To retrieve system variables in PowerShell, you can use the `Get-ChildItem` cmdlet to access the environment variables stored in the `Env:` drive.
Get-ChildItem Env:
What are System Variables?
System variables are critical in defining how an operating system and applications interact with the environment in which they run. They hold various configuration details such as paths to installation directories, user settings, and environmental data that software may need to function correctly.
Types of System Variables
System variables can primarily be categorized into two main types:
-
Environment Variables: These variables are available system-wide and can be accessed by all processes. They typically hold information like the system path or user profile directories.
-
PowerShell Variables: These are specific to the PowerShell session and include data that a user or script defines. They do not persist between sessions unless explicitly saved.
Commonly used system variables in Windows include `%PATH%`, `%TEMP%`, and `%USERPROFILE%`, each playing a crucial role in system operation and application behavior.
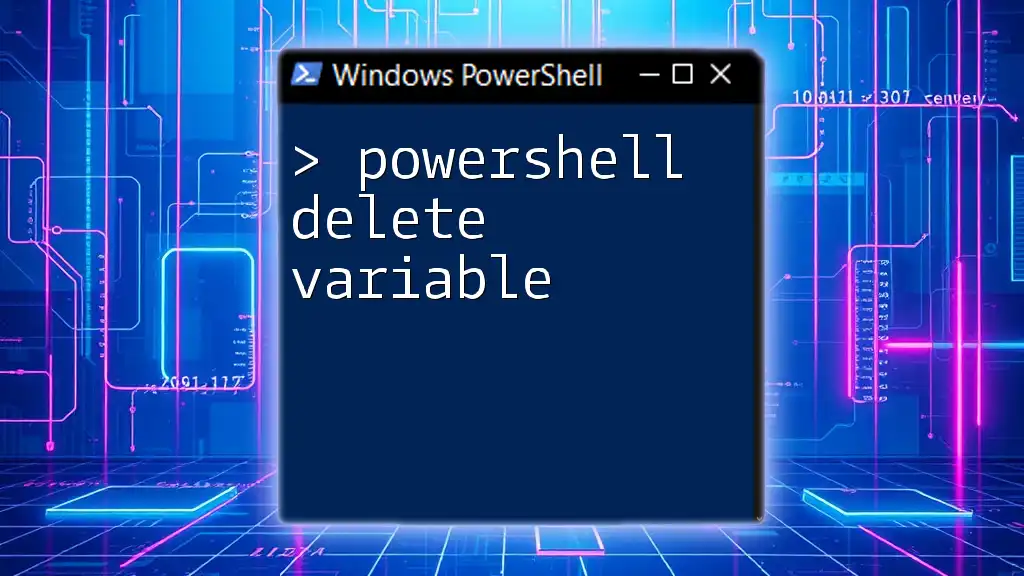
Accessing System Variables with PowerShell
Using the Get-ChildItem Cmdlet
One of the simplest ways to access system variables in PowerShell is through the `Get-ChildItem` cmdlet. When used with the `Env:` provider, it allows you to view all environment variables currently set in your session.
To list all environment variables, you can run the following command:
Get-ChildItem Env:
This command will display an overview of all environment variables, their names, and their respective values. Understanding this output is essential; for instance, the `%PATH%` variable shows the directories PowerShell or other applications will search for executable files.
Using the [System.Environment] Class
An alternative approach to accessing system variables involves utilizing the .NET `System.Environment` class. This method provides a more structured way to retrieve system variables and can return them in a different format.
To get all environment variables, use:
[System.Environment]::GetEnvironmentVariables()
This command retrieves a collection of environment variables and displays them in a key-value format, where each key represents the variable's name and its corresponding value. Comparing the outputs of `Get-ChildItem` and the `System.Environment` call reveals varying structures, providing flexibility depending on user preference.
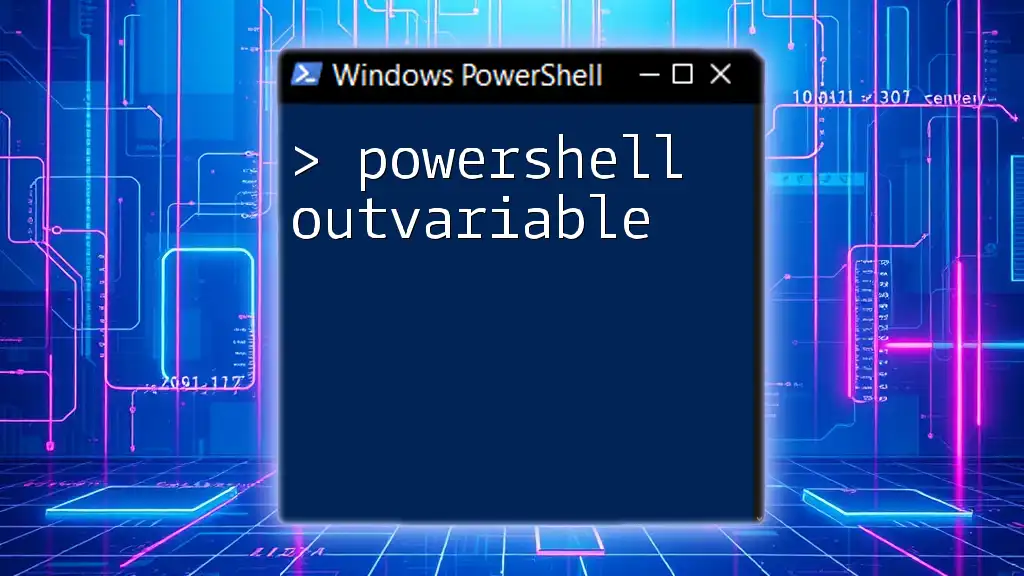
Filtering and Finding Specific System Variables
Using Where-Object for Filtering
When you need to focus on specific system variables, using the `Where-Object` cmdlet is invaluable. This cmdlet allows you to refine your search dynamically.
For example, to search for all variables containing "PATH", execute:
Get-ChildItem Env: | Where-Object {$_.Name -like '*PATH*'}
This command filters the environment variables and returns only those whose names include "PATH", making it significantly easier to locate critical variables amidst many others. The ability to filter enhances your PowerShell productivity, especially when dealing with numerous system variables.
Retrieving a Single System Variable
If you wish to retrieve a specific system variable, access it directly using the `$env:` drive:
$env:PATH
This command provides an immediate value of the `PATH` variable, which is fundamental for any applications needing access to specific executable locations within the system. Understanding the significance of this variable aids in troubleshooting software issues related to path configurations.
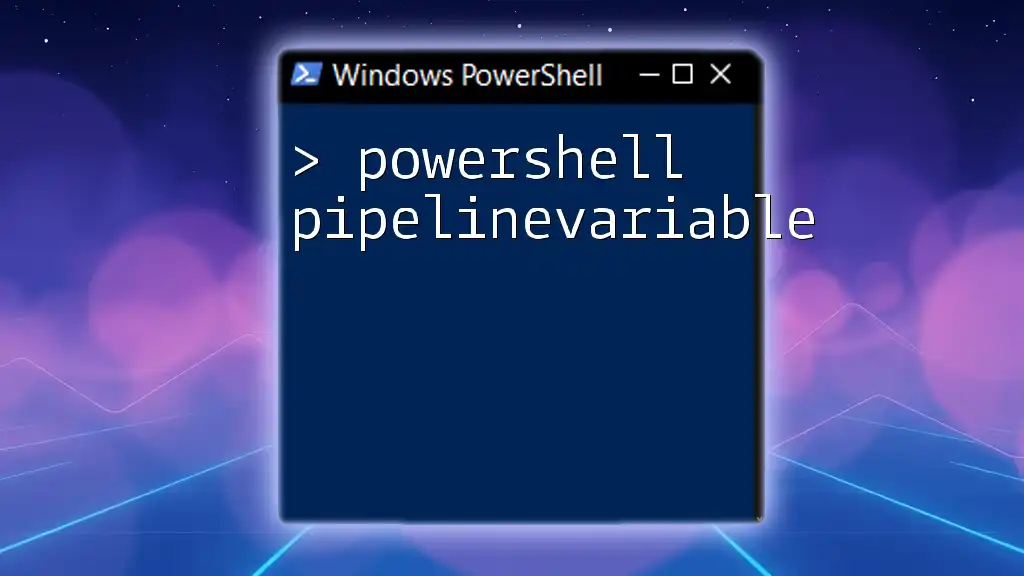
Setting and Modifying System Variables
Creating a New System Variable
PowerShell enables you to create new environment variables within your system. This is especially useful for custom configurations that third-party applications might rely on.
To create a new variable for the user profile, use:
[System.Environment]::SetEnvironmentVariable('MyVariable', 'Value', 'User')
This command sets `MyVariable` to the value `Value`. The 'User' scope ensures that the variable is accessible only to the current user, preserving system integrity without impacting other profiles.
Modifying Existing System Variables
Updating existing system variables is equally straightforward. For example, if you want to modify the value of `MyVariable`, execute:
[System.Environment]::SetEnvironmentVariable('MyVariable', 'NewValue', 'User')
This action overwrites the previous value of `MyVariable`, showcasing how PowerShell can adapt your environment dynamically to meet changing application needs. Always exercise caution when modifying variables to avoid unintentional disruptions.
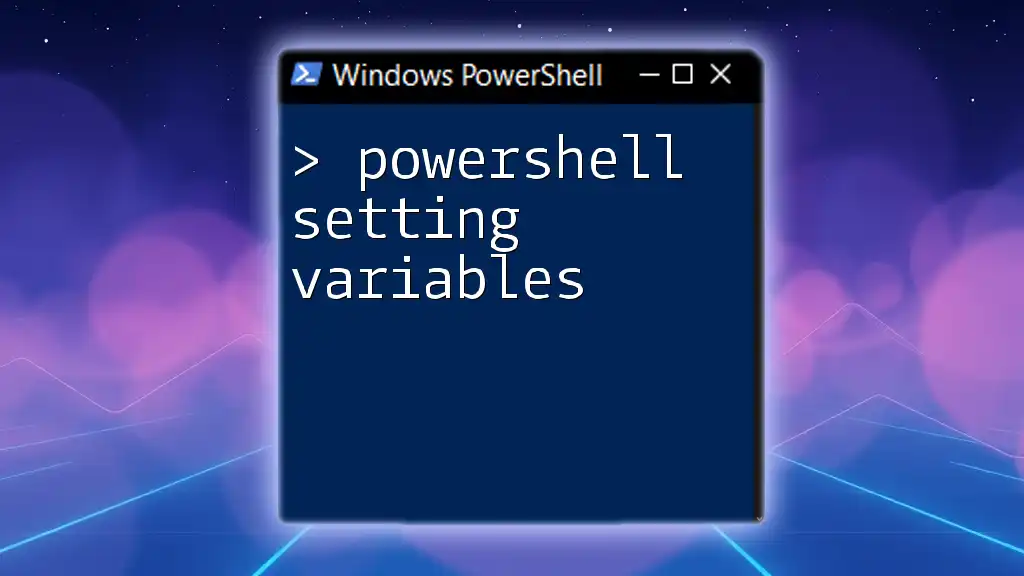
Removing System Variables
Deleting a System Variable
If you need to remove an environment variable, PowerShell allows you to do so easily. To delete `MyVariable`, use:
[System.Environment]::SetEnvironmentVariable('MyVariable', $null, 'User')
Setting the variable's value to `$null` indicates that it should be deleted from the environment. Always double-check which variable you are removing to prevent loss of critical configuration.
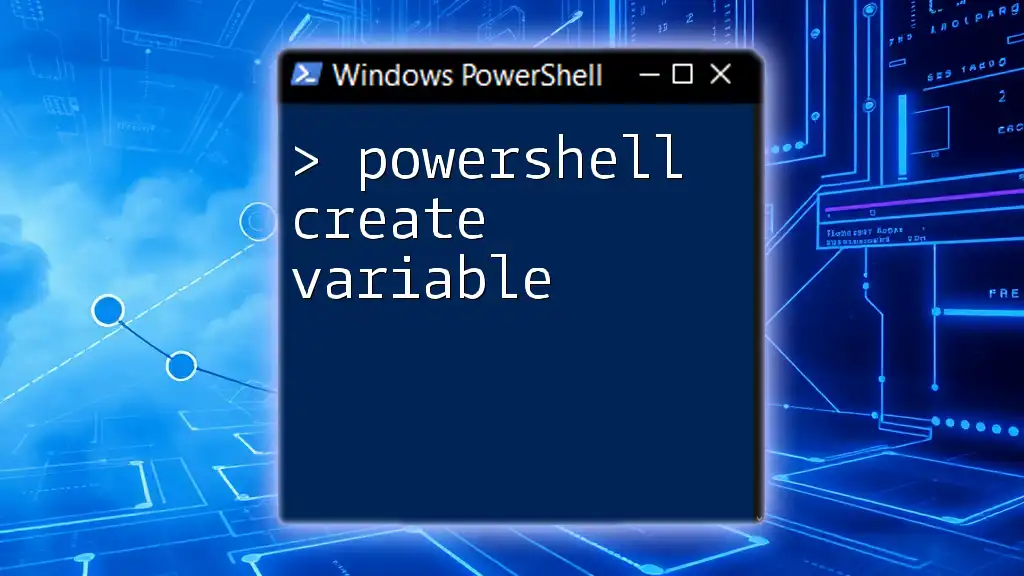
Best Practices and Tips
How to List All Available Variables Efficiently
Managing a large set of environment variables can be a challenge. Utilizing concise commands helps keep track of available variables efficiently. Regularly listing available variables, especially after making adjustments, is a best practice that fosters organizational consistency.
Script Automation for Managing Variables
Writing scripts to automate variable management tasks can save considerable time, especially over larger systems. By retaining core commands in reusable scripts, you streamline processes that may otherwise require repetitive manual input.
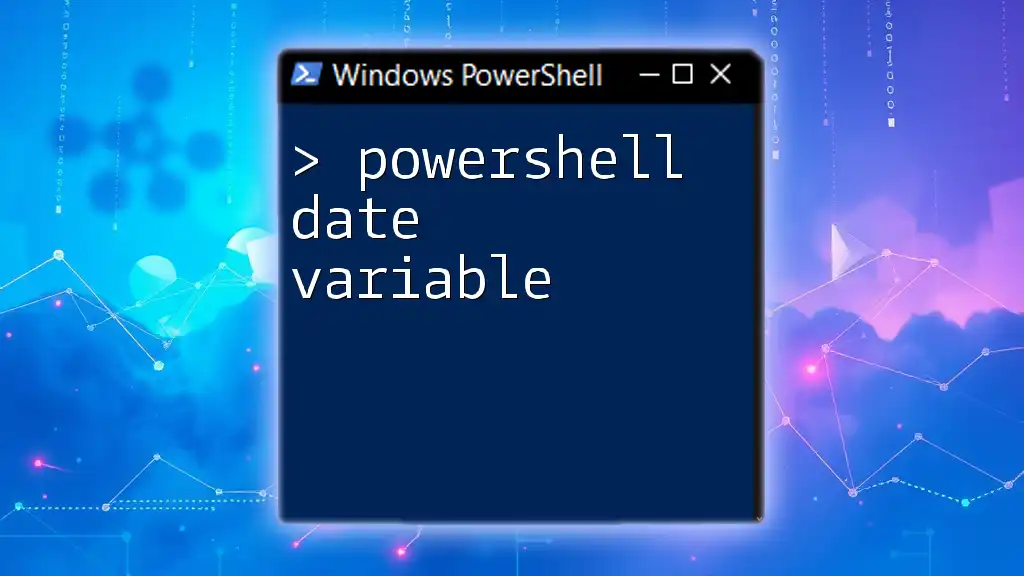
Common Use Cases for System Variables
Development Scenarios
For developers, comprehending system variables is crucial. These variables often determine paths for libraries, configuration files, and executable binaries. For instance, adapting the `PATH` variable facilitates seamless execution of scripts written in various programming languages without encountering file path issues.
System Administration Tasks
System administrators frequently utilize system variables to configure user settings and environment parameters for servers and workstations. By managing these variables effectively, administrators can standardize software behavior across numerous systems.
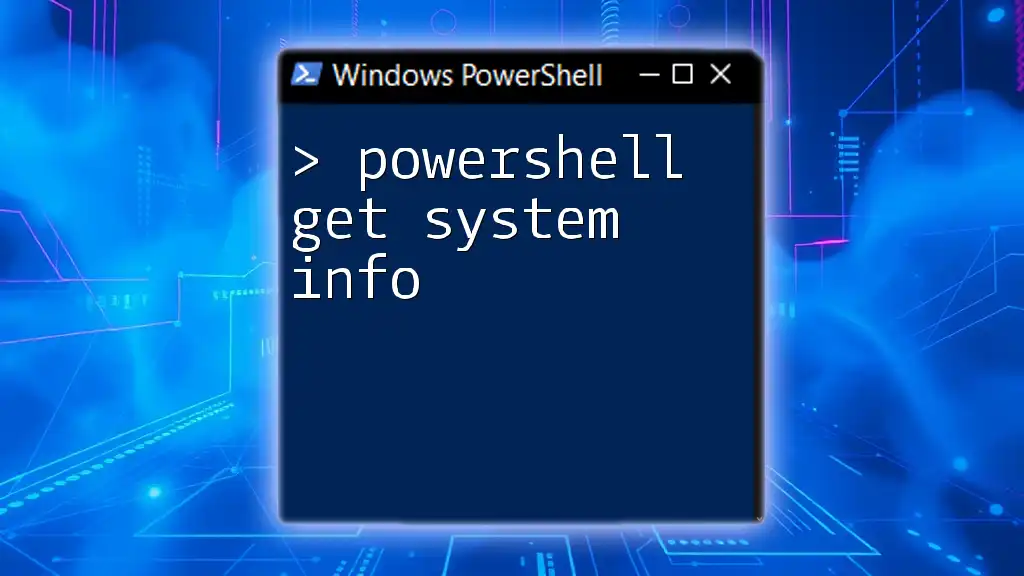
Troubleshooting Common Issues
Accessing Variables
Occasionally, users encounter access errors when attempting to read or modify environment variables. Common errors include permission issues or typos. It's invaluable to ensure that PowerShell sessions run with appropriate permissions, particularly when modifying system-level variables.
Persistent Changes
Changes to environment variables may not take immediate effect in all applications. Users must often restart their terminal or application to ensure that new settings are recognized. Familiarity with how various applications handle environment variables improves troubleshooting efficiency during variable modification processes.
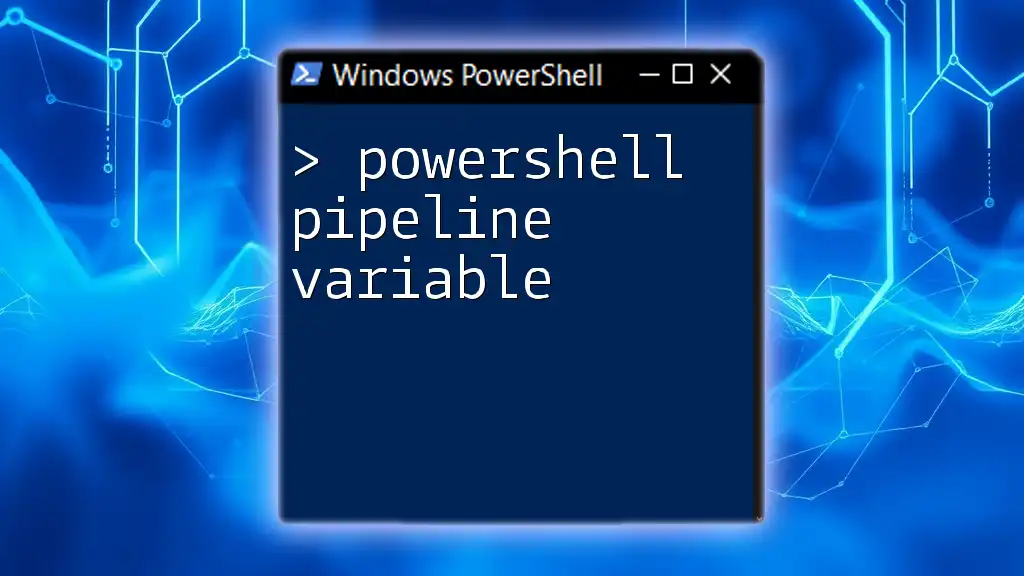
Conclusion
PowerShell provides robust tools for accessing and managing system variables, making it an essential skill for anyone looking to enhance their computing workflows. By understanding how to retrieve, create, modify, and delete these variables, users can significantly streamline their processes. Experimenting further with PowerShell commands related to system variables will deepen your expertise and enhance your productivity in any technical environment.
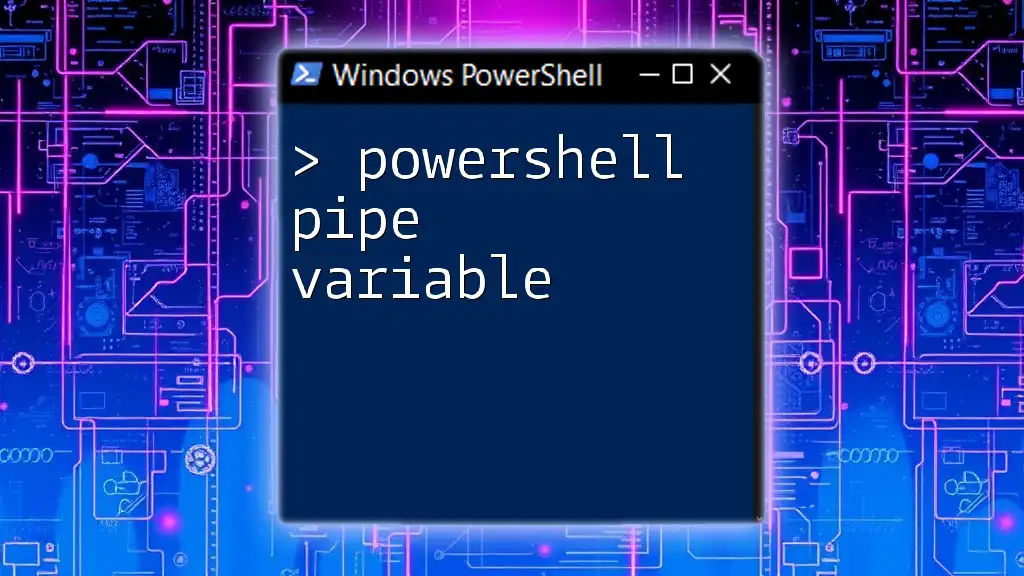
Additional Resources
- Links to Official Documentation: Explore the [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/) for more in-depth information on cmdlets and environment variables.
- Recommendations for Practice Scripts: Experiment with various commands discussed here by creating and running your own scripts. This practical approach will bolster your understanding and command of PowerShell.