A PowerShell regex tester allows users to validate and experiment with regular expressions to ensure they function correctly in string manipulation and pattern matching.
# Example of using a regex in PowerShell to match an email pattern
$email = "test@example.com"
if ($email -match '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$') {
Write-Host "Valid email format"
} else {
Write-Host "Invalid email format"
}
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language designed specifically for system administration and automation tasks. It combines the speed and flexibility of scripting with the ease of use of traditional command-line interfaces. PowerShell allows users to automate repetitive tasks, manage system configurations, and control systems more efficiently.
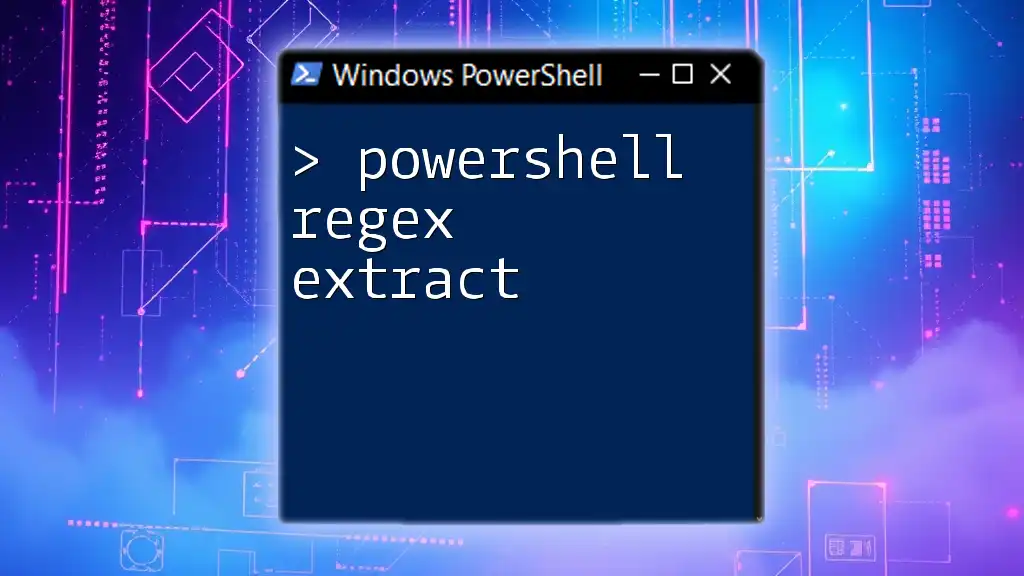
Understanding Regular Expressions
Regular expressions (Regex) are sequences of characters that form a search pattern. They are used for string matching and manipulation. Regex is crucial for tasks such as validation, searching, and replacing text within strings. Whether you're working with user input validation, complex search queries, or data extraction, understanding Regex can significantly enhance your scripting capabilities.
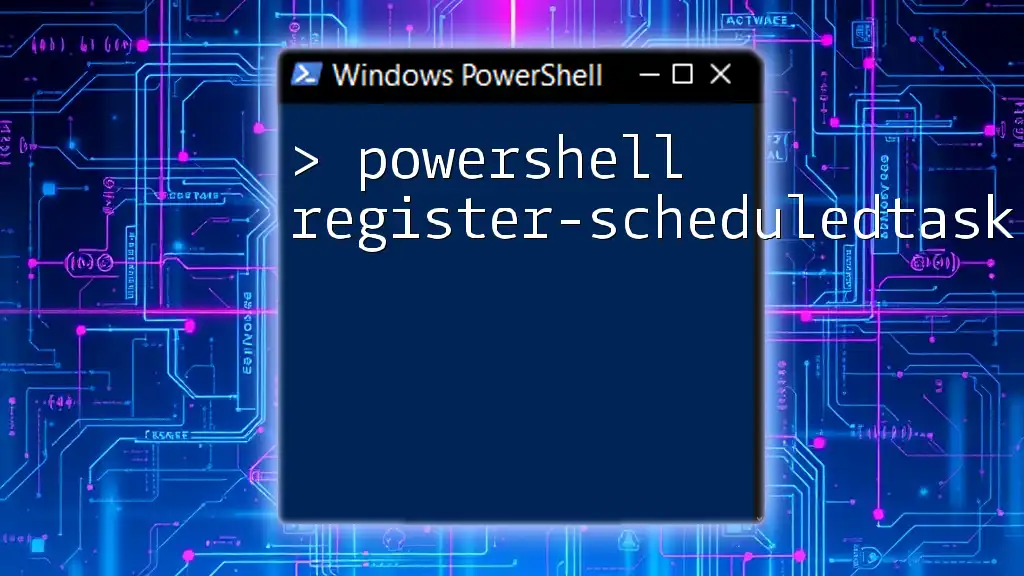
Why Use PowerShell for Regex?
Using PowerShell for Regex offers several advantages:
- Integration: PowerShell seamlessly integrates with various data sources and systems, allowing users to leverage Regex in automation scripts effectively.
- Complex Task Automation: It enables users to automate complex data processing tasks, simplifying operations that would otherwise be tedious.
Common use cases of PowerShell regex include:
- Validating user input formats, such as phone numbers or email addresses.
- Extracting specific phrases or data from logs and other text files.
- Performing advanced search and replace operations in files.
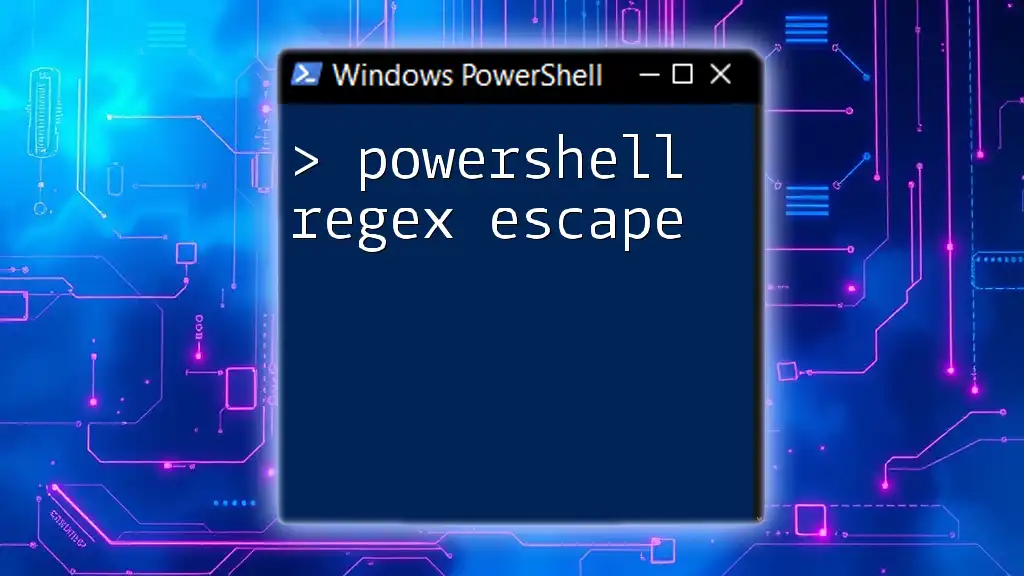
Getting Started with PowerShell Regex
Basic Syntax of PowerShell Regex
PowerShell Regex follows standard Regex syntax, enabling users to build complex patterns. Some fundamental elements include:
- Literal characters: Matches the exact character.
- Metacharacters: Special characters with specific meanings, such as `.` (any character), `^` (beginning of a line), and `$` (end of a line).
Using the `-match` Operator
The `-match` operator is a fundamental tool in PowerShell for testing if a string matches a specific Regex pattern. For example, consider the following simple check:
$string = "Hello World"
if ($string -match "World") {
"Matched!"
}
In this example, the script checks if the substring "World" is present in the variable `$string`. If a match is found, it outputs "Matched!".
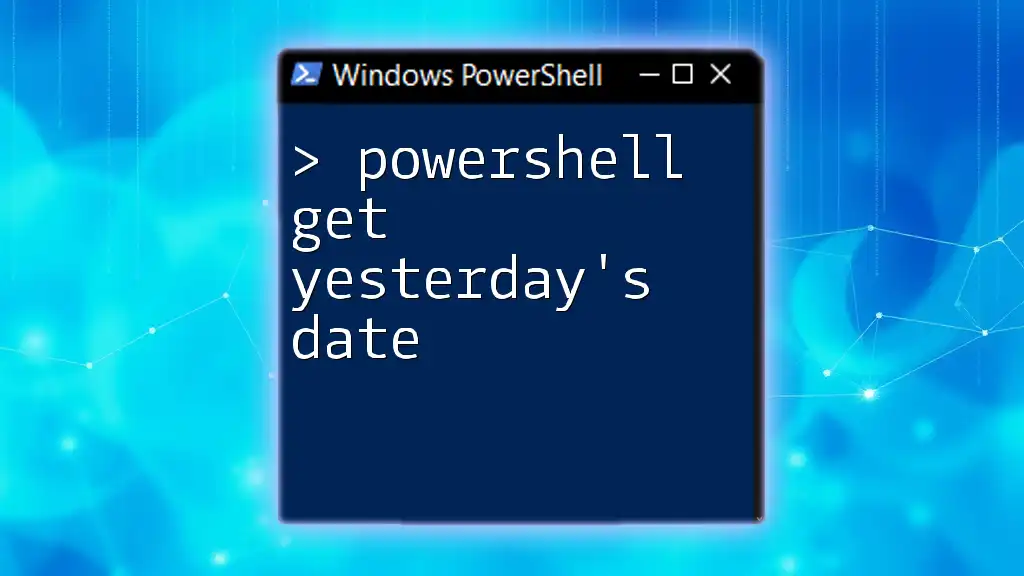
PowerShell Regex Tester Tools
Built-in PowerShell Cmdlets for Testing Regex
PowerShell offers built-in cmdlets like `Select-String` and `-replace` that allow users to work with Regex efficiently.
Creating a PowerShell Regex Tester Script
Creating a simple script to test Regex patterns can enhance your workflow. Here’s a basic example of a PowerShell function that checks for matches:
function Test-RegEx {
[CmdletBinding()]
param (
[string]$Pattern,
[string]$InputString
)
if ($InputString -match $Pattern) {
"Match successful"
} else {
"No match found"
}
}
Example of the PowerShell Regex Tester Script
You can use the `Test-RegEx` function to test various patterns. For instance, if you want to verify if a string follows a specific pattern:
Test-RegEx -Pattern "^[A-Za-z]+\s[A-Za-z]+$" -InputString "John Doe"
This code checks if "John Doe" consists of two words with alphabetic characters.
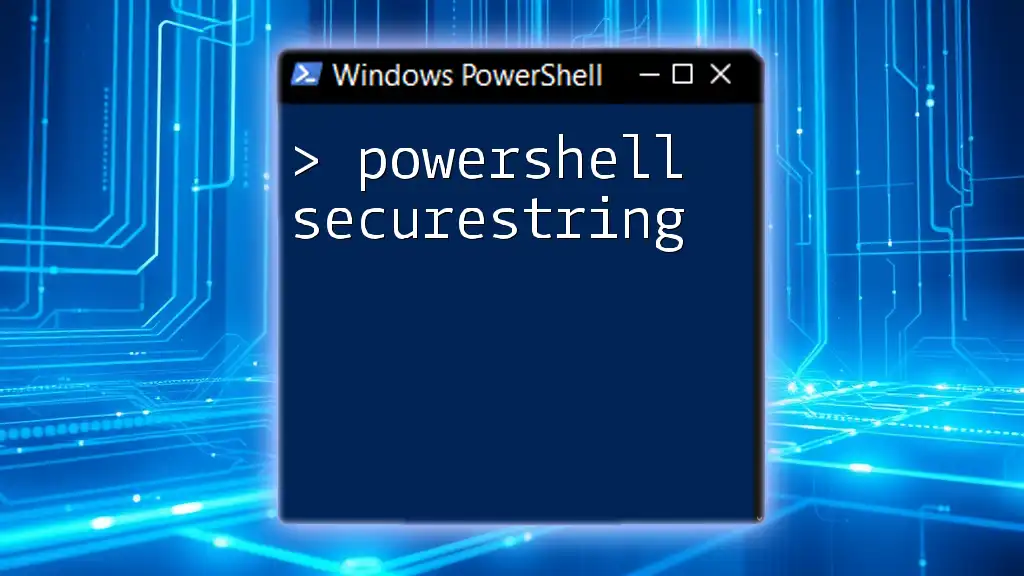
Advanced Regex Patterns in PowerShell
Understanding Regex Metacharacters
Metacharacters in Regex serve special functions. Understanding these is critical for building effective patterns. Here are a few common metacharacters:
- `.`: Matches any single character.
- `*`: Matches zero or more occurrences of the preceding element.
- `?`: Matches zero or one occurrence of the preceding element.
Creating Complex Patterns
Creating complex patterns allows you to craft precise matches for your needs. For example, to match a standard email format, you might use the following pattern:
$emailPattern = "^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}$"
$testEmail = "example@domain.com"
if ($testEmail -match $emailPattern) {
"Valid email!"
}
In this example, the pattern checks for a valid email format by ensuring that it follows the common structure of an email address.
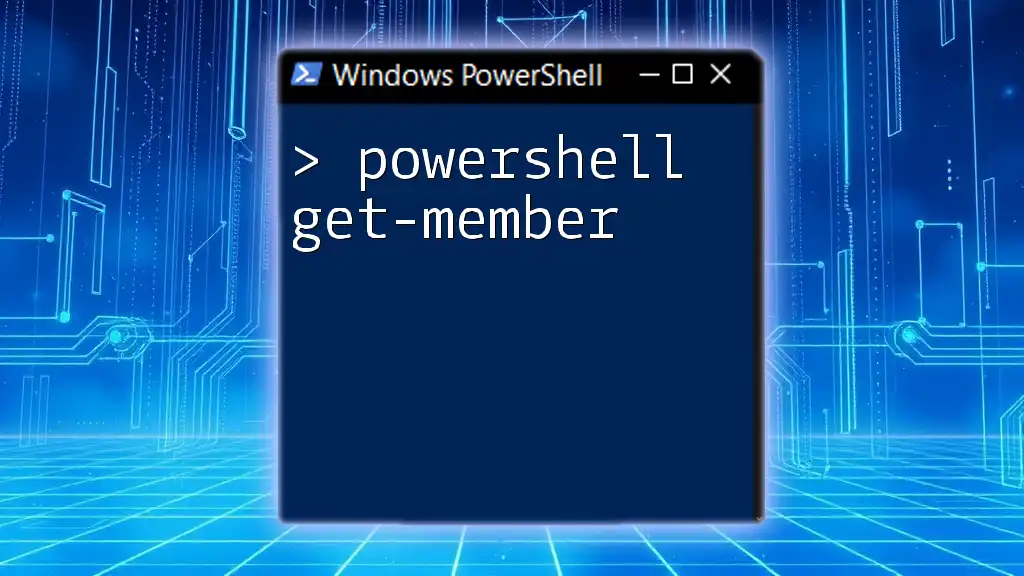
Real-World Applications of PowerShell Regex
Log File Analysis
PowerShell regex is particularly effective for parsing and analyzing log files. For instance, you can use Regex to filter out specific error messages or timestamps, helping troubleshoot issues quickly.
Data Cleanup and Validation
Regular expressions can automate the validation processes in data entry. By using regex patterns, you can ensure that inputs match expected formats, reducing the risk of incorrect data entries.
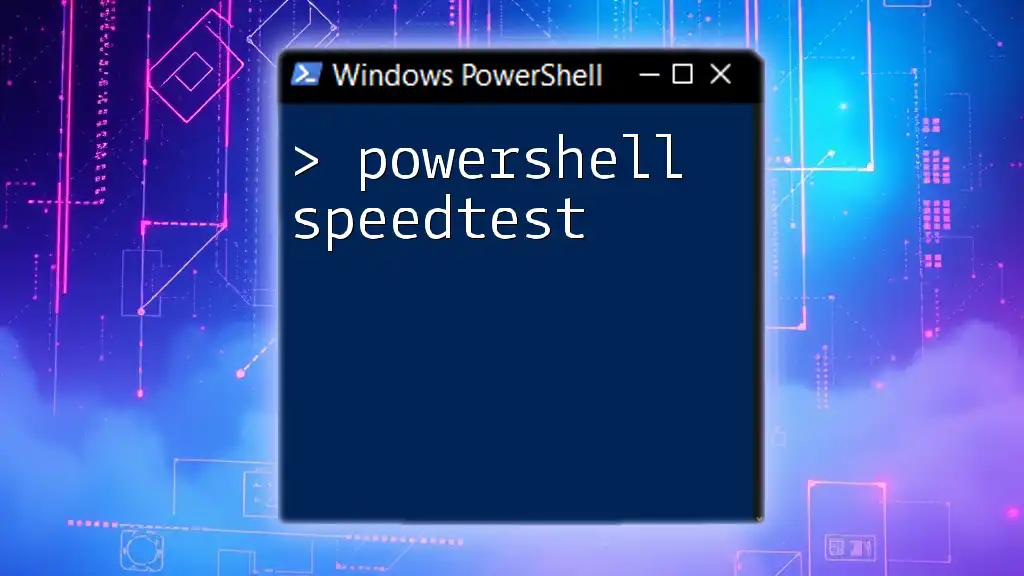
Best Practices for Using PowerShell Regex
Tips for Writing Effective Regex Patterns
- Start simple: Begin with straightforward patterns before incorporating more complex elements.
- Comment your code: When writing complex patterns, add comments for clarity to help others (or yourself) understand the logic later.
Common Pitfalls and How to Avoid Them
Be aware of common mistakes such as:
- Greedy matching: This can lead to unexpected results if not carefully controlled. Use quantifiers wisely.
- Misunderstanding anchors: Anchors like `^` and `$` can change the meaning of your pattern dramatically. Make sure to use them appropriately.
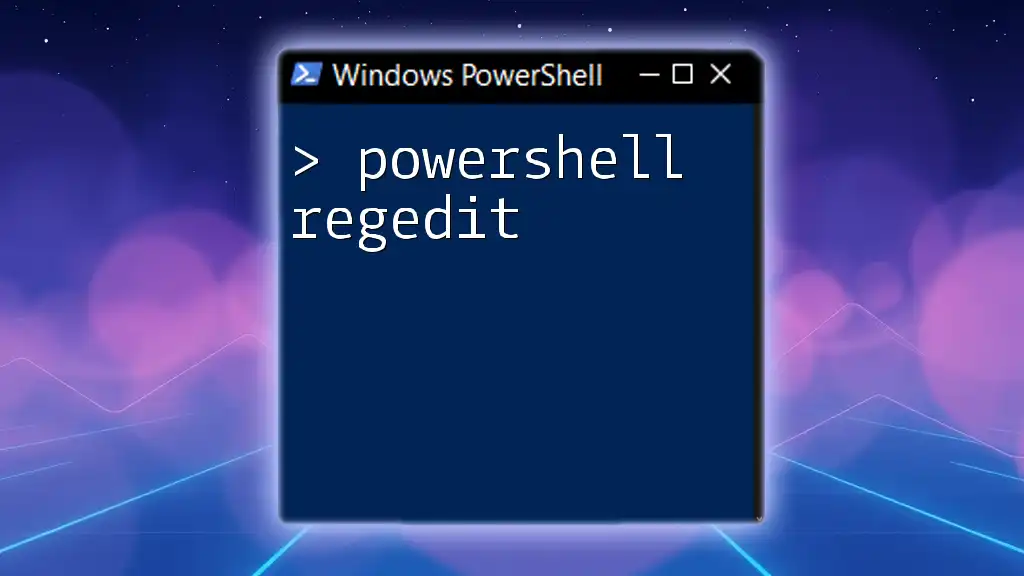
Resources for Further Learning
Books and Online Courses
To deepen your understanding, consider engaging with resources such as books and online courses that focus on PowerShell and Regex. They can provide structured knowledge and hands-on practice.
Community and Forums
Engaging with the PowerShell and Regex communities can substantially enhance your learning. Participating in forums allows you to seek help, share knowledge, and stay updated on best practices.
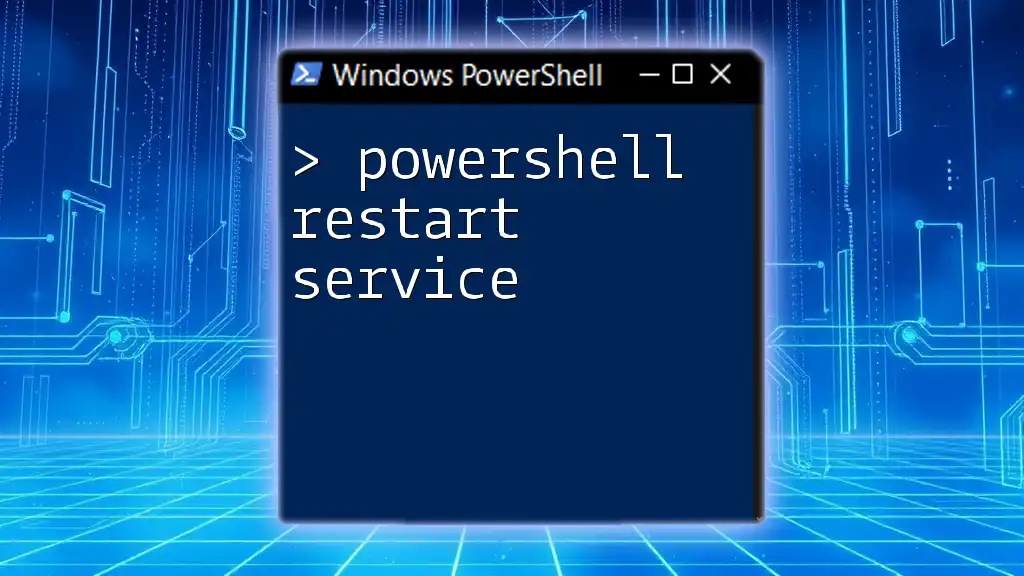
Conclusion
The importance of a PowerShell Regex tester cannot be overlooked, as it provides a practical approach to verifying and testing expressions. By mastering Regex within PowerShell, you can unlock significant efficiencies in your scripting tasks and data processing.
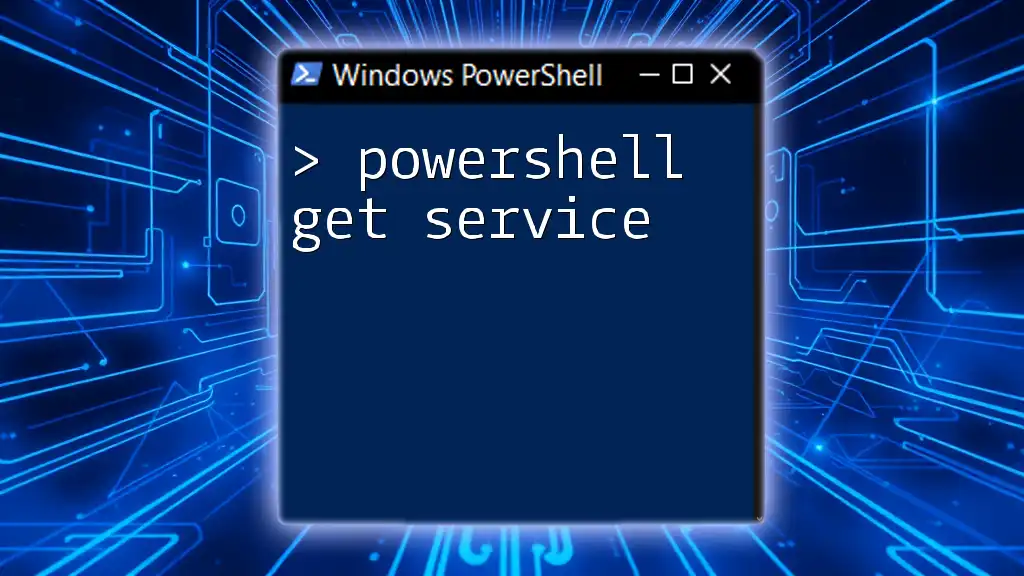
Call to Action
If you're interested in enhancing your PowerShell skills further, consider joining our PowerShell teaching program. Unlock the full potential of regex and scripting with expert guidance and hands-on learning opportunities!