In PowerShell, the `Change` command typically refers to altering the properties of files, folders, or system settings, allowing users to efficiently manage their environment with simple commands. Here's an example of changing the file attributes:
Set-ItemProperty -Path 'C:\example\file.txt' -Name Attributes -Value 'ReadOnly'
What Can You Change with PowerShell?
File and Directory Manipulation
PowerShell is a powerful tool for managing files and directories. You can change file attributes, modify file contents, and even rearrange directory structures with just a few commands. One of the most common commands for changing the contents of a file is `Set-Content`. This command allows you to overwrite the contents of a file or create a new file with specified data.
For example, consider you want to change the contents of a text file:
Set-Content -Path "C:\example.txt" -Value "New Content"
This command will replace whatever is currently in `example.txt` with "New Content." It’s essential to use this command cautiously, as it does not prompt for confirmation before overwriting data.
Environment Variables
Environment variables are a crucial part of any system environment. They store important configuration information accessible throughout the system. You can change these variables using the `Set-Item` command, which modifies the values of items within the provider path.
For instance, if you need to change an environment variable named `MY_ENV_VAR`, the command would look like this:
Set-Item -Path Env:MY_ENV_VAR -Value "NewValue"
This command updates the `MY_ENV_VAR` variable to a new value. Always remember that changes to environment variables can affect all applications and services on your machine, so exercise care when modifying them.
Services and Processes
In Windows, services are critical components that run in the background to facilitate various functionalities. PowerShell allows you to change settings related to these services using the `Set-Service` command. This can be particularly helpful if you want to modify the startup type of a service or stop/start services on your system.
For example, to change the startup type of the Print Spooler service to 'Automatic', you can use:
Set-Service -Name "Spooler" -StartupType "Automatic"
This command will ensure the Print Spooler service starts automatically with Windows, which can be vital for maintaining printer availability.
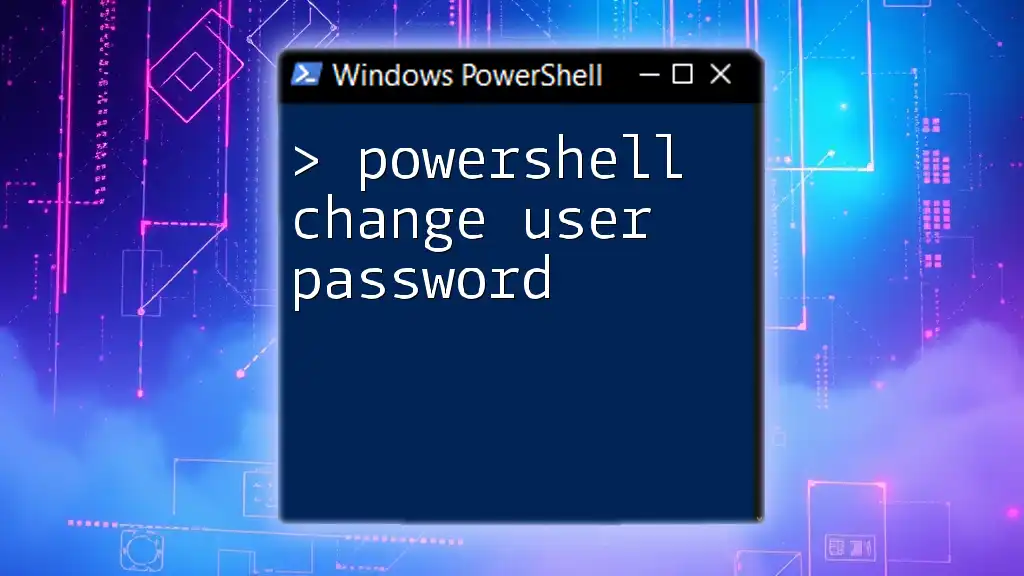
Key PowerShell Commands for Changing Properties
Get-Command
When you're unsure about which commands are available for changing properties, using `Get-Command` can be invaluable. You can filter commands based on nouns to discover commands associated with changes:
Get-Command -Noun *Change*
This command will list all relevant commands, giving you insight into the options you have at your disposal for making changes.
Set-Content
As mentioned earlier, `Set-Content` is an essential command for updating file content. You can add text to a file or overwrite it entirely, making it very versatile. For instance, if you want to append data rather than replace it, you could use the `Add-Content` command, but for direct replacement, `Set-Content` is the key.
Set-Item
The `Set-Item` command is not limited to environment variables. It can also modify other attributes in various providers. Its versatility allows it to function both with file system paths and registry paths, making it an essential command in your PowerShell toolkit.
Set-Service
Understanding how to use `Set-Service` to manipulate Windows services is vital for anyone managing systems. Besides changing the startup type, you can also stop or start services on demand. For example, to stop the Print Spooler service:
Stop-Service -Name "Spooler"
You can then start it again with:
Start-Service -Name "Spooler"
These commands give you control over when and how services are running, which is particularly useful during troubleshooting or maintenance tasks.
Other Useful Commands
Besides the commands mentioned, there are additional PowerShell commands that can help you manage changes effectively:
- Invoke-Command: This command allows you to execute commands on remote systems, broadening the scope of changes you can implement across networks.
- Update-Help: Keeping your PowerShell help files up to date ensures you have the latest information at your fingertips for all commands, including those that perform changes.
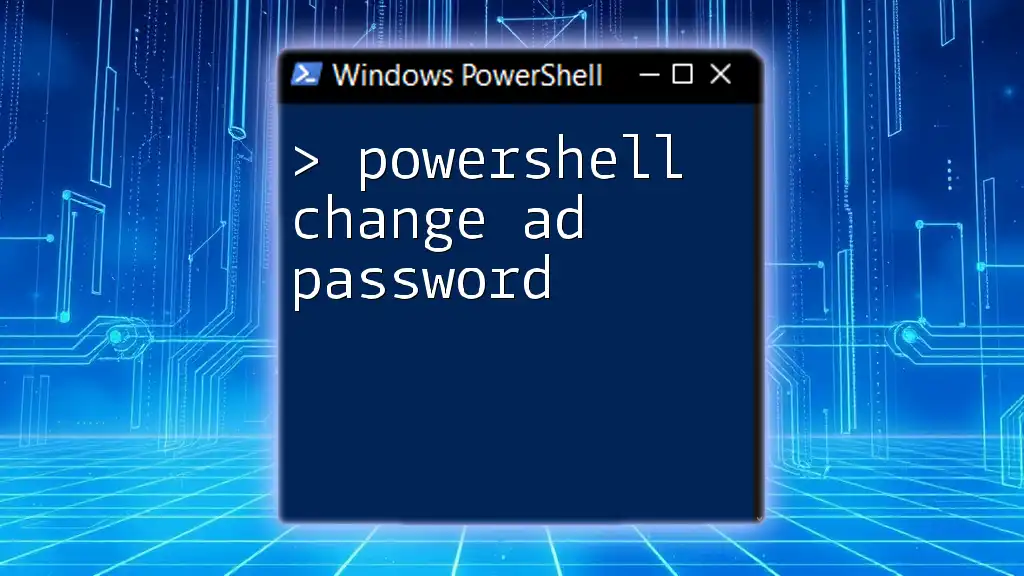
Best Practices When Using PowerShell for Changes
While powerful, PowerShell commands must be executed with caution. Here are some critical best practices to keep in mind:
-
Always Run as Administrator: Many commands, particularly those that affect system settings or services, require elevated permissions. Running PowerShell as an administrator ensures you can execute these commands successfully.
-
Test Changes Before Applying: Use the `-WhatIf` parameter to preview changes that a command will make. For instance, before changing a service's status, you can assess what will happen with:
Set-Service -Name "Spooler" -Status "Running" -WhatIf
This way, you can verify the changes without making any actual updates until you are sure.
- Be Mindful of Parameters: Understanding each command's parameters is crucial. A small mistake in an input can lead to unintended changes that may affect system stability.
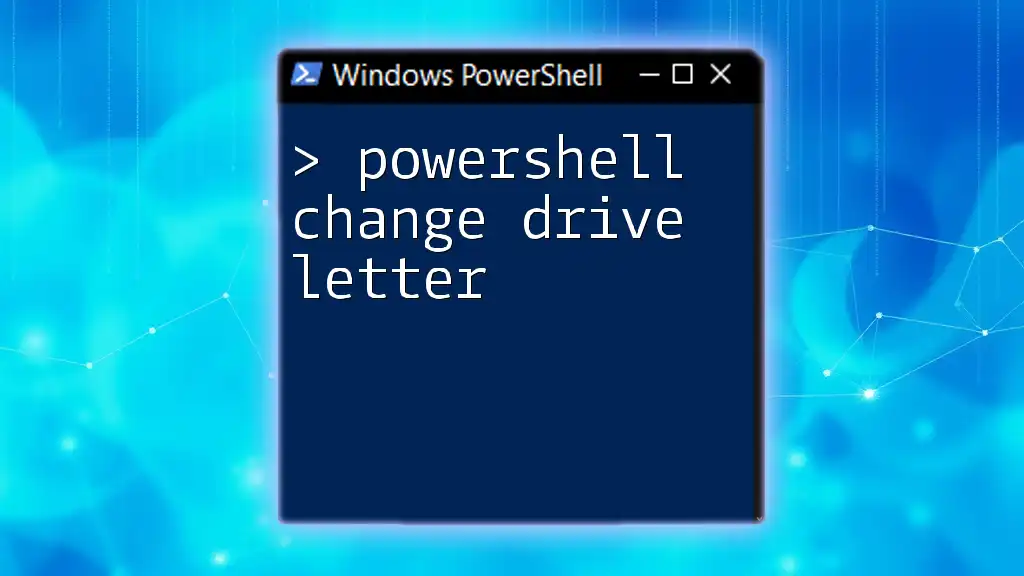
Troubleshooting Common Issues
-
Permissions Problems: If a command does not execute, the first thing to check is whether you have the necessary permissions. You can use the `Get-Acl` command to review permissions on files or services.
-
Failed Commands: If a command fails, carefully review the error message. PowerShell often provides useful troubleshooting information, which can guide you on what went wrong and how to fix it.
-
Log Files for Tracking Changes: Keeping track of changes made via PowerShell can be challenging. Always log your commands and their outputs, especially when making numerous changes. You can redirect output to a log file using:
Start-Transcript -Path "C:\PowerShell\logfile.txt"
This command starts logging all commands and output until you stop it with `Stop-Transcript`.
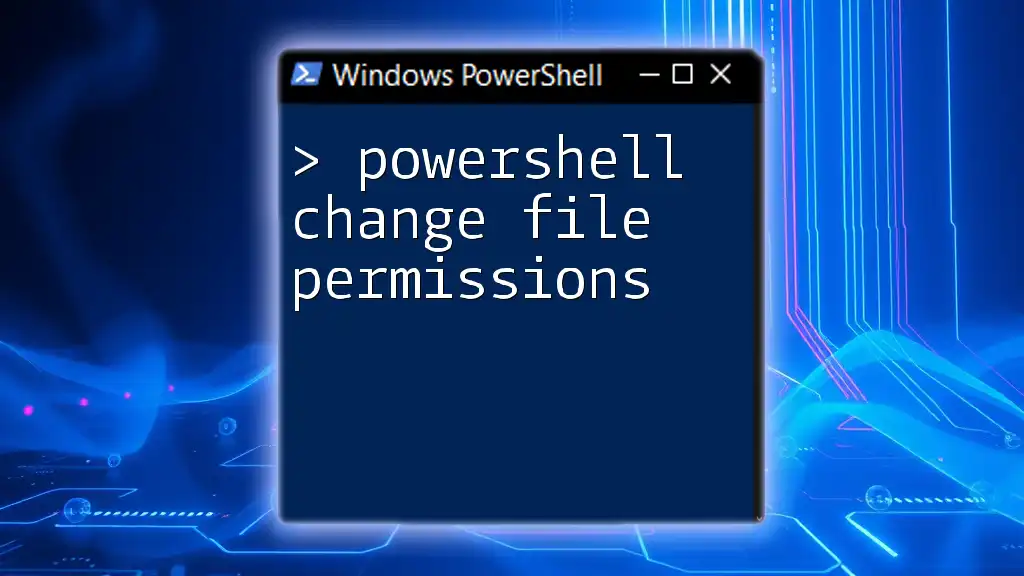
Conclusion
Mastering PowerShell change commands is essential for optimizing your workflow and efficiently managing system settings. From updating environment variables to controlling services, these commands unlock tremendous potential for automation and system administration. As you practice these skills, you'll find numerous ways to streamline your processes and enhance your productivity.
Additional Resources
For more in-depth knowledge, refer to official Microsoft PowerShell documentation and consider enrolling in advanced PowerShell courses to expand your understanding.
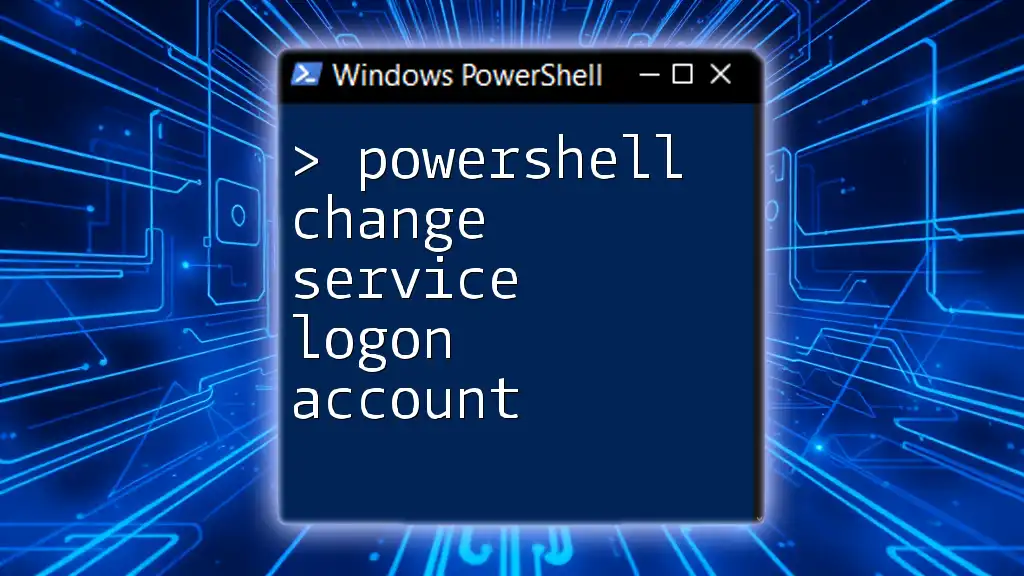
Call to Action
Join our growing community of PowerShell enthusiasts! Engage in discussions, share your knowledge, and keep learning with others who share your passion for this powerful tool. Sign up for our newsletter for daily tips and tricks to enhance your PowerShell proficiency.