In PowerShell, you can truncate a string by specifying the desired length with the `Substring` method, as shown in the following code snippet:
$originalString = "Hello, World!"
$truncatedString = $originalString.Substring(0, 5) # Result: "Hello"
Write-Host $truncatedString
Understanding Strings in PowerShell
What is a String?
In PowerShell, a string is a sequence of characters used to represent text. Strings are one of the most commonly used data types, allowing you to store and manipulate text data efficiently. They can include letters, numbers, symbols, and even whitespace.
Example of string data types in PowerShell:
$string1 = "Hello, World!"
$string2 = 'PowerShell is powerful.'
$string3 = "12345" # This is also a string
The Need for String Manipulation
String manipulation is essential in various scenarios, such as formatting outputs, processing data, and creating reports. More specifically, string truncation allows you to shorten a text string to fit within defined boundaries. It's particularly useful in situations where text length may exceed display limits or where concise information is preferred.

Truncating Strings in PowerShell
Basic String Truncation
Truncating a string in PowerShell can be easily achieved using the `Substring` method, which allows you to extract a portion of the string. This method takes two parameters: the starting index and the length of characters to include.
Example: Truncating to a specific length:
$string = "Hello, PowerShell!"
$maxLength = 10
$truncatedString = $string.Substring(0, $maxLength)
Write-Output $truncatedString # Output: Hello, Pow
Explanation
In this example, the `Substring` method extracts the first ten characters from the original string, giving you "Hello, Pow". Remember, string indexing in PowerShell is zero-based, meaning that the first character of a string has an index of 0.
Conditional String Truncation
Sometimes, you only want to truncate a string if it exceeds a certain length. This can be handled using a simple conditional function.
Example: Truncate only if the string exceeds a specified length:
function Truncate-String {
param (
[string]$inputString,
[int]$maxLength
)
if ($inputString.Length -gt $maxLength) {
return $inputString.Substring(0, $maxLength)
}
return $inputString
}
$input = "Learning PowerShell is fun!"
$truncated = Truncate-String -inputString $input -maxLength 15
Write-Output $truncated # Output: Learning Power
Explanation
Here, the `Truncate-String` function checks if the input string’s length exceeds the specified maximum length. If it does, the function truncates the string and returns it. If not, it returns the original string. Using functions promotes reusability and cleaner code, making your scripts easier to maintain.
Using Regular Expressions for Custom Truncation
For more advanced string manipulations, you can utilize Regular Expressions (regex). This approach allows you to truncate strings based on specific patterns.
Example: Truncate based on a pattern:
$string = "This is a color: Blue"
$truncatedString = $string -replace "color: .*$", "color: ..."
Write-Output $truncatedString # Output: This is a color: ...
Explanation
In this example, the `-replace` operator modifies the string by using a regex pattern that identifies everything from "color: " to the end of the string. The matched portion is replaced with "color: ...", effectively truncating anything that follows. This method provides flexibility for complex truncation needs.
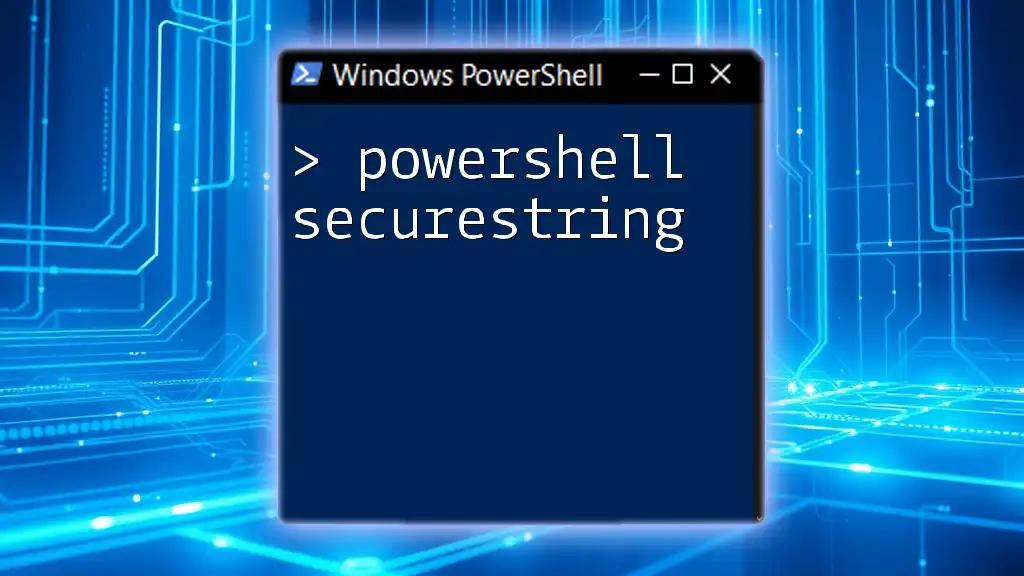
Practical Use Cases for String Truncation
Shortening File Names
In many situations, file names may be too lengthy and exceed designated character limits. Truncating these file names can enhance usability and clarity.
Example: Truncating long file names:
$fileName = "This is a very long file name that exceeds the limit.txt"
$truncatedFileName = Truncate-String -inputString $fileName -maxLength 30
Write-Output $truncatedFileName # Output: This is a very long fil...
Formatting Command Outputs
When generating outputs, especially in scripts that process or display data, it is crucial to ensure that the output remains readable and manageable.
Example: Truncating output data in a formatted table:
$data = @("John Doe", "Jane Smith", "Alexander Hamilton")
foreach ($name in $data) {
$output = Truncate-String -inputString $name -maxLength 10
Write-Output $output
}
Explanation
By truncating the names in the output, you create a more organized display that is easier to read while avoiding unnecessary clutter. String truncation plays a significant role in maintaining clean and intelligible outputs.
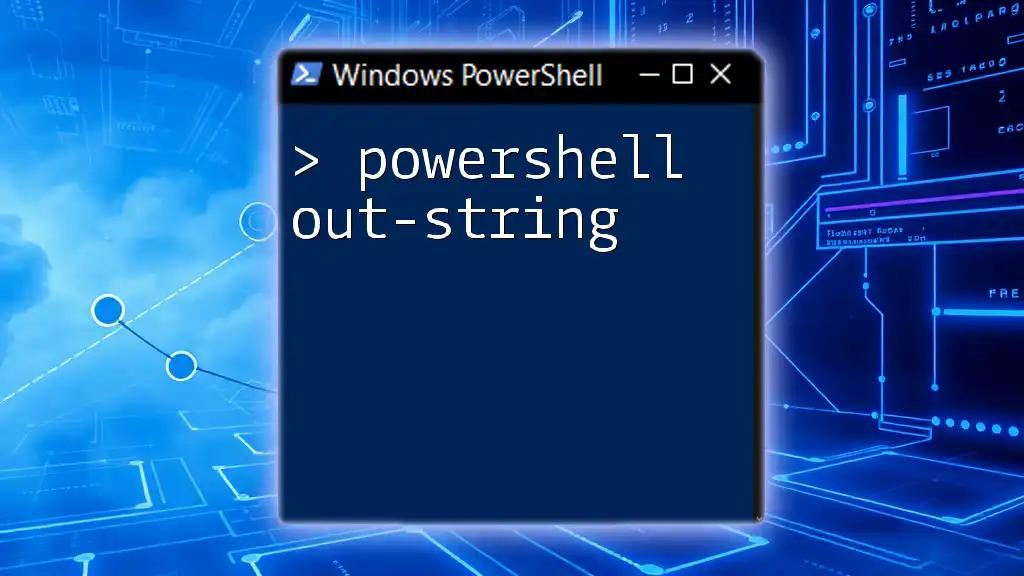
Best Practices for Truncating Strings
Performance Considerations
When manipulating strings, especially in extensive loops or large datasets, efficiency becomes crucial. Avoid unnecessary calls to string methods by caching results when possible. This will optimize performance and minimize processing time.
Readability and Maintenance
Strive for clear, understandable code by structuring your functions well and following consistent naming conventions. Commenting your code can also significantly enhance its readability, especially for others (or for yourself in the future) who may work on the script later.

Conclusion
In conclusion, understanding how to truncate strings in PowerShell effectively empowers you to handle string manipulation in various scenarios. Mastering this technique opens up a world of possibilities for formatting outputs, processing data, and managing information efficiently. As you continue your exploration of PowerShell, consider diving deeper into other string manipulation techniques to further enhance your scripting capabilities.

Additional Resources
For more comprehensive knowledge, refer to the official PowerShell documentation, recommended readings on string manipulation, or community forums where you can connect with fellow PowerShell enthusiasts.