PowerShell regions are a way to organize and collapse blocks of code for better readability and manageability within scripts.
Here’s a code snippet demonstrating how to define a region in PowerShell:
# region Greeting Function
function Get-Greeting {
Write-Host 'Hello, World!'
}
# endregion
What Are PowerShell Regions?
PowerShell regions are a feature that allows developers to group blocks of code together, making scripts easier to read and manage. The primary purpose of regions is to enhance code organization, particularly in larger scripts where multiple functionalities are implemented. By using regions, you can segment your code logically, which minimizes clutter and aids in navigation.
When you define a region, you effectively create a collapsible section that can hide or show its contents, allowing developers to focus on specific parts of their script. This can be particularly useful during the development and debugging phases, where isolating pieces of code can help in troubleshooting and optimization.
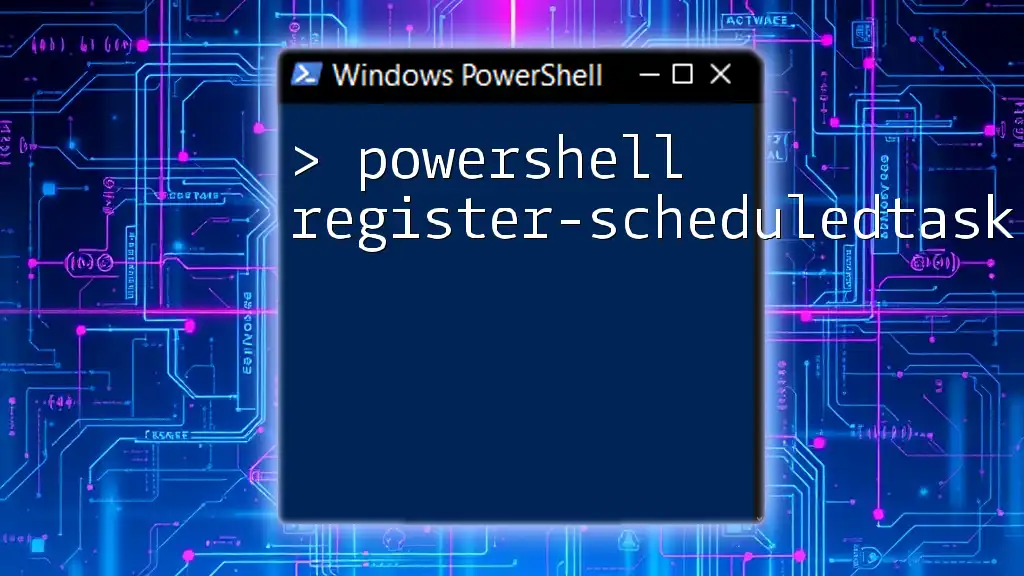
How to Create Regions
Basic Syntax of Regions
Creating regions in PowerShell is straightforward. You simply use the `#region` directive followed by the name of the region, and then you close it with the `#endregion` directive. This syntax is designed for readability and is easy to remember.
#region MyRegion
# Your code goes here
#endregion
Example of Creating a Region
To illustrate how to effectively use regions, consider the following example where various functionalities are organized into regions. This not only improves readability but allows developers to locate specific code sections quickly.
#region User Management
function Create-User {
# Code for creating a user
}
function Remove-User {
# Code for removing a user
}
#endregion
#region Report Generation
function Generate-Report {
# Code for generating report
}
#endregion
In this example, the script is divided into two clear regions: User Management and Report Generation. Each section is dedicated to a specific task, making the overall code easier to follow.
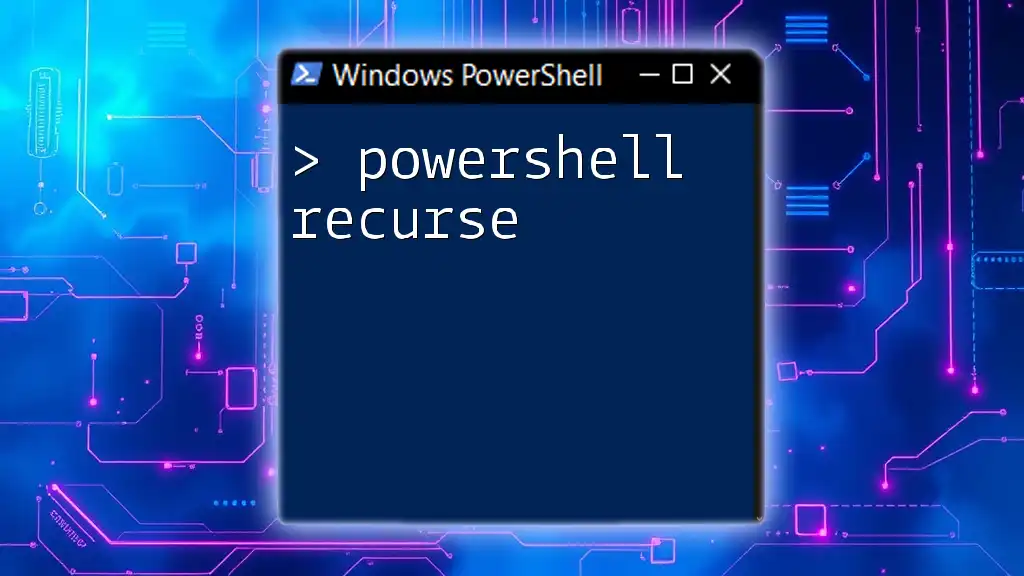
Benefits of Using Regions
Code Organization
One of the biggest advantages of using PowerShell regions is enhanced code organization. In large scripts, numerous functions and declarations can clutter the code. By grouping related functionalities together in regions, you can create a hierarchical structure that provides clarity.
Collaboration and Maintenance
In a team environment, collaboration is key to successful scripting projects. Regions enable multiple developers to work on segments of a script without stepping on each other’s toes. When the code is organized into logical parts, it’s easier for team members to pick up where others left off, facilitating ongoing maintenance.
Debugging Ease
Effective debugging is crucial for any programming task. Regions can streamline this process by allowing you to isolate code. If a certain section isn’t working as expected, you can collapse all other regions, focusing solely on the problematic code. This makes it easier to identify issues and implement fixes.
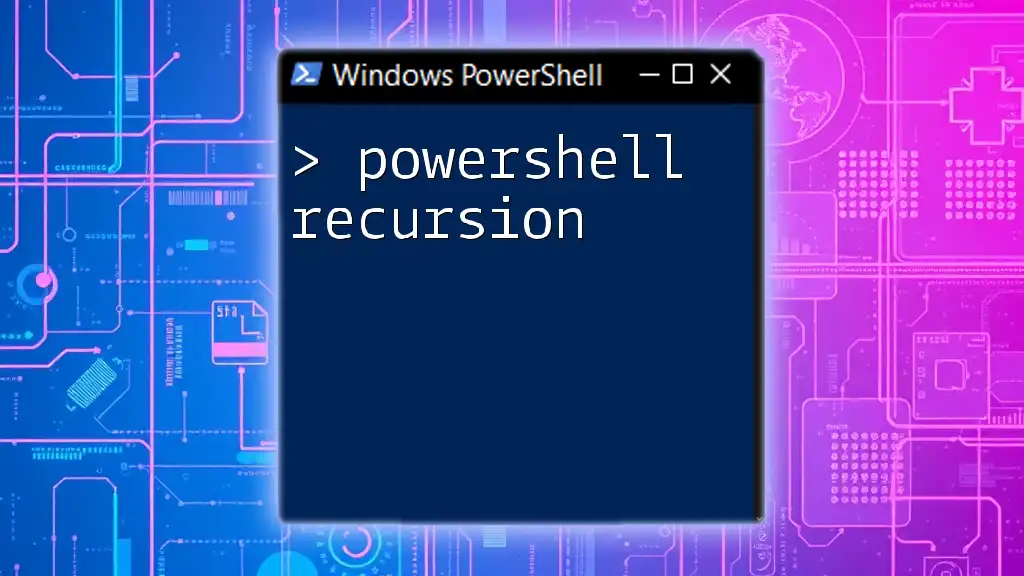
Best Practices for Using Regions
Naming Conventions
The effectiveness of regions largely depends on their names. Be clear and descriptive when naming your regions. For instance, instead of a vague name like `#region A`, opt for something like `#region User Management`. Descriptive names enhance readability and allow anyone reviewing the code to understand its purpose immediately.
Keeping Regions Concise
It’s essential to keep regions focused. Each region should encapsulate only a specific functionality or process. If a region grows too large, consider splitting it into smaller regions. A manageable size enhances clarity and usability, making it easier for yourself and others to navigate.
Documenting Regions
While regions provide structure, documentation within these segments is also vital. Adding comments to explain the purpose of each section and its functions can significantly improve the maintainability of your script. This is especially important in team settings, where different developers might work on the same codebase.
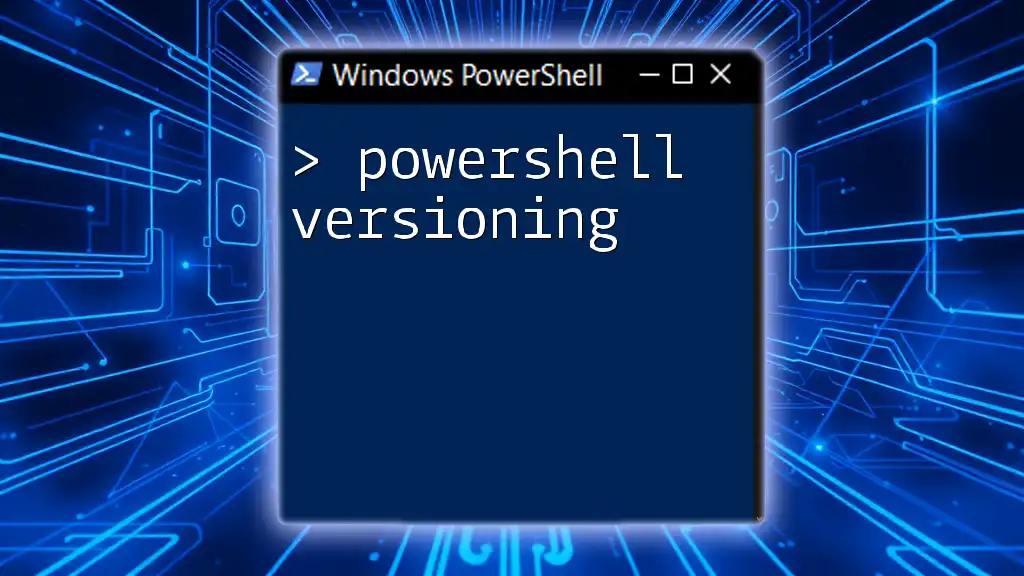
Common Mistakes to Avoid
Overusing Regions
While regions are beneficial, overusing them can lead to confusion. If every small piece of code is enclosed in its own region, the script may become more difficult to read. Use them judiciously to ensure clarity remains a priority.
Failing to Update Regions
As scripts evolve, regions should be kept relevant. Failing to update regions when functionalities change can create inconsistencies, making code harder to maintain. Regularly review and revise your region structures to reflect current practices.
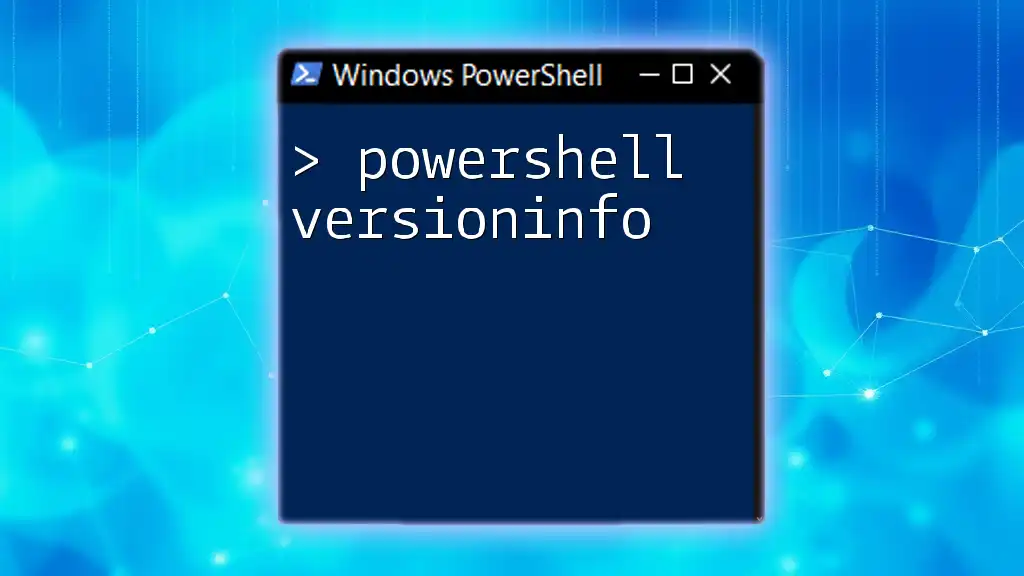
Advanced Region Techniques
Using Regions in Functions and Modules
Regions aren’t limited to the main script; they can be effectively used within functions and modules as well. This helps maintain organized and readable code at all levels. Here’s an example of how to implement regions inside a function:
function Sample-Function {
#region Initialization
# Initialization code here
#endregion
#region Processing
# Code to process data
#endregion
}
By encapsulating sections within a function, developers can focus on specific tasks without being distracted by unrelated code.
Region Shortcuts in IDEs
Many popular Integrated Development Environments (IDEs) and editors, such as Visual Studio Code and PowerShell ISE, provide shortcuts for handling regions. Familiarizing yourself with these shortcuts can significantly enhance your efficiency when working with larger scripts.
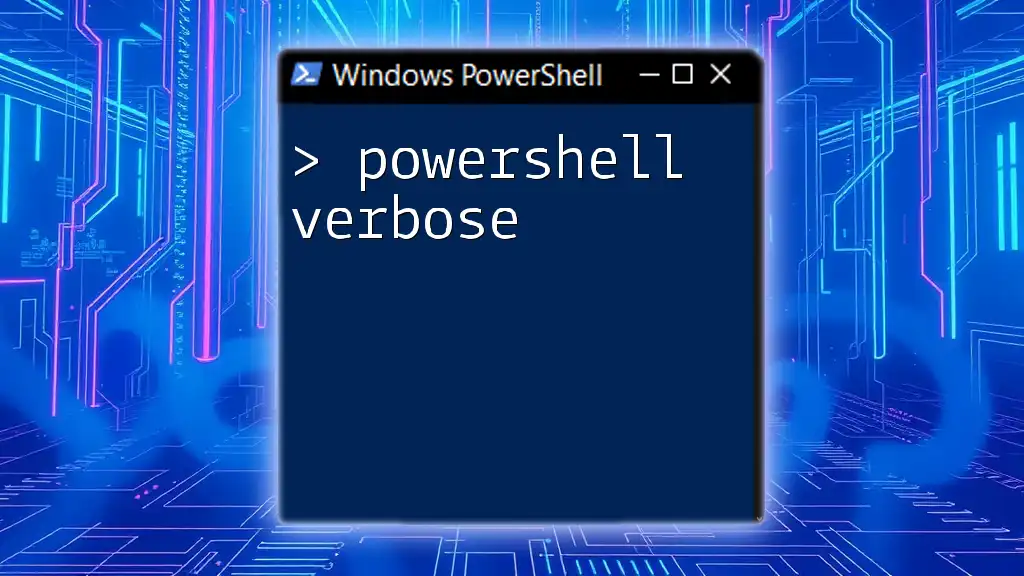
Conclusion
Incorporating PowerShell regions into your scripting practice can dramatically improve not only the readability of your code but also its maintainability. By leveraging regions, you can organize and isolate functionalities, making it easier to debug, collaborate, and troubleshoot. Adhering to best practices, such as using clear naming conventions and keeping your regions concise, will further solidify your script management skills.
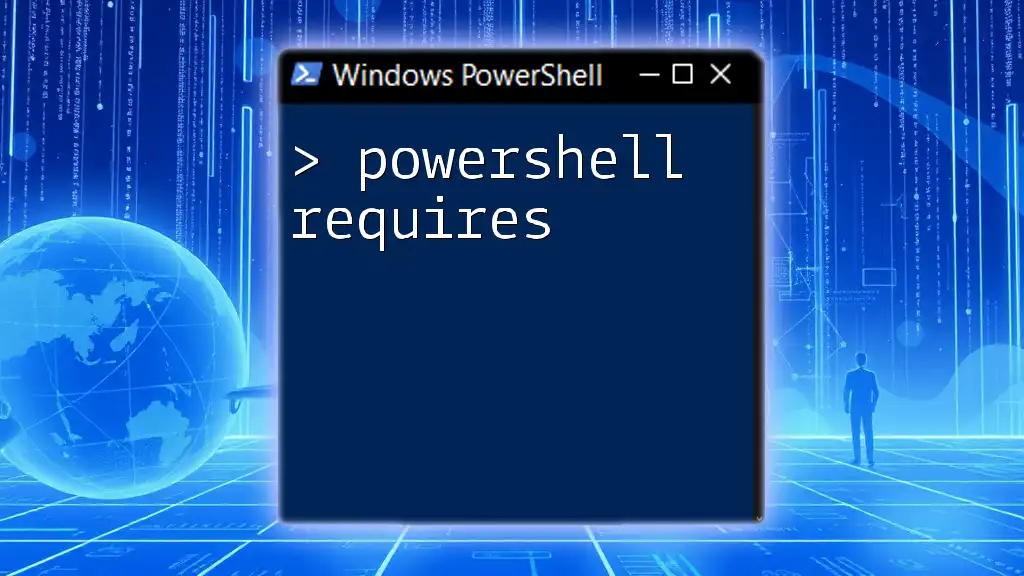
Call to Action
If you're eager to dive deeper into PowerShell and enhance your scripting abilities, consider signing up for our comprehensive course. You’ll learn valuable commands and techniques that will elevate your PowerShell expertise to the next level.
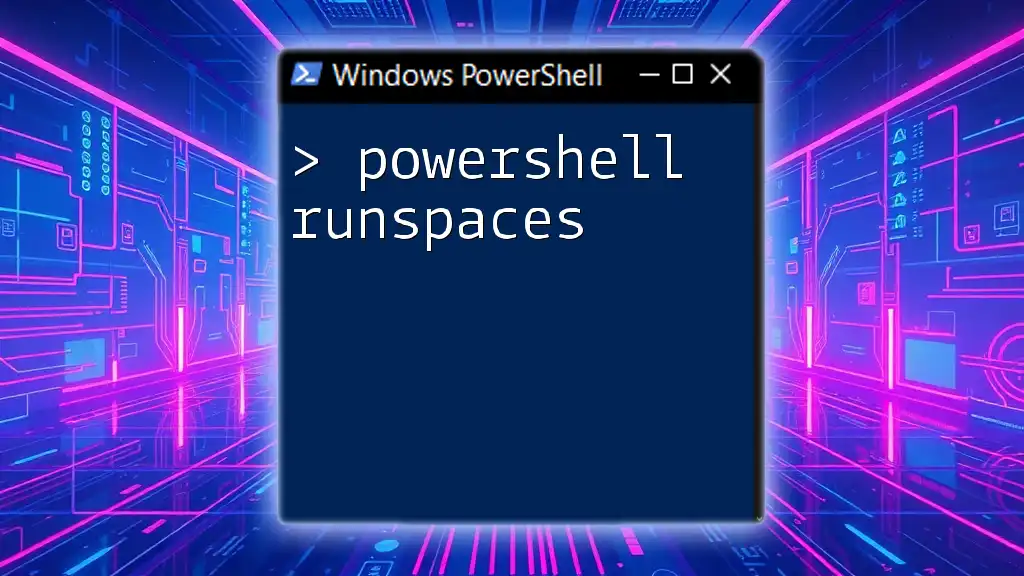
Additional Resources
For further reading, consider visiting the official PowerShell documentation and exploring community forums where you can glean additional tips and tricks for effective scripting.