The PowerShell `switch` statement can utilize regular expressions to match input against patterns for more dynamic control flow.
Here's a short code snippet demonstrating how to use `switch` with regex:
$input = "apple"
switch -Regex ($input) {
"a.*" { Write-Host "Starts with 'a'" }
"e.*" { Write-Host "Starts with 'e'" }
".*le$" { Write-Host "Ends with 'le'" }
default { Write-Host "No match found" }
}
Understanding the Basics of PowerShell Switch
What is the Switch Statement?
The `switch` statement in PowerShell is a versatile control flow structure that allows you to evaluate an expression against multiple conditions, resulting in a clean and concise way to manage branching logic. Unlike traditional `if-else` statements that can become cumbersome with many conditions, `switch` simplifies this process by allowing for multiple cases to be handled efficiently.
Syntax of the Switch Statement
The basic syntax of a `switch` statement follows this structure:
switch ($variable) {
"case1" { # action for case1 }
"case2" { # action for case2 }
default { # action if no cases match }
}
This clear layout allows you to specify the variable to evaluate and define actions for various matching cases.
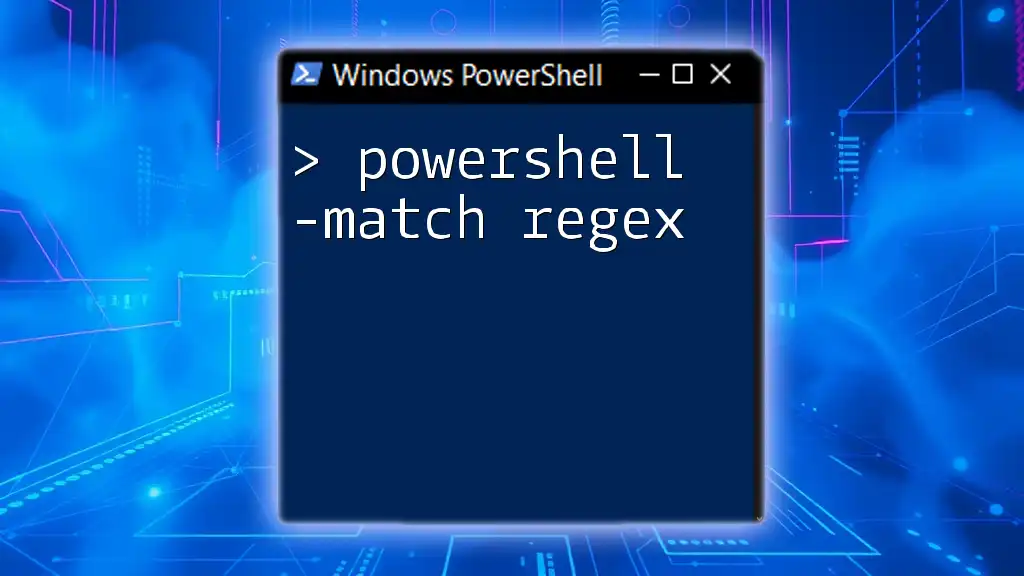
Introduction to Regular Expressions (Regex)
What is Regex?
Regular expressions (regex) are patterns used to match character combinations in strings. They are invaluable for searching, replacing, and validating text. Regex syntax consists of more than just plain text; it includes a set of special characters that give regex its power to define complex searching patterns.
Basic Regex Patterns
To effectively use regex in PowerShell, it’s essential to understand some basic patterns:
- Character Classes (`[abc]`): Matches any character within the brackets.
- Anchors (`^` and `$`): `^` asserts position at the start of a string, while `$` asserts position at the end.
- Quantifiers (`*`, `+`, `?`): Define the number of occurrences of a character or group.
Regex in PowerShell Context
PowerShell integrates regex deeply, allowing you to use it through various cmdlets and operators. For instance, the `-match` operator utilizes regex for pattern matching, and you can use regex with string manipulation methods to extract or replace text.
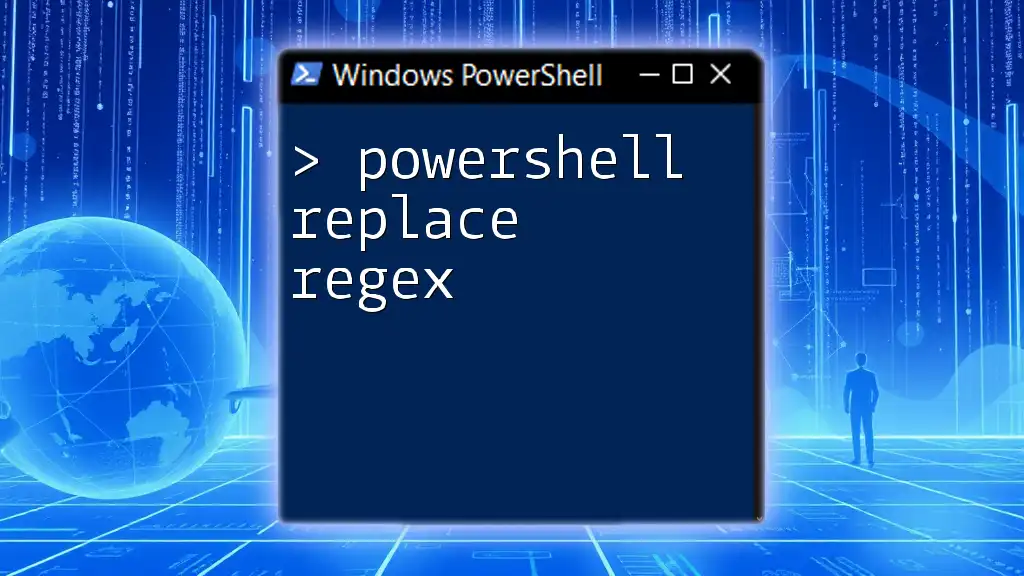
Using Switch with Regex in PowerShell
Why Use Regex with Switch?
Integrating regex with the `switch` statement brings enhanced flexibility, particularly for scenarios involving string pattern matching. By employing regex, you can:
- Handle complex matching scenarios with ease.
- Validate inputs efficiently.
- Improve code readability and maintainability.
The Syntax of Switch with Regex
To employ regex within a `switch` statement, you utilize the `-Regex` parameter. The syntax looks like this:
switch -Regex ($input) {
'pattern1' { # action for pattern1 }
'pattern2' { # action for pattern2 }
default { # action if no pattern matches }
}
In this context, each case is a regex pattern that determines which action to execute based on the input evaluated.
Practical Examples
Example 1: Parsing Strings
Consider a scenario where you want to identify different types of file extensions. You can do this effectively using `switch` with regex. Here’s how:
$files = @("document.pdf", "image.jpg", "script.ps1", "archive.zip")
switch -Regex ($files) {
'\.pdf$' { "This is a PDF file" }
'\.jpg$' { "This is a JPEG image" }
'\.ps1$' { "This is a PowerShell script" }
default { "Unknown file type" }
}
In this example, each case uses a regular expression to match file extensions, demonstrating how `switch` can quickly categorize various file types.
Example 2: User Input Validation
Another great use of regex with `switch` is validating the formats of user input, such as emails. Here's a demonstration:
$emailInputs = @("test@example.com", "not-an-email", "admin@domain.org")
switch -Regex ($emailInputs) {
'^[\w-\.]+@([\w-]+\.)+[\w-]{2,}$' { "Valid Email: $_" }
default { "Invalid Email: $_" }
}
In this case, the regex pattern checks for valid email structures, allowing users to quickly identify which inputs are correct and which are not.
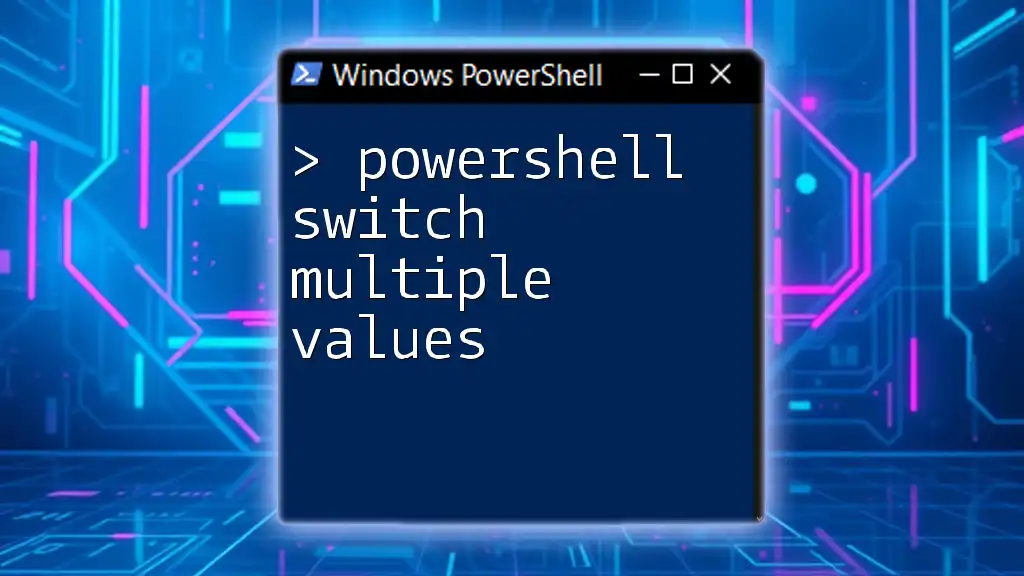
Advanced Techniques with PowerShell Switch and Regex
Combining Conditions
Using the `switch` statement combined with regex means you can easily combine multiple conditions to create complex evaluations. Here’s an example where we filter out bad words while also checking for errors:
switch -Regex ($inputs) {
'^(?!.*badword).*' { "Clean input" }
'.*error.*' { "Input contains error" }
default { "Unchecked input" }
}
In this code, the first case uses a negative lookahead assertion to ensure that a specified word does not appear in the input, showcasing how regex can refine conditional logic.
Capturing Groups in Regex
Regex capturing groups allow you to extract portions of the string that match a specific pattern. This capability can be extremely useful in scenarios where you need to process matched text further:
switch -Regex ($input) {
'([A-Za-z]+) (.*)' { "Word: $matches[1], Rest: $matches[2]" }
}
In this example, `($matches)` captures groups from the input string, allowing you to reference and utilize those captured values immediately.
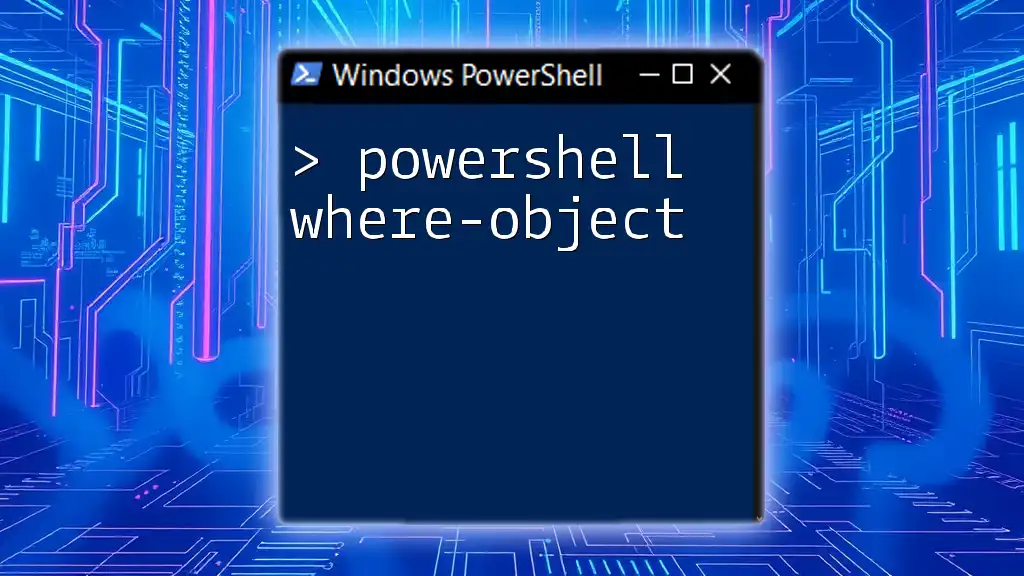
Common Mistakes and Troubleshooting
Common Issues Using Switch with Regex
While `switch -Regex` is powerful, it’s not without its challenges. Some frequent pitfalls include:
- Misunderstanding patterns: Incorrect regex can lead to unexpected results.
- Performance: Complex patterns might slow down execution if not optimized.
Tips for Writing Effective Regex
To ensure success with regex, consider these best practices:
- Start simple and iterate; build complexity gradually.
- Use online regex testers to experiment and validate patterns.
- Comment your regex patterns liberally to describe their intent.
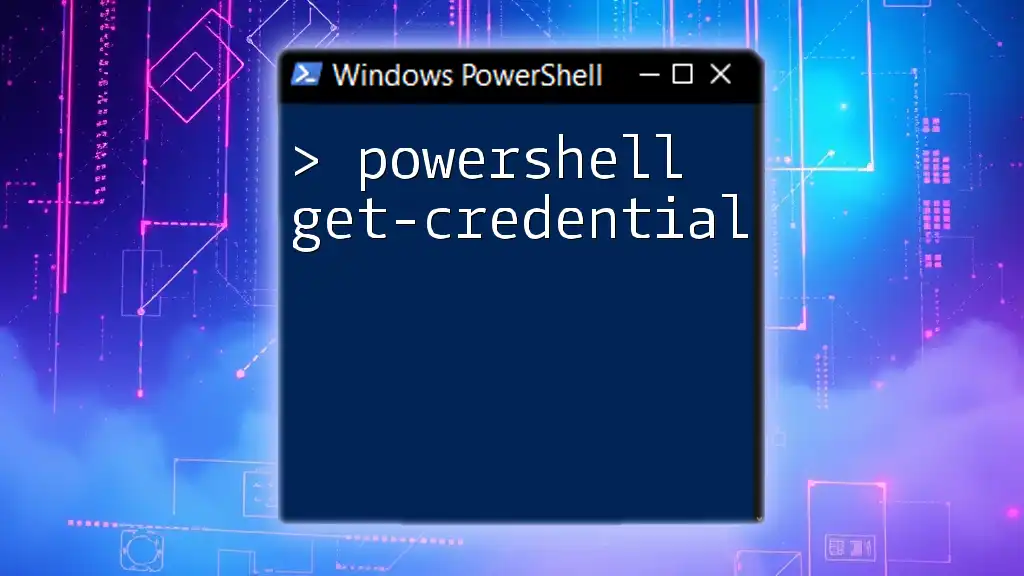
Conclusion
In this guide, we explored the interplay between `switch` and regex in PowerShell, discovering how these tools can simplify and enhance your scripting capabilities. By leveraging the power of regex patterns combined with the `switch` statement, you can manage complex conditionals, validate user input effectively, and maintain code clarity.
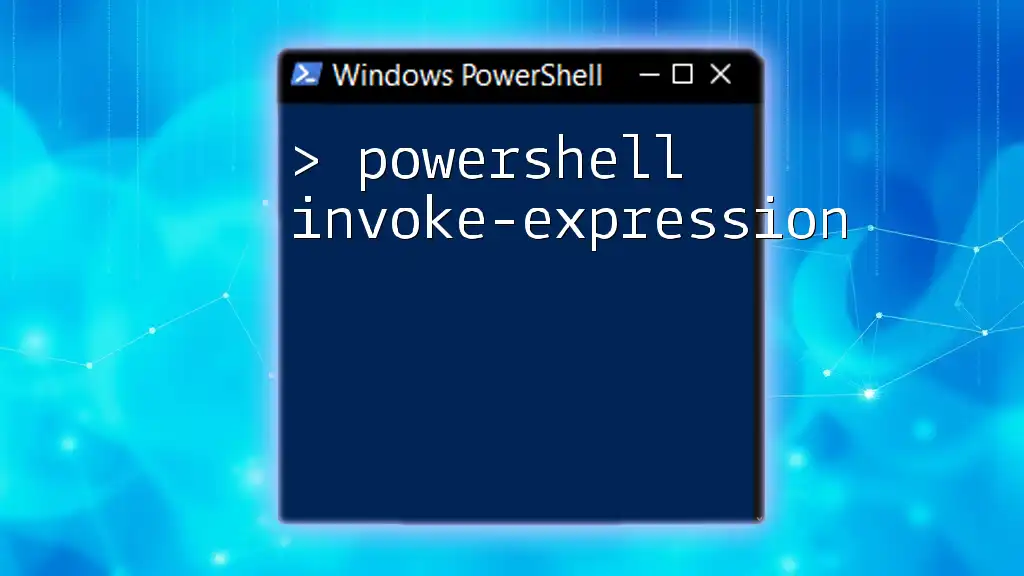
Call to Action
Now it’s your turn to dive into PowerShell and experiment with `switch` and regex! Share your experiences and any interesting patterns you've created. Don’t forget to subscribe for more tips and tricks on mastering PowerShell!
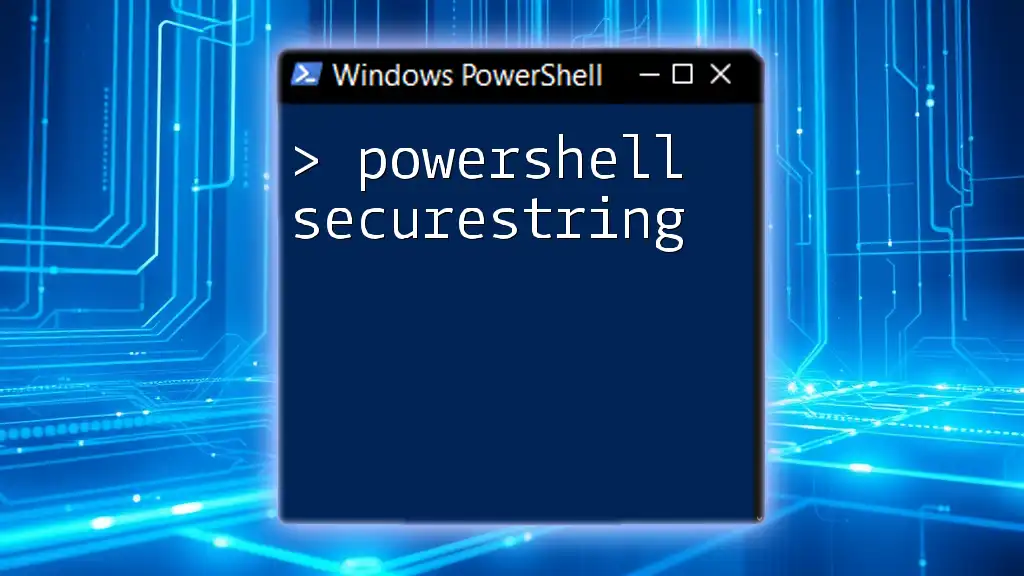
Additional Resources
For those interested in deepening their understanding of PowerShell and regex, check out the official documentation, regex testing tools, and community forums dedicated to PowerShell scripting. You'll find a wealth of information and support to enhance your skills.