In PowerShell, you can easily retrieve the first character of a string by using indexing, as shown in the following code snippet:
$string = "Hello, World!"
$firstCharacter = $string[0]
Write-Host $firstCharacter
Understanding Strings in PowerShell
What is a String?
In programming, a string is a sequence of characters used to represent text. In PowerShell, strings are fundamental, utilized in various scenarios such as defining parameters, storing user input, and manipulating data. Understanding how to work with strings is crucial for effective scripting.
How Strings are Represented
Strings in PowerShell can be defined using single or double quotes.
- Single quotes (`'`) treat everything inside them as a literal string, making no substitutions.
- Double quotes (`"`) allow for variable expansion and string interpolation, making them useful for dynamic content.
For example:
$singleQuoteString = 'This is a string with a single quote!'
$doubleQuoteString = "This string contains a variable: $anotherString"
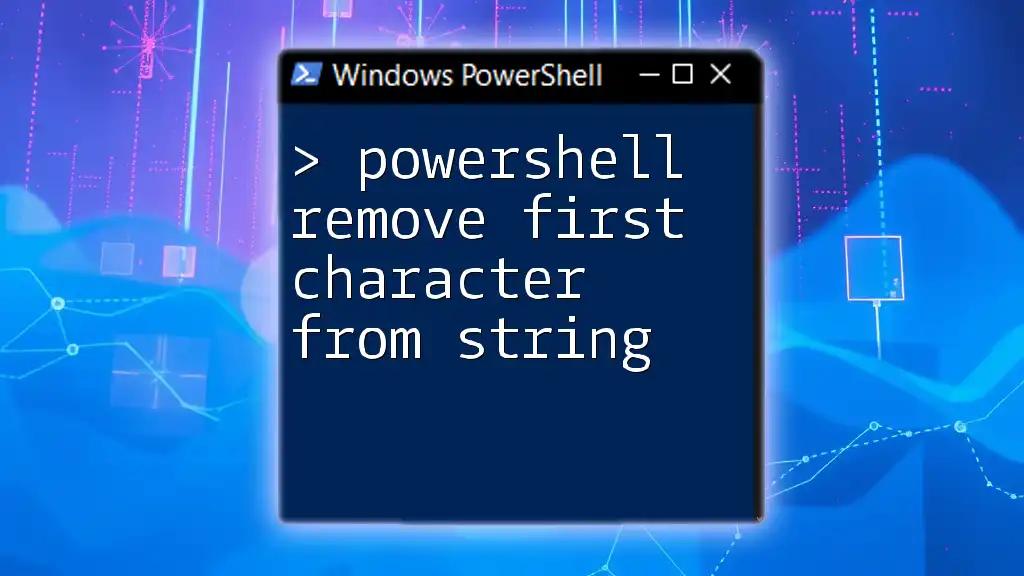
Accessing the First Character of a String
Indexing in PowerShell
Strings in PowerShell are zero-indexed, meaning the first character of any string can be accessed with an index value of `0`. Thus, to extract the first character of a string, you simply refer to the index position.
Basic Syntax for Extracting the First Character
Suppose we declare a string:
$myString = "Hello"
You can easily access the first character by using the index notation:
$firstChar = $myString[0]
Code Explanation
In the above code snippet, `$myString` is the variable that holds the string "Hello." By using `$myString[0]`, we retrieve the character at index `0`, which is `H`. This method is straightforward and provides a quick way to obtain the first character of any string.
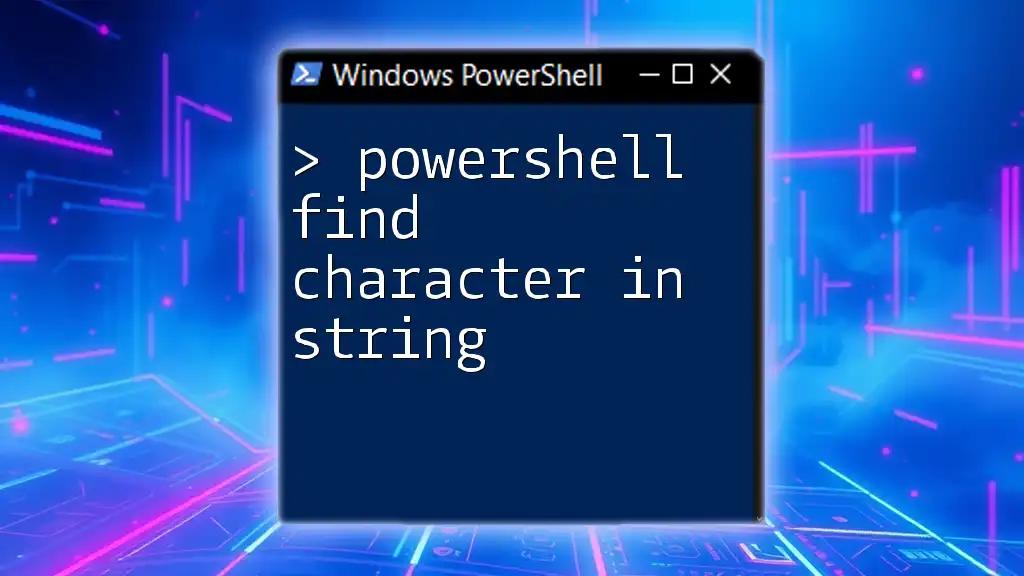
Advanced Methods to Get the First Character of a String
Using the Substring Method
Another effective way to retrieve the first character is through the `Substring` method. This method is versatile and can specify a starting index and the number of characters to extract.
For instance:
$firstChar = $myString.Substring(0, 1)
Here, `Substring(0, 1)` tells PowerShell to start at index `0` and return `1` character, which will also yield `H`.
Advantages and Use Cases of Substring
Using the `Substring` method can be beneficial when you're dealing with variable-length strings or when you need more control over string manipulation. For example, it allows for more complex substring extraction beyond just the first character.
Using the Split Method
Aside from indexing and substring methods, the `Split` method can be employed to extract the first character, though it’s less common. The `Split` method divides a string into an array based on a defined delimiter.
For example:
$firstChar = $myString.Split('')[0]
This method might seem a bit convoluted for simply getting the first character, but it showcases flexibility in handling string-to-array conversions.
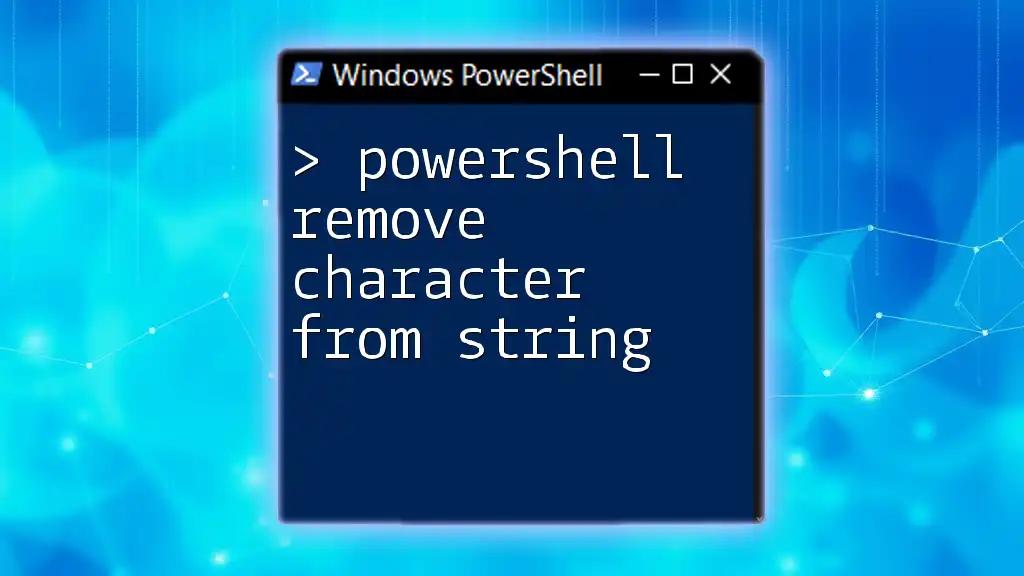
Practical Examples
Extracting the First Character from User Input
Handling user input is an essential part of scripting. You can prompt the user and extract the first character from their response easily. Here’s a simple example:
$userInput = Read-Host "Enter a string"
$firstChar = $userInput[0]
Write-Output "The first character is: $firstChar"
This snippet prompts the user for input and displays the first character of the entered string. If the input is empty, accessing `$userInput[0]` directly may cause an error, which leads us to our next section.
Working with Arrays of Strings
You might often find the need to process multiple strings simultaneously. Here’s how to extract the first character from an array of strings:
$stringsArray = @("Apple", "Banana", "Cherry")
foreach ($string in $stringsArray) {
$firstChar = $string[0]
Write-Output "The first character of $string is: $firstChar"
}
This code iterates over each string in the array and retrieves its first character using simple indexing.
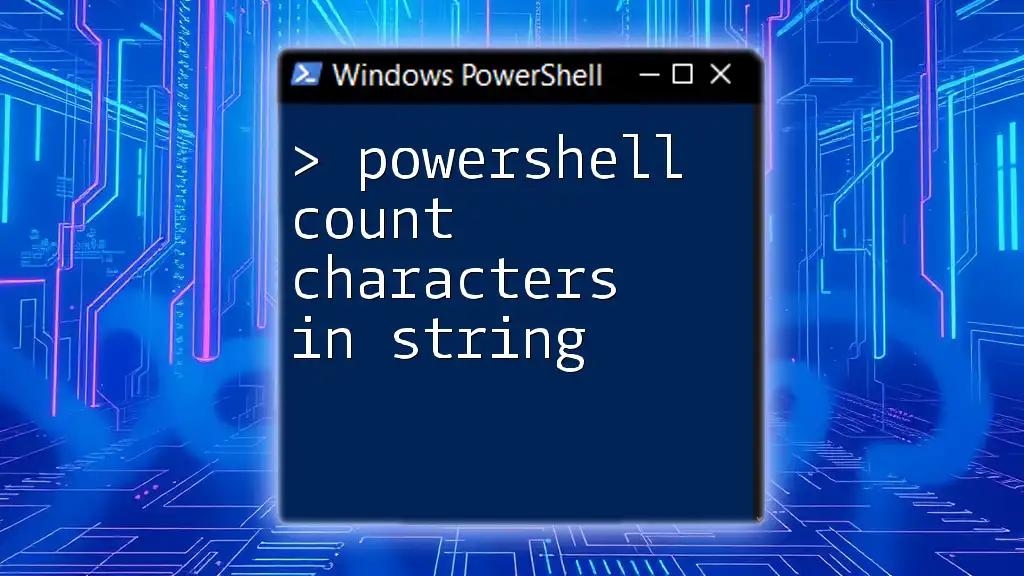
Common Errors and Troubleshooting
Accessing Out of Bound Index
One common issue arises from attempting to access an index that does not exist. For instance:
$emptyString = ""
$firstChar = $emptyString[0] # This will throw an error
This would throw an `IndexOutOfRangeException`, notifying you that the index is out of bounds.
Handling Empty Strings Gracefully
To avoid errors, it’s wise to implement a check to handle empty strings gracefully:
if ($string.Length -gt 0) {
$firstChar = $string[0]
} else {
Write-Output "String is empty!"
}
This code ensures that the script checks whether the string has any characters before attempting to retrieve the first one.

Conclusion
In this guide, we've covered multiple methods to get the first character of a string in PowerShell, emphasizing both basic and advanced strategies. From using direct indexing to employing the `Substring` and `Split` methods, mastering these techniques will enhance your scripting efficiency. I encourage you to practice these methods and explore string manipulation further. Feel free to share your experiences and challenges in the comments!

Additional Resources
For those eager to deepen their understanding of string manipulation in PowerShell, consider exploring additional tutorials and courses related to PowerShell strings, where you'll uncover more advanced techniques and scenarios.