The PowerShell `switch` statement allows you to evaluate multiple conditions against a single variable efficiently, executing corresponding code blocks for each matching value. Here's a simple example:
$fruit = "apple"
switch ($fruit) {
"apple" { Write-Host "You selected an apple." }
"banana" { Write-Host "You selected a banana." }
"orange" { Write-Host "You selected an orange." }
default { Write-Host "Unknown fruit." }
}
Understanding the Switch Statement
What is the Switch Statement?
The `switch` statement in PowerShell is a control structure that allows you to evaluate an expression and execute different code blocks based on the matching criteria. It streamlines decision-making in scripts, making your code not only cleaner but also easier to maintain.
Syntax of the Switch Statement
The basic syntax follows this pattern:
switch (<expression>) {
<case1> { <statement1> }
<case2> { <statement2> }
default { <default_statement> }
}
In this structure:
- `<expression>` is evaluated.
- Each `<case>` is compared with the result of the expression.
- The `default` statement runs if no cases match.
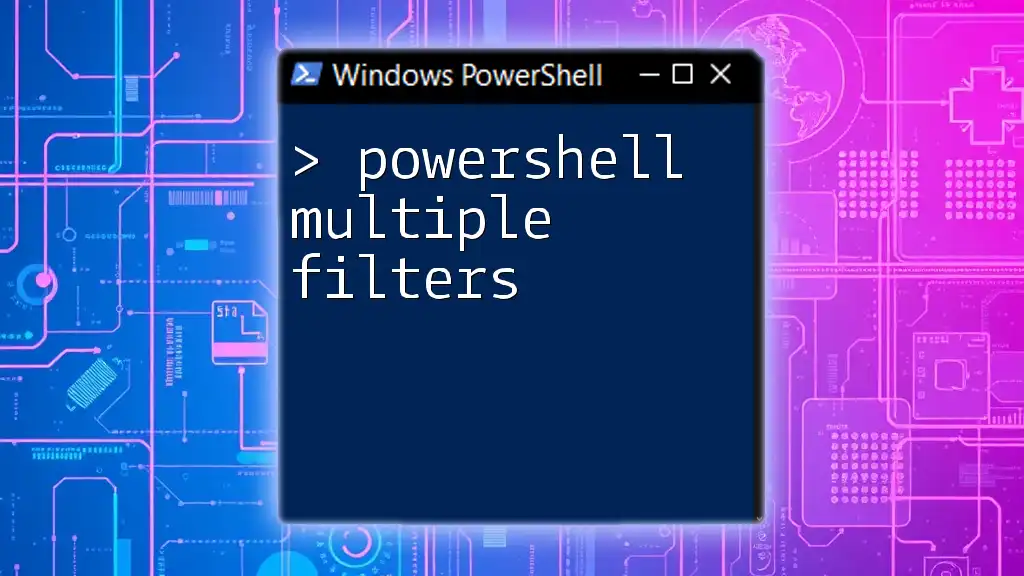
Using Switch with Multiple Values
Why Use Multiple Values?
Utilizing multiple values in a `switch` statement can significantly simplify your code. Instead of writing numerous individual `if...else` statements for each case, you can handle multiple cases succinctly. This approach enhances code readability and reduces redundancy.
Basic Example of Switch with Multiple Values
Imagine you have a variable that may contain specific strings, and you want to execute different code depending on its value. Here’s a simple illustration:
switch ($variable) {
"Value1" { "Matched Value1" }
"Value2" { "Matched Value2" }
default { "No Match" }
}
In this example, the script checks the value of `$variable`. If it contains "Value1," it outputs "Matched Value1"; if it’s "Value2," it outputs "Matched Value2". If it doesn’t match either, it defaults to "No Match."
Combining Multiple Values in a Single Case
PowerShell allows you to combine multiple values into a single case, reducing the need for repetitive code blocks. Here’s how that looks:
switch ($fruit) {
"Apple", "Orange" { "It's citrus or apple." }
"Banana" { "It's a banana." }
default { "Unknown fruit." }
}
In this case, if `$fruit` is either "Apple" or "Orange," it executes the same block of code: "It's citrus or apple." This makes the code cleaner and easier to manage when dealing with similar conditions.
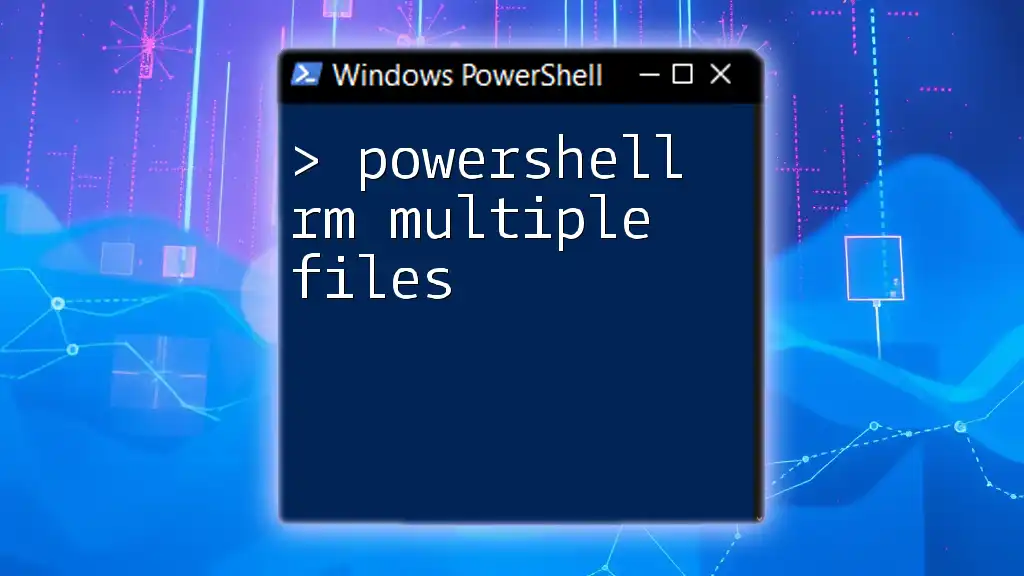
Advanced Techniques: Using Switch with Conditions
Adding Conditions to Switch Cases
The flexibility of the `switch` statement allows for conditions to be specified directly in the cases. This functionality is exceptionally powerful for handling a range of scenarios without convoluted logic. Here’s what that looks like:
switch ($input) {
{$_ -lt 10} { "Less than 10" }
{$_ -ge 10 -and $_ -lt 20} { "Between 10 and 20" }
default { "20 or more" }
}
In this example, the script will check if `$input` is less than 10 and then less than 20. The use of curly braces `{}` allows for more expressive logic while leveraging the `switch` statement to maintain readability.
Switch with Collections
Handling Arrays
One of the great features of the `switch` statement is its ability to work seamlessly with arrays. This can considerably simplify logic when evaluating multiple items against a set of conditions. Here’s an example:
$colors = @("Red", "Green", "Blue")
switch ($colors) {
"Red" { "Red color" }
"Green" { "Green color" }
"Blue" { "Blue color" }
}
The script processes each value in the `$colors` array, and based on the current value, executes the corresponding block. This functionality is excellent for working with lists where many values need to be evaluated.
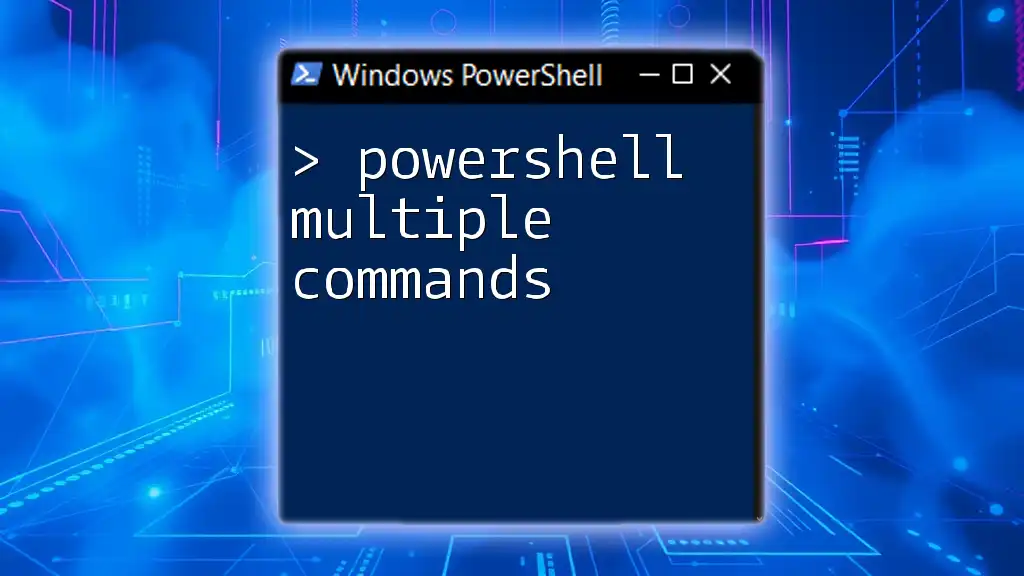
Leveraging Regular Expressions in Switch Statements
Introduction to Regular Expressions
Regular expressions (regex) offer a powerful means to match patterns in strings. When combined with the `switch` statement, they can help execute more complex matching conditions.
Using Regex in Switch
Integrating regex into your `switch` statements increases flexibility and control over your data. Here’s a handy example:
switch -Regex ($input) {
"error.*" { "This is an error log." }
"warn.*" { "This is a warning log." }
default { "Unknown log type." }
}
In this example, if `$input` matches any string starting with "error," the script recognizes it as an error log, and so on for warnings. This powerful combination lets you categorize and handle information dynamically.
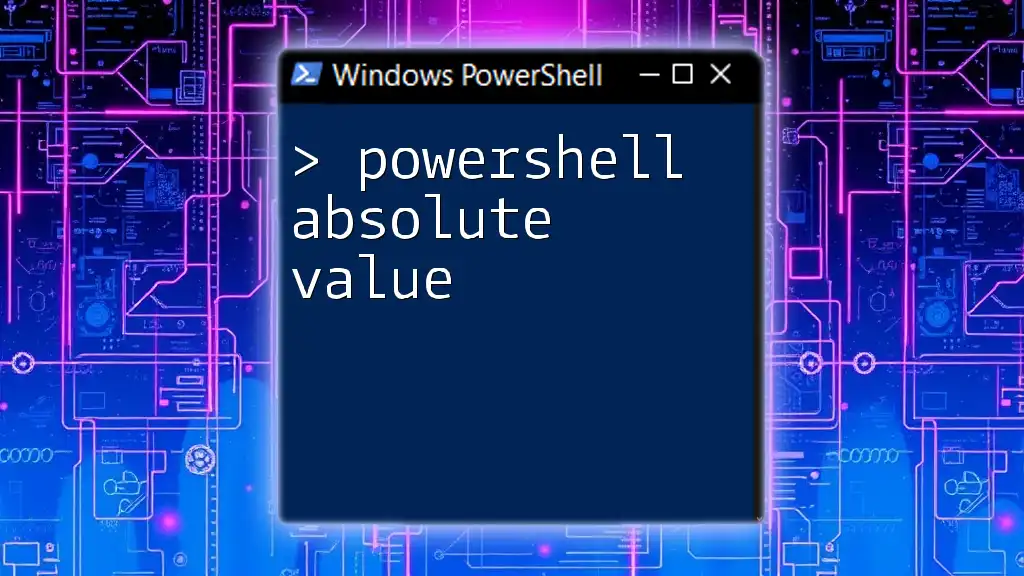
Real-World Scenarios: Practical Applications of Switch
Data Validation
A common application of the `switch` statement is in validating user input. For instance, you could use `switch` to determine if a given input fits predefined categories. This enhances user experience by providing clear feedback on their input.
Workflow Automation
In automation scripts, `switch` can be highly effective for controlling the flow of execution based on user selections or variable states. For example, in a deployment script, you might want to run different commands based on the environment:
switch ($environment) {
"Production" { Deploy-Production }
"Staging" { Deploy-Staging }
"Development" { Deploy-Development }
default { Write-Host "Unknown environment!" }
}
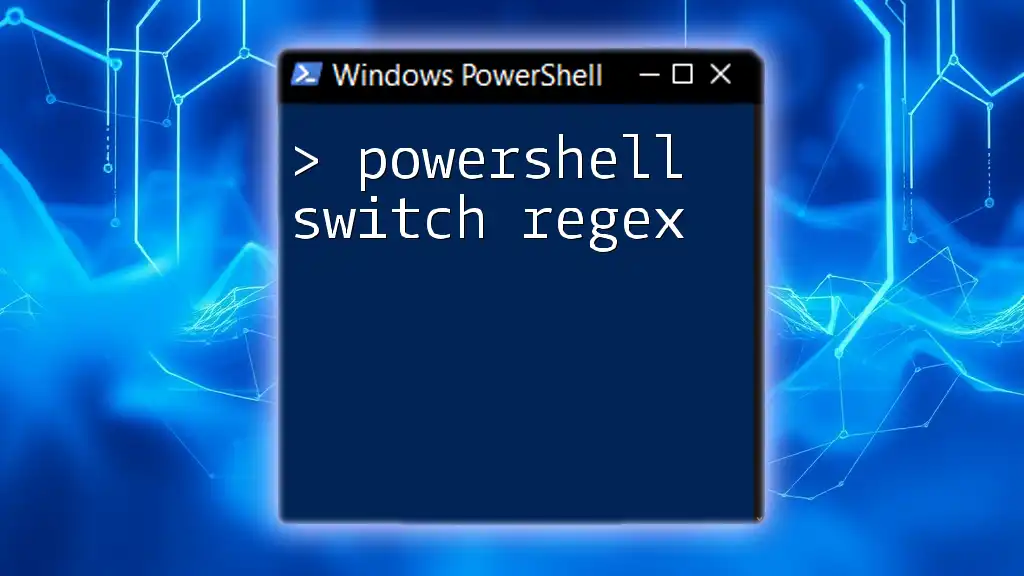
Troubleshooting Common Issues
Common Mistakes
As with any powerful tool, the `switch` statement has its pitfalls. One common mistake is neglecting to use the `default` case, which can lead to silent failures when inputs don’t match any specified cases. Always remember to handle unexpected values.
Another common issue is mismatched data types. If you compare a string with a number, the `switch` will not find a match. Ensure you're consistently using the right types to avoid confusion.
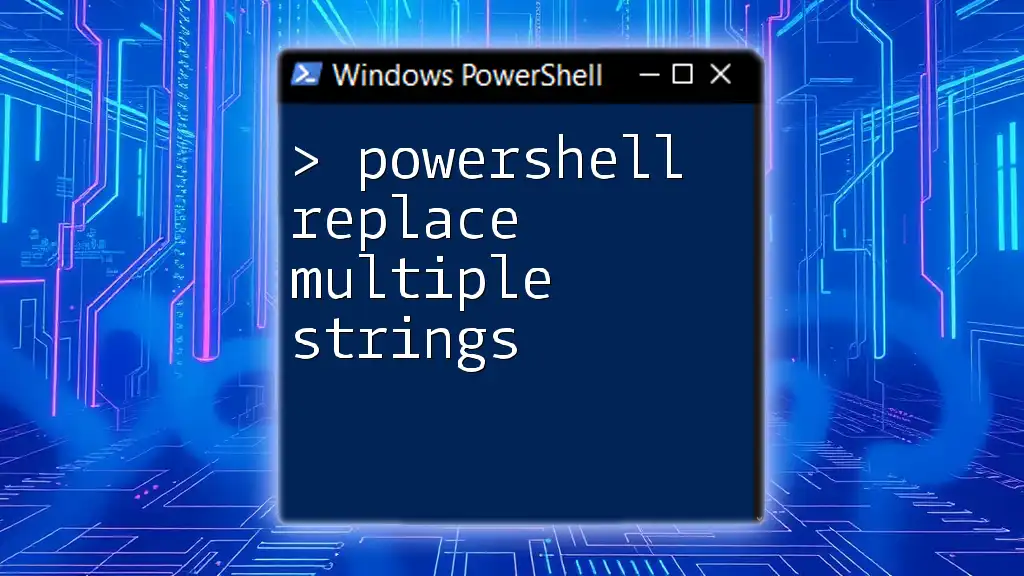
Conclusion
The `PowerShell switch` statement, especially when using multiple values, can dramatically improve the efficiency and readability of your scripts. Its versatility and ease of use allow for streamlined decision-making in both simple and complex scenarios. By practicing with the examples and patterns outlined, you'll enhance your scripting skills and harness the full potential of PowerShell.
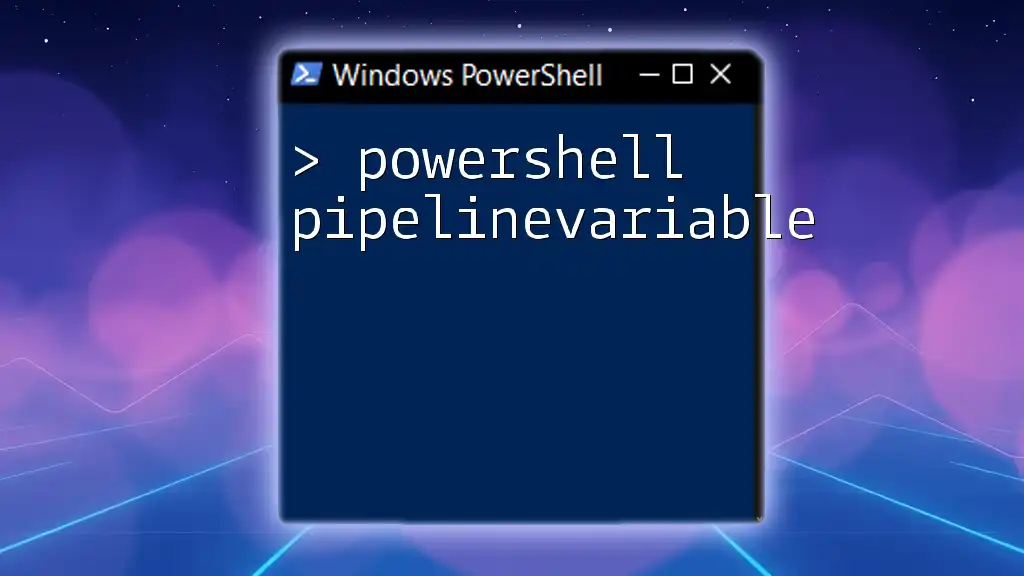
Additional Resources
For further reading, check out the official Microsoft documentation on PowerShell and the `switch` statement. There are also numerous books and tutorials available that dive deeper into practical PowerShell scripting techniques.
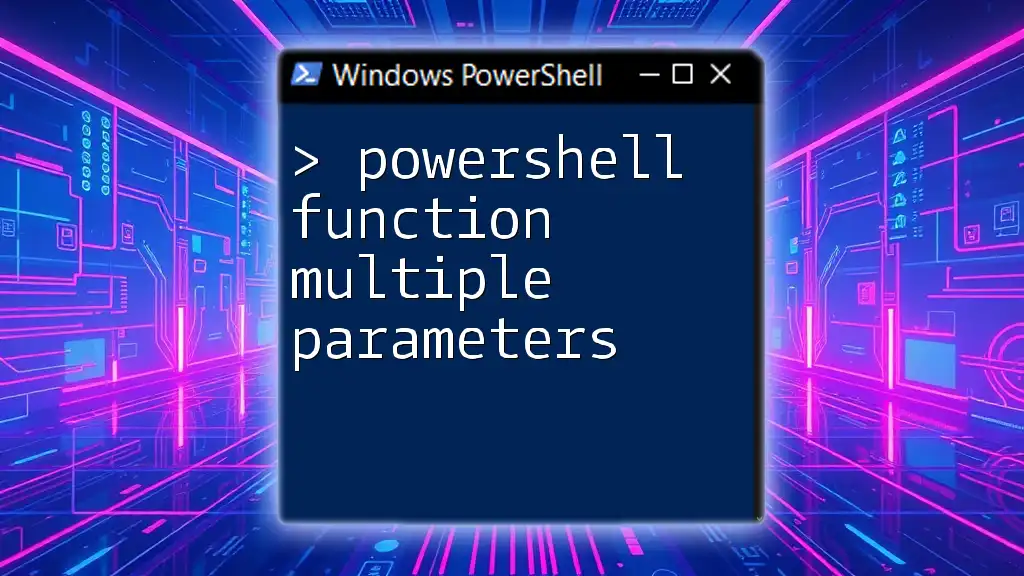
FAQs
Common Questions about PowerShell Switch
One frequent query is how to manage performance when using multiple cases in a `switch`. Performance largely remains steady as PowerShell processes comparisons efficiently. Another common concern involves using `switch` in large scripts, and the answer is that its clarity often outweighs any marginal performance changes, especially in the context of maintainability.