In PowerShell, regex escape sequences allow you to treat special characters literally, enabling you to match them in your regular expressions.
Here’s a code snippet demonstrating how to escape a regex special character, such as a period (`.`):
# Using regex to match a literal period
$pattern = '\.'
$string = 'This is a sentence with a period.'
if ($string -match $pattern) {
Write-Host 'Match found!'
} else {
Write-Host 'No match.'
}
Introduction to Regex in PowerShell
What is Regex?
Regular Expressions (Regex) are powerful tools for string matching and manipulation. They provide a means to specify patterns in text, allowing developers to search, validate, and replace text efficiently. In PowerShell, Regex is commonly used for tasks such as filtering output, validating formats, and parsing strings.
Why Escaping is Important
Within the context of Regex, some characters hold special meanings, which means they function differently than you might expect. If you want to match these special characters literally, you need to escape them. Failing to do so can lead to errors, unexpected behavior, or incorrect results. By mastering the concept of escaping in PowerShell Regex, you'll avoid common pitfalls and enhance your scripts' reliability.
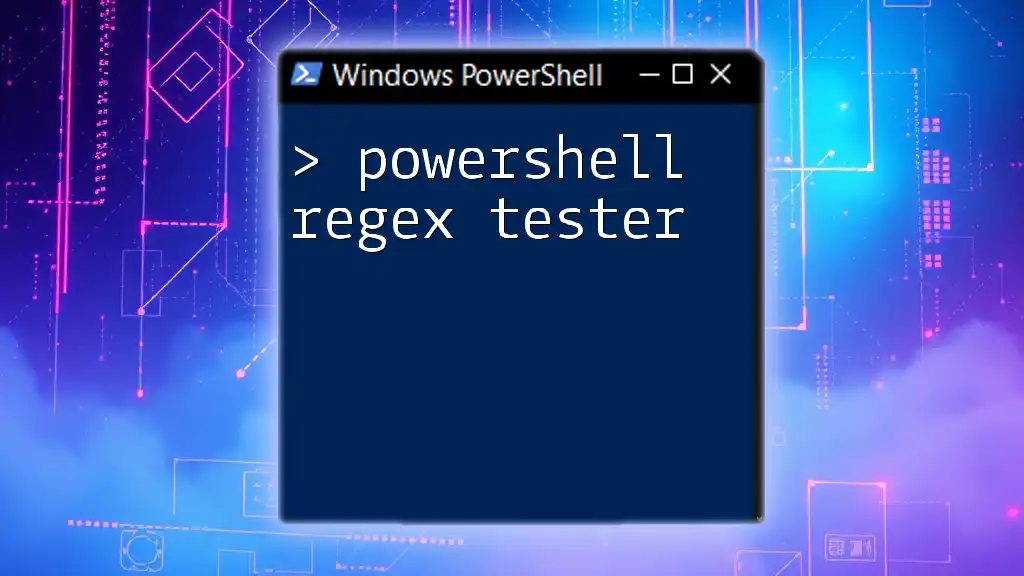
Essentials of Escaping Characters
What Needs Escaping?
PowerShell Regex requires you to escape certain special characters to match them as literals. The characters that typically need escaping include:
- `.` (period): Matches any character
- `*` (asterisk): Matches zero or more occurrences of the previous character
- `?` (question mark): Matches zero or one occurrence of the previous character
- `+` (plus): Matches one or more occurrences
- `[` and `]`: Define a character class
- `(` and `)`: Create capture groups
- `{` and `}`: Define occurrence quantifiers
- `|` (pipe): Acts as a logical OR
- `\` (backslash): The escape character itself
Understanding how each character impacts Regex functionality is essential for effective string parsing.
The Escape Character in PowerShell
In PowerShell, the backslash (`\`) serves as the primary escape character. If you intend to match a special character, prepend it with a backslash. In some cases, particularly when strings are housed within quotes, you may need to escape the backslash itself—this is known as double escaping (i.e., using `\\`).
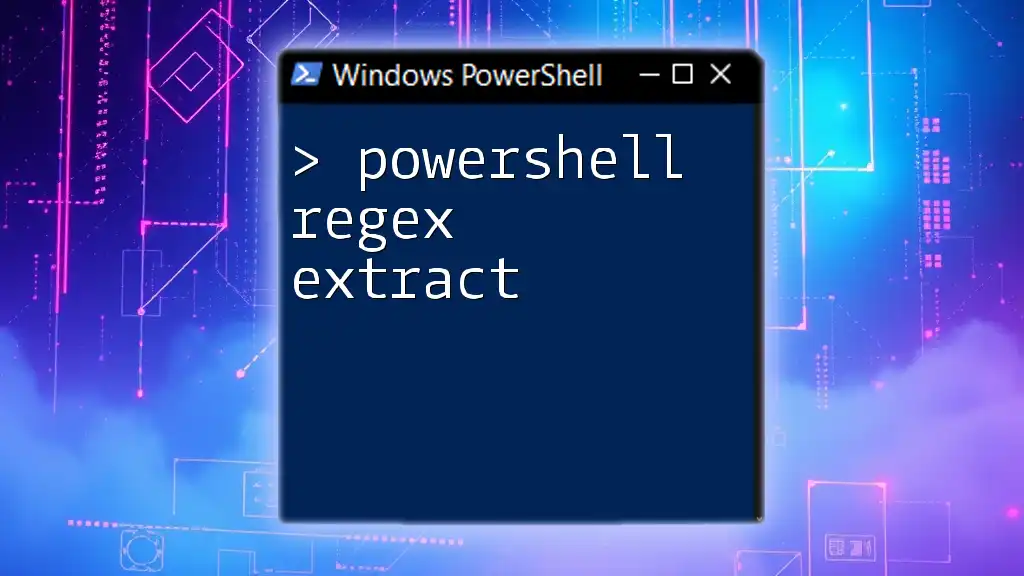
PowerShell Syntax for Regex
Basic Syntax
PowerShell allows you to utilize Regex with the `-match` and `-replace` operators. The `-match` operator returns a Boolean indicating whether a match was found, while `-replace` allows for substitutions. For instance, consider this simple usage of `-match` without any escaping:
$string = "Hello World!"
if ($string -match "World") { "Match found!" }
In this case, the term "World" is matched directly. However, if we wanted to match "Hello" while ensuring the period that follows is treated literally, we would need to escape it:
Using Escape Sequences
Here’s how to appeal to the literal period:
$string = "Hello. Do you see the dot?"
if ($string -match "Hello\.") { "Match found!" }
By escaping the period with a backslash, you ensure that only the literal "Hello." is matched.
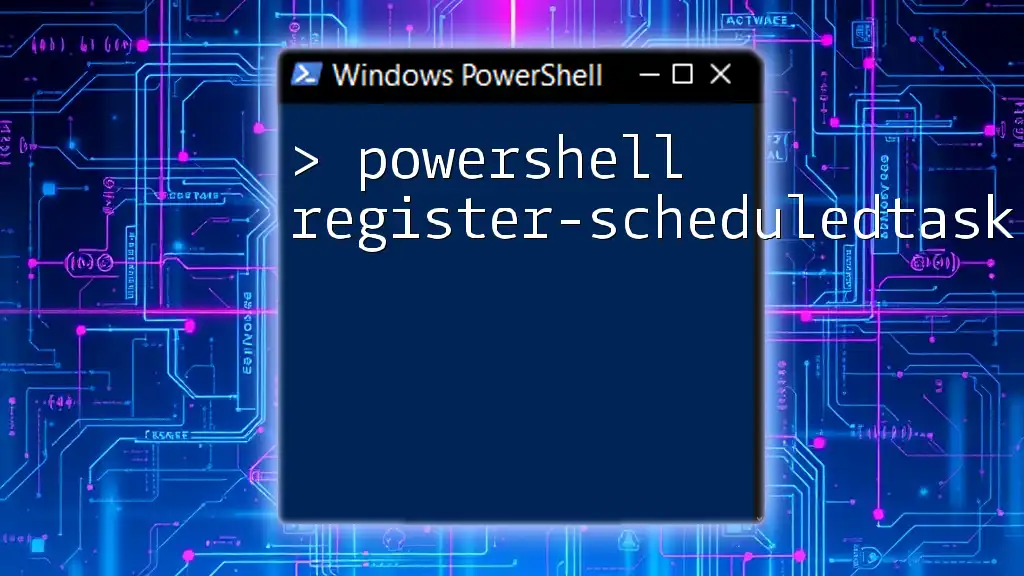
Practical Examples of Escaping in PowerShell
Matching File Patterns
When working with file paths in PowerShell, it is common to face scenarios where you need to escape backslashes. For example:
$filePath = "C:\Program Files\MyApp\file.txt"
if ($filePath -match "C:\\Program Files\\MyApp\\file\.txt") { "File found!" }
This code snippet illustrates how to effectively escape backslashes in file paths, allowing for precise matching.
Finding Specific Characters
Suppose you need to find the presence of a question mark in a string; you must escape it, as follows:
$text = "Is it working?"
if ($text -match "Is it working\?") { "Exact match!" }
Here, escaping the question mark allows you to perform an exact match, as opposed to matching zero or one occurrence.
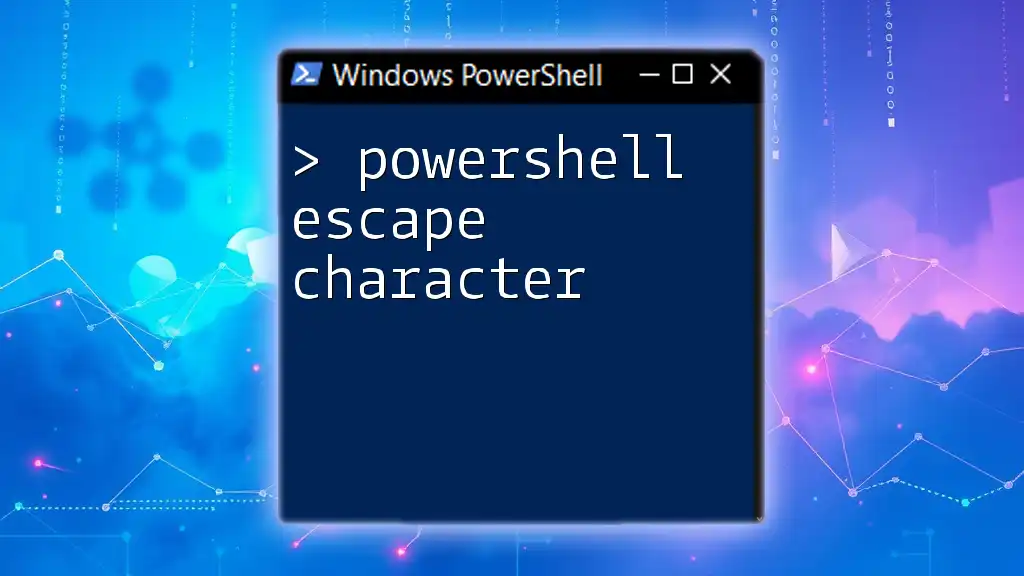
Advanced Techniques for Regex Escaping
Using Double Quotes vs Single Quotes
It’s crucial to understand the difference between single and double quotes in PowerShell. While single quotes treat everything inside them as a literal string, double quotes allow for variable interpolation. Here’s an example demonstrating this:
$patternSingle = 'Hello\.World' // single quotes
$patternDouble = "Hello\.World" // double quotes
In the first pattern, the period is treated literally due to single quotes. In the second, the escape does not apply as expected due to double quotes not interpreting the backslash correctly unless formatted adequately within a string.
Combining Escaping with Other Regex Techniques
Escape sequences can be combined with anchors like `^` and `$` to denote start and end of strings, respectively. For example:
$string = "Hello at the start!"
if ($string -match "^Hello") { "Start match!" }
In this example, we successfully match the string "Hello" at the start using the caret (^) as an anchor, whereas the need for escaping is critical if Regex is combined with special characters.
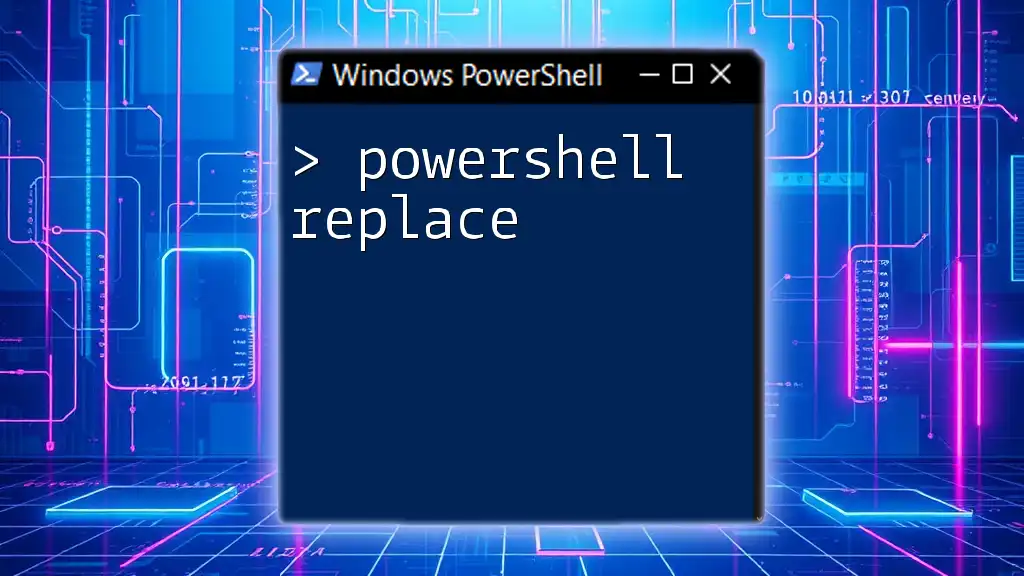
Common Mistakes and Troubleshooting
Frequent Errors in Regex Usage
When dealing with PowerShell Regex, misunderstandings about which characters require escaping can lead to frustrating issues. It's common for beginners to overlook the need to escape characters like `|` or `+`, resulting in unintended matches or Regex failures.
Using `Try/Catch` for Safe Execution
To handle errors gracefully, especially when working with user input or unverified strings, you can implement `try/catch` blocks around your Regex operations, like so:
try {
if ($string -match "Unescaped\|Character") { "Matched!" }
} catch {
Write-Host "An error occurred: $_"
}
This ensures that if your Regex fails, you can catch the error and handle it without crashing your script.
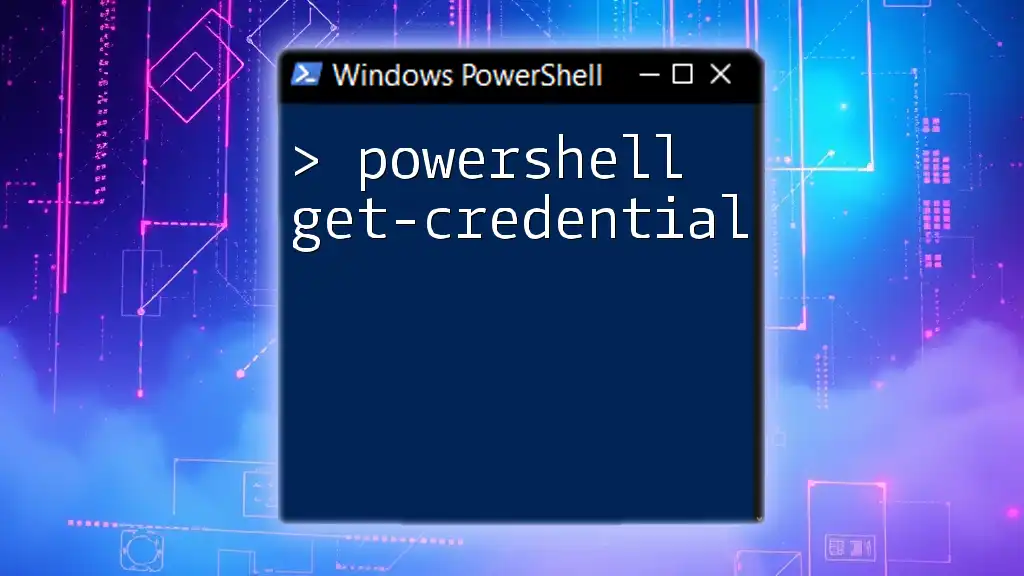
Conclusion
Recap of the Importance of Escaping in Regex
As you have learned, mastering the concept of escaping in PowerShell Regex is crucial for achieving accurate matches and manipulating strings effectively. By understanding what needs escaping and applying it properly, you'll significantly improve the performance and reliability of your PowerShell scripts.
Encouragement for Practical Application
Don’t hesitate to practice your Regex skills regularly. Use different scenarios and explore how escaping works with various strings. The more you use it, the more comfortable you will become, ultimately enhancing your PowerShell proficiency.
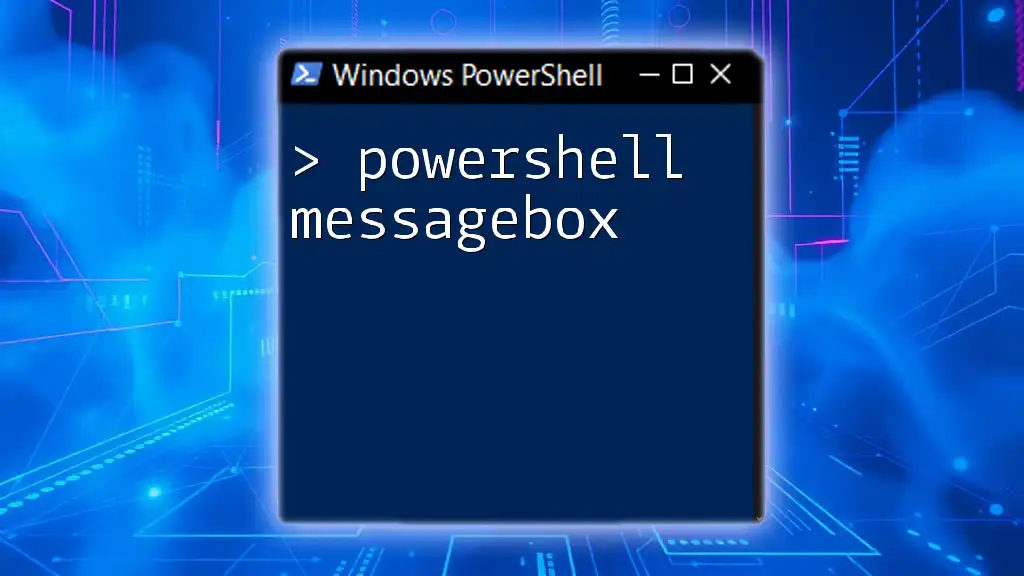
Additional Resources
Further Reading on PowerShell and Regex
For more in-depth knowledge, consider checking official PowerShell documentation or specialized Regex topics available online.
Useful Online Regex Tools and Cheat Sheets
Many online resources and tools can help you test and experiment with Regex patterns effectively, making your learning journey smoother and more interactive.