In PowerShell, you can evaluate multiple conditions using `if` statements combined with `-and` or `-or`, allowing you to execute specific commands based on diverse logical scenarios.
Here’s a simple code snippet demonstrating the use of multiple `if` conditions:
$a = 10
$b = 20
if ($a -lt 15 -and $b -gt 15) {
Write-Host 'Both conditions are true!'
} elseif ($a -eq 10 -or $b -eq 20) {
Write-Host 'At least one of the conditions is true!'
} else {
Write-Host 'Neither condition is met.'
}
Understanding the If Statement in PowerShell
What is an If Statement?
An if statement is a fundamental part of programming that allows you to execute a block of code based on whether a particular condition evaluates to true or false. The syntax of a basic if statement in PowerShell looks like this:
if ($condition) {
# Code to execute if the condition is true
}
Here, `$condition` represents any expression that evaluates to true or false. If true, the code within the braces is executed.
The Need for Multiple Conditions
In real-world scenarios, a single condition is often not sufficient to achieve complex decision-making. That's where the concept of multiple conditions becomes essential. For example, when managing user permissions, you might need to check both the user role and status to determine access levels. Using multiple conditions can significantly enhance the capability of your scripts, making them more adaptable to various situations.
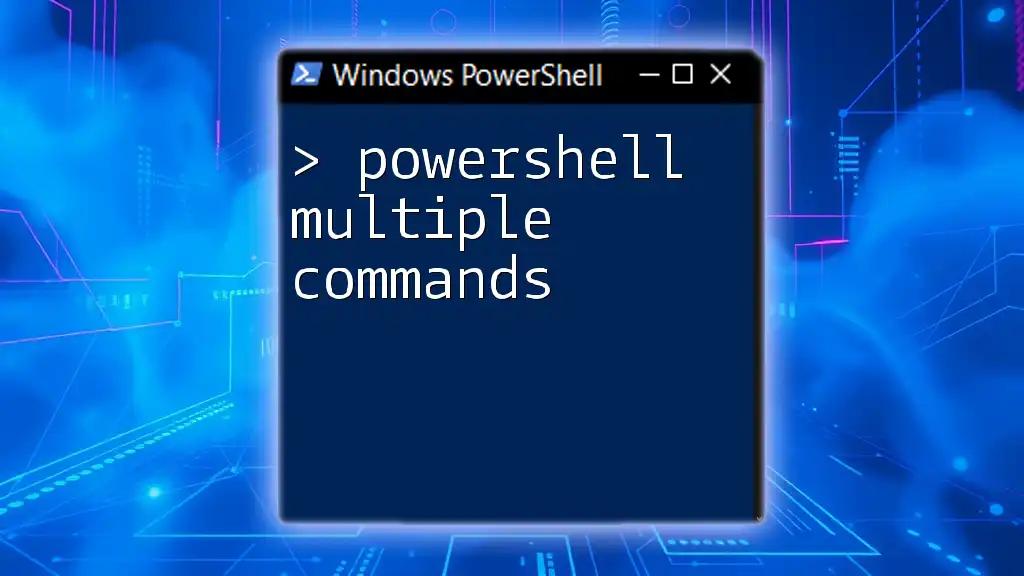
Powershell If Statement with Multiple Conditions
Using Logical Operators
The AND Operator
The AND operator (`-and`) enables you to check whether two or more conditions are true simultaneously. This can be particularly useful in scenarios where all conditions must be met for a specific action to execute.
Here's how it works:
if ($condition1 -and $condition2) {
# Code to execute if both conditions are true
}
In this case, the block of code will only run if both `$condition1` and `$condition2` result in true.
The OR Operator
In contrast, the OR operator (`-or`) allows you to execute code when at least one of the specified conditions is true. This is invaluable when you want to cover multiple acceptable conditions.
Here's a simple example:
if ($condition1 -or $condition2) {
# Code to execute if at least one condition is true
}
In this instance, as long as either `$condition1` or `$condition2` evaluates to true, the code within the braces will execute.

PowerShell If and Multiple Conditions: Combining Expressions
Nested If Statements
Nested if statements are useful when you need to make additional checks inside a primary condition. They're particularly beneficial when deeper logic is required.
For example:
if ($condition1) {
if ($condition2) {
# Code to execute if both conditions are true
}
}
In this example, the inner if statement will only be evaluated if `$condition1` is true. This structure allows for layered checks, applying additional logic as necessary.
Combining AND and OR Operators
You can also combine AND and OR operators to create more complex conditional checks. Understanding operator precedence—where `-and` has higher precedence than `-or`—is vital to ensure your conditions are interpreted correctly.
Here’s an example that illustrates this concept:
if (($condition1 -and $condition2) -or $condition3) {
# Code to execute if the combined conditions are true
}
In this snippet, the code executes if either both `$condition1` and `$condition2` are true or if `$condition3` is true. This flexibility allows you to cover a wider range of scenarios.
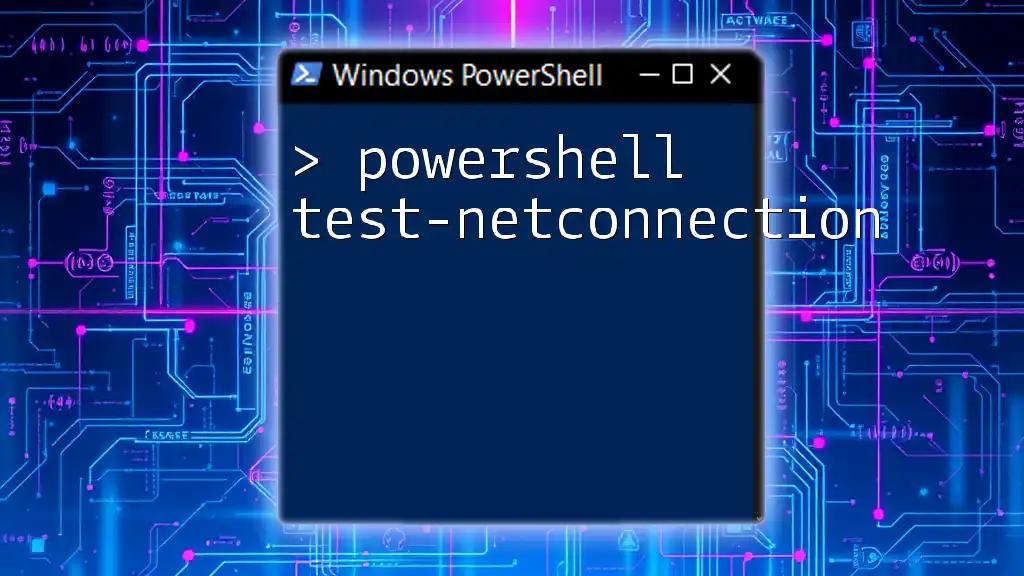
Using Switch Statements as an Alternative
Although if statements are powerful, sometimes a switch statement can be more straightforward for handling multiple conditions. A switch statement provides a clear structure when checking a variable against various values.
Here’s a typical layout for a switch statement:
switch ($variable) {
"value1" { # Do something }
"value2" { # Do something else }
default { # Do something if none match }
}
This approach can simplify your logic, especially when the conditions directly relate to the value of a single variable.
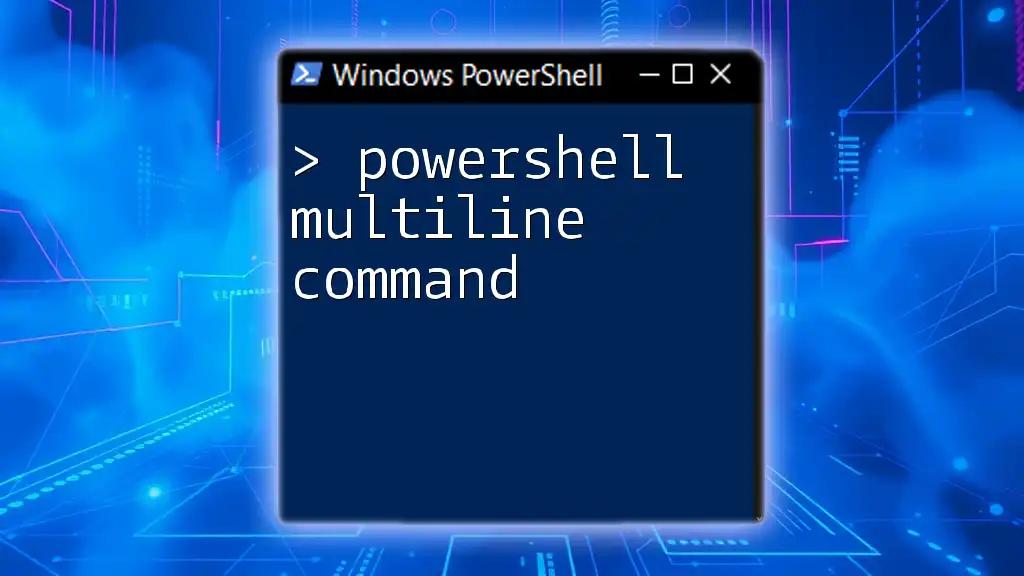
Best Practices for Using PowerShell If Statement with Multiple Conditions
Readability and Maintainability
Readability is crucial when writing scripts with multiple conditions. A clean, easy-to-follow code structure can significantly reduce debugging time and improve overall maintainability. Here are some tips:
- Comment your code: Clarifying what each condition checks can help future users (or yourself) understand your thought process.
- Use consistent formatting: Indentation and spacing make reading complicated conditions much easier.
- Break complex expressions: Instead of long, complicated conditions, consider breaking them down into simpler logic or using intermediate variables.
Debugging Multiple Conditions
When working with multiple conditions, you might encounter common errors such as syntax errors or logic errors. Ensuring that each condition evaluates correctly before running your script can save you considerable time. Here are some simple debugging strategies:
- Run components of your conditions separately to isolate issues.
- Use Write-Host or logging to output variable states and understand how your conditions are being evaluated.
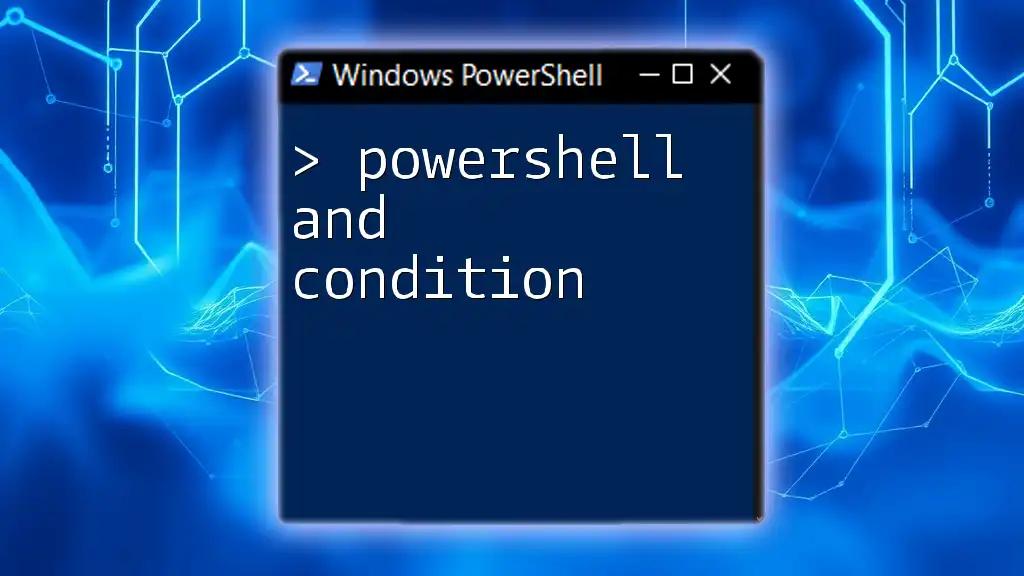
Conclusion
Understanding and effectively using PowerShell multiple if conditions can elevate your scripting capabilities, allowing for intricate decision-making and workflow automation. By utilizing logical operators, nesting, and even exploring alternatives like switch statements, you can build more dynamic and responsive scripts. Remember, the goal is to create clear, maintainable conditions that serve your specific needs.
As you practice these techniques, you’ll not only become fluent in PowerShell but also be equipped to tackle complex scripting challenges with confidence. So, keep experimenting and refining your skills!