The PowerShell exclude filter allows you to specify criteria to exclude certain items from a collection, enhancing the efficiency of your script by focusing on the relevant data.
Here’s a simple example using the `Where-Object` cmdlet to exclude files with a `.txt` extension from a directory listing:
Get-ChildItem | Where-Object { $_.Extension -ne '.txt' }
Understanding Filtering in PowerShell
What is Filtering?
Filtering in PowerShell refers to the process of selecting specific data based on certain criteria. This enables users to manipulate and analyze data more effectively, focusing only on what is relevant. Filtering can be applied in a myriad of scenarios, from retrieving specific system information to analyzing logs and processes.
Importance of Exclusion Filters
Exclusion filters are particularly valuable because they allow you to remove unwanted data from your results. Instead of cluttering your output with irrelevant entries, you can focus on what truly matters. This can be especially useful when dealing with large datasets, where performance and readability are crucial.
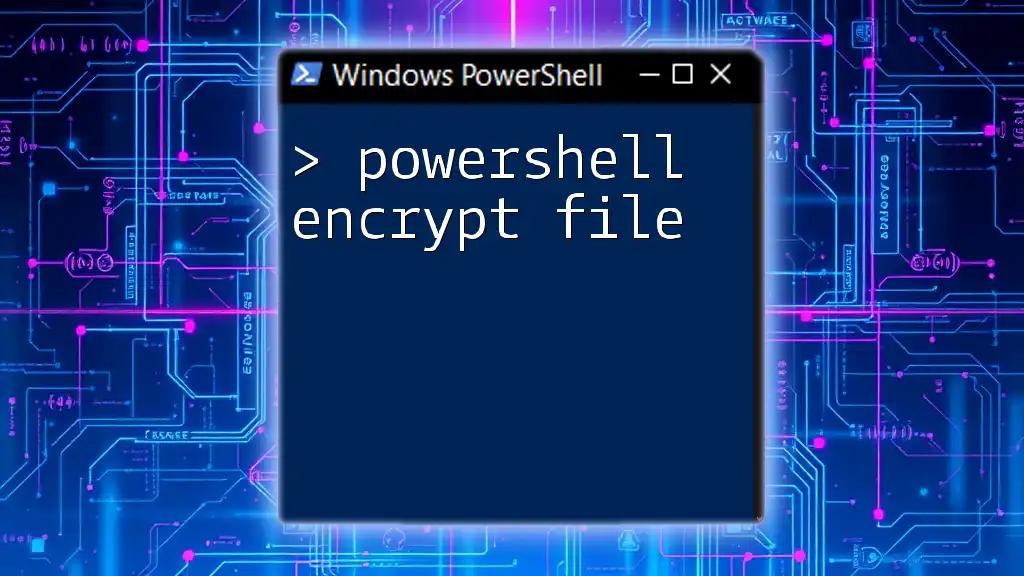
Using the Exclude Filter
Syntax of the Exclude Filter
The basic syntax for using the exclude filter in PowerShell typically involves the `Where-Object` cmdlet along with a conditional statement. A common structure looks like this:
Get-Command | Where-Object { $_.Name -notlike "*Exclude*" }
In this example:
- `Get-Command` retrieves all available commands.
- `Where-Object` filters those commands based on the name criteria defined within the parentheses.
- The `-notlike` operator is employed to exclude any command names containing "Exclude".
How to Apply Exclude Filters
Excluding Specific Items
Excluding specific items can be as simple as using the `-Exclude` parameter when fetching items from a directory. Here's how you can utilize this feature:
Get-ChildItem -Path "C:\MyFolder" -Exclude "*.txt"
In this case, the command will list all files in `C:\MyFolder` except for those with the `.txt` extension. This allows you to quickly focus on all other file types without manually sifting through each entry.
Using Exclude with Pipeline Commands
Pipelines enhance the power of PowerShell commands by allowing you to chain multiple commands together. For instance, if you want to filter out processes that start with "Search," you can use:
Get-Process | Where-Object { $_.Name -notlike "Search*" }
Here, `Get-Process` retrieves a list of all currently running processes, and the `Where-Object` cmdlet filters out any processes whose names begin with "Search." This is a practical application of the exclude filter in a dynamic real-time environment.
Advanced Techniques with Exclude Filter
Combining Exclude Filters with Other Conditions
To make your filtering even more efficient, you can combine exclude filters with other logical conditions. For instance, if you want to get all running services but exclude a specific one named "W32Time," you can write:
Get-Service | Where-Object { $_.Status -eq 'Running' -and $_.Name -notlike 'W32Time' }
In this command:
- The status of the service is checked to ensure it's running (`-eq 'Running'`).
- The `-and` logical operator helps combine conditions.
- The `-notlike` operator excludes "W32Time" from the results.
This method is beneficial for creating targeted reports where certain services need to be excluded for clarity.
Using Exclude Filters in Scripting
One powerful way to leverage the exclude filter is in function creation. Writing modular scripts allows for reusability and more concise code. For example, you can create a function to get custom files while excluding unwanted extensions:
function Get-CustomFiles {
param (
[string]$path,
[string[]]$excludeExtensions
)
Get-ChildItem -Path $path | Where-Object { ($_ | Select-Object -ExpandProperty Extension) -notin $excludeExtensions }
}
In this scenario:
- `Get-CustomFiles` takes a path and an array of file extensions to exclude.
- The command retrieves items from the specified path and identifies the file extensions, excluding any that are listed in the `$excludeExtensions` parameter.
This not only enhances the flexibility of your scripts but also promotes clarity and efficient coding practices.

Performance Considerations
Utilizing exclude filters can significantly improve script performance, especially when iterating over large datasets. By narrowing down to only relevant data, you reduce the processing load and increase the speed of command execution. However, it's important to consider scenarios where using exclude filters may not be viable, such as in cases with extremely large databases, where filtering may lead to performance degradation.
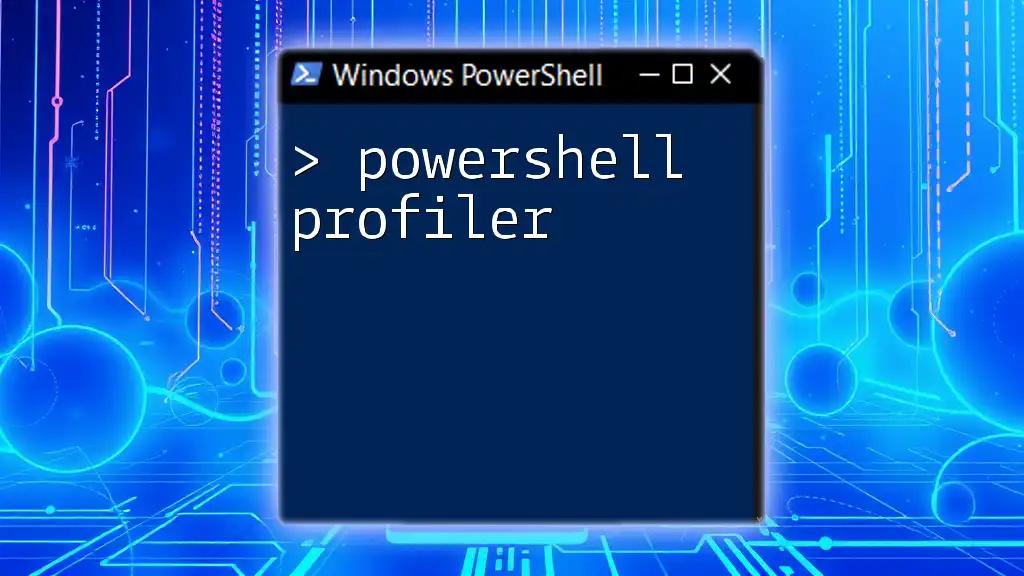
Best Practices for Using Exclude Filters
To make the most of your exclude filters, consider the following best practices:
- Be Specific: Use precise criteria when excluding data to avoid unintentionally leaving out important information.
- Test Incrementally: When working with complex commands, test them incrementally to ensure each part works correctly before combining them.
- Readability Matters: Maintain readability in your scripts; consider adding comments to clarify the purpose of each filter.
Common Pitfalls and How to Avoid Them
A few common pitfalls include:
- Over-Excluding: Excluding too many items may lead to missing crucial data. Always double-check your criteria.
- Ignoring Performance Implications: While excluding filters can enhance performance, be cautious with overly complex conditions, as they may have the opposite effect.
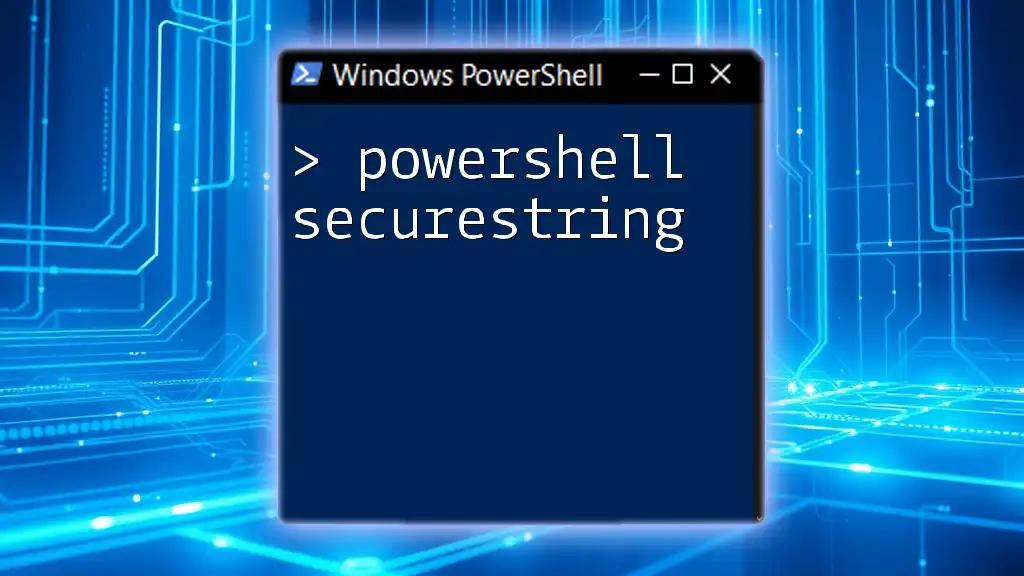
Conclusion
PowerShell's exclude filter is a versatile tool that enhances data management efficiency. By mastering the use of exclude filters, you can streamline your operations, focusing on relevant data and improving overall performance. Practice with the examples provided above, and consider exploring further learning resources to deepen your understanding and application of PowerShell filters in your projects.