To retrieve the thumbprint of a certificate in PowerShell, you can use the following command:
Get-ChildItem -Path Cert:\LocalMachine\My | Select-Object -Property Thumbprint
Understanding Certificate Thumbprints
What is a Certificate Thumbprint?
A certificate thumbprint, also known as a fingerprint, is a unique identifier for a digital certificate. It is generated by applying a hash algorithm, typically SHA-1 or SHA-256, to the certificate data. This hash value is a fixed-size string, regardless of the size of the data it represents, making it a concise way to identify certificates securely.
Importance of Using Thumbprints
Using thumbprints is crucial for certificate validation. They help to ensure that the certificate in question has not been altered or tampered with. When managing security operations and automations, such as deploying SSL certificates or verifying server identities, relying on the thumbprint ensures you have the correct and validated certificate.
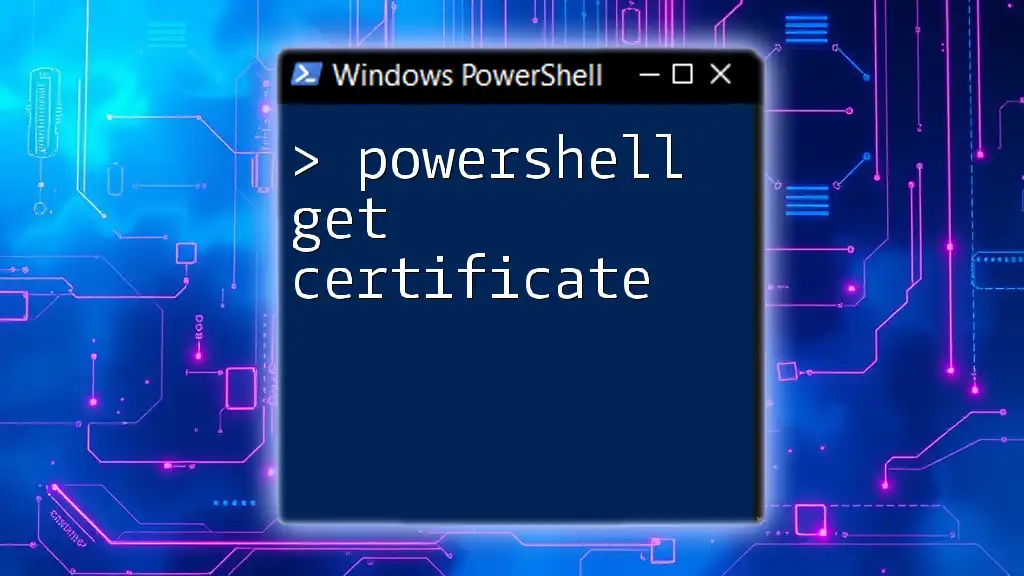
Getting Started with PowerShell for Certificates
Prerequisites
Before diving into PowerShell commands for managing certificates, ensure you have:
- PowerShell version 5.0 or higher, as it provides enhanced cmdlet capabilities.
- A basic understanding of how to run PowerShell commands on your system.
Accessing the Certificate Store
Certificates are stored in the Windows Certificate Store, which can be accessed through PowerShell. Certificates are organized into stores such as LocalMachine and CurrentUser. To explore these stores, you would typically use the `Cert:` PSDrive.
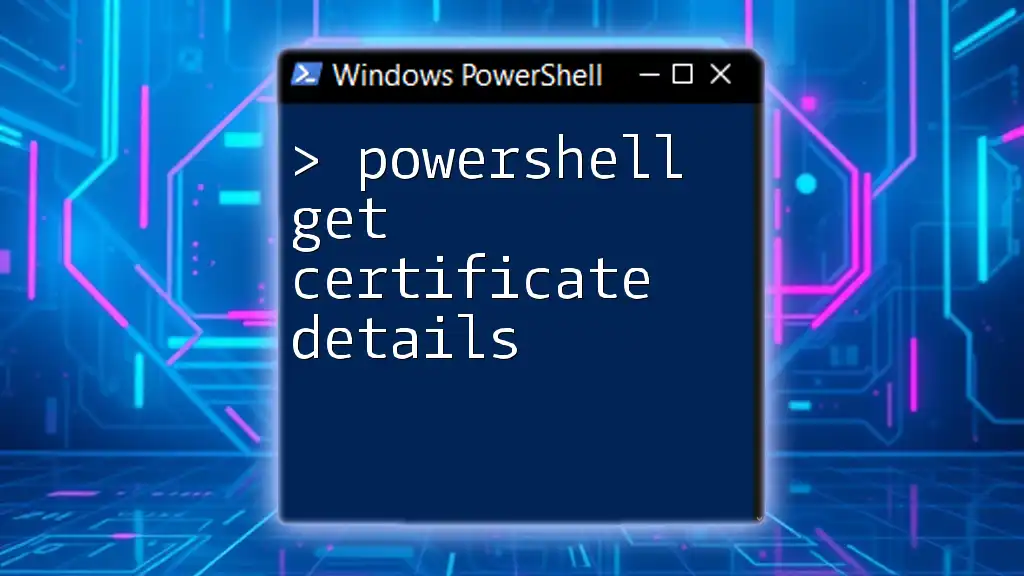
PowerShell Get Certificate Thumbprint
Basic Command Structure
To get the thumbprints from a specific certificate store, you can use:
Get-ChildItem -Path Cert:\<StoreLocation> | Select-Object Thumbprint
Replace `<StoreLocation>` with your desired certificate store, like `LocalMachine\My`. This command retrieves all certificates in the specified store and selects their thumbprints.
Finding Certificate Thumbprint
To get more granular, you can find thumbprints for specific certificates. For instance:
Get-ChildItem Cert:\LocalMachine\My | Select-Object Subject, Thumbprint
This command lists each certificate's Subject alongside its Thumbprint. The output will help you identify which thumbprint corresponds to which certificate, allowing for easier management.
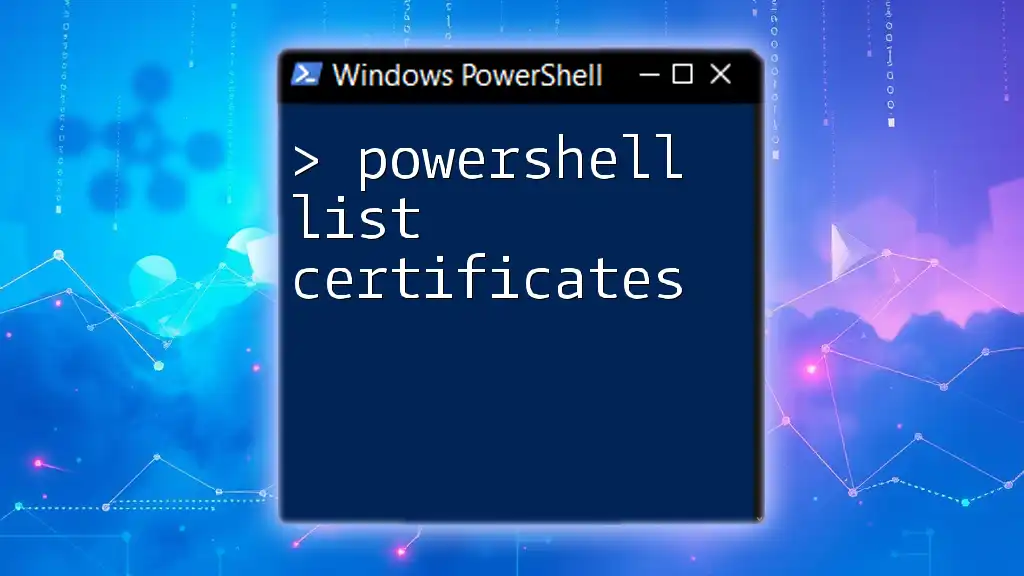
PowerShell Get Cert by Thumbprint
Using Thumbprint to Retrieve Certificate
To retrieve a specific certificate using its thumbprint, you can craft a PowerShell command similar to this:
$thumbprint = "YOUR_THUMBPRINT"
Get-ChildItem Cert:\LocalMachine\My | Where-Object { $_.Thumbprint -eq $thumbprint }
In this command:
- Replace `YOUR_THUMBPRINT` with the actual thumbprint you want to find.
- This filters the certificates in `LocalMachine\My`, returning only the one that matches the given thumbprint.
Description of Command Breakdown
- `Get-ChildItem Cert:\LocalMachine\My`: Lists all certificates in the targeted store.
- `Where-Object { $_.Thumbprint -eq $thumbprint }`: Filters the results to find the specific certificate where the thumbprint matches the variable.
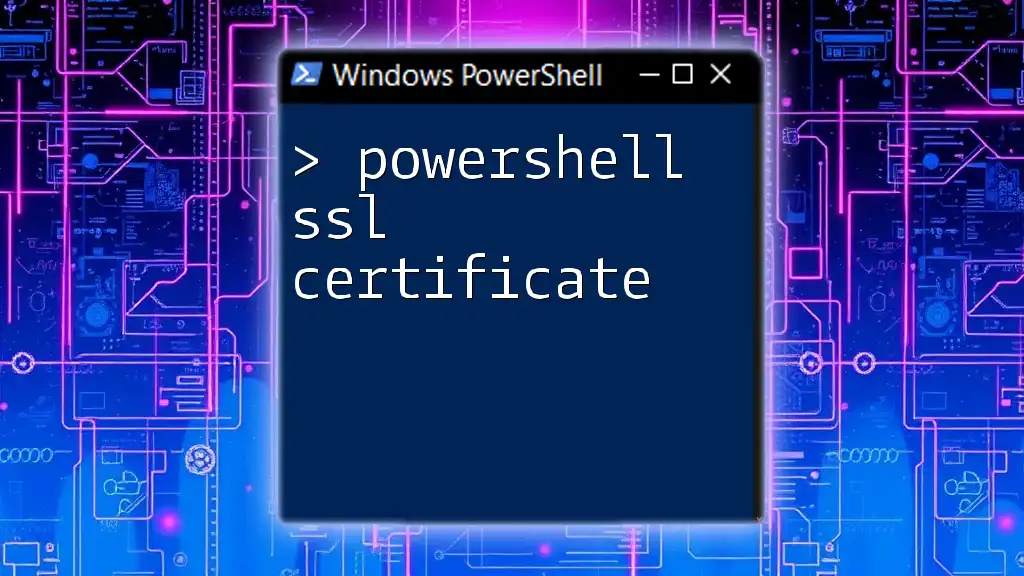
PowerShell Get Certificate by Thumbprint
Detailed Command Examples
You can also directly retrieve a certificate by its thumbprint with a command like this:
Get-Item Cert:\LocalMachine\My\$thumbprint
This command fetches the exact certificate associated with the provided thumbprint. It's a straightforward way to access a certificate without listing all entries first.
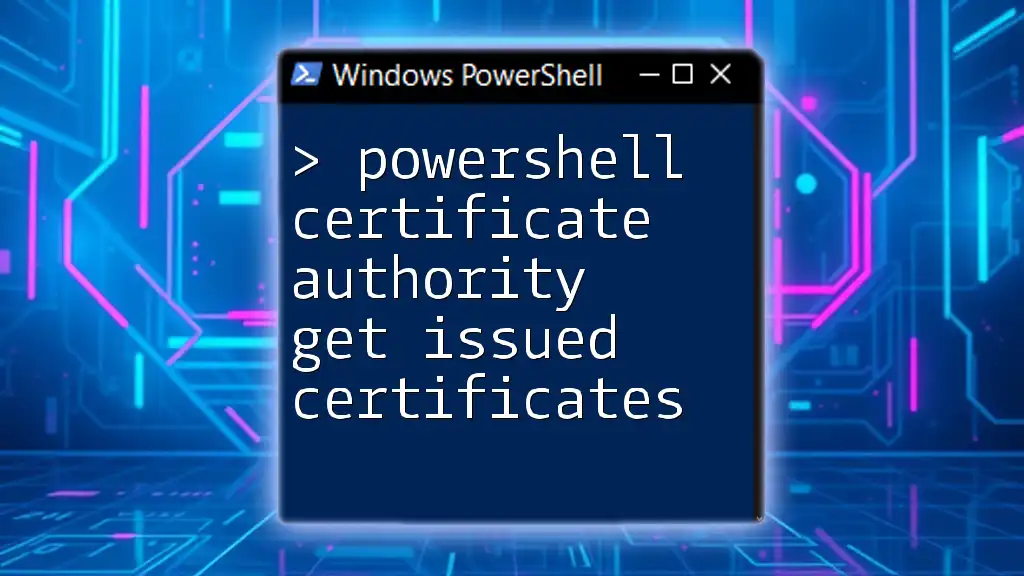
PowerShell Find Certificate by Thumbprint
Searching for Certificates
If you want to dig deeper and search through all certificate stores on your machine for a specific thumbprint, you can use:
Get-ChildItem Cert:\ -Recurse | Where-Object { $_.Thumbprint -eq $thumbprint }
This command:
- Uses `-Recurse` to drill down into every store, which is helpful if you're unsure where the certificate is located.
- Again, filters based on the thumbprint to find an exact match.
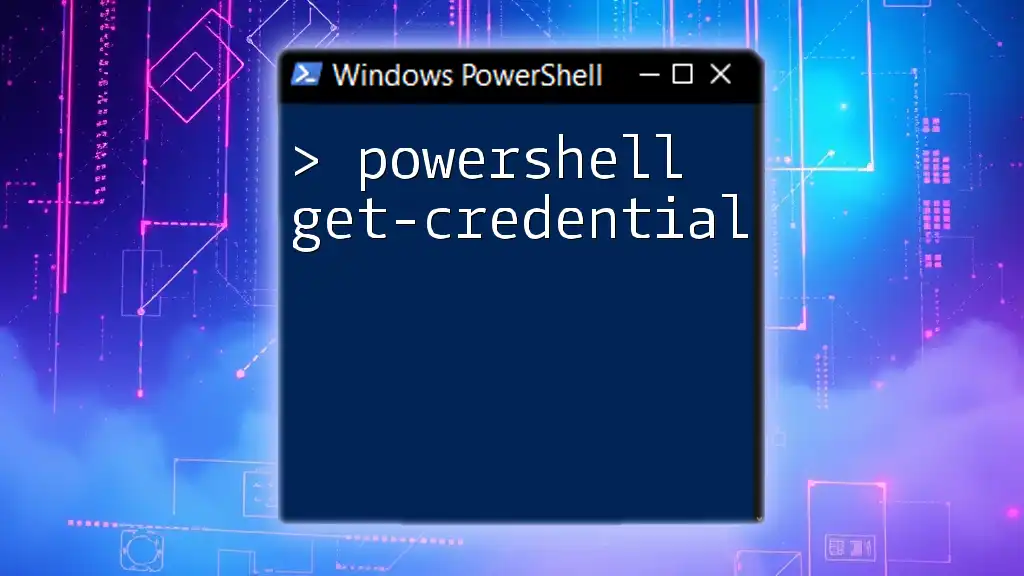
PowerShell Get SSL Certificate Thumbprint
Working with SSL Certificates
SSL certificates are vital in securing web communications. To retrieve the thumbprint for SSL certificates specifically, you might use a command like:
Get-ChildItem Cert:\LocalMachine\My | Where-Object { $_.Issuer -like "*Your Issuer*" }
In this command:
- The `-like` operator allows searching for certificates by the issuer, which is crucial when managing multiple certificates from various sources.
Discussion on the Significance of SSL in Web Security
Understanding SSL certificates is essential as they protect sensitive data in transit. By effectively managing SSL certificate thumbprints through PowerShell, you ensure that your web applications remain secure and trustworthy.
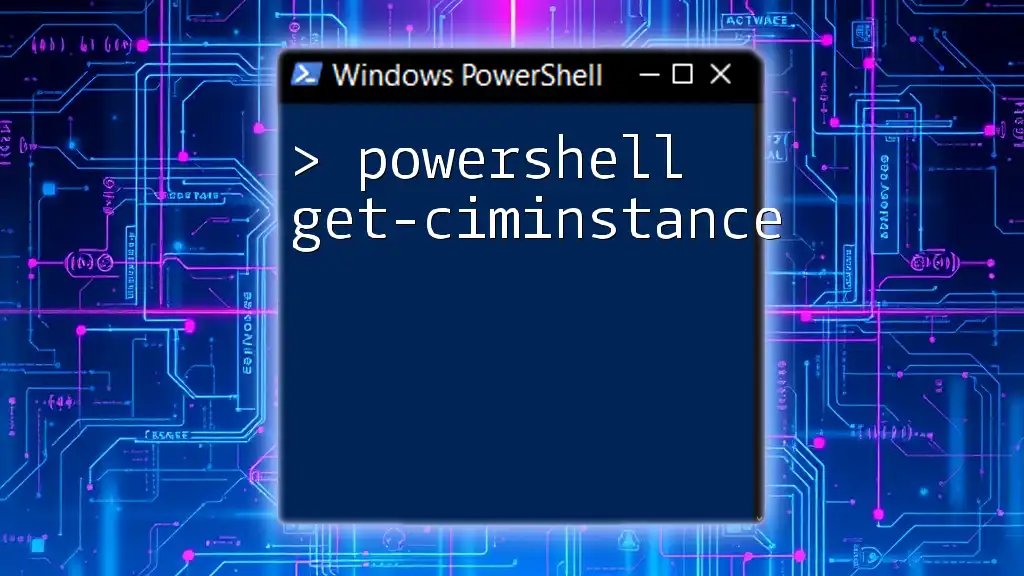
Additional Tips with PowerShell Certificate Management
Best Practices for Managing Certificates
- Regular Monitoring: Keep an eye on certificate expiration dates and renew them proactively.
- Automation: Use scheduled PowerShell scripts to check for expired certificates or to generate reports on certificate statuses.
Troubleshooting Common Issues
Some common errors when attempting to retrieve certificates may include insufficient permissions or incorrect thumbprints. Always verify your access rights to the certificate store and ensure that your thumbprint is accurate.
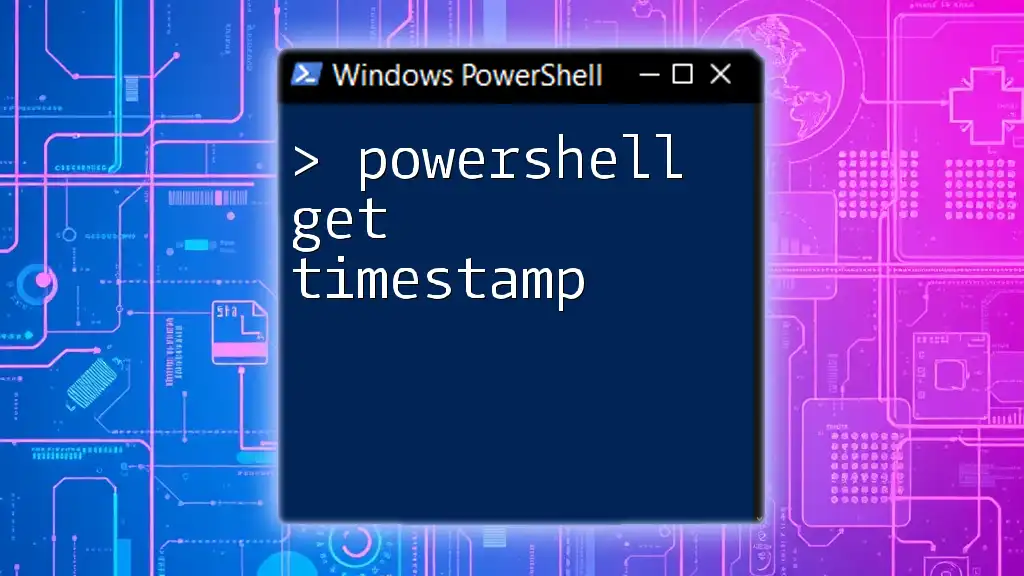
Conclusion
In managing certificates through PowerShell, understanding the concept of certificate thumbprints is vital. They serve as a reliable method for validating and identifying certificates, ensuring the integrity of your security measures. By utilizing the commands highlighted in this guide, you can streamline your certificate management processes and enhance your overall security posture.
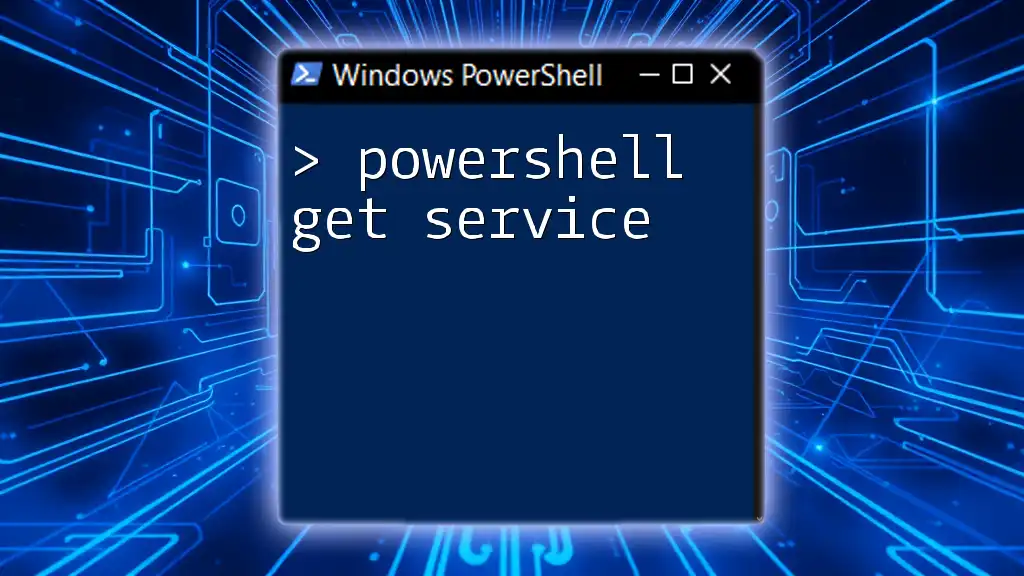
Resources
For further reading on PowerShell and certificates, visit the official Microsoft documentation. Explore additional tools and community scripts to enhance your certificate management skills, ensuring you can effectively navigate PowerShell's powerful capabilities.