The `Select-String -Context` command in PowerShell allows you to search for a specific pattern in text while also displaying specified lines of context before and after each match, enhancing your ability to understand the context of the found strings.
Select-String -Path "C:\example\file.txt" -Pattern "error" -Context 2,3
Understanding `Select-String`
What is `Select-String`?
`Select-String` is a powerful PowerShell cmdlet that serves as a text searching tool within files. It enables users to search for specific patterns or strings in text files and is similar to the Unix command `grep`. This cmdlet is particularly useful for system administrators, developers, and anyone who regularly works with log files or text documents, as it helps identify relevant information quickly.
Syntax of `Select-String`
The basic syntax for `Select-String` is structured as follows:
Select-String -Path <string[]> -Pattern <string> [-CaseSensitive] [-Context <int>,<int>]
- -Path: Specifies the location of the file(s) to search.
- -Pattern: Defines the string or regular expression to search for.
- -CaseSensitive: (optional) Makes the search case-sensitive.
- -Context: (optional) Specifies the number of lines to show before and after the matching line.
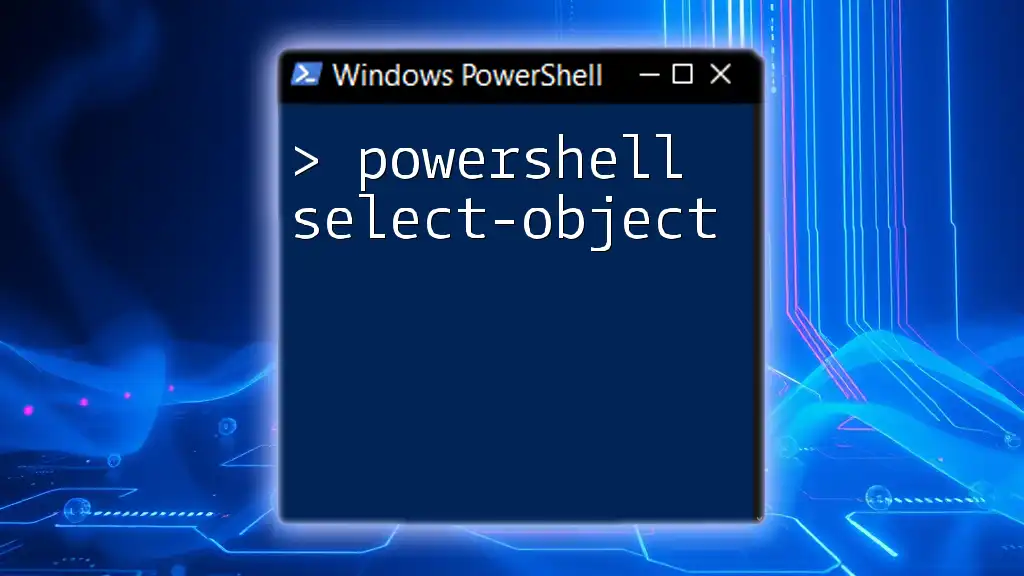
The `-Context` Parameter
Overview of the `-Context` Parameter
The `-Context` parameter enhances the utility of `Select-String` by allowing users to view surrounding lines in addition to the matched pattern, providing greater context and insight. This is incredibly useful when analyzing log files where the critical error or warning may have preceding or subsequent lines that offer more detailed information about the situation.
Syntax for `-Context`
When using the `-Context` parameter, the syntax follows this pattern:
-Context <LinesBefore>, <LinesAfter>
For example, using `-Context 2, 2` means you will see two lines before and two lines after the matching pattern.
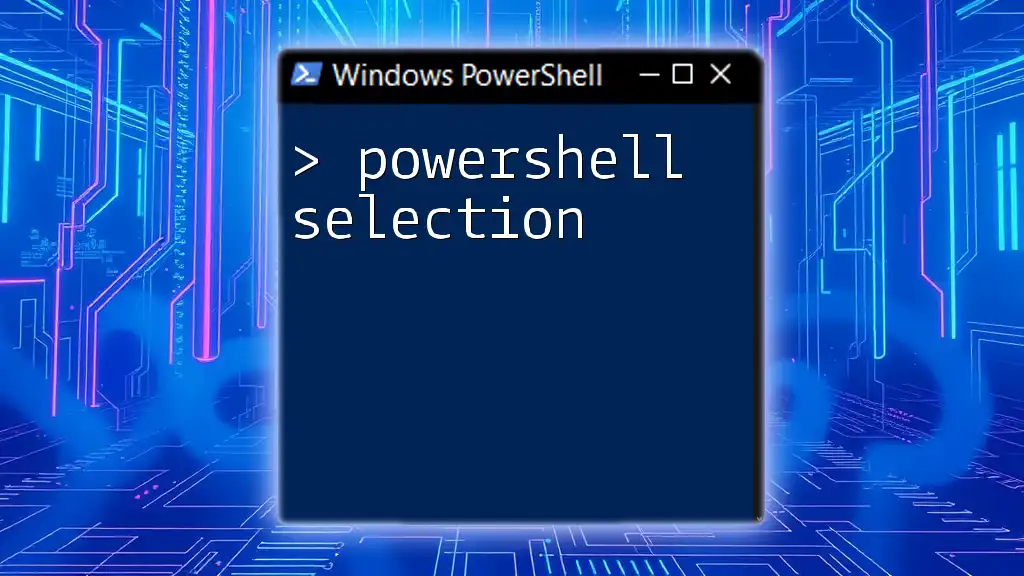
Practical Examples of `Select-String -Context`
Example 1: Basic Usage of `-Context`
In this example, we will search for the term "Error" in a log file and view two lines before and after each occurrence.
Select-String -Path "C:\Logs\example.log" -Pattern "Error" -Context 2, 2
When executed, this command will return all instances where "Error" appears in `example.log`, along with the two lines immediately preceding and succeeding each match. This context can shine a light on what led to the error.
Example 2: Searching Multiple Files
If you want to check for warnings across multiple log files, you can expand your search using wildcards. Here's how you might do that:
Select-String -Path "C:\Logs\*.log" -Pattern "Warning" -Context 3, 1
This command searches all `.log` files in the specified directory for the pattern "Warning." The result includes three lines before each match and one line after, aiding in a broader understanding of each occurrence within context. This is particularly useful for diagnosing recurring issues within a series of logs.
Example 3: Combining with Other Parameters
You can refine your search further by combining the `-Context` parameter with the `-CaseSensitive` switch. For instance:
Select-String -Path "C:\Logs\example.log" -Pattern "failed" -Context 1, 1 -CaseSensitive
In this case, the search is not only looking for "failed" in a case-sensitive manner but also shows one line before and one after the match. This command helps identify precise instances relevant to cases that might affect system performance or failure.
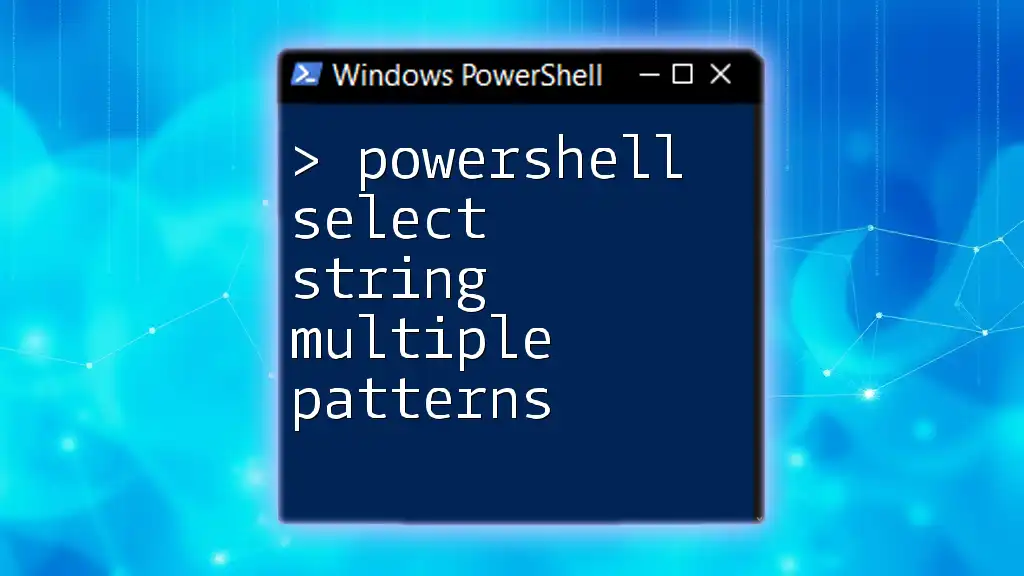
Advanced Uses of `Select-String -Context`
Using Regular Expressions with `-Context`
The flexibility of `Select-String` grows exponentially when you incorporate regular expressions. You can search for complex patterns while still gaining context. For example, searching for both "Exception" and "Error" using the following command:
Select-String -Path "C:\Logs\example.log" -Pattern "Exception|Error" -Context 2, 2
This command will return lines containing either "Exception" or "Error," along with the specified context, enabling you to analyze multiple potential issues simultaneously.
Context in Pipelines
You can also integrate `Select-String` with other commands via PowerShell's pipeline feature. Here’s an example, utilizing `Get-Content` to read a file:
Get-Content "C:\Logs\example.log" | Select-String -Pattern "Critical" -Context 2, 2
This command first retrieves the contents of `example.log` and then pipes the output to `Select-String` to search for the term "Critical" with context. This way, you gain flexibility in processing larger text inputs without directly specifying the file in `Select-String`.
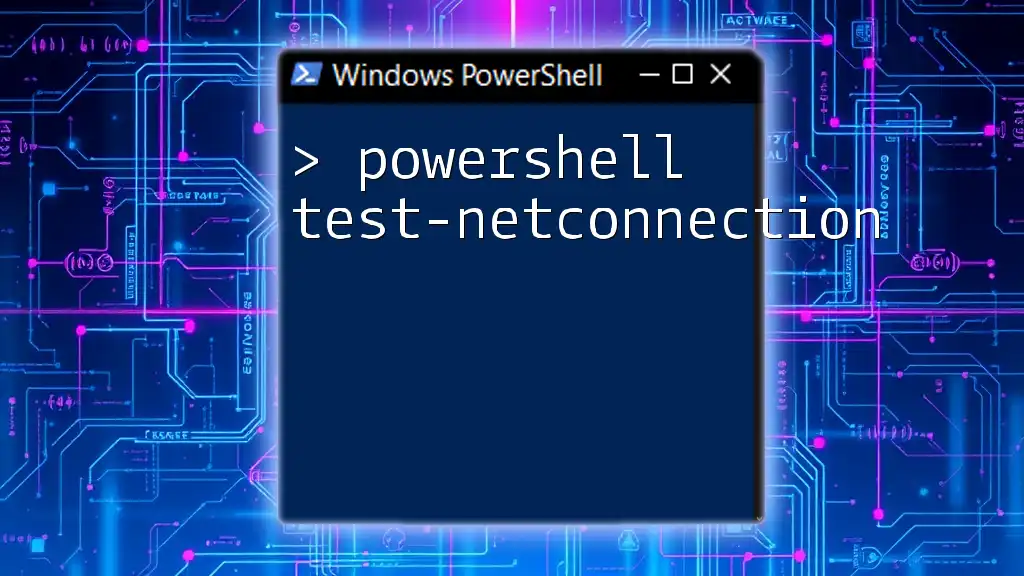
Troubleshooting and Best Practices
Common Errors and Solutions
While `Select-String` is a powerful tool, users may run into common issues like:
- File not found errors: Ensure the file path is correct.
- Pattern not matching: Double-check the pattern and syntax for any typos.
If results aren't as expected, review the parameters and consider testing simpler patterns first to isolate the issue.
Best Practices for Using `Select-String`
When leveraging `Select-String`, consider the following best practices:
- Be specific with your patterns: The more precise your search term, the easier it will be to analyze output.
- Leverage context wisely: Choose how much context to view based on your needs to avoid overwhelming amounts of data.
- Practice with regex: Familiarize yourself with regular expressions to enhance your search capabilities.
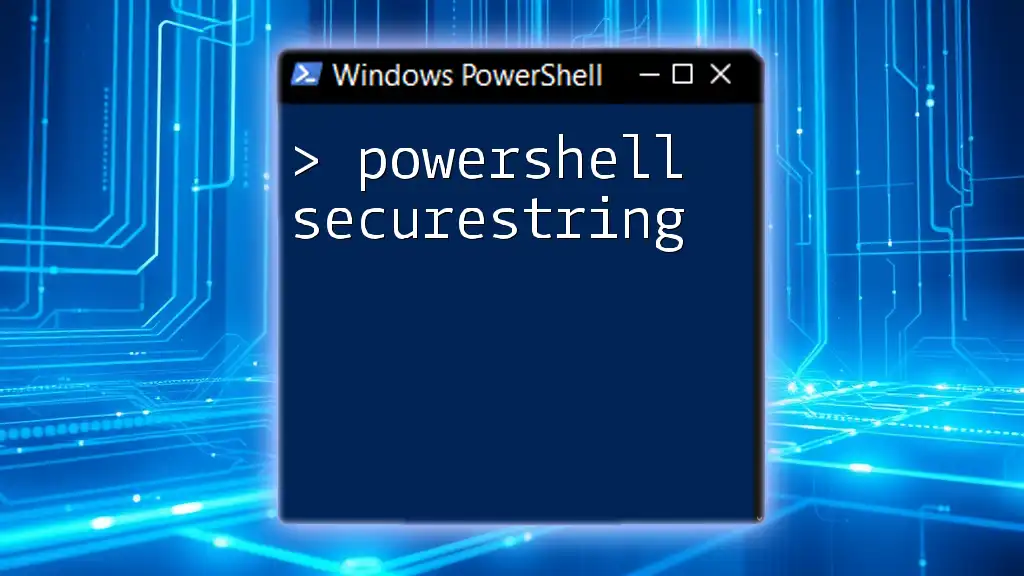
Conclusion
The `Select-String -Context` functionality in PowerShell is an invaluable asset for anyone dealing with text searching, particularly in log file analysis. By enhancing searches with relevant context, users can diagnose issues with greater accuracy and efficiency. By practicing with this cmdlet and exploring its capabilities, users can significantly improve their PowerShell proficiency and troubleshooting skills.
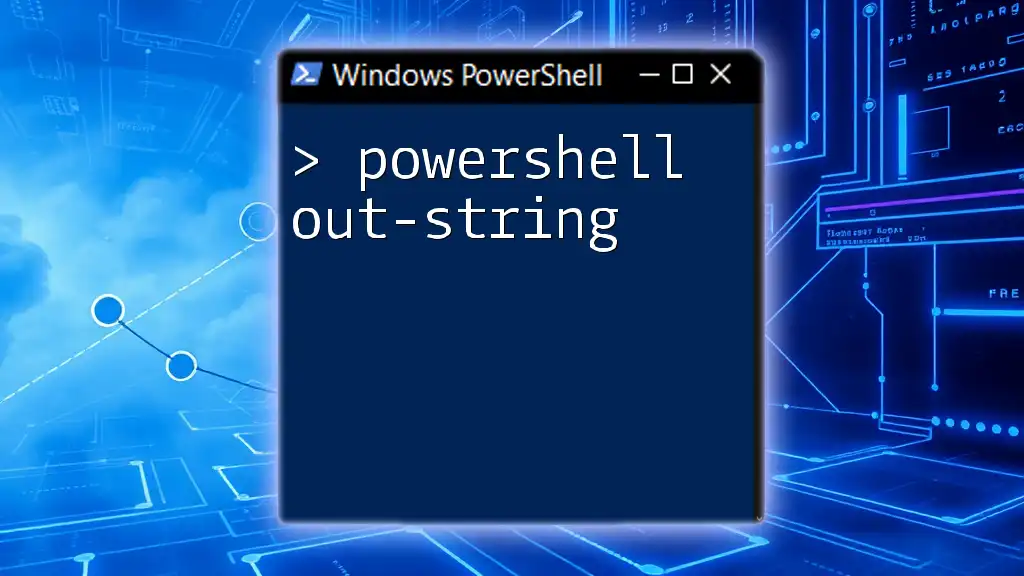
Further Resources
To deepen your understanding of PowerShell, including the `Select-String` cmdlet, you can explore the following resources:
- The official PowerShell documentation.
- Additional reading materials that focus on text processing and command-line tools.
- Recommended PowerShell courses and tutorials for advanced users looking to master their skills.