In PowerShell, you can easily obtain yesterday's date by using the `Get-Date` cmdlet with a `-Days` parameter to subtract one day from the current date.
(Get-Date).AddDays(-1)
Understanding the DateTime Object in PowerShell
What is a DateTime Object?
In PowerShell, a DateTime object represents an instant in time, typically expressed as a date and time. Working with DateTime objects allows you to perform various operations, such as comparing dates, formatting them, and manipulating them mathematically.
To create a DateTime object, you can simply invoke `Get-Date`, which generates the current date and time.
$currentDateTime = Get-Date
$currentDateTime
Properties of DateTime
DateTime objects come with several helpful properties that allow you to access specific aspects of the date and time. Key properties include:
- Year: The year component of the date.
- Month: The month component (1-12).
- Day: The day component of the month.
- Hour: The hour component (0-23).
- Minute: The minute component (0-59).
- Second: The second component (0-59).
Here's a code snippet that demonstrates how to access these properties:
$today = Get-Date
"Year: $($today.Year), Month: $($today.Month), Day: $($today.Day)"
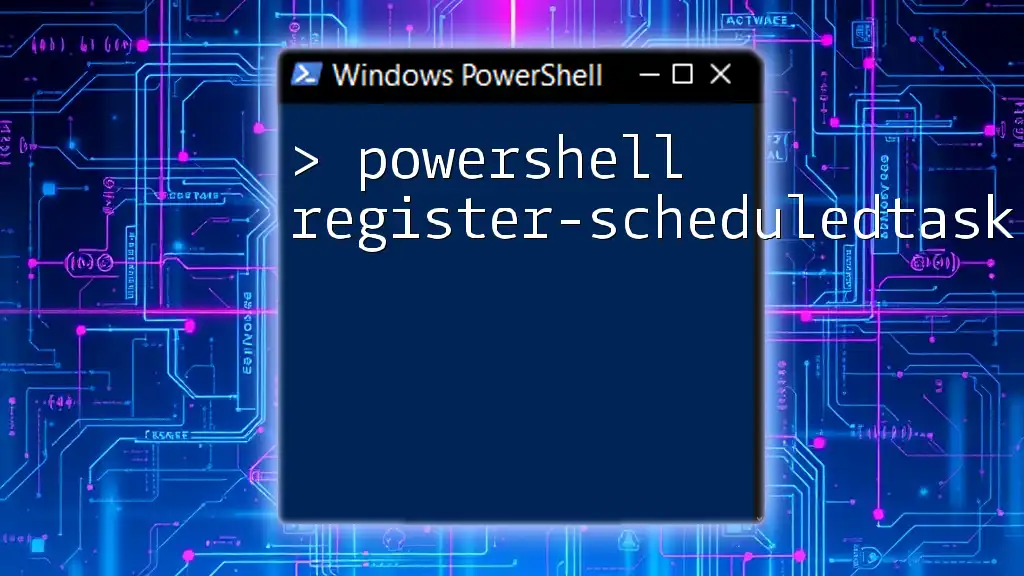
Getting the Current Date
Using Get-Date Cmdlet
The Get-Date cmdlet is the most straightforward way to retrieve the current date and time in PowerShell. When you run this command, it outputs the full date and time.
Get-Date
You can also customize the output to show only specific components or format it to your liking.
Accessing Individual Components
PowerShell makes it easy to extract specific date components. For example, if you want just the current year, you can do:
$currentYear = (Get-Date).Year
$currentYear
This approach gives you flexibility in how you use date information in your scripts.
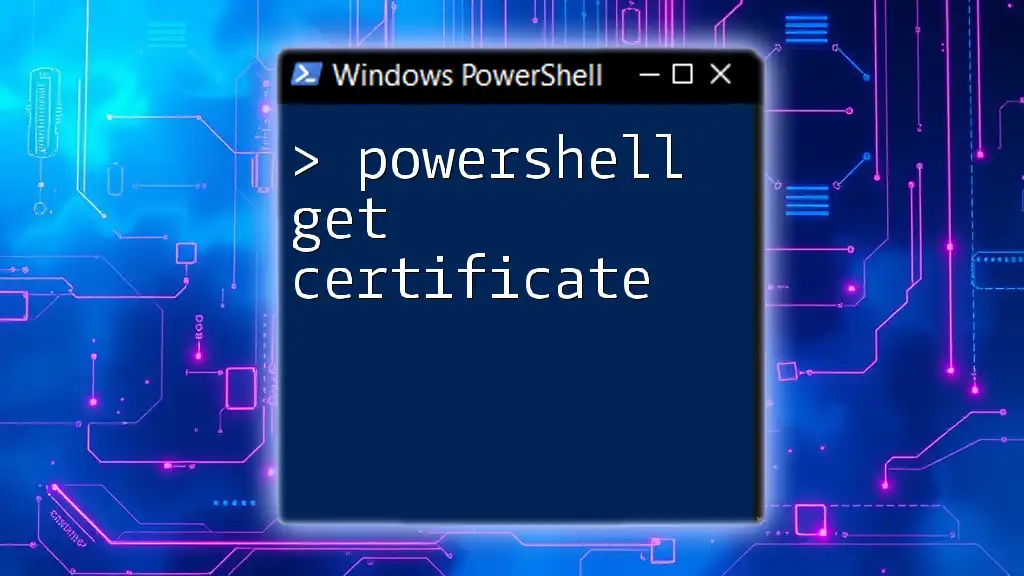
Calculating Yesterday's Date
Using the AddDays Method
One of the simplest ways to get yesterday's date in PowerShell is by using the AddDays method of the DateTime object. You can achieve this by passing -1 as an argument to subtract one day from the current date.
$yesterday = (Get-Date).AddDays(-1)
$yesterday
This command will return the DateTime for the previous day, and it's a common technique in PowerShell scripting.
Alternative Methods to Get Yesterday's Date
Using Get-Date with -Day Parameter
Another method involves using the Get-Date cmdlet with a modified day parameter. This approach involves some arithmetic to ensure you correctly capture yesterday's date.
Get-Date -Day ((Get-Date).Day - 1)
Using Date Manipulation with TimeSpan
You can also use a TimeSpan object to manipulate dates. This method provides an alternate means of subtracting a day from the current date.
$yesterday = (Get-Date) - [TimeSpan]::FromDays(1)
$yesterday
This can be particularly useful in more complex date manipulations.
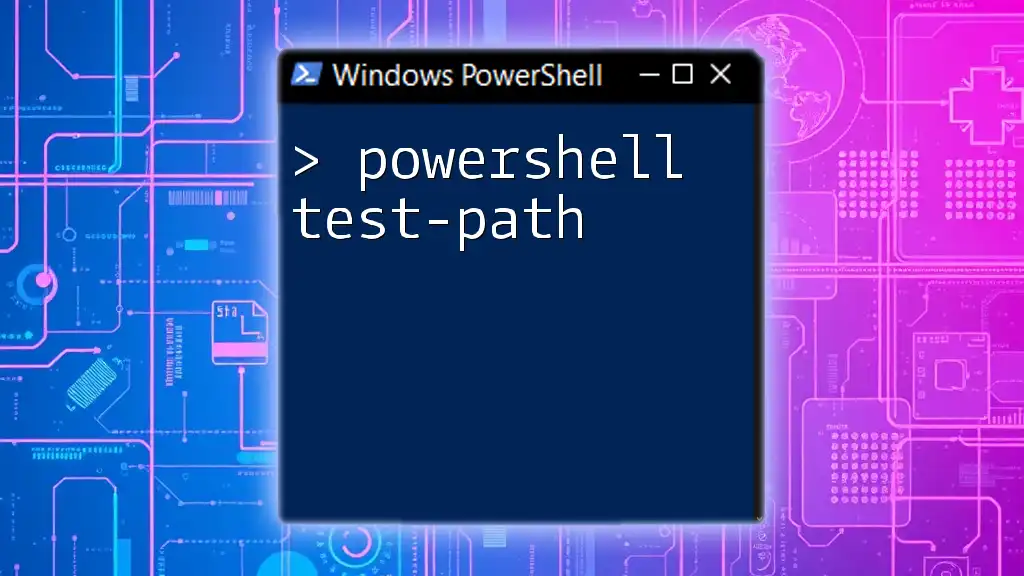
Formatting Yesterday's Date
Common Date Formats
When working with dates, it may be necessary to format them for output or logging purposes. PowerShell makes this easy with the ToString method.
$formattedYesterday = $yesterday.ToString("MM/dd/yyyy")
$formattedYesterday
This command formats yesterday's date into a more readable form, which can be tailored to your needs.
Custom Formatting Options
The -Format parameter in the Get-Date cmdlet allows for custom date formatting directly in the command.
Get-Date -Format "yyyy-MM-dd"
This flexibility enables you to create outputs that match your project's requirements for dates.
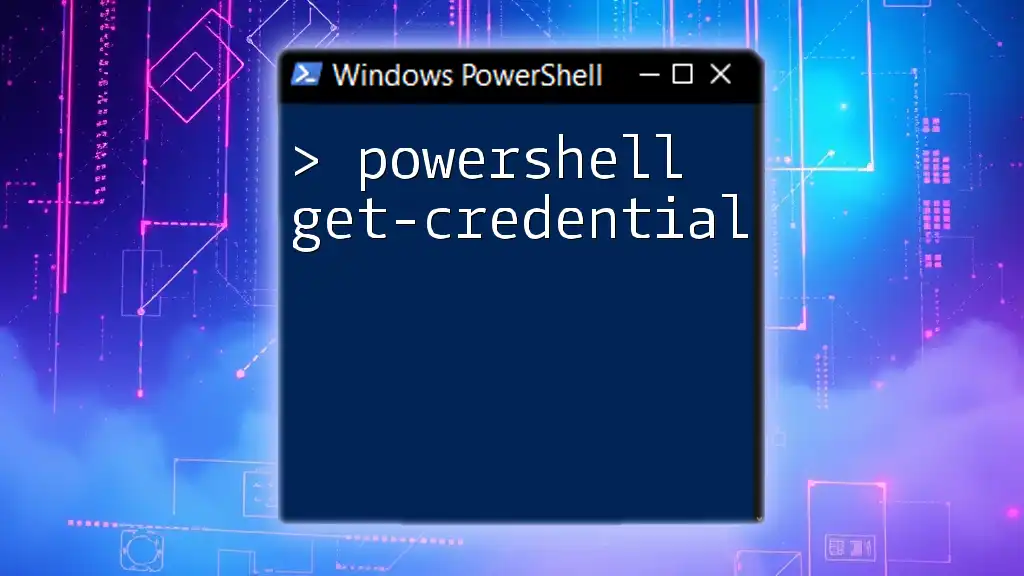
Use Cases for Getting Yesterday's Date
Scripting and Automation
Getting yesterday's date is exceptionally useful in scripting and automation tasks. For instance, if you are running daily reports, you might want to filter results based on yesterday's data. This can be incorporated into your scripts seamlessly.
Log Management
Yesterday’s date can also be vital for log management tasks. Filtering logs based on date is a common requirement. Here’s a basic example demonstrating how to filter logs using yesterday's date:
Get-EventLog -LogName Application | Where-Object { $_.TimeGenerated -gt $yesterday }
This command retrieves events from the Application log that occurred after yesterday's date.
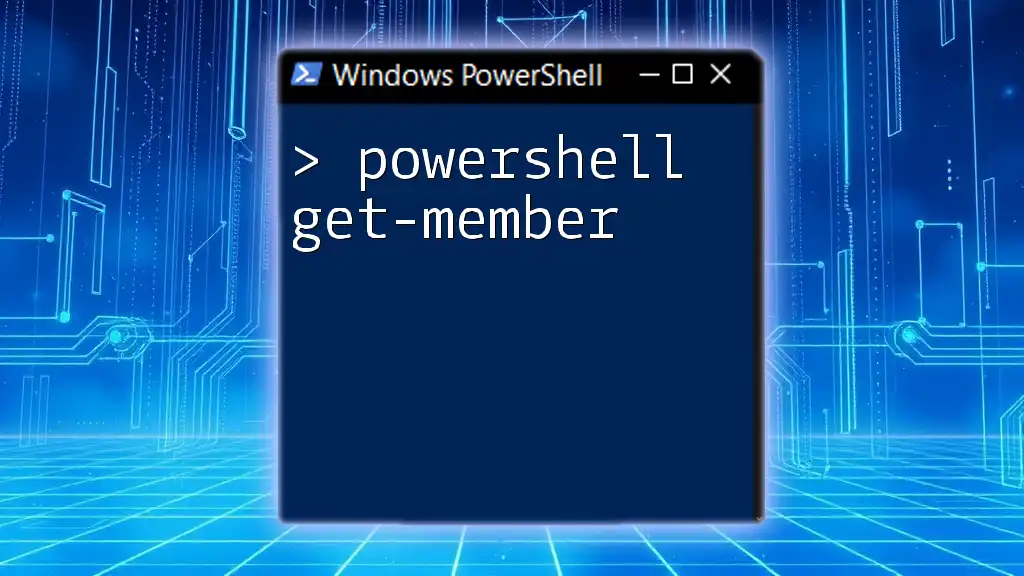
Troubleshooting Common Issues
Handling Edge Cases
When working with dates, be mindful of month-end and year-end scenarios. For example, if today is the 1st of a month, simply subtracting a day could lead to incorrect results. The AddDays method will automatically handle these transitions for you.
FAQs Related to Date Manipulation
Common questions often arise, such as "What if today is the first of the month?" PowerShell commands leverage DateTime logic which inherently manages these date transitions smoothly, ensuring you get accurate results without additional code.
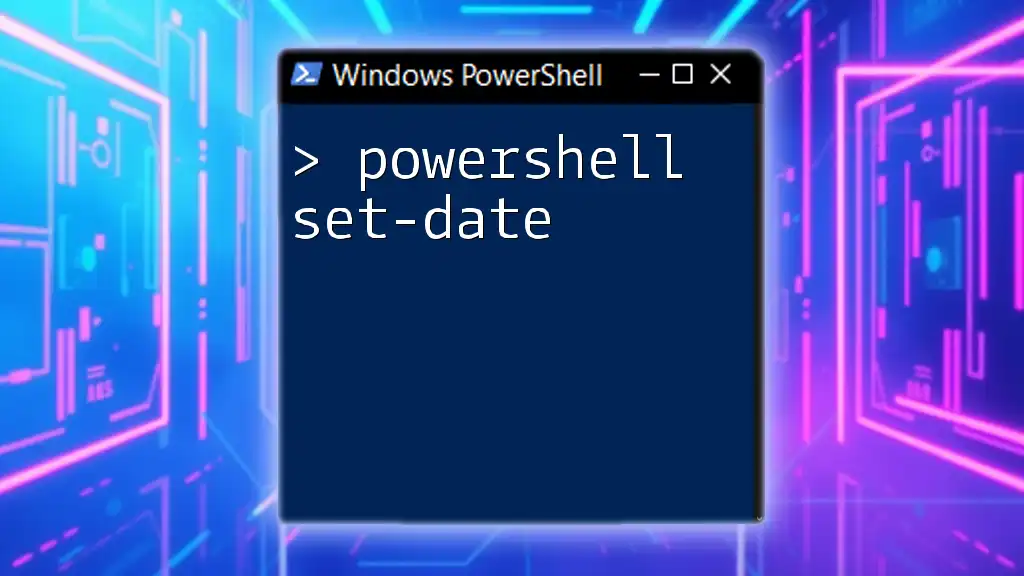
Conclusion
In summary, mastering how to get yesterday's date in PowerShell is crucial for efficient scripting and automation. By utilizing the methods described above, you can integrate date manipulation into your workflows seamlessly. The ability to extract, format, and utilize date information correctly will greatly enhance your PowerShell scripting capabilities.
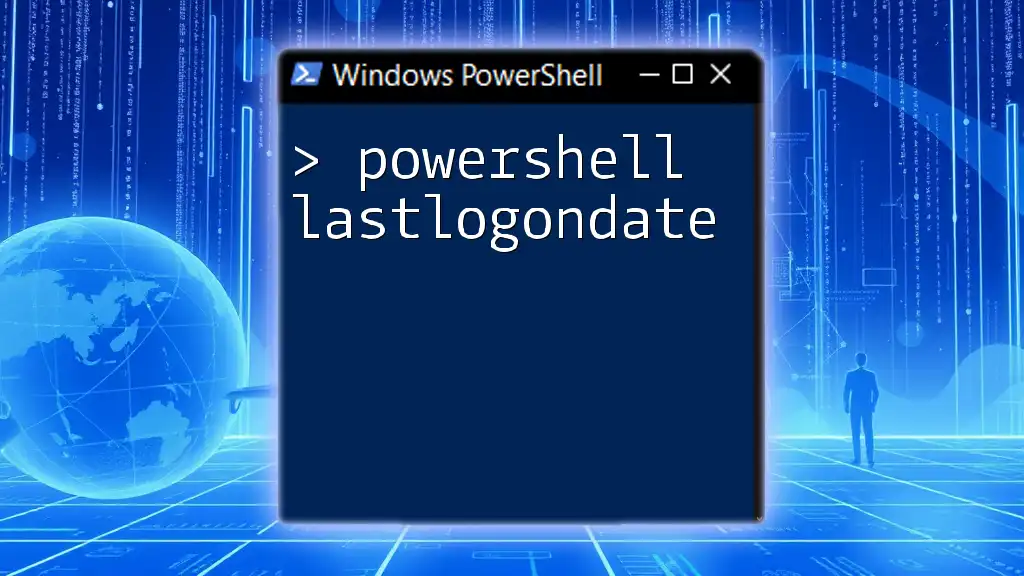
Call to Action
Feel free to share your own tips or experiences with using dates in PowerShell. Don't forget to subscribe to our updates for more intriguing articles and techniques in PowerShell scripting.
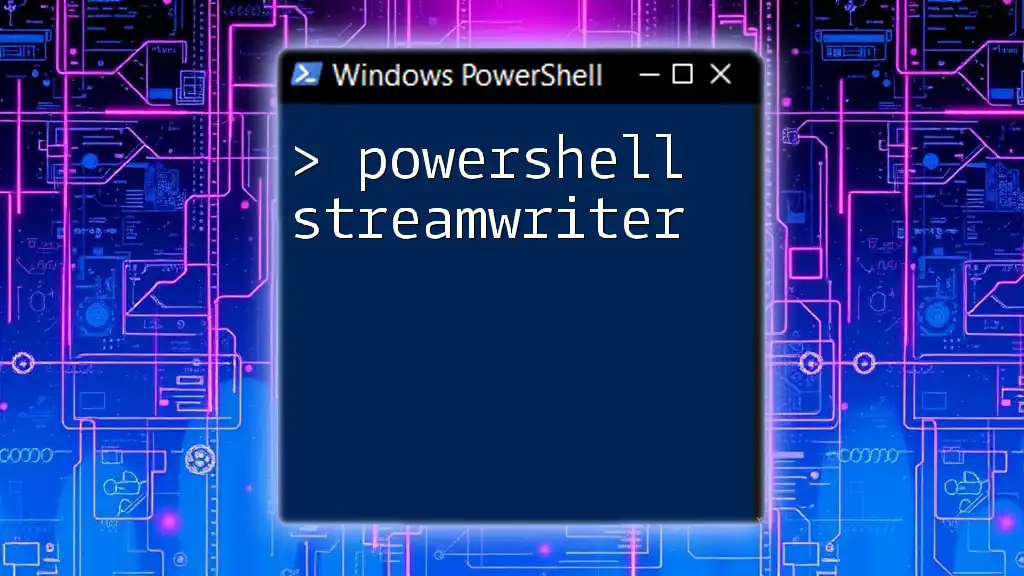
Resources
For further reading, refer to the official PowerShell documentation on DateTime objects and cmdlets for more advanced features and capabilities.