In PowerShell, the `Substring` method allows you to extract a portion of a string based on specified start index and length, while regular expressions (regex) can be used for more advanced string matching and manipulation.
Here’s a simple example using both:
# Using Substring
$string = "PowerShell is powerful"
$sub = $string.Substring(0, 10)
Write-Host $sub # Output: PowerShell
# Using Regex to match a pattern
if ($string -match '(\w+Shell)') {
Write-Host "Matched: $($matches[0])" # Output: Matched: PowerShell
}
Understanding Substrings in PowerShell
What is a Substring?
A substring is simply a sequence of characters within a string. For instance, in the string `"Hello, PowerShell!"`, the substring `"Power"` is derived from the characters located between specific indices. When working with PowerShell, extracting substrings can be crucial for data manipulation and analysis, especially in scenarios involving file names, user input, or log messages.
How to Extract Substrings in PowerShell
To extract a substring in PowerShell, we can utilize the `.Substring()` method of the string object. This method allows you to specify the starting position and the length of the substring to be captured.
Syntax of `.Substring()`:
$string.Substring(startIndex, length)
- startIndex: The zero-based index from where extraction begins.
- length: The total number of characters to extract.
Example:
$string = "Hello, PowerShell!"
$substring = $string.Substring(7, 11)
Write-Output $substring # Output: PowerShell
In this example, we start at index `7` and take `11` characters, resulting in the substring `PowerShell`.

Introduction to Regex
What is Regex?
Regular Expressions, or Regex, is a powerful tool for defining search patterns in texts. They enable string manipulation by matching, replacing, and splitting strings based on specified criteria, increasing efficiency and reducing the time spent on string handling.
Basic Syntax of Regex
Regex consists of a combination of characters and metacharacters that define a search pattern. For instance:
- `\d` matches any digit (0-9).
- `\w` matches any word character (letters, numbers, underscores).
- `.` matches any character except a newline.
Examples:
- `\d{4}` matches exactly four digits.
- `\w+` matches one or more word characters.

Using Regex in PowerShell
How to Utilize Regex in PowerShell
In PowerShell, Regex can be used with operators such as `-match`, `-replace`, and `-split`.
- `-match`: Performs a regex comparison. If a match is found, it returns `$true`, and the matched result is stored in the automatic `$matches` array.
- `-replace`: Replaces all occurrences of a pattern within a string.
- `-split`: Splits a string into an array based on a regex pattern.
Examples of Regex in Action
Matching Patterns:
$text = "2023-10-05"
if ($text -match '\d{4}-\d{2}-\d{2}') {
Write-Output "Valid date format"
}
In this code snippet, we check if `text` matches the pattern of a date format.
Replacing Content:
$newText = $text -replace '\d{4}', 'Year'
Write-Output $newText # Output: Year-10-05
Here, we demonstrate how to replace four-digit numbers with the word `Year`.
Splitting Strings:
$csv = "one,two,three"
$array = $csv -split ','
This example uses regex to split a CSV string into an array based on the comma delimiter.
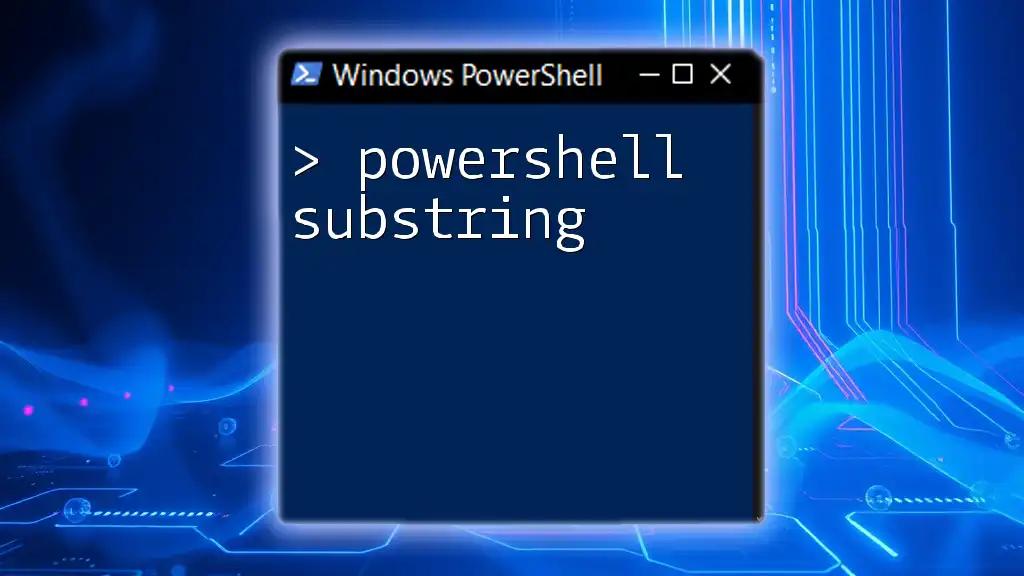
Substring Extraction with Regex
Combining Substrings and Regex
Combining substrings with regex can be very effective for extracting specific patterns from larger strings. When regex is applied, PowerShell can easily retrieve components of the string based on defined patterns.
Example:
$dateString = "Today is 2023-10-05"
if ($dateString -match '(\d{4})-(\d{2})-(\d{2})') {
$year = $matches[1]
Write-Output "Year: $year" # Output: Year: 2023
}
In this example, we capture the year using regex. The matched groups are accessible through the `$matches` array.
Using Named Groups
Named capturing groups in regex enhance readability and maintainability of your code. This feature allows you to retrieve matched components by name rather than by index.
Example:
$string = "Order Number: 12345"
if ($string -match 'Order Number: (?<orderNumber>\d+)') {
$orderNumber = $matches['orderNumber']
Write-Output "Extracted Order Number: $orderNumber" # Output: Extracted Order Number: 12345
}
The named group `orderNumber` makes it clearer and easier to understand which part of the matched string we are referencing.
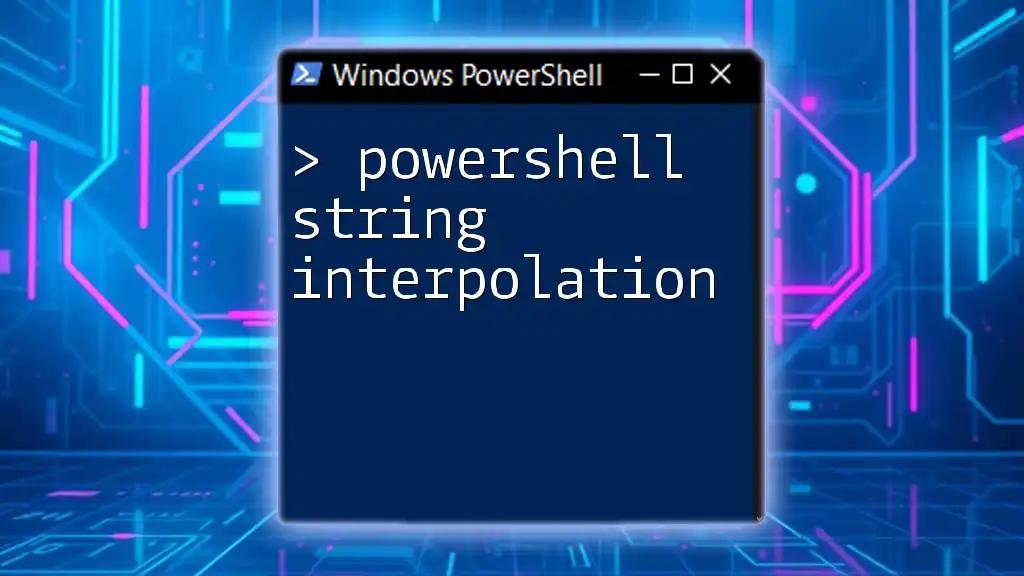
Performance Considerations
Efficiency Tips for Using Regex in PowerShell
While regex is a powerful tool, it can also be resource-intensive, especially for complex patterns or large datasets. Here are some tips to enhance your regex performance:
- Optimize Patterns: Avoid excessive backtracking by using simpler regex expressions when possible.
- Use Anchors: Use `^` for the start and `$` for the end of strings to limit the search scope.
When to Avoid Regex
In certain scenarios, regex may not be the best choice. For small or simple string manipulations, PowerShell’s native string methods, such as `.IndexOf()`, `.Replace()`, or `.Split()`, can often be more efficient and easier to read.

Common Pitfalls
Misunderstanding Regex Syntax
Regex syntax can be complex, with many nuances. A common pitfall is misunderstanding quantifiers (like `*`, `+`, `?`) and grouping mechanisms.
Debugging Regex Issues in PowerShell
When facing issues with regex, testing and debugging become vital. You can use online regex testers, or libraries like `PSScriptAnalyzer`, to validate your patterns before implementation.

Conclusion
Throughout this guide, we've explored the intricate relationship between PowerShell substring and regex, highlighting their combined potential for effective string manipulation. As you delve into your PowerShell scripts, remember that practice is key. Experiment with regex patterns and substring extraction, and don’t hesitate to seek resources that can help deepen your understanding.
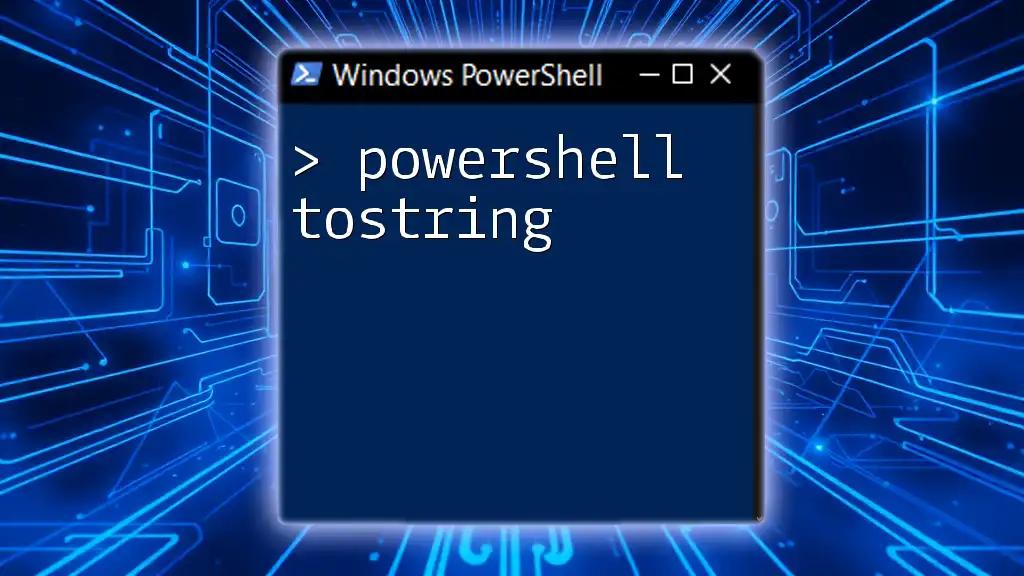
FAQs
What is the difference between `-match` and `-replace`?
The `-match` operator checks if a string matches a regex pattern and can capture matches for further processing. Conversely, the `-replace` operator is used to substitute matched patterns with specified replacement text.
Can I use Regex for JSON parsing in PowerShell?
While it's technically possible to use regex for simple JSON parsing in PowerShell, it’s usually best to utilize the native `ConvertFrom-Json` cmdlet for more complex scenarios, ensuring accuracy and efficiency.
What other languages support Regex?
Many programming languages support regex, including Python, Java, JavaScript, C#, and Ruby. Each language has slight variations in regex syntax and capabilities, so it's beneficial to familiarize yourself with the specifics of the language you are using.