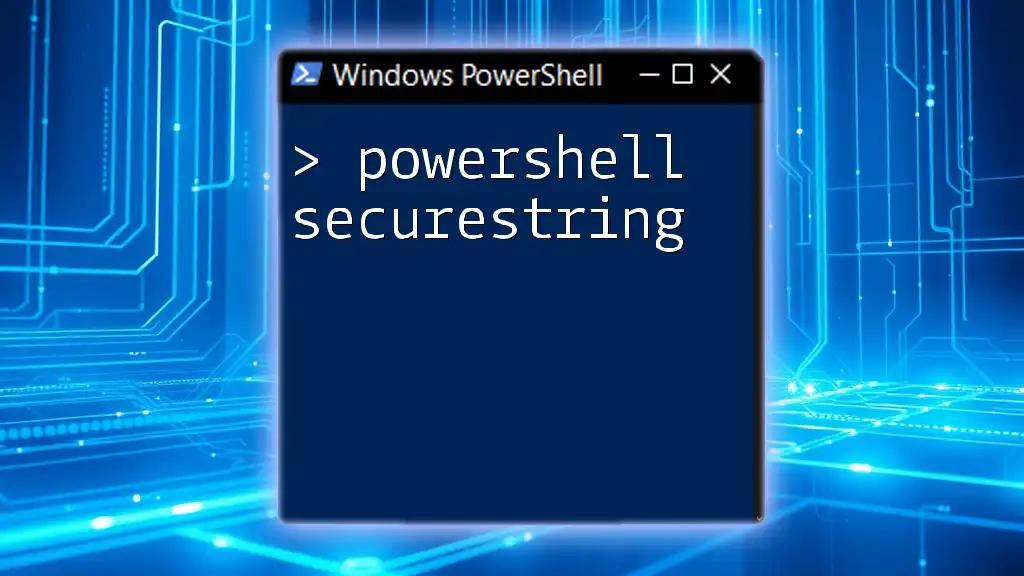
Conclusion
Finding characters in strings is an essential skill in PowerShell that can streamline your scripting process. By utilizing the various methods discussed—such as `IndexOf`, `Contains`, loops, and regex—you empower yourself to handle text efficiently and effectively. Practice these techniques, and watch how they enhance your PowerShell capabilities!
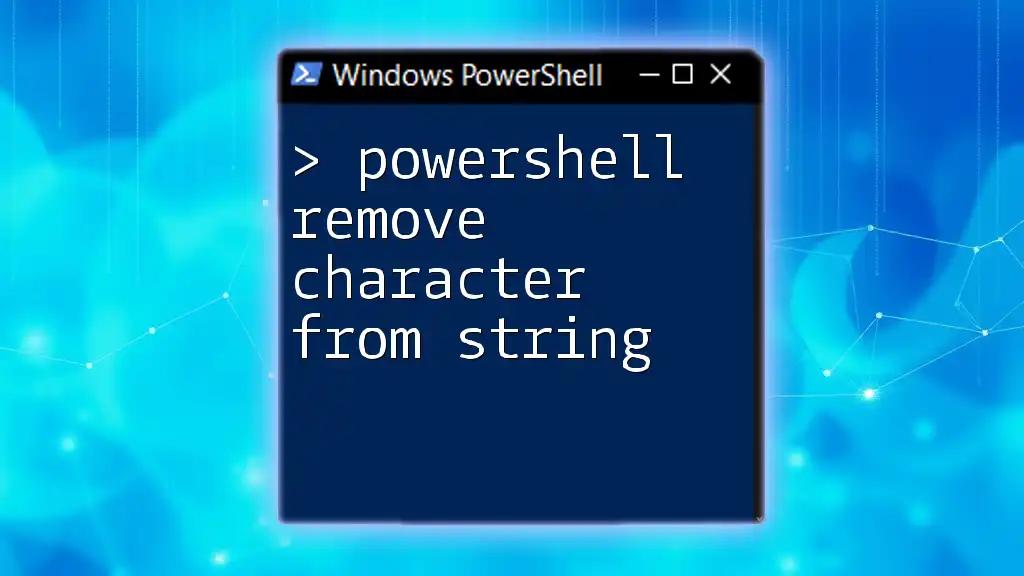
Call to Action
If you found this guide helpful, consider following us for more tips and tutorials on PowerShell. Subscribe for updates on upcoming articles and courses designed to elevate your scripting skills!