To retrieve a username from a Security Identifier (SID) in PowerShell, you can use the following command snippet:
$SID = 'S-1-5-21-1234567890-123456789-1234567890-1001'; (New-Object System.Security.Principal.NTAccount($SID)).Translate([System.Security.Principal.SecurityIdentifier]).Value
This command will take the specified SID and convert it into the corresponding username.
Understanding Security Identifiers (SIDs)
What is a SID?
A Security Identifier (SID) is a unique value used to identify a security principal (such as a user or group) in Windows operating systems. It is an essential component of the Windows security architecture, serving as a way to manage permissions and access control.
The structure of a SID consists of several segments that provide information such as the authority that issued the SID, domain or local machine identifiers, and a relative identifier (RID) that uniquely identifies the principal. Understanding SIDs is crucial for system administrators to effectively manage security and access.
Why Retrieve Usernames from SIDs?
Retrieving usernames from SIDs can be vital for numerous reasons. As a system administrator or security officer, you might need to:
- Audit user accounts: Check which accounts have certain permissions or access rights.
- Analyze compliance: Ensure that user permissions align with company policies.
- Investigate security breaches: Identify accounts involved in unauthorized access or suspicious activity.
For example, if a security incident occurs and you have the SID of an account that was involved, knowing how to retrieve the associated username is essential for your investigation.
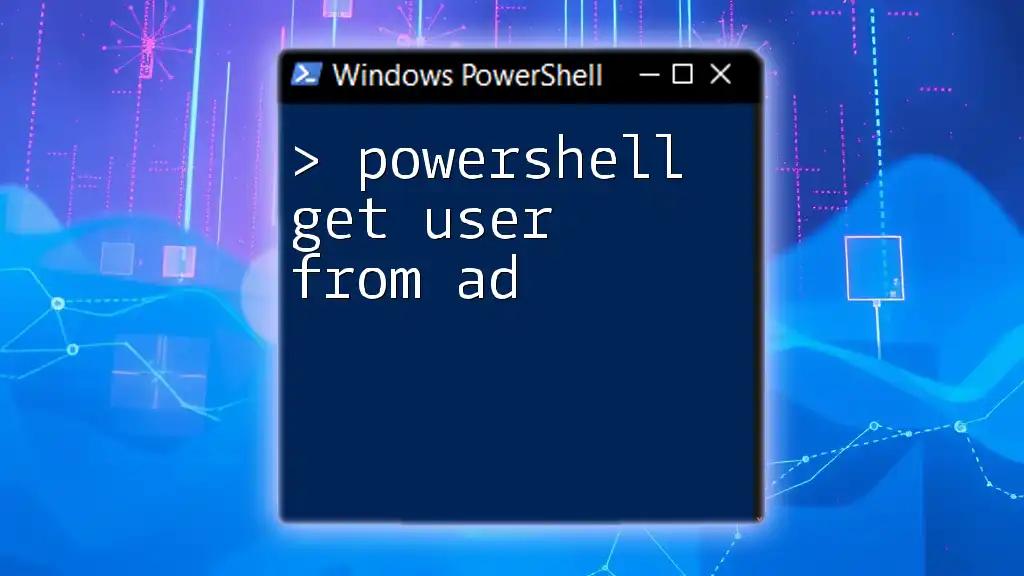
Getting Started with PowerShell
Introduction to PowerShell
PowerShell is a robust task automation and configuration management framework from Microsoft. It consists of a command-line shell and an associated scripting language, built on the .NET framework. PowerShell enables administrators to manage the operating system, automate tasks, and retrieve information with ease through cmdlets (commands built into PowerShell).
Setting Up Your Environment
Before you can start using PowerShell to get a username from a SID, ensure you have the necessary permissions to access and execute commands. You can open PowerShell through the Windows Terminal or PowerShell ISE (Integrated Scripting Environment).
Make sure you are running PowerShell with administrator privileges if you intend to work with system accounts or access secure information.

Methods to Get Username from SID in PowerShell
Using the `System.Security.Principal.SecurityIdentifier` Class
One straightforward method to retrieve a username from a SID is by utilizing the .NET class `System.Security.Principal.SecurityIdentifier`. This method effectively translates a SID to its corresponding username.
Here’s how you can do this:
$sid = "S-1-5-21-1234567890-1234567890-1234567890-1001"
$identity = New-Object System.Security.Principal.SecurityIdentifier($sid)
$username = $identity.Translate([System.Security.Principal.NTAccount])
Write-Output $username.Value
In this snippet:
- The SID is defined as a string.
- A new object of the `SecurityIdentifier` class is created using the SID.
- The `Translate` method converts the SID into an NT account format, which is more easily readable as a username. The result is then printed out.
Using the `Get-WmiObject` Cmdlet
Another method to derive the username from a SID is through the `Get-WmiObject` cmdlet, which enables you to query Windows Management Instrumentation (WMI) data. This approach is effective for local accounts.
You can use the following code snippet:
$sid = "S-1-5-21-1234567890-1234567890-1234567890-1001"
$user = Get-WmiObject Win32_UserAccount | Where-Object { $_.SID -eq $sid }
Write-Output $user.Name
In this example:
- The SID is set as a string.
- The `Get-WmiObject` cmdlet retrieves user accounts from the system.
- The `Where-Object` filter checks for matching SIDs and outputs the username related to that SID.
Using the `System.DirectoryServices` Namespace
For a more comprehensive approach, especially in domain environments, you can use the `System.DirectoryServices` namespace to query Active Directory (AD) for user accounts associated with SIDs.
Here’s a sample code snippet:
$sid = "S-1-5-21-1234567890-1234567890-1234567890-1001"
$user = New-Object System.DirectoryServices.DirectorySearcher
$user.Filter = "(&(objectCategory=person)(objectClass=user)(samAccountType=805306368)(securityIdentifier=$sid))"
$result = $user.FindOne()
Write-Output $result.Properties["sAMAccountName"]
This code:
- Initializes a new `DirectorySearcher` object to query Active Directory.
- Sets a filter that looks for user objects with the specified SID.
- The `FindOne` method retrieves the first matching user, and the username is displayed via the `Properties` property.

Best Practices When Working with SIDs
Validating SIDs
Before attempting to retrieve usernames, it’s wise to ensure that the SID is valid. Validating prevents errors and unexpected results during your script execution. Below is a simple function to validate SIDs:
function Test-SID {
param (
[string]$sid
)
try {
$identity = New-Object System.Security.Principal.SecurityIdentifier($sid)
return $true
} catch {
return $false
}
}
This function:
- Takes a SID as a parameter.
- Attempts to create a `SecurityIdentifier` object. If it succeeds, the SID is valid; if it fails, it indicates that the SID is invalid.
Handling Errors and Exceptions
When working with SIDs and PowerShell, you will encounter various scenarios that might lead to errors, especially if a SID does not correspond to an existing entity. Implement proper error handling practices by using try-catch blocks.
For instance, if you’re using `Get-WmiObject`, you can check for null results and provide informative messages to help troubleshoot issues.

Additional Resources
Documentation and References
For further reading, refer to the official Microsoft documentation on the System.Security.Principal.SecurityIdentifier class and WMI. This documentation provides deeper insights and additional examples.
PowerShell Community and Forums
Engaging with the PowerShell community can be incredibly beneficial. Platforms like Stack Overflow, Reddit, and Discord servers dedicated to PowerShell can help you troubleshoot problems, share knowledge, and learn best practices.

Conclusion
In summary, retrieving a username from a SID can be accomplished through various methods in PowerShell, such as using the `System.Security.Principal.SecurityIdentifier` class, WMI, or the `System.DirectoryServices` namespace. Each method has its use cases, especially depending on whether you're operating in a local or domain environment. With the information and examples provided in this guide, you now have the tools to effectively perform this task.
Practice using these commands and integrate them into your regular PowerShell usage to enhance your administrative capabilities. As you become more proficient, consider exploring further topics in PowerShell and how they can benefit your workflows.