Using multiple filters in PowerShell allows you to refine the output of commands by applying simultaneous criteria, enhancing your data retrieval efficiency. Here's a practical example demonstrating how to list services that are both running and have names that start with 'W':
Get-Service | Where-Object { $_.Status -eq 'Running' -and $_.Name -like 'W*' }
Understanding PowerShell Filters
What are Filters?
In PowerShell, filters are tools that allow users to narrow down data to a subset that meets specific criteria. Filtering is crucial for managing and processing large datasets efficiently, as it enables users to focus on the information that they need without sifting through irrelevant data.
The Basics of Cmdlets
Cmdlets are the fundamental building blocks of PowerShell operations. Each cmdlet performs a specific function, and many built-in cmdlets support filtering capabilities. For example, `Get-Command`, `Get-Process`, and `Get-ChildItem` allow users to retrieve data while applying filters to refine the output.

How Filtering Works in PowerShell
Single Vs. Multiple Filters
PowerShell provides the flexibility of using both single and multiple filters. Single filters are straightforward, allowing for basic queries. However, the true power of PowerShell emerges when using multiple filters. This capability allows users to construct more complex queries, enhancing the specificity of the data retrieved.
Basic Syntax for Filtering
The primary way to apply filters in PowerShell is through the `Where-Object` cmdlet. The syntax is basic yet powerful, making it easy to filter objects based on their properties. For instance:
Get-Process | Where-Object { $_.CPU -gt 1 }
In this example, only processes using more than 1 CPU will be displayed.
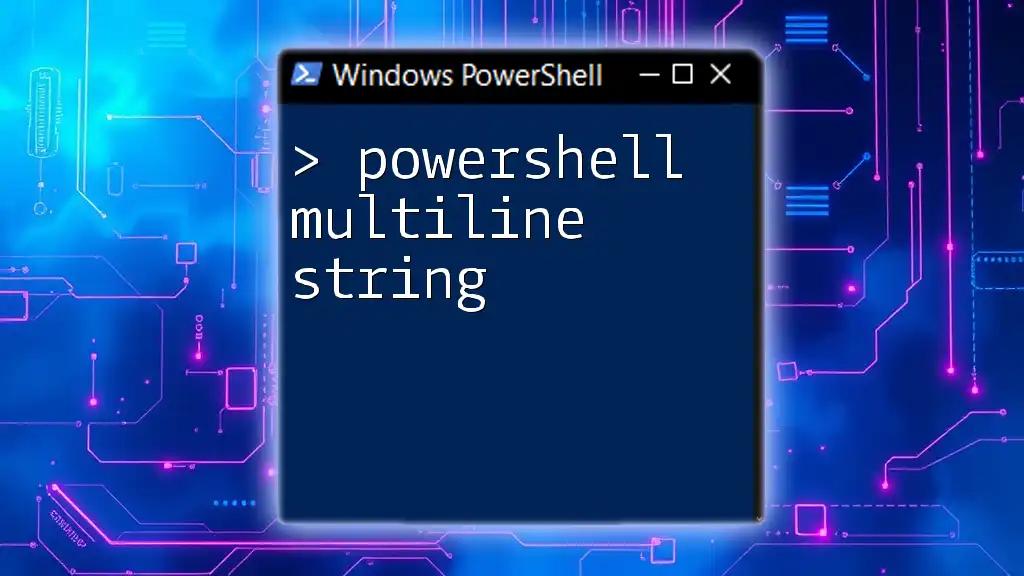
Applying Multiple Filters in PowerShell
Using Logical Operators
To filter data based on multiple conditions, PowerShell allows for the use of logical operators:
-
AND Operator: Use this operator to combine conditions where both must be true.
For example, to filter processes based on their name and CPU usage, you can use:
Get-Process | Where-Object { $_.Name -eq "notepad" -and $_.CPU -gt 1 }
-
OR Operator: This operator is useful when you want to retrieve items that meet at least one of several conditions.
For example, to get services that are either running or stopped:
Get-Service | Where-Object { $_.Status -eq "Running" -or $_.Status -eq "Stopped" }
-
NOT Operator: Use this operator to exclude objects that meet a certain condition.
An example would be finding files that are not hidden:
Get-ChildItem | Where-Object { -Not $_.Attributes -match "Hidden" }
Combining Filters with `Where-Object`
You can also combine conditions within a single `Where-Object` clause for more depth. For example, filtering a list of files by their extension and size can be done as follows:
Get-ChildItem "C:\MyFiles" | Where-Object { $_.Extension -eq ".txt" -and $_.Length -lt 1MB }
This command retrieves all `.txt` files that are smaller than 1MB.
Using the `FilterHashtable`
Another advanced technique for filtering is using a FilterHashtable, which is particularly useful for cmdlets like `Get-EventLog`. This approach simplifies the syntax when you have multiple criteria to filter by.
For example, to retrieve logs for a specific event ID and entry type:
Get-EventLog -LogName Application -FilterHashtable @{EventID=1000; EntryType=Error}
This method enhances readability and structure in your code.
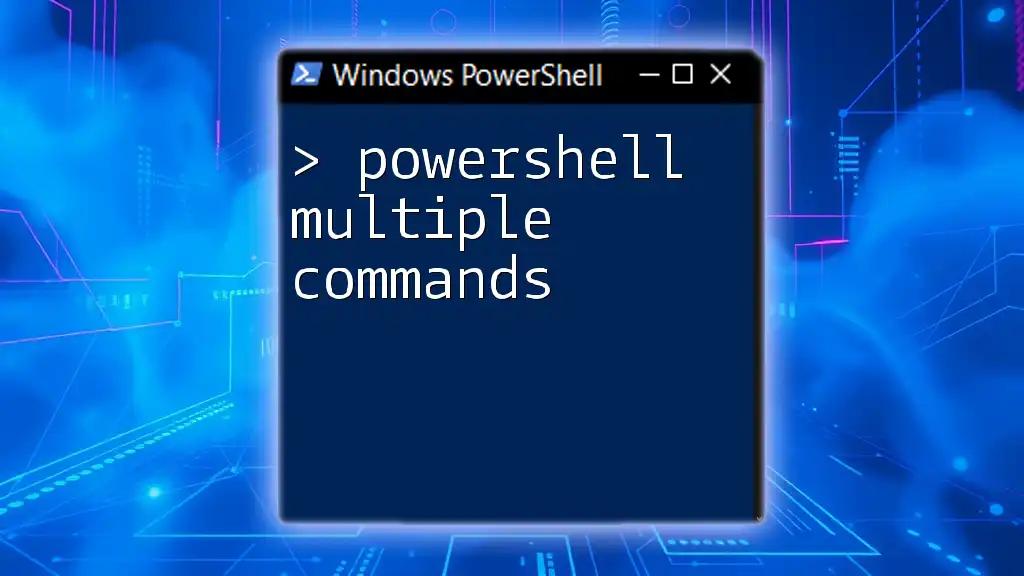
Advanced Techniques for Multiple Filters
Using Regular Expressions
Regular expressions (regex) can add another layer of power to your filtering capabilities in PowerShell. They allow for pattern matching in strings. For example, to filter event logs where the source matches a disk-related term, you can execute:
Get-EventLog -LogName System | Where-Object { $_.Source -match "disk" }
This allows you to match multiple sources without explicitly listing them.
Chaining Cmdlets
One of the strengths of PowerShell is its ability to chain cmdlets together, allowing for data manipulation to occur through a pipeline that incorporates filtering.
For instance, to retrieve processes that exceed a certain CPU threshold and then sort the results based on CPU usage in descending order, you can combine `Get-Process` and `Sort-Object` like this:
Get-Process | Where-Object { $_.CPU -gt 1 } | Sort-Object CPU -Descending
This example demonstrates how to filter and sort data seamlessly.

Performance Considerations
Efficiency of Filtering
While filters are incredibly useful, it’s crucial to understand how they can affect performance, especially with large datasets. Complex filtering criteria can lead to longer execution times, so it is wise to optimize your commands wherever possible.
When to Use Filters
Filters should be employed judiciously. If you find yourself working with large datasets, ask whether filtering will save time and effort. For smaller datasets, applying filters may be unnecessary and slow down processing.
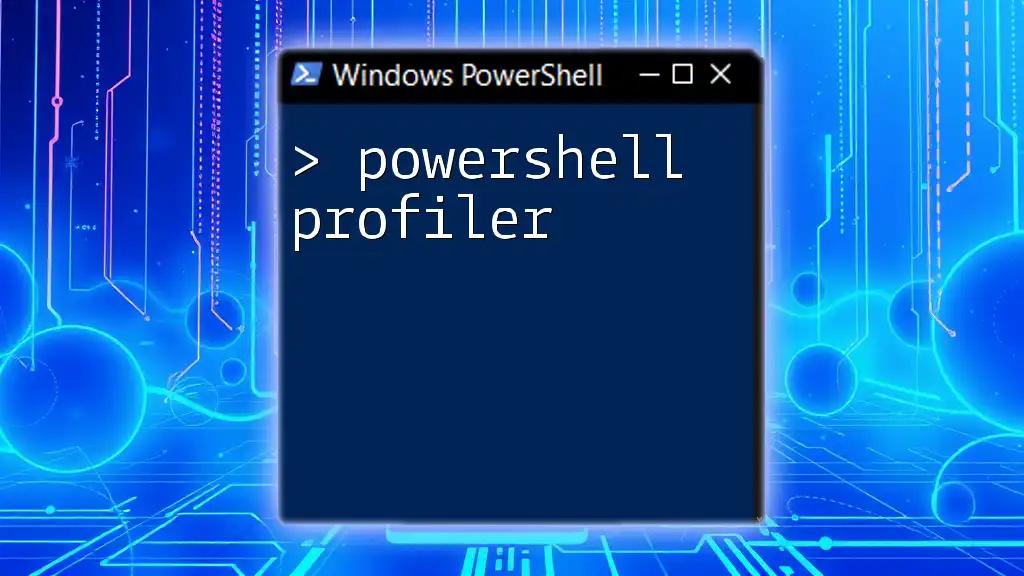
Troubleshooting Common Issues with Filters
Common Errors and Solutions
When using multiple filters in PowerShell, it’s easy to encounter errors such as misuse of logical operators or syntax errors. Understanding these errors makes it easier to fix them. For example, incorrect placement of parentheses can lead to unexpected results.
Debugging Filtering Issues
Using debugging tools can reveal underlying issues within your commands. Using `Write-Debug` and `Write-Host` can help you inspect the flow of data as filters are applied. An example might look like this:
$debugPreference = "Continue"
Get-Process | Where-Object { $_.CPU -gt 1 } | Write-Debug
This approach can pinpoint where things may be going awry.
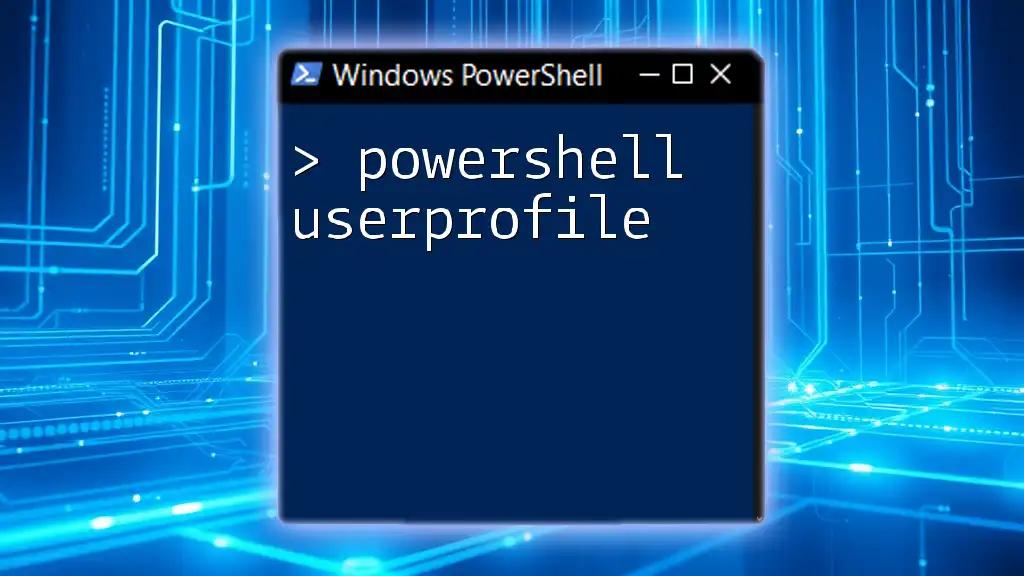
Conclusion
By mastering the use of PowerShell multiple filters, you enhance your capability to manage and process data efficiently. Filters allow you to navigate large datasets effectively, ensuring that you can extract the most relevant information precisely when you need it.
As you delve into PowerShell commands, remember to experiment with combined filters, logical operators, and advanced techniques to maximize your productivity in managing tasks.
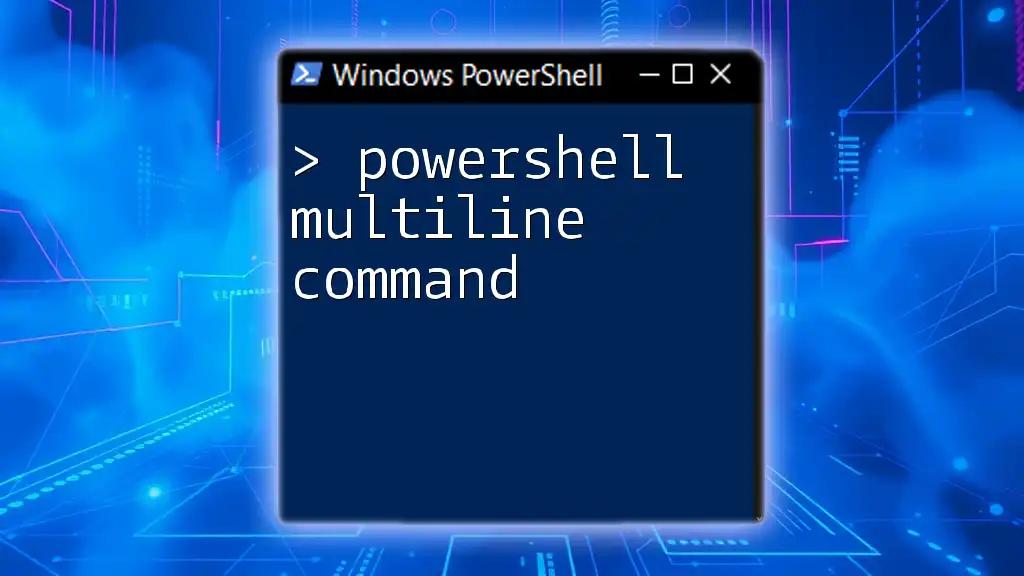
Additional Resources
For further exploration, consider checking the official PowerShell documentation, community forums, and tutorial websites to deepen your understanding and gain insights from experienced PowerShell users.