The PowerShell command to retrieve the host name of your computer is `Get-ComputerInfo | Select-Object -ExpandProperty CsName`.
Here’s the code snippet in markdown format:
Get-ComputerInfo | Select-Object -ExpandProperty CsName
This command will display the host name, allowing you to quickly identify your machine on a network.
Understanding PowerShell Commands
What is PowerShell?
PowerShell is a task automation and configuration management framework developed by Microsoft. It combines a command-line shell and an associated scripting language, which enables users to run scripts and commands to manage systems and automate administrative tasks. A powerful feature of PowerShell is its cmdlets—simple, built-in functions that allow users to execute predefined operations smoothly.
The Need for Host Names
In the world of networking and systems administration, host names play a crucial role. They are human-readable labels that identify a device on a network, making it easier to manage resources. Retrieving the host name can be critical for various scenarios, including configuring network settings, monitoring system health, or troubleshooting connectivity issues.

Getting the Host Name in PowerShell
Using the `Get-ComputerInfo` Cmdlet
One of the simplest ways to retrieve the host name in PowerShell is by using the `Get-ComputerInfo` cmdlet. This cmdlet provides a wide range of information about the computer on which it is executed, including the host name.
Example code snippet:
Get-ComputerInfo | Select-Object -Property CsName
Explanation: In the example above, the result displays the system's host name found under the `CsName` property. This method is simple and concise, making it suitable for quick retrieval of the host name.
Using the `hostname` Command
For those who prefer brevity and speed, PowerShell offers the `hostname` command. This command is designed to return the host name directly without any additional overhead.
Example code snippet:
hostname
Explanation: When this command is executed, it outputs the host name of the machine. It is particularly useful for those who want to retrieve the host name quickly without using more complex cmdlets.
Utilizing the `System.Net.Dns` Namespace
Another way to get the host name is through the .NET framework. PowerShell users can access the `System.Net.Dns` namespace to retrieve the host name programmatically.
Example code snippet:
[System.Net.Dns]::GetHostName()
Explanation: This method utilizes the underlying capabilities of .NET to fetch the host name. It can be particularly useful when integrating PowerShell scripts with .NET applications or when additional DNS functionalities are required.
Retrieving Host Name with `Get-WmiObject`
WMI (Windows Management Instrumentation) is another powerful tool within PowerShell that provides a wealth of system information. By using `Get-WmiObject`, you can easily extract the host name from the Win32_ComputerSystem class.
Example code snippet:
(Get-WmiObject -Class Win32_ComputerSystem).Name
Explanation: This command queries WMI for detailed system information, allowing retrieval of the host name directly from the specified class. This approach is particularly helpful when users need more information about the system alongside the host name.

When to Use Each Method
Comparing Methods
Choosing the right approach to retrieve the host name depends on your specific needs and preferences:
- Get-ComputerInfo: Best for users seeking additional computer details alongside the host name.
- hostname: Ideal for quick and straightforward retrieval without additional information.
- System.Net.Dns: Utilized in .NET integrated scenarios or when needing advanced DNS functionalities.
- Get-WmiObject: Suitable for more detailed queries where additional system information is required.
Understanding these differences can help users select the best method for their specific administrative tasks.
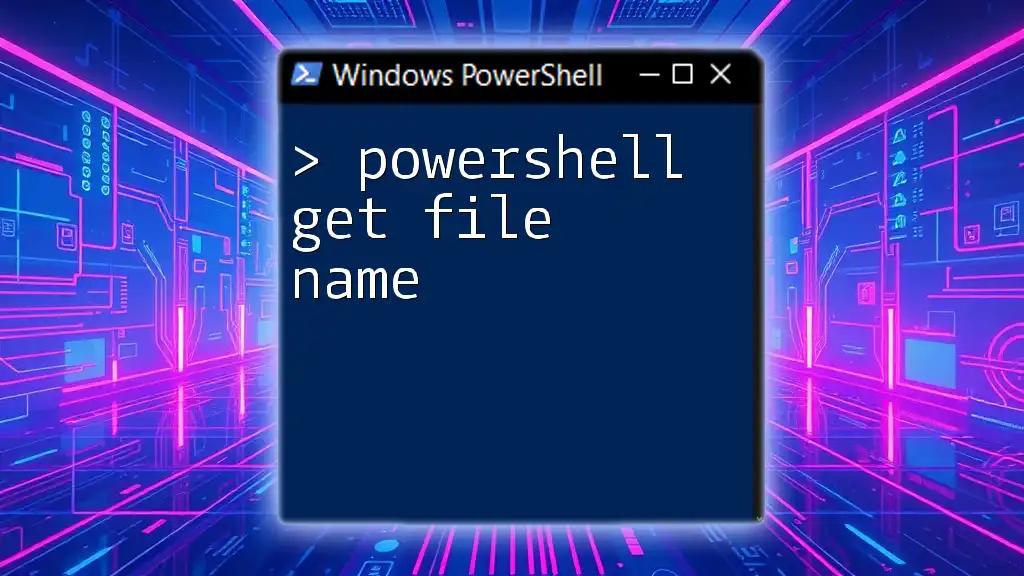
Advanced Usage Scenarios
Retrieving Host Name for Remote Machines
PowerShell also allows administrators to fetch the host name of remote machines, enhancing its utility in network management. This requires employing the remoting feature of PowerShell.
Example code snippet:
Invoke-Command -ComputerName "RemotePC" -ScriptBlock { (Get-WmiObject -Class Win32_ComputerSystem).Name }
Explanation: In the above example, the Invoke-Command cmdlet initiates a remote session to "RemotePC" and retrieves the host name using `Get-WmiObject`. However, administrators should be aware of security implications, network configurations, and prerequisites needed for successful remoting.
Using Host Name in Scripts
Storing the retrieved host name in a variable is a practical way to incorporate it into larger scripts.
Example code snippet:
$hostname = (Get-ComputerInfo).CsName
Write-Host "The host name is: $hostname"
Explanation: In this snippet, the host name is saved to the `$hostname` variable, which can be displayed or used in further script processes, enhancing script versatility.

Troubleshooting Common Issues
Typical Errors and Solutions
While retrieving the host name in PowerShell is generally straightforward, users may encounter a few common errors:
- Permission Denied: Always ensure that you have the necessary permissions to execute the commands, especially when working with remote systems.
- Network Issues: If you cannot retrieve a host name for a remote machine, verify the network connection and that the target machine is powered on and accessible.
- Cmdlet Not Found: Ensure that the PowerShell version is compatible and that you're using the correct cmdlet/cmdlet syntax.
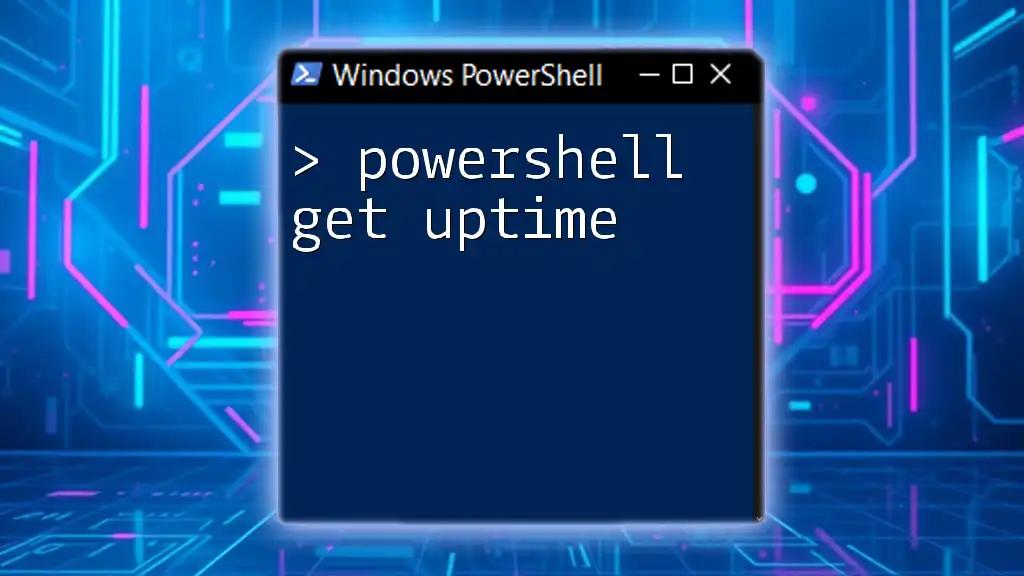
Conclusion
Retrieving the host name using PowerShell is an essential skill for system administrators and IT professionals. With various methods available, users can choose the approach that best fits their needs. As you explore the use of PowerShell get host name, remember to practice these commands in your environment.
For more tips and tutorials on PowerShell, consider exploring additional resources or joining our community to enhance your learning experience!