To extract the filename from a given path in PowerShell, you can use the `Split-Path` cmdlet with the `-Leaf` parameter, which returns just the file name portion of the path. Here's a code snippet to illustrate this:
$path = 'C:\Folder\Subfolder\example.txt'
$filename = Split-Path -Path $path -Leaf
Write-Host $filename
Understanding File Paths
What Is a File Path?
A file path is a string that provides the location of a file or directory in a file system. Understanding how to navigate file paths is crucial for effective scripting, especially when using PowerShell.
File paths can be categorized into two types:
-
Absolute Paths: These paths provide the complete location of a file from the root of the file system. For example, `C:\Folder\Subfolder\File.txt` is an absolute path, as it starts from the drive letter.
-
Relative Paths: These paths are relative to the current directory. For example, if you're currently in `C:\Folder\`, the relative path to `File.txt` would simply be `Subfolder\File.txt`.
Components of a File Path
A file path consists of several components:
- Drive Letter: Indicates the storage device (e.g., `C:\`).
- Folder Names: The directories leading to the file (e.g., `Folder\Subfolder`).
- Filename: The actual name of the file (e.g., `File.txt`).
- File Extension: Suffix indicating the file type (e.g., `.txt`, `.jpg`).
Understanding these components helps you manipulate files effectively with PowerShell.
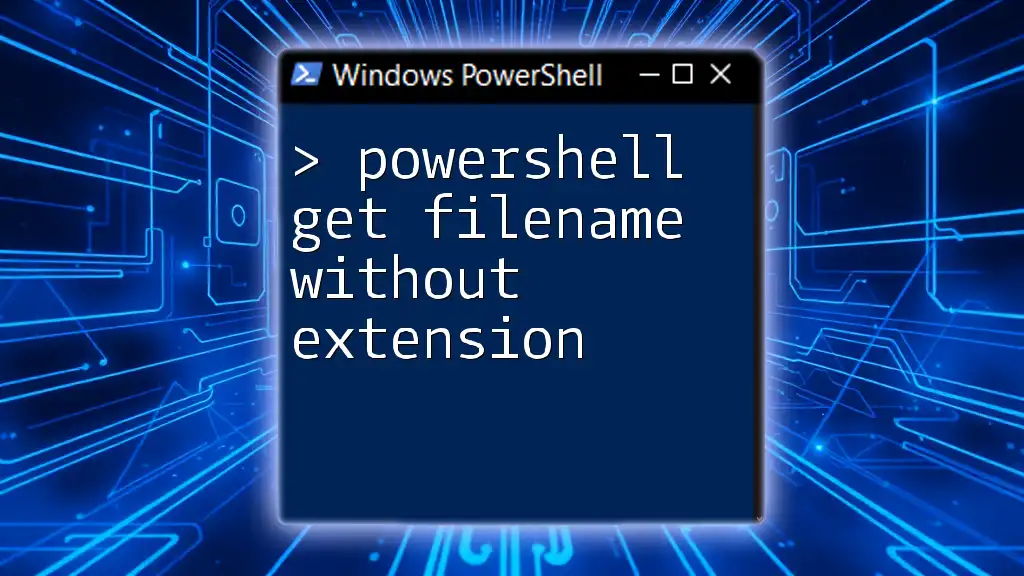
PowerShell Get Filename
Why Use PowerShell for Filename Extraction?
PowerShell enables users to perform file management tasks quickly and efficiently. By leveraging built-in cmdlets, you can automate the process of extracting filenames from paths, which is especially beneficial when dealing with large datasets or performing repetitive tasks.
Basic Syntax of File Path Commands
In PowerShell, certain cmdlets are essential for file manipulation:
- Get-Item: Retrieves the item at the specified location in the file system.
- Split-Path: Splits a file path into its components, allowing you to isolate the filename easily.
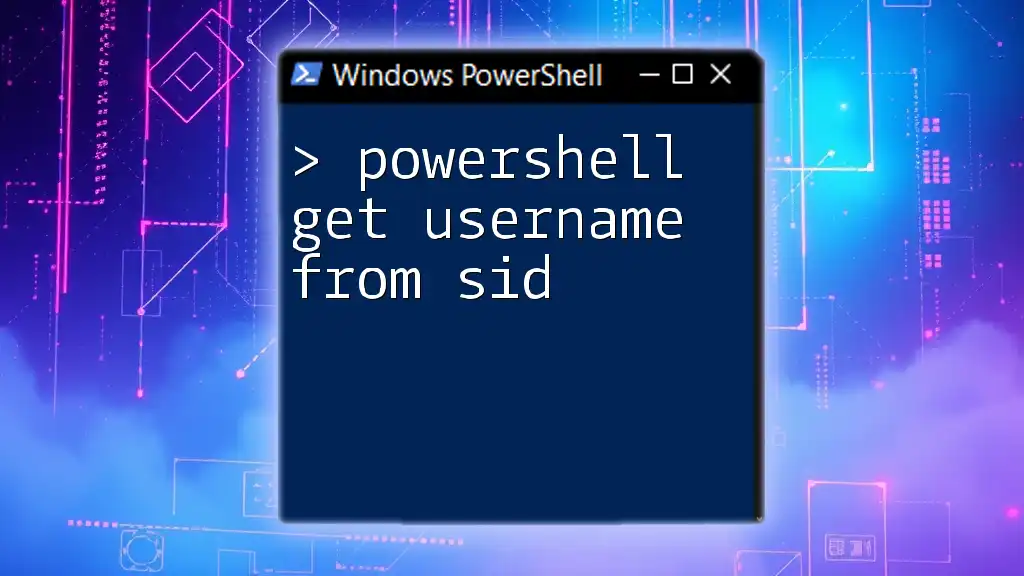
Getting a Filename from a Path in PowerShell
Using Split-Path Cmdlet
What is Split-Path?
`Split-Path` is a powerful cmdlet used to break down file paths into their respective components. Its main purpose is to allow users to isolate specific parts of the path, including the filename.
Syntax of Split-Path
To extract just the filename from a path, you can use the `-Leaf` parameter. Here’s how to do it:
Split-Path -Path "C:\Folder\Subfolder\File.txt" -Leaf
Example of Extracting Filename
The above command will return:
File.txt
This simple command effectively extracts the filename, stripping away the rest of the path. This is useful in scripts where filenames need to be logged or processed.
Using Get-Item with Select-Object
What is Get-Item?
`Get-Item` retrieves an item at a specified path and allows you to access its properties, including the file’s name.
Combining Get-Item with Select-Object
You can combine `Get-Item` with `Select-Object` to access the filename directly:
(Get-Item "C:\Folder\Subfolder\File.txt").Name
Example Usage
When you run this command, it will return:
File.txt
This method provides a straightforward way to obtain the filename without the surrounding directory structure, perfect for logging or processing tasks.
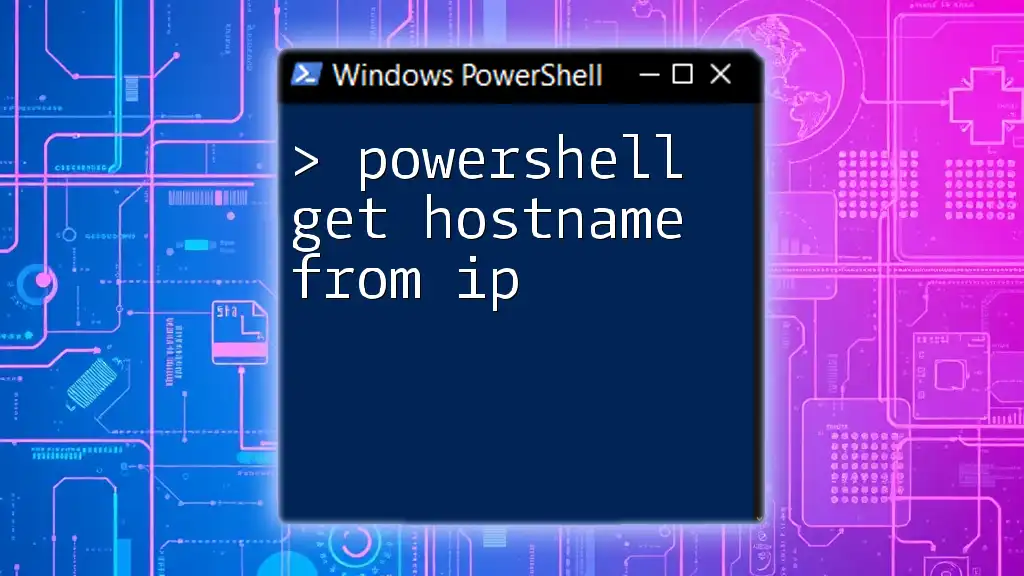
Advanced Techniques for Filename Extraction
Handling Multiple Files
Using Get-ChildItem for Multiple Files
When you need to retrieve the filenames of multiple files within a folder, `Get-ChildItem` is your go-to cmdlet. It fetches all files in the specified directory. For example:
Get-ChildItem "C:\Folder\Subfolder\*" | Select-Object -ExpandProperty Name
This command will list all filenames in the specified folder without the path.
Understanding the Output and Use Cases
If the folder contains `File1.txt`, `File2.txt`, and `File3.txt`, the output will be:
File1.txt
File2.txt
File3.txt
This is particularly useful for file management tasks where you might need to loop through a list of files for processing or analysis.
Error Handling and Validations
Dealing with Invalid Paths
When your scripts deal with file operations, it’s essential to check the existence of paths to prevent errors. You can use `Test-Path` to verify if a path exists before performing operations:
if (Test-Path "C:\Folder\Subfolder\File.txt") {
# proceed with Split-Path
} else {
Write-Host "The file does not exist."
}
Best Practices for Error Handling
Incorporating error handling into your scripts enhances user experience. Always provide user-friendly messages or logs to address issues like non-existent paths, helping users troubleshoot easily.
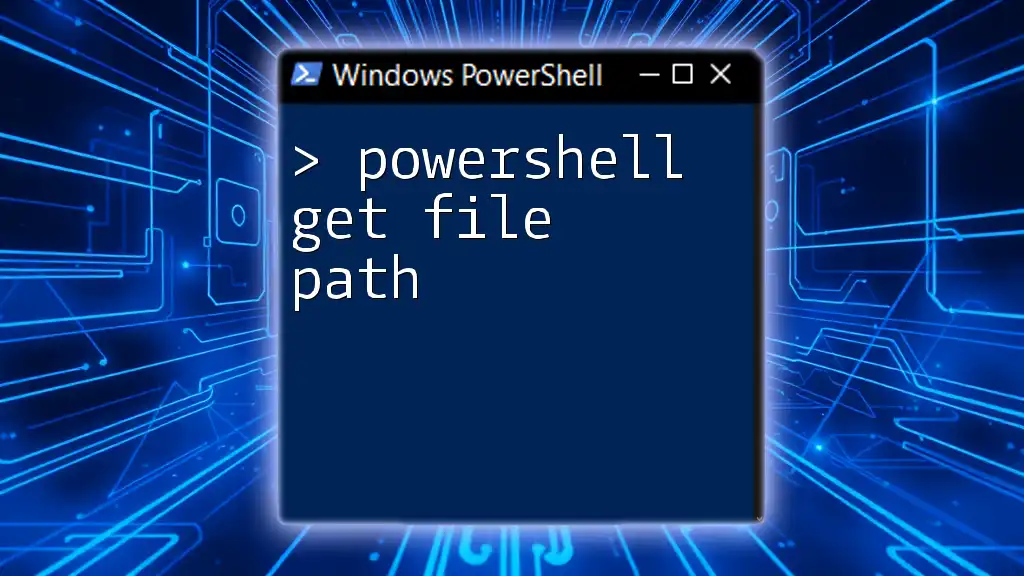
Conclusion
Extracting filenames from paths in PowerShell is a straightforward process that can significantly enhance your productivity. By using cmdlets like `Split-Path` and `Get-Item`, you can streamline your file management tasks with ease.
Understanding these techniques not only empowers you to handle files efficiently but also prepares you for more complex scripting tasks.
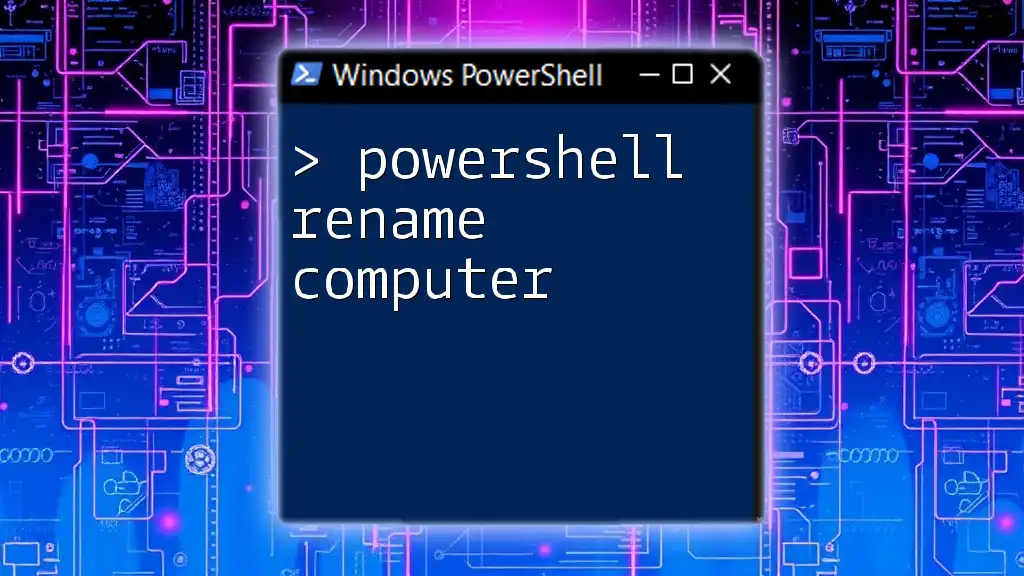
Call to Action
If you found this guide helpful, consider following our company for more tips and tricks on PowerShell commands. We invite you to share your experiences with extracting filenames using PowerShell in the comments below!
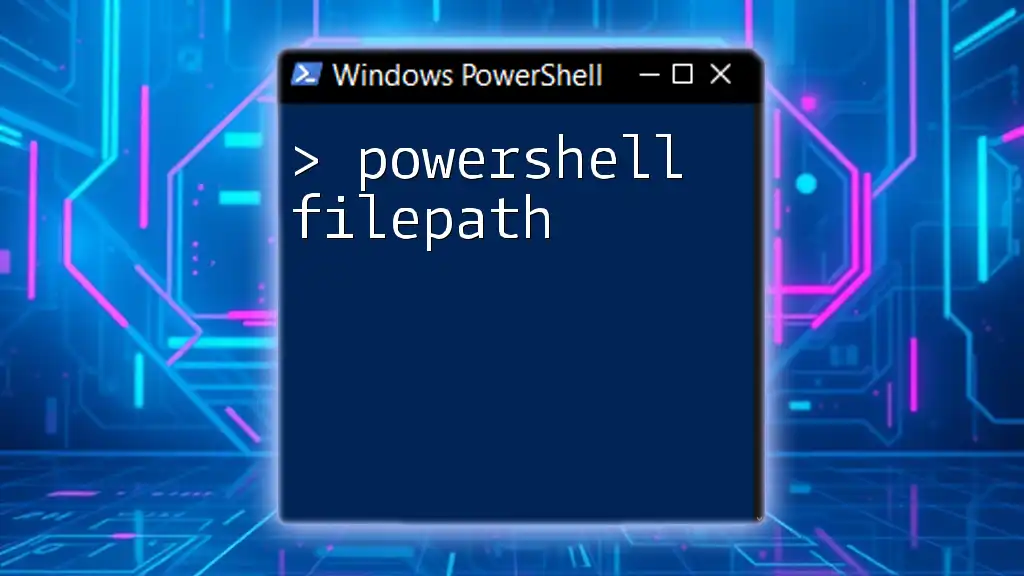
Additional Resources
For further learning, refer to the official PowerShell documentation, where you'll find extensive information about cmdlets and their functionalities. Additionally, consider exploring recommended PowerShell resources to deepen your understanding and improve your scripting skills.