To subtract dates in PowerShell, you can simply use the `New-TimeSpan` cmdlet to find the difference between two date objects.
$startDate = Get-Date '2023-10-01'
$endDate = Get-Date '2023-10-15'
$timeSpan = New-TimeSpan -Start $startDate -End $endDate
$timeSpan.Days # Output will be the number of days between the two dates
Understanding Dates in PowerShell
PowerShell DateTime Objects
PowerShell represents dates using DateTime objects, which are crucial for date and time manipulation within scripts. When you use PowerShell, understanding how it handles these objects allows you to perform various operations effectively.
To create DateTime objects in PowerShell, you can utilize the `Get-Date` cmdlet. This cmdlet not only retrieves the current date and time but also allows you to construct DateTime objects for specific dates.
Here's an example of creating two DateTime objects:
$date1 = Get-Date "2023-10-01"
$date2 = Get-Date "2023-10-15"
With these two DateTime objects, you can now proceed to perform various calculations, including subtracting dates.
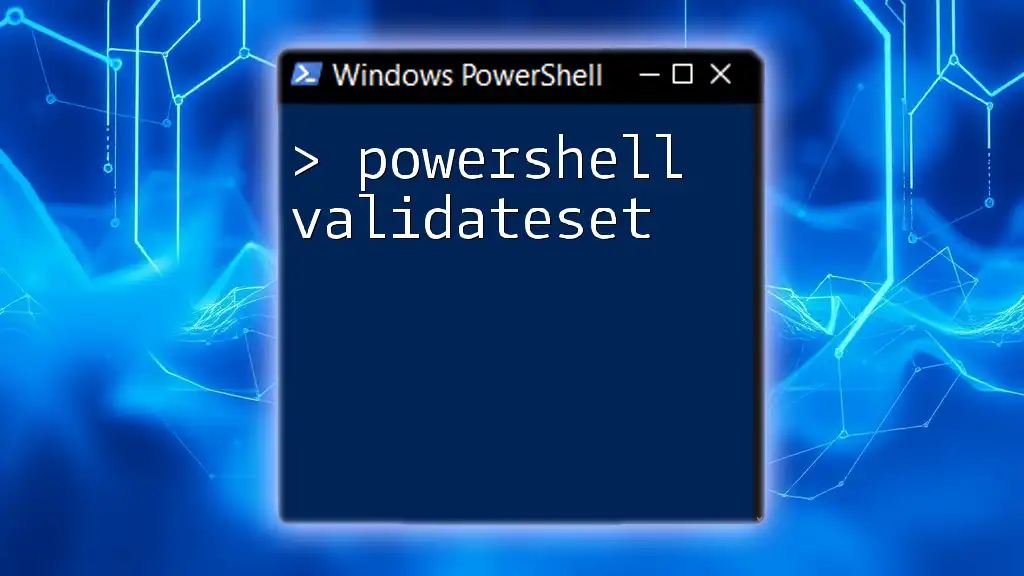
Subtracting Dates in PowerShell
Basic Date Subtraction
The simplest way to subtract two dates in PowerShell is by directly performing the subtraction operation. This action yields a TimeSpan object, which represents the duration between the two DateTime values.
To see how this works and to find the difference between the two dates you created earlier:
$dateDifference = $date2 - $date1
Write-Output "The difference is: $dateDifference"
Understanding the Output
When you subtract dates, the output will be in the form of a TimeSpan object, which contains useful properties such as `Days`, `Hours`, `Minutes`, and `Seconds`. These properties can be extracted for further analysis.
To break down the output further, you can access specific components of the TimeSpan:
Write-Output "Days: $($dateDifference.Days)"
Write-Output "Hours: $($dateDifference.Hours)"
Write-Output "Minutes: $($dateDifference.Minutes)"
This allows you to view the date difference in greater detail, making it easier to format the output to suit your needs.
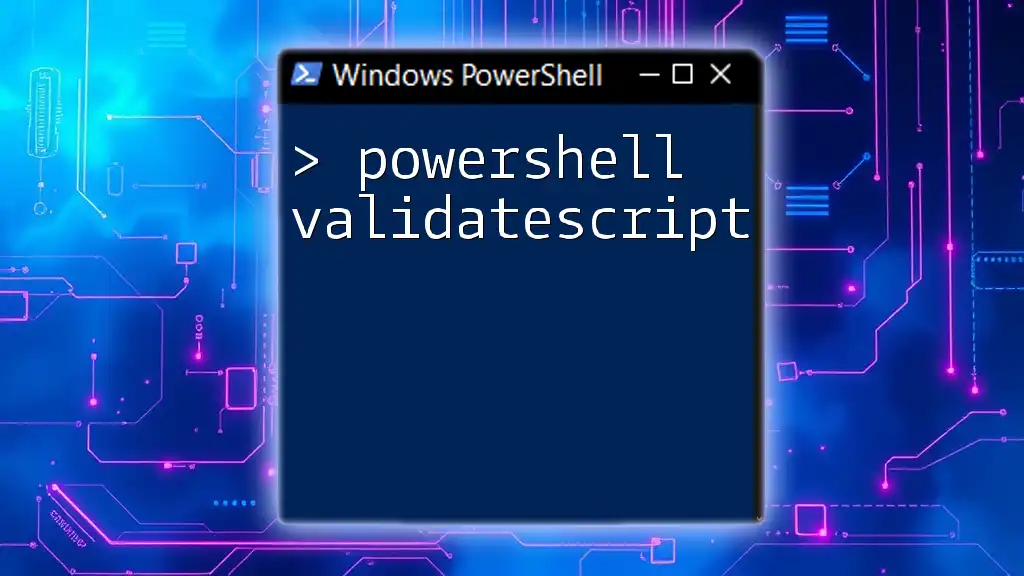
Using `New-TimeSpan` for Date Differences
What is New-TimeSpan?
The `New-TimeSpan` cmdlet provides another method to calculate the difference between dates, giving a more explicit and descriptive way to handle date subtraction. By specifying the start and end dates, you can obtain the duration without performing direct subtraction.
Here’s how to use `New-TimeSpan`:
$timeSpan = New-TimeSpan -Start $date1 -End $date2
Write-Output "Total days: $($timeSpan.Days)"
This will calculate the number of days between `date1` and `date2`, returning a clear, structured output.
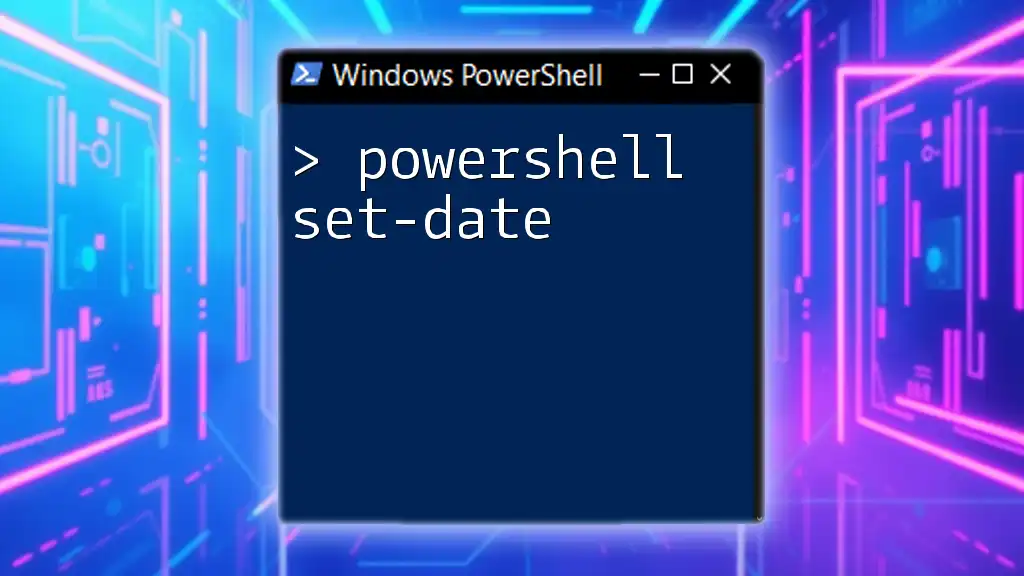
Advanced Date Difference Scenarios
Subtracting Dates Considering Time Components
In many cases, dates aren't restricted to only the date component; they may also include time. This factor is especially important for precise calculations. If you want to consider hours, minutes, and seconds while subtracting:
$date1 = Get-Date "2023-10-01 14:30:00"
$date2 = Get-Date "2023-10-15 09:00:00"
$timeDifference = $date2 - $date1
Write-Output "Difference: $timeDifference"
In this example, you will get a TimeSpan that reflects the total difference, including the time components.
Working with Date Formats
PowerShell can handle various date formats, but it’s essential to parse them correctly. Using the `ParseExact` method allows you to define the expected format, eliminating common parsing errors.
Here’s how to parse a date from a string effectively:
$date1 = [DateTime]::ParseExact("01/10/2023", "dd/MM/yyyy", $null)
By specifying the date format, you ensure that PowerShell interprets the date as intended.
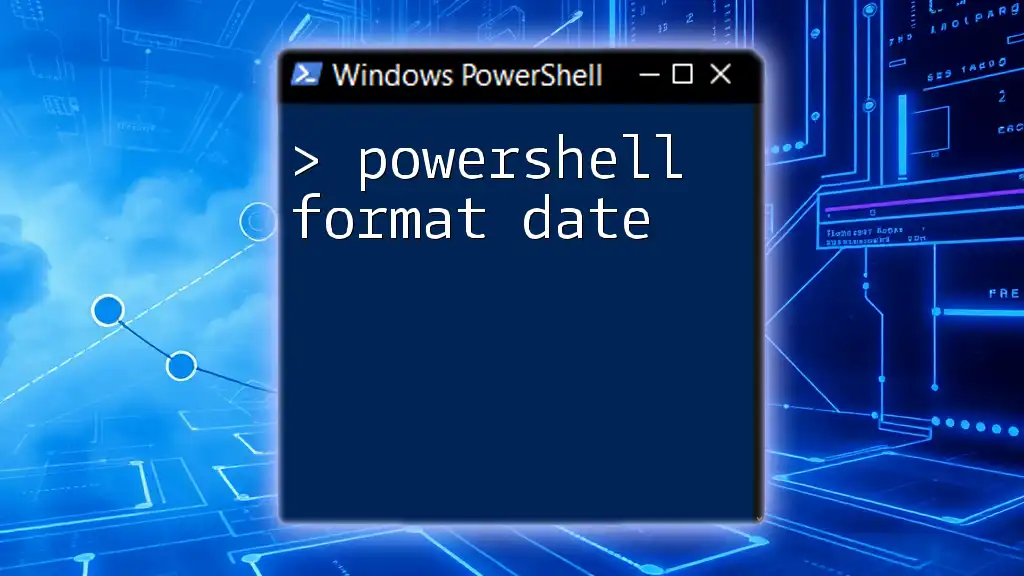
Practical Examples
Example 1: Calculate Age from a Birthdate
A common use case for subtracting dates is calculating age based on a birthdate. Here’s a concise way to do this:
$birthdate = Get-Date "1990-04-01"
$currentDate = Get-Date
$age = New-TimeSpan -Start $birthdate -End $currentDate
Write-Output "Age: $($age.Years) years"
The above script calculates the difference between the current date and the birthdate, providing a straightforward way to determine how many years old someone is.
Example 2: Time Until Next Event
Another practical application is determining the time remaining until a significant future event. This can be quite beneficial for planning purposes. Here’s how to accomplish that:
$nextEvent = Get-Date "2023-12-25"
$timeUntilEvent = New-TimeSpan -Start (Get-Date) -End $nextEvent
Write-Output "Time until next event: $($timeUntilEvent.Days) days"
This output will tell you precisely how many days are left until Christmas, allowing you to better organize and plan accordingly.
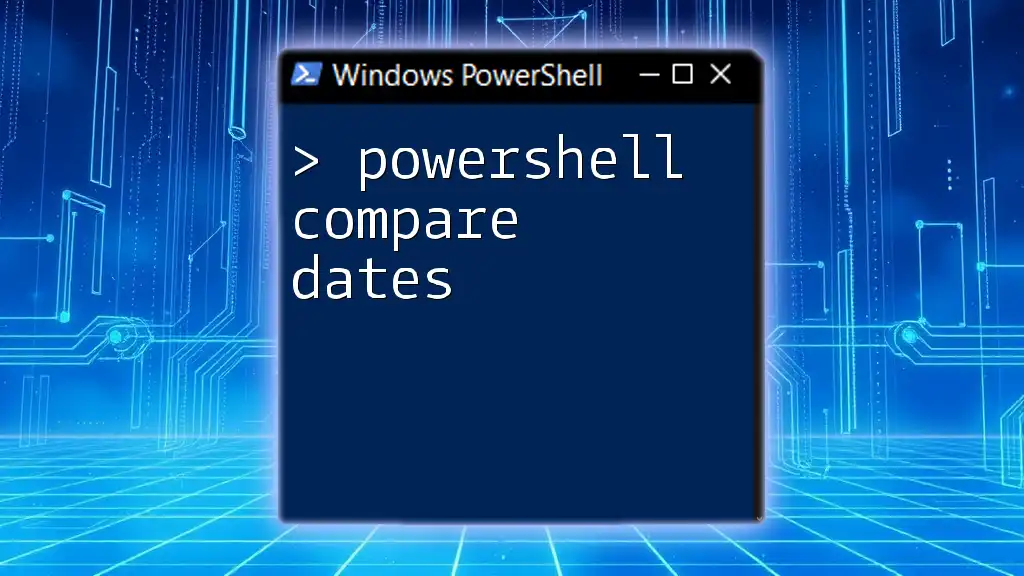
Best Practices for Date Manipulation in PowerShell
Consistency with Date Formats
When working on scripts that involve date manipulation, maintaining a consistent date format is crucial. Establishing a standard format can help prevent misinterpretations and reduce potential bugs.
Utilizing PowerShell's Built-in Cmdlets
Whenever possible, it’s best to utilize PowerShell’s built-in functions and cmdlets for enhanced performance and reliability. Functions like `Get-Date`, `New-TimeSpan`, and direct date arithmetic simplify scripting and make your code more readable.
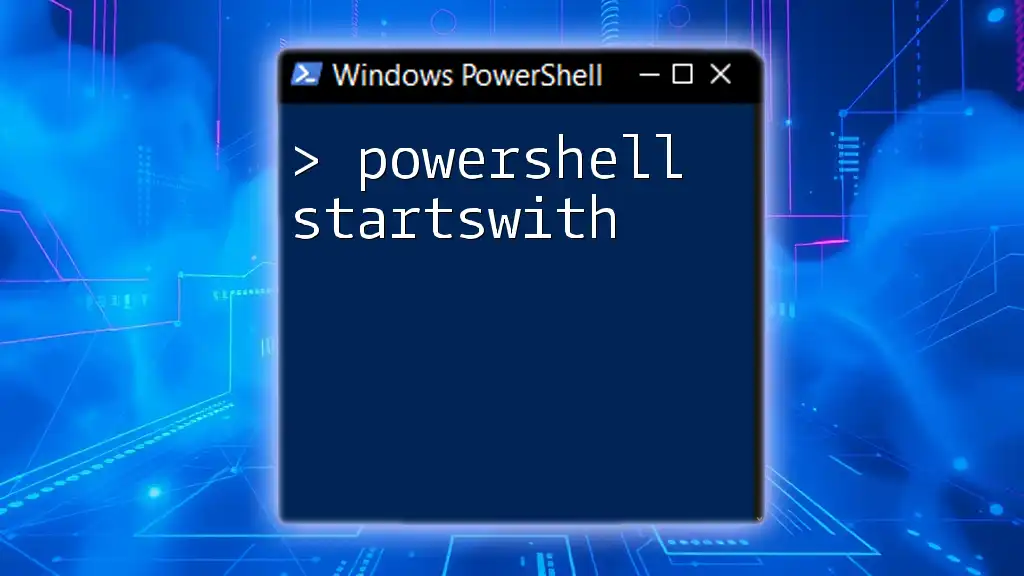
Conclusion
In summary, understanding how to efficiently subtract dates in PowerShell unlocks various possibilities for data manipulation and analysis. By using DateTime objects, new cmdlets, and proper formatting, you can create dynamic and effective scripts that serve a multitude of applications in systems administration, project management, and beyond. Practice these techniques to become proficient in managing time effectively in your PowerShell scripts.
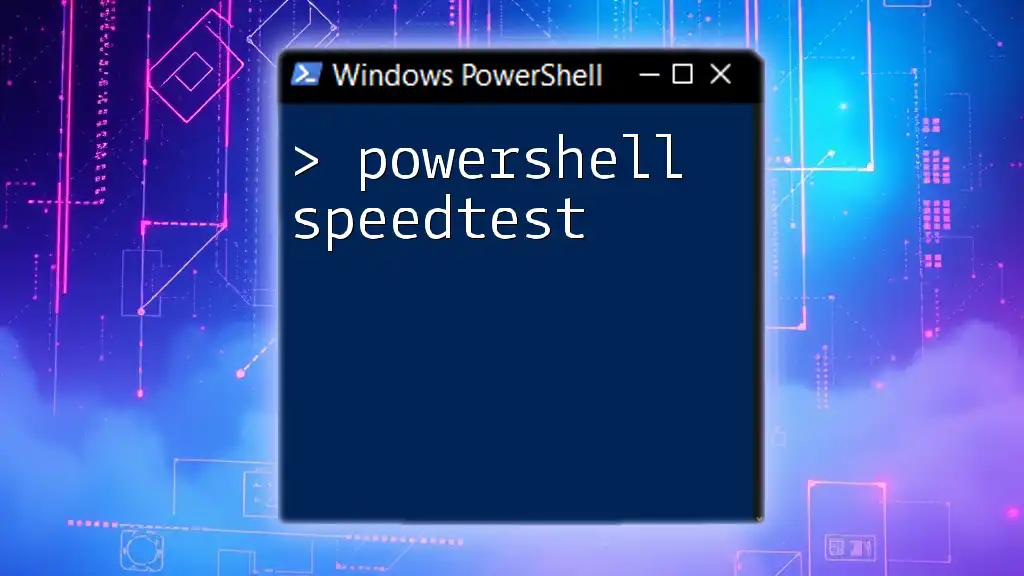
Further Resources
For those looking to deepen their understanding of PowerShell date manipulation, consider exploring additional reading materials, such as PowerShell documentation or community forums where you can engage with others interested in mastering PowerShell scripting.